Update docs (22121)
Signed-off-by: Nester.zhou <ester.zhou@huawei.com>
Showing
| W: | H:
| W: | H:
125.9 KB
268.1 KB
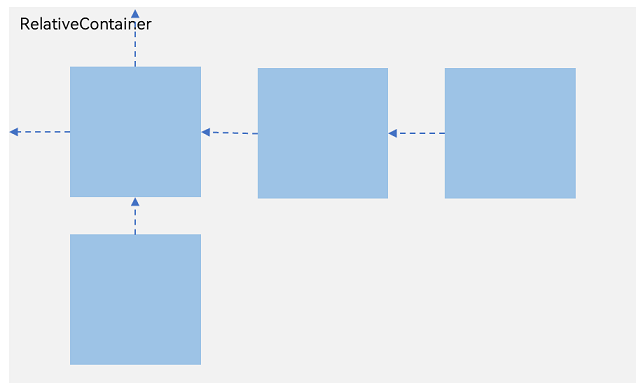
| W: | H:
| W: | H:
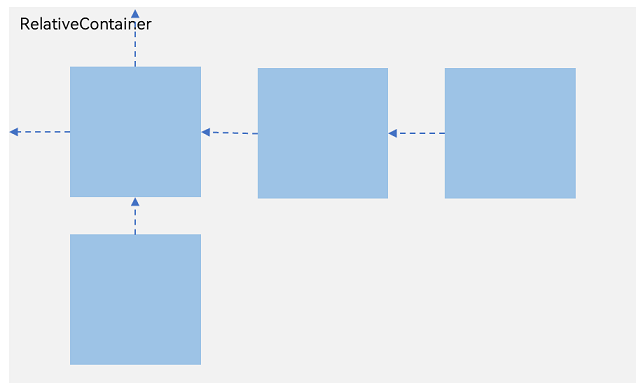
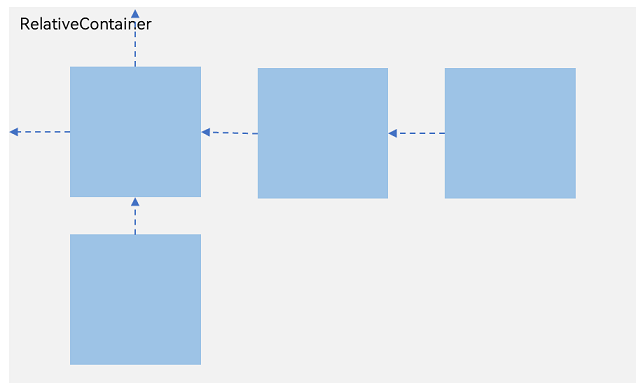
Signed-off-by: Nester.zhou <ester.zhou@huawei.com>
7.1 KB | W: | H:
22.7 KB | W: | H:
125.9 KB
268.1 KB
9.7 KB | W: | H:
23.4 KB | W: | H: