1.3.1 release.
Support Cygwin and Mingw. Dynamically allocate timers when needed (multithreading releated behaviors kept as before). Sharply reduced the memory occupation of timers. Expand the range of timer ID from [0, 256) to [0, 65536). Add new macro ASCS_ALIGNED_TIMER to align timers. Realign member variables to save a few memory. Amend doc. Supplement English docs. Avoid chinese file names. Simplified config.mk Make demos easier to use. Optimize file_server and file_client. Supplement _LARGEFILE_SOURCE definition for file_server and file_client.
Showing
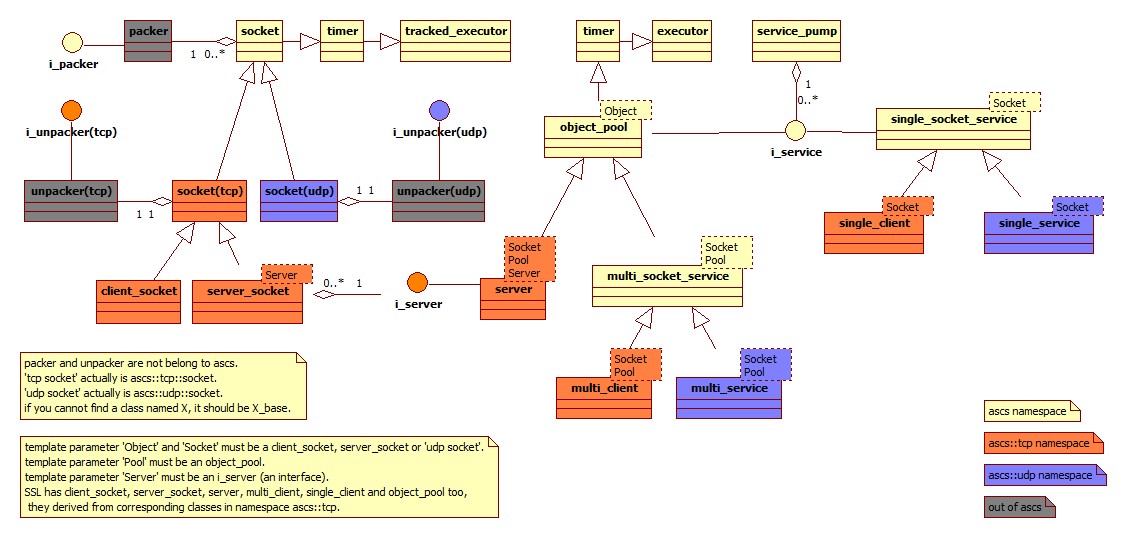
| W: | H:
| W: | H:
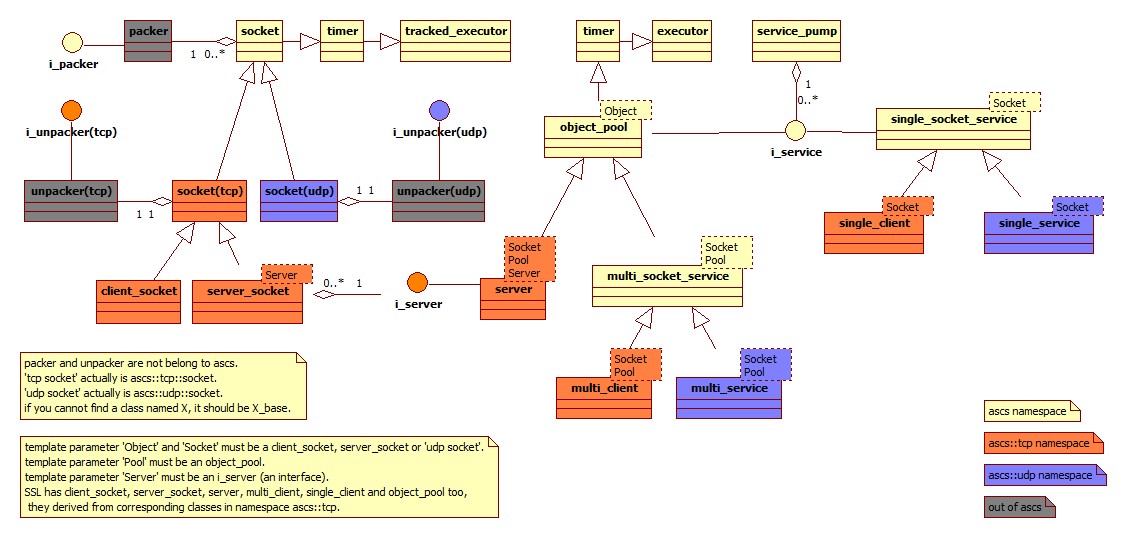
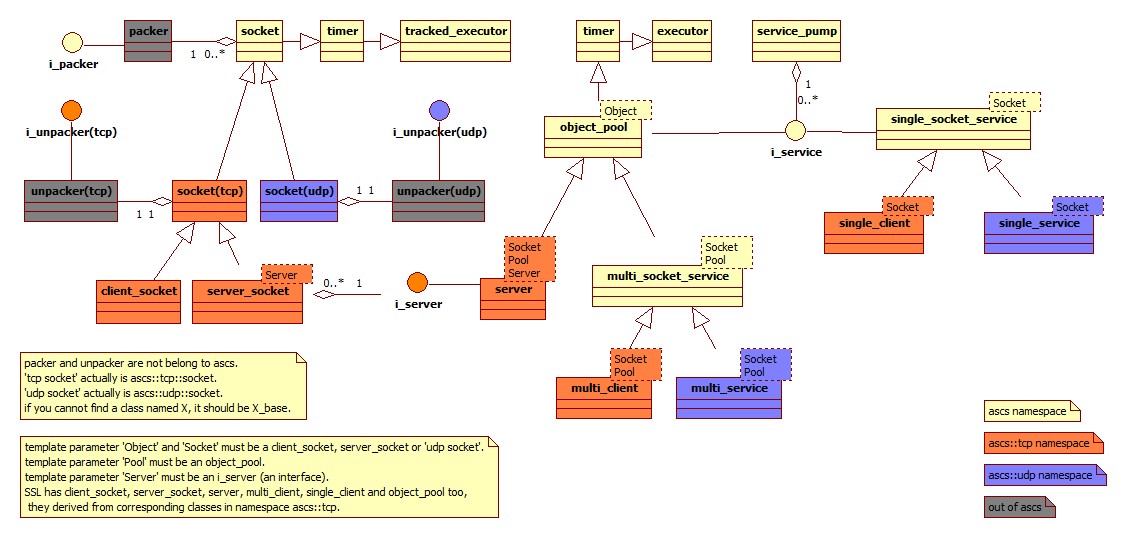
文件已添加
文件已添加
doc/ascs开发文档.docx
已删除
100644 → 0
文件已删除
examples/mingw-build.bat
0 → 100644