Skip to content
体验新版
项目
组织
正在加载...
登录
切换导航
打开侧边栏
于光远
skill_tree_algorithm
提交
4c49dc90
S
skill_tree_algorithm
项目概览
于光远
/
skill_tree_algorithm
与 Fork 源项目一致
Fork自
CSDN 技术社区 / skill_tree_algorithm
通知
1
Star
1
Fork
0
代码
文件
提交
分支
Tags
贡献者
分支图
Diff
Issue
1
列表
看板
标记
里程碑
合并请求
0
DevOps
流水线
流水线任务
计划
Wiki
0
Wiki
分析
仓库
DevOps
项目成员
Pages
S
skill_tree_algorithm
项目概览
项目概览
详情
发布
仓库
仓库
文件
提交
分支
标签
贡献者
分支图
比较
Issue
1
Issue
1
列表
看板
标记
里程碑
合并请求
0
合并请求
0
Pages
DevOps
DevOps
流水线
流水线任务
计划
分析
分析
仓库分析
DevOps
Wiki
0
Wiki
成员
成员
收起侧边栏
关闭侧边栏
动态
分支图
创建新Issue
流水线任务
提交
Issue看板
体验新版 GitCode,发现更多精彩内容 >>
提交
4c49dc90
编写于
10月 28, 2021
作者:
每日一练社区
浏览文件
操作
浏览文件
下载
电子邮件补丁
差异文件
update exercises
上级
83593f32
变更
3
隐藏空白更改
内联
并排
Showing
3 changed file
with
458 addition
and
26 deletion
+458
-26
data/1.算法初阶/1.蓝桥杯/等差数列/solution.md
data/1.算法初阶/1.蓝桥杯/等差数列/solution.md
+90
-13
data/1.算法初阶/1.蓝桥杯/等差素数列/solution.md
data/1.算法初阶/1.蓝桥杯/等差素数列/solution.md
+128
-4
data/1.算法初阶/1.蓝桥杯/等腰三角形/solution.md
data/1.算法初阶/1.蓝桥杯/等腰三角形/solution.md
+240
-9
未找到文件。
data/1.算法初阶/1.蓝桥杯/等差数列/solution.md
浏览文件 @
4c49dc90
...
...
@@ -15,24 +15,14 @@
#### 输出格式
输出一个整数表示答案。
#### 数据范围
```
2≤N≤100000,
0≤Ai≤109
1
2
```
#### 输入样例:
```
5
2 6 4 10 20
1
2
```
#### 输出样例:
```
10
1
```
#### 样例解释
包含 2、6、4、10、20 的最短的等差数列是 2、4、6、8、10、12、14、16、18、20。
...
...
@@ -41,7 +31,17 @@
## aop
### before
```
cpp
#include <iostream>
#include <algorithm>
using
namespace
std
;
const
int
N
=
1e5
+
10
;
int
a
[
N
];
int
gcd
(
int
a
,
int
b
)
{
return
b
?
gcd
(
b
,
a
%
b
)
:
a
;
}
```
### after
```
cpp
...
...
@@ -50,21 +50,98 @@
## 答案
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
for
(
int
i
=
0
;
i
<
n
;
i
++
)
cin
>>
a
[
i
];
sort
(
a
,
a
+
n
);
int
d
=
0
;
for
(
int
i
=
1
;
i
<
n
;
i
++
)
d
=
gcd
(
d
,
a
[
i
]
-
a
[
0
]);
if
(
d
)
cout
<<
(
a
[
n
-
1
]
-
a
[
0
])
/
d
+
1
;
else
cout
<<
n
;
return
0
;
}
```
## 选项
### A
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
for
(
int
i
=
0
;
i
<
n
;
i
++
)
cin
>>
a
[
i
];
sort
(
a
,
a
+
n
);
int
d
=
0
;
for
(
int
i
=
1
;
i
<
n
;
i
++
)
d
=
gcd
(
d
,
a
[
i
]
-
a
[
0
]);
if
(
d
)
cout
<<
(
a
[
n
]
-
a
[
0
])
/
d
+
1
;
else
cout
<<
n
;
return
0
;
}
```
### B
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
for
(
int
i
=
0
;
i
<
n
;
i
++
)
cin
>>
a
[
i
];
sort
(
a
,
a
+
n
);
int
d
=
0
;
for
(
int
i
=
1
;
i
<
n
;
i
++
)
d
=
gcd
(
d
,
a
[
i
]
-
a
[
0
]);
if
(
d
)
cout
<<
(
a
[
n
-
1
]
-
a
[
0
])
/
d
;
else
cout
<<
n
;
return
0
;
}
```
### C
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
for
(
int
i
=
0
;
i
<
n
;
i
++
)
cin
>>
a
[
i
];
sort
(
a
,
a
+
n
);
int
d
=
0
;
for
(
int
i
=
1
;
i
<
n
;
i
++
)
d
=
gcd
(
d
,
a
[
i
]
-
a
[
0
]);
if
(
d
)
cout
<<
(
a
[
n
+
1
]
-
a
[
0
])
/
d
+
1
;
else
cout
<<
n
;
return
0
;
}
```
data/1.算法初阶/1.蓝桥杯/等差素数列/solution.md
浏览文件 @
4c49dc90
...
...
@@ -15,30 +15,154 @@
## aop
### before
```
cpp
#include <cstdio>
#include <algorithm>
#include <set>
using
namespace
std
;
typedef
long
long
LL
;
set
<
int
>
all
;
bool
isPrimt
(
LL
t
)
{
for
(
int
i
=
2
;
i
<
t
/
2
;
++
i
)
{
if
(
t
%
i
==
0
)
return
false
;
}
return
true
;
}
```
### after
```
cpp
const
int
N
=
5000
;
int
main
()
{
LL
a
[
N
];
a
[
0
]
=
2
;
a
[
1
]
=
3
;
all
.
insert
(
2
);
all
.
insert
(
3
);
int
index
=
2
;
LL
t
=
5
;
while
(
index
<
N
)
{
if
(
isPrimt
(
t
))
{
a
[
index
++
]
=
t
;
all
.
insert
(
t
);
}
t
++
;
}
printf
(
"%d
\n
"
,
f
(
a
,
N
));
return
0
;
}
```
## 答案
```
cpp
int
f
(
LL
a
[],
int
n
)
{
for
(
int
i
=
0
;
i
<
n
;
++
i
)
{
LL
first
=
a
[
i
];
for
(
int
delta
=
1
;
delta
<
a
[
n
-
1
]
-
first
;
++
delta
)
{
int
m
=
first
;
for
(
int
j
=
1
;
j
<
10
;
++
j
)
{
m
+=
delta
;
if
(
all
.
find
(
m
)
==
all
.
end
())
break
;
if
(
m
>
a
[
n
-
1
])
break
;
if
(
j
==
9
)
return
delta
;
}
}
}
return
-
1
;
}
```
## 选项
### A
```
cpp
int
f
(
LL
a
[],
int
n
)
{
for
(
int
i
=
0
;
i
<
n
;
++
i
)
{
LL
first
=
a
[
i
];
for
(
int
delta
=
1
;
delta
<
a
[
n
]
-
first
;
++
delta
)
{
int
m
=
first
;
for
(
int
j
=
1
;
j
<
10
;
++
j
)
{
m
+=
delta
;
if
(
all
.
find
(
m
)
==
all
.
end
())
break
;
if
(
m
>
a
[
n
-
1
])
break
;
if
(
j
==
9
)
return
delta
;
}
}
}
return
-
1
;
}
```
### B
```
cpp
int
f
(
LL
a
[],
int
n
)
{
for
(
int
i
=
0
;
i
<
n
;
++
i
)
{
LL
first
=
a
[
i
];
for
(
int
delta
=
1
;
delta
<
a
[
n
+
1
]
-
first
;
++
delta
)
{
int
m
=
first
;
for
(
int
j
=
1
;
j
<
10
;
++
j
)
{
m
+=
delta
;
if
(
all
.
find
(
m
)
==
all
.
end
())
break
;
if
(
m
>
a
[
n
-
1
])
break
;
if
(
j
==
9
)
return
delta
;
}
}
}
return
-
1
;
}
```
### C
```
cpp
int
f
(
LL
a
[],
int
n
)
{
for
(
int
i
=
0
;
i
<
n
;
++
i
)
{
LL
first
=
a
[
i
];
for
(
int
delta
=
1
;
delta
<
a
[
n
+
1
]
+
first
;
++
delta
)
{
int
m
=
first
;
for
(
int
j
=
1
;
j
<
10
;
++
j
)
{
m
+=
delta
;
if
(
all
.
find
(
m
)
==
all
.
end
())
break
;
if
(
m
>
a
[
n
-
1
])
break
;
if
(
j
==
9
)
return
delta
;
}
}
}
return
-
1
;
}
```
data/1.算法初阶/1.蓝桥杯/等腰三角形/solution.md
浏览文件 @
4c49dc90
...
...
@@ -4,7 +4,7 @@
先用1,2,3,…的自然数拼一个足够长的串
用这个串填充三角形的三条边。从上方顶点开始,逆时针填充。
比如,当三角形高度是8时:
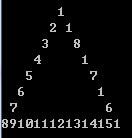
,表示三角形的高度
输出,用数字填充的等腰三角形。
...
...
@@ -15,26 +15,29 @@
5
#### 程序应该输出:




{
int
n
;
cin
>>
n
;
int
x
=
2
*
n
-
1
;
for
(
int
i
=
1
;
i
<=
1500
;
i
++
)
{
string
str1
;
ss
<<
i
;
ss
>>
str1
;
str
+=
str1
;
ss
.
clear
();
}
int
length
=
4
*
n
-
4
;
for
(
int
i
=
1
;
i
<=
n
;
i
++
)
{
if
(
i
!=
n
&&
i
!=
1
)
{
for
(
int
j
=
1
;
j
<=
n
+
i
-
1
;
j
++
)
{
if
(
j
==
n
-
i
+
1
)
{
cout
<<
str
[
i
-
1
];
}
else
if
(
j
==
2
*
n
-
(
n
-
i
+
1
))
{
cout
<<
str
[
4
*
n
-
4
-
(
i
-
1
)]
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
if
(
i
==
1
)
{
for
(
int
x
=
1
;
x
<=
n
;
x
++
)
{
if
(
x
==
n
)
{
cout
<<
'1'
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
{
for
(
int
m
=
1
;
m
<=
2
*
n
-
1
;
m
++
)
{
cout
<<
str
[
n
+
(
m
-
2
)];
}
}
}
return
0
;
}
```
## 选项
### A
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
int
x
=
2
*
n
-
1
;
for
(
int
i
=
1
;
i
<=
1500
;
i
++
)
{
string
str1
;
ss
<<
i
;
ss
>>
str1
;
str
+=
str1
;
ss
.
clear
();
}
int
length
=
4
*
n
-
4
;
for
(
int
i
=
1
;
i
<=
n
;
i
++
)
{
if
(
i
!=
n
&&
i
!=
1
)
{
for
(
int
j
=
1
;
j
<=
n
+
i
-
1
;
j
++
)
{
if
(
j
==
n
-
i
+
1
)
{
cout
<<
str
[
i
-
1
];
}
else
if
(
j
==
2
*
n
-
(
n
-
i
+
1
))
{
cout
<<
str
[
4
*
n
-
4
-
i
]
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
if
(
i
==
1
)
{
for
(
int
x
=
1
;
x
<=
n
;
x
++
)
{
if
(
x
==
n
)
{
cout
<<
'1'
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
{
for
(
int
m
=
1
;
m
<=
2
*
n
-
1
;
m
++
)
{
cout
<<
str
[
n
+
(
m
-
2
)];
}
}
}
return
0
;
}
```
### B
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
int
x
=
2
*
n
-
1
;
for
(
int
i
=
1
;
i
<=
1500
;
i
++
)
{
string
str1
;
ss
<<
i
;
ss
>>
str1
;
str
+=
str1
;
ss
.
clear
();
}
int
length
=
4
*
n
-
4
;
for
(
int
i
=
1
;
i
<=
n
;
i
++
)
{
if
(
i
!=
n
&&
i
!=
1
)
{
for
(
int
j
=
1
;
j
<=
n
+
i
-
1
;
j
++
)
{
if
(
j
==
n
-
i
+
1
)
{
cout
<<
str
[
i
-
1
];
}
else
if
(
j
==
2
*
n
-
(
n
-
i
+
1
))
{
cout
<<
str
[
4
*
n
-
4
-
(
i
+
1
)]
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
if
(
i
==
1
)
{
for
(
int
x
=
1
;
x
<=
n
;
x
++
)
{
if
(
x
==
n
)
{
cout
<<
'1'
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
{
for
(
int
m
=
1
;
m
<=
2
*
n
-
1
;
m
++
)
{
cout
<<
str
[
n
+
(
m
-
2
)];
}
}
}
return
0
;
}
```
### C
```
cpp
int
main
()
{
int
n
;
cin
>>
n
;
int
x
=
2
*
n
-
1
;
for
(
int
i
=
1
;
i
<=
1500
;
i
++
)
{
string
str1
;
ss
<<
i
;
ss
>>
str1
;
str
+=
str1
;
ss
.
clear
();
}
int
length
=
4
*
n
-
4
;
for
(
int
i
=
1
;
i
<=
n
;
i
++
)
{
if
(
i
!=
n
&&
i
!=
1
)
{
for
(
int
j
=
1
;
j
<=
n
+
i
-
1
;
j
++
)
{
if
(
j
==
n
-
i
+
1
)
{
cout
<<
str
[
i
-
1
];
}
else
if
(
j
==
2
*
n
-
(
n
-
i
+
1
))
{
cout
<<
str
[
4
*
n
-
4
+
i
]
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
if
(
i
==
1
)
{
for
(
int
x
=
1
;
x
<=
n
;
x
++
)
{
if
(
x
==
n
)
{
cout
<<
'1'
<<
endl
;
}
else
{
cout
<<
'.'
;
}
}
}
else
{
for
(
int
m
=
1
;
m
<=
2
*
n
-
1
;
m
++
)
{
cout
<<
str
[
n
+
(
m
-
2
)];
}
}
}
return
0
;
}
```
编辑
预览
Markdown
is supported
0%
请重试
或
添加新附件
.
添加附件
取消
You are about to add
0
people
to the discussion. Proceed with caution.
先完成此消息的编辑!
取消
想要评论请
注册
或
登录