Skip to content
体验新版
项目
组织
正在加载...
登录
切换导航
打开侧边栏
PaddlePaddle
PaddleHub
提交
e4d3bbce
P
PaddleHub
项目概览
PaddlePaddle
/
PaddleHub
接近 2 年 前同步成功
通知
284
Star
12117
Fork
2091
代码
文件
提交
分支
Tags
贡献者
分支图
Diff
Issue
200
列表
看板
标记
里程碑
合并请求
4
Wiki
0
Wiki
分析
仓库
DevOps
项目成员
Pages
P
PaddleHub
项目概览
项目概览
详情
发布
仓库
仓库
文件
提交
分支
标签
贡献者
分支图
比较
Issue
200
Issue
200
列表
看板
标记
里程碑
合并请求
4
合并请求
4
Pages
分析
分析
仓库分析
DevOps
Wiki
0
Wiki
成员
成员
收起侧边栏
关闭侧边栏
动态
分支图
创建新Issue
提交
Issue看板
未验证
提交
e4d3bbce
编写于
3月 22, 2021
作者:
jm_12138
提交者:
GitHub
3月 22, 2021
浏览文件
操作
浏览文件
下载
电子邮件补丁
差异文件
add vlpr module (#1329)
上级
3027b5cf
变更
2
隐藏空白更改
内联
并排
Showing
2 changed file
with
145 addition
and
0 deletion
+145
-0
modules/thirdparty/image/text_recognition/Vehicle_License_Plate_Recognition/README.md
...t_recognition/Vehicle_License_Plate_Recognition/README.md
+83
-0
modules/thirdparty/image/text_recognition/Vehicle_License_Plate_Recognition/module.py
...t_recognition/Vehicle_License_Plate_Recognition/module.py
+62
-0
未找到文件。
modules/thirdparty/image/text_recognition/Vehicle_License_Plate_Recognition/README.md
0 → 100644
浏览文件 @
e4d3bbce
## 概述
Vehicle_License_Plate_Recognition 是一个基于 CCPD 数据集训练的车牌识别模型,能够检测出图像中车牌位置并识别其中的车牌文字信息,大致的模型结构如下,分为检测车牌和文字识别两个模块:
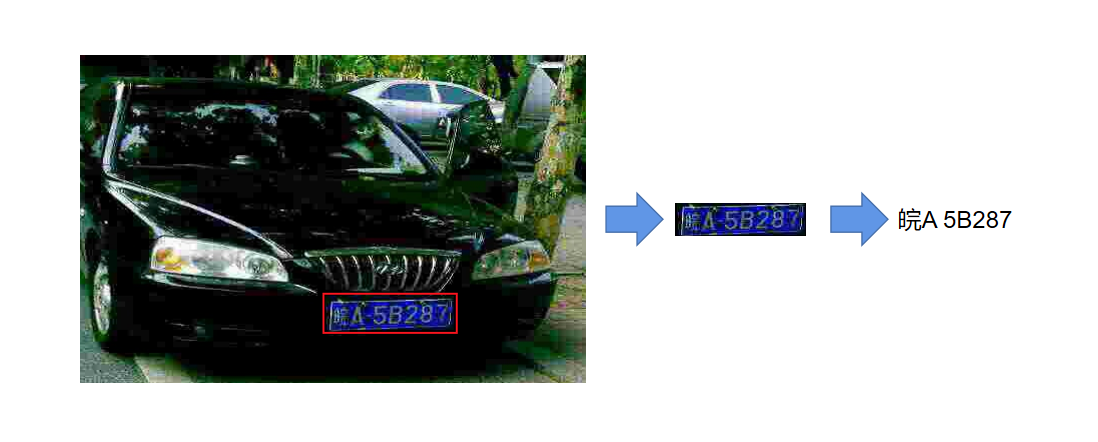
## API
```
python
def
plate_recognition
(
images
)
```
车牌识别 API
**参数**
*
images(str / ndarray / list(str) / list(ndarray)):待识别图像的路径或者图像的 Ndarray(RGB)
**返回**
*
results(list(dict{'license', 'bbox'})): 识别到的车牌信息列表,包含车牌的位置坐标和车牌号码
**代码示例**
```
python
import
paddlehub
as
hub
# 加载模型
model
=
hub
.
Module
(
name
=
'Vehicle_License_Plate_Recognition'
)
# 车牌识别
result
=
model
.
plate_recognition
(
"test.jpg"
)
# 打印结果
print
(
result
)
```
[{'license': '苏B92912', 'bbox': [[131.0, 251.0], [368.0, 253.0], [367.0, 338.0], [131.0, 336.0]]}]
## 服务部署
PaddleHub Serving 可以部署一个在线车牌识别服务。
## 第一步:启动PaddleHub Serving
运行启动命令:
```
shell
$
hub serving start
--modules
Vehicle_License_Plate_Recognition
```
这样就完成了一个车牌识别的在线服务API的部署,默认端口号为8866。
**NOTE:**
如使用GPU预测,则需要在启动服务之前,请设置CUDA
\_
VISIBLE
\_
DEVICES环境变量,否则不用设置。
## 第二步:发送预测请求
配置好服务端,以下数行代码即可实现发送预测请求,获取预测结果
```
python
import
requests
import
json
import
cv2
import
base64
def
cv2_to_base64
(
image
):
data
=
cv2
.
imencode
(
'.jpg'
,
image
)[
1
]
return
base64
.
b64encode
(
data
.
tostring
()).
decode
(
'utf8'
)
# 发送HTTP请求
data
=
{
'images'
:[
cv2_to_base64
(
cv2
.
imread
(
"test.jpg"
))]}
headers
=
{
"Content-type"
:
"application/json"
}
url
=
"http://127.0.0.1:8866/predict/Vehicle_License_Plate_Recognition"
r
=
requests
.
post
(
url
=
url
,
headers
=
headers
,
data
=
json
.
dumps
(
data
))
# 打印预测结果
print
(
r
.
json
()[
"results"
])
```
[{'bbox': [[260.0, 100.0], [546.0, 104.0], [544.0, 200.0], [259.0, 196.0]], 'license': '苏DS0000'}]
## 查看代码
https://github.com/jm12138/License_plate_recognition
## 依赖
paddlepaddle >= 2.0.0
paddlehub >= 2.0.4
paddleocr >= 2.0.2
\ No newline at end of file
modules/thirdparty/image/text_recognition/Vehicle_License_Plate_Recognition/module.py
0 → 100644
浏览文件 @
e4d3bbce
import
os
import
cv2
import
base64
import
numpy
as
np
import
paddle.nn
as
nn
from
paddleocr
import
PaddleOCR
from
paddlehub.module.module
import
moduleinfo
,
serving
@
moduleinfo
(
name
=
"Vehicle_License_Plate_Recognition"
,
type
=
"CV/text_recognition"
,
author
=
"jm12138"
,
author_email
=
""
,
summary
=
"Vehicle_License_Plate_Recognition"
,
version
=
"1.0.0"
)
class
Vehicle_License_Plate_Recognition
(
nn
.
Layer
):
def
__init__
(
self
):
super
(
Vehicle_License_Plate_Recognition
,
self
).
__init__
()
self
.
vlpr
=
PaddleOCR
(
det_model_dir
=
os
.
path
.
join
(
self
.
directory
,
'det_vlpr'
),
rec_model_dir
=
os
.
path
.
join
(
self
.
directory
,
'ch_ppocr_server_v2.0_rec_infer'
)
)
@
staticmethod
def
base64_to_cv2
(
b64str
):
data
=
base64
.
b64decode
(
b64str
.
encode
(
'utf8'
))
data
=
np
.
frombuffer
(
data
,
np
.
uint8
)
data
=
cv2
.
imdecode
(
data
,
cv2
.
IMREAD_COLOR
)
return
data
def
plate_recognition
(
self
,
images
=
None
):
assert
isinstance
(
images
,
(
list
,
str
,
np
.
ndarray
))
results
=
[]
if
isinstance
(
images
,
list
):
for
item
in
images
:
for
bbox
,
text
in
self
.
vlpr
.
ocr
(
item
):
results
.
append
({
'license'
:
text
[
0
],
'bbox'
:
bbox
})
elif
isinstance
(
images
,
(
str
,
np
.
ndarray
)):
for
bbox
,
text
in
self
.
vlpr
.
ocr
(
images
):
results
.
append
({
'license'
:
text
[
0
],
'bbox'
:
bbox
})
return
results
@
serving
def
serving_method
(
self
,
images
):
if
isinstance
(
images
,
list
):
images_decode
=
[
self
.
base64_to_cv2
(
image
)
for
image
in
images
]
elif
isinstance
(
images
,
str
):
images_decode
=
self
.
base64_to_cv2
(
images
)
return
self
.
plate_recognition
(
images_decode
)
编辑
预览
Markdown
is supported
0%
请重试
或
添加新附件
.
添加附件
取消
You are about to add
0
people
to the discussion. Proceed with caution.
先完成此消息的编辑!
取消
想要评论请
注册
或
登录