Merge branch 'develop' of https://github.com/PaddlePaddle/Paddle into dev_add_axis
Showing
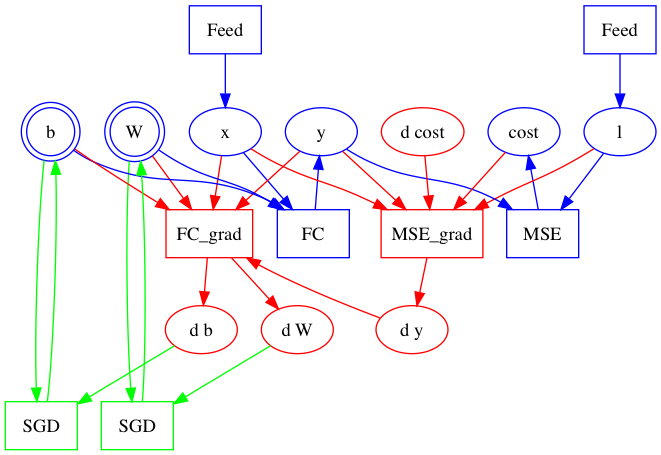
| W: | H:
| W: | H:
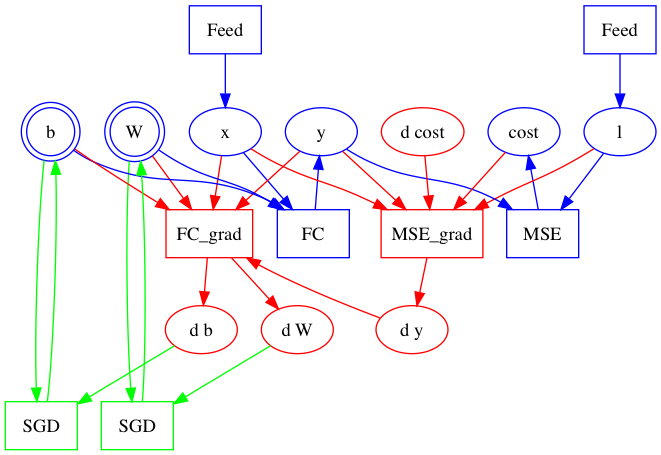
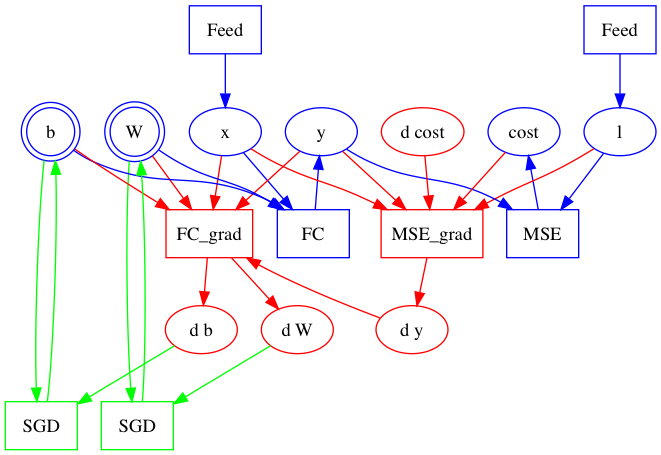

| W: | H:
| W: | H:



| W: | H:
| W: | H:


54.1 KB | W: | H:
58.3 KB | W: | H:
46.1 KB | W: | H:
50.2 KB | W: | H:
28.5 KB | W: | H:
31.5 KB | W: | H: