Merge branch 'develop' into feature/messagepack
Showing
此差异已折叠。
doc/examples/emplace.cpp
0 → 100644
doc/examples/emplace.link
0 → 100644
doc/examples/emplace.output
0 → 100644
doc/examples/emplace_back.cpp
0 → 100644
doc/examples/emplace_back.link
0 → 100644
doc/examples/emplace_back.output
0 → 100644
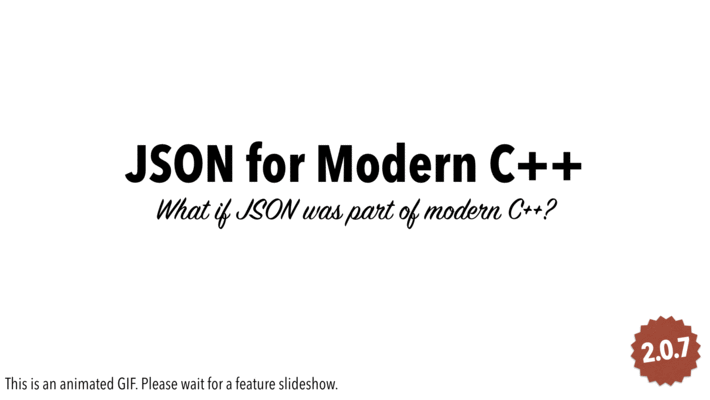
| W: | H:
| W: | H:
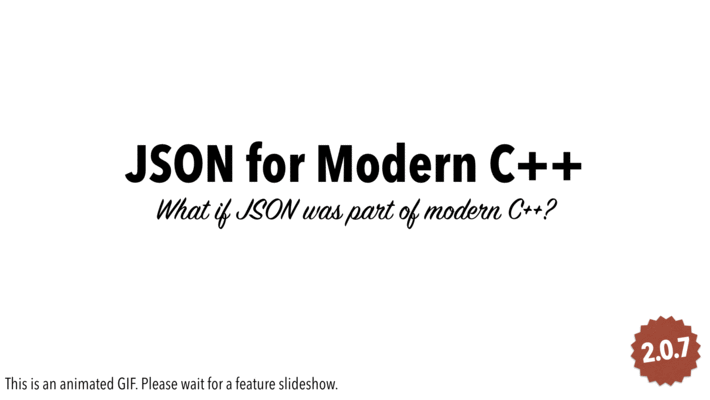
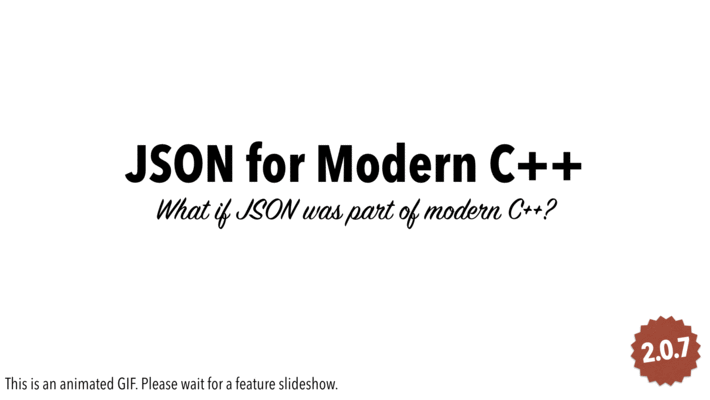