Merge branch 'develop' of https://github.com/baidu/Paddle into inference
Showing

| W: | H:
| W: | H:


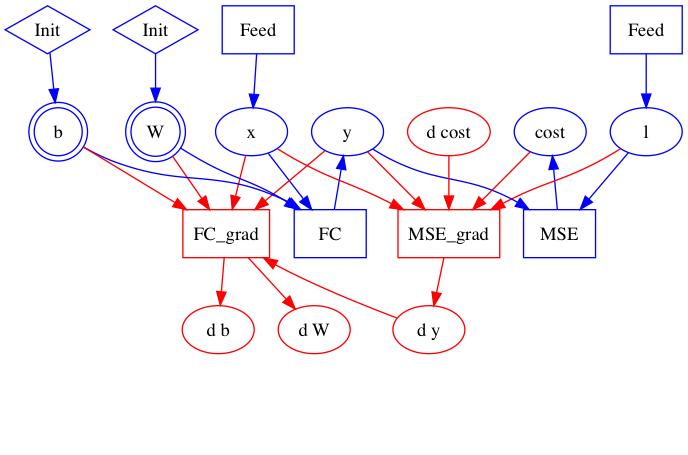
| W: | H:
| W: | H:
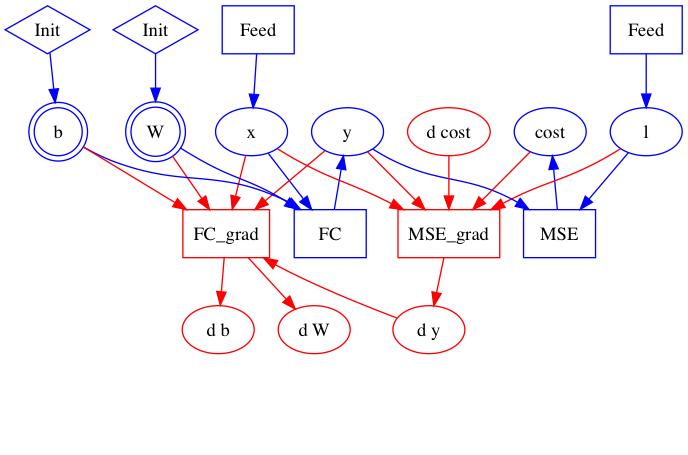
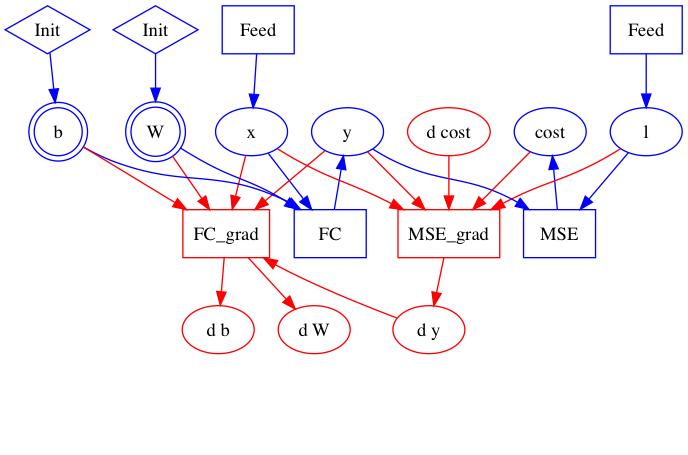
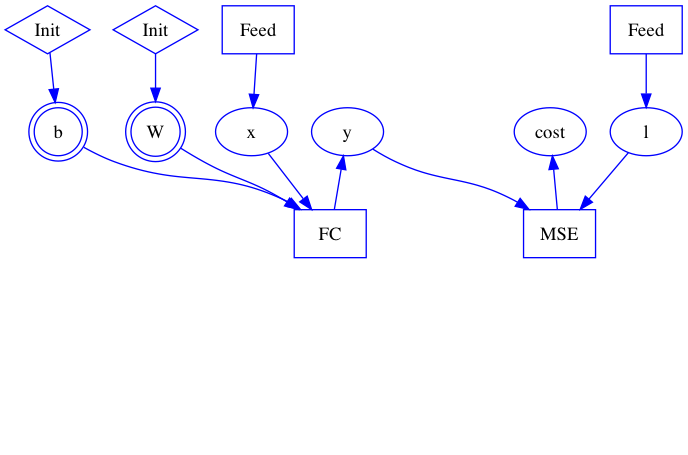
| W: | H:
| W: | H:
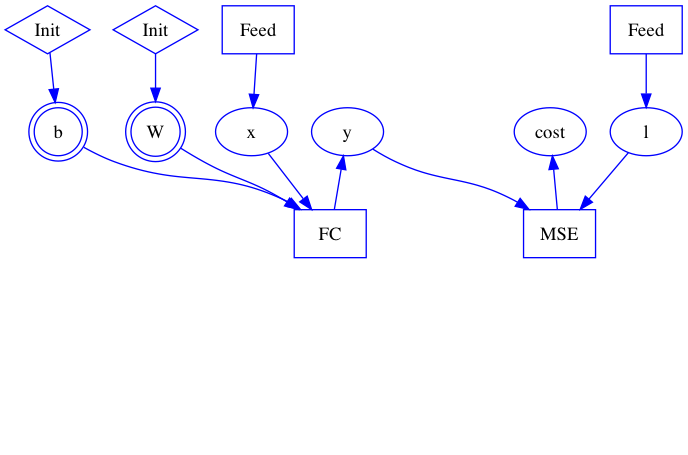
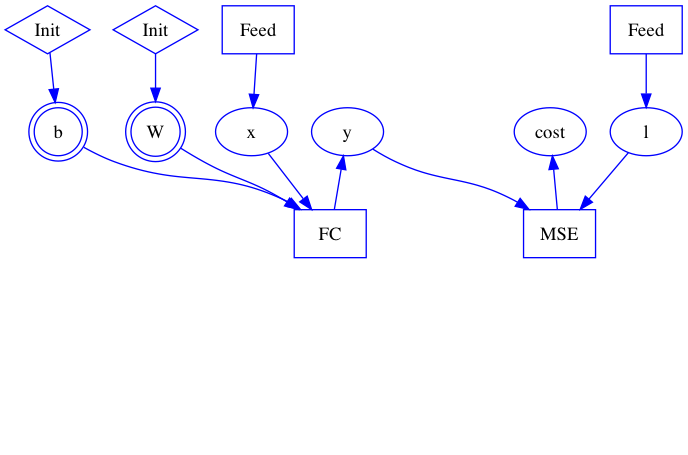
paddle/operators/adam_op.cc
0 → 100644
paddle/operators/adam_op.cu
0 → 100644
paddle/operators/adam_op.h
0 → 100644