Merge pull request #536 from lepdou/delete_namespace
delete namespace
Showing
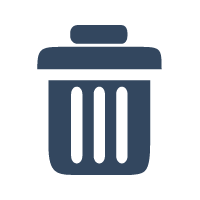
| W: | H:
| W: | H:
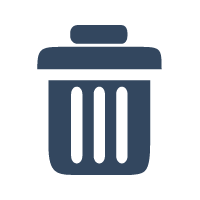
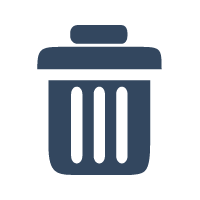
4.7 KB
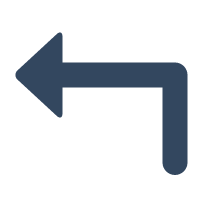
| W: | H:
| W: | H:
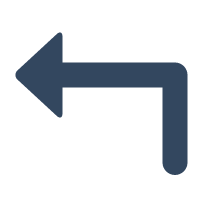
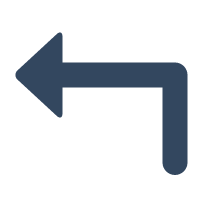
4.2 KB
2.2 KB
4.2 KB
从无法访问的项目Fork
delete namespace
3.4 KB | W: | H:
4.0 KB | W: | H:
4.7 KB
2.7 KB | W: | H:
2.3 KB | W: | H:
4.2 KB
2.2 KB
4.2 KB