Support add picture with offset and scaling.
Showing
无法预览此类型文件
test/images/excel.bmp
已删除
100644 → 0
32.1 KB
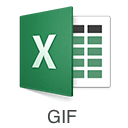
| W: | H:
| W: | H:
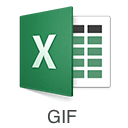
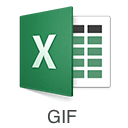
test/images/excel.ico
已删除
100644 → 0
66.1 KB

| W: | H:
| W: | H:


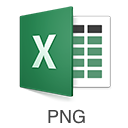
| W: | H:
| W: | H:
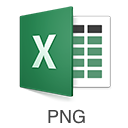
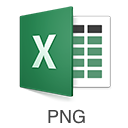
test/images/excel.tif
已删除
100644 → 0
文件已删除