!21421 翻译完成:20953+20904+20954+20554 media 文件夹更新
Merge pull request !21421 from wusongqing/TR20953
Showing
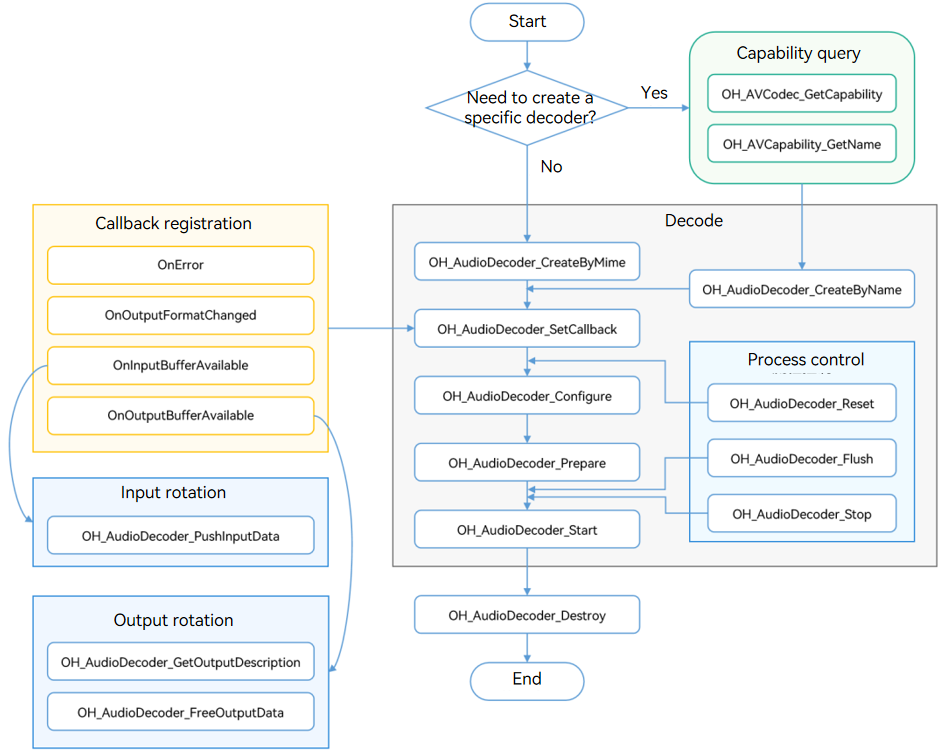
| W: | H:
| W: | H:
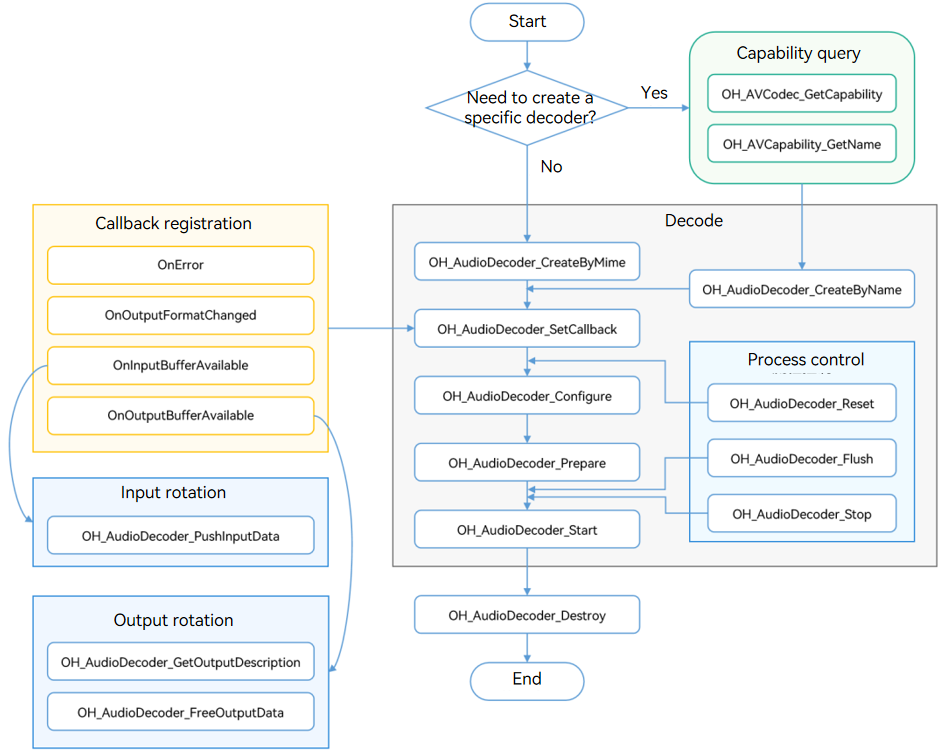
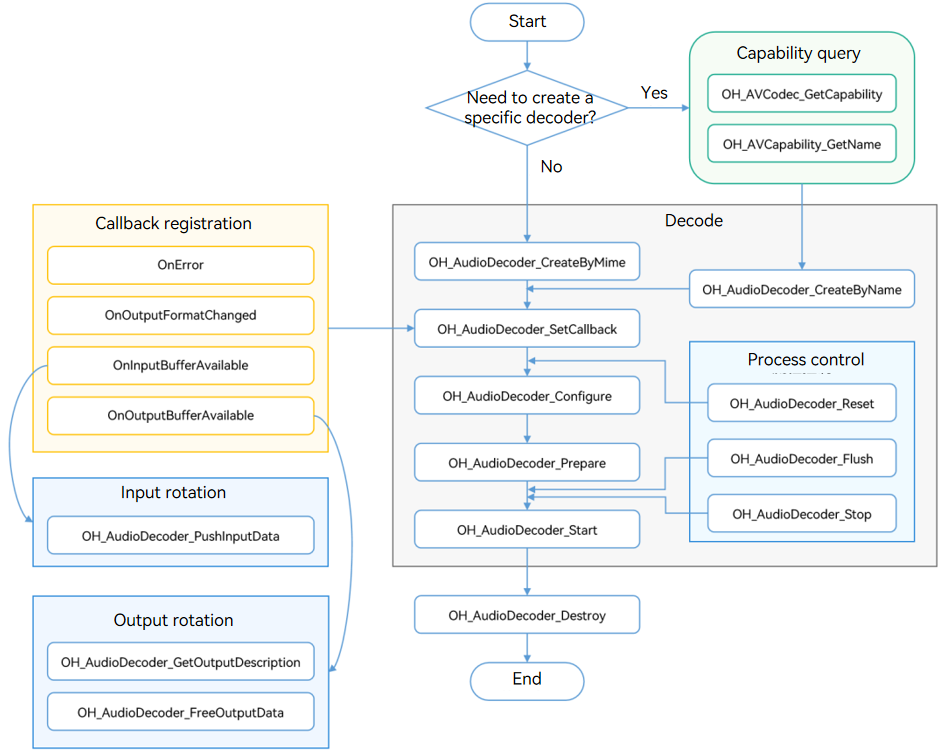
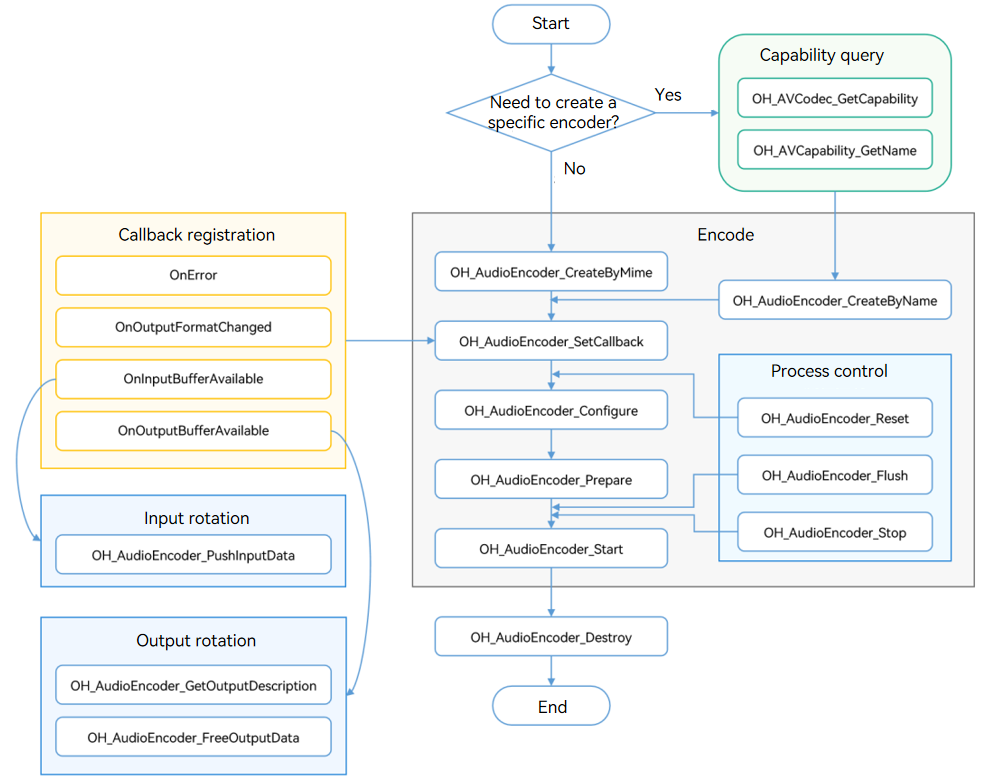
| W: | H:
| W: | H:
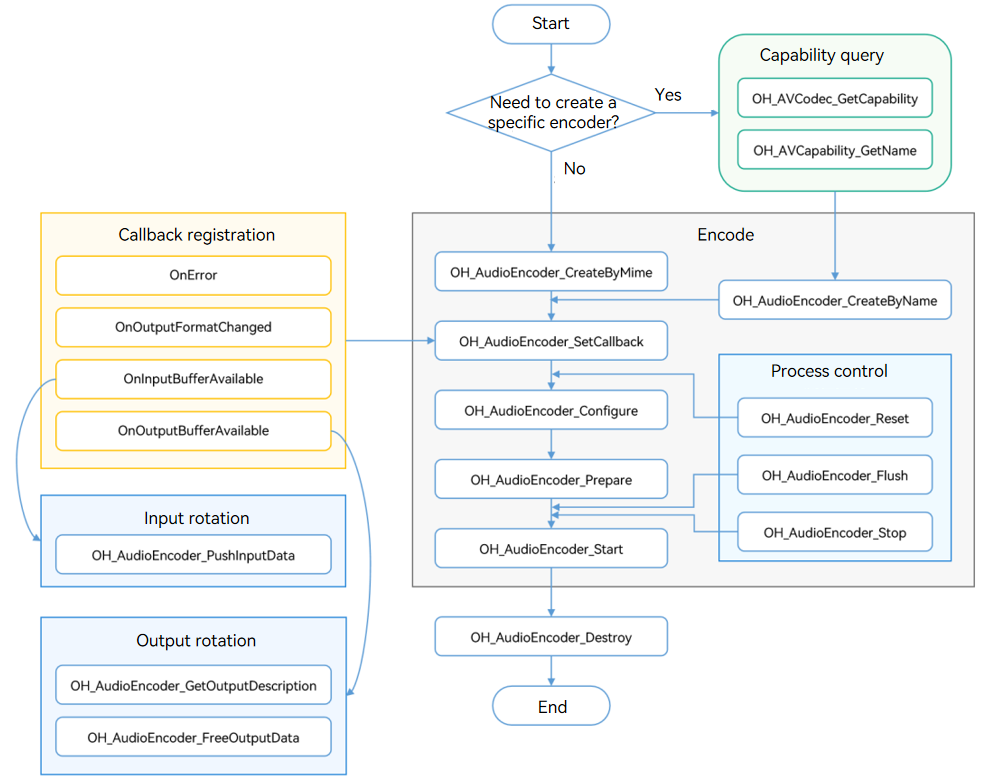
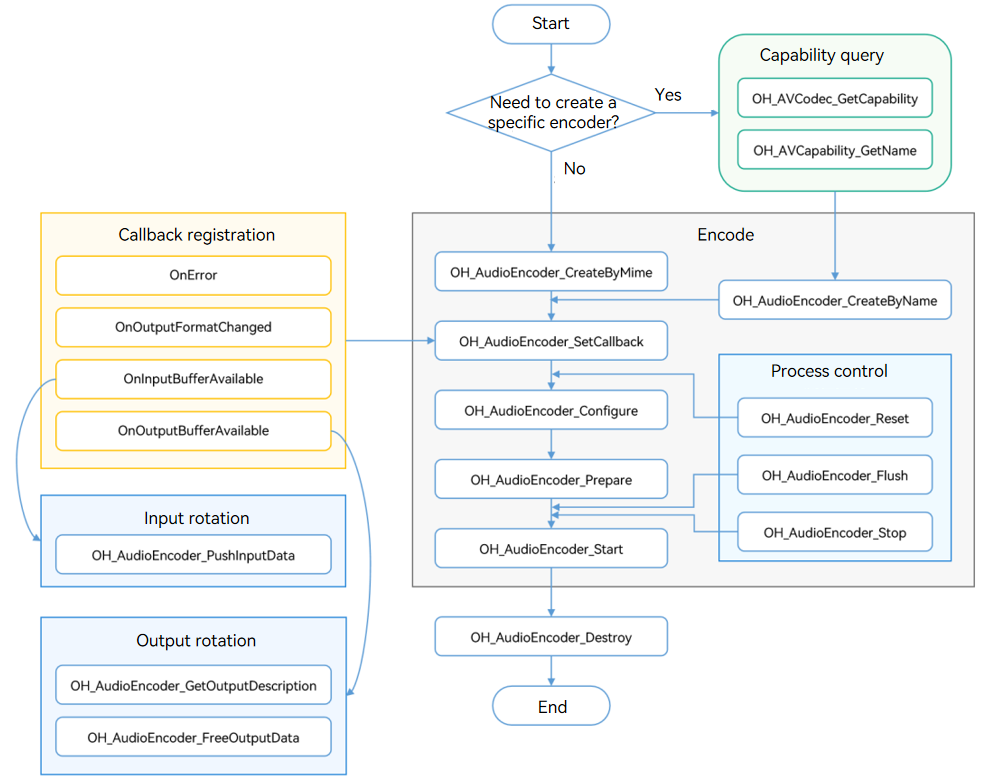
161.8 KB
169.6 KB
Merge pull request !21421 from wusongqing/TR20953
132.0 KB | W: | H:
114.4 KB | W: | H:
136.6 KB | W: | H:
120.4 KB | W: | H:
161.8 KB
169.6 KB