cherry-pick docs from master
Signed-off-by: wusongqing<wusongqing@huawei.com>
Showing
35.9 KB
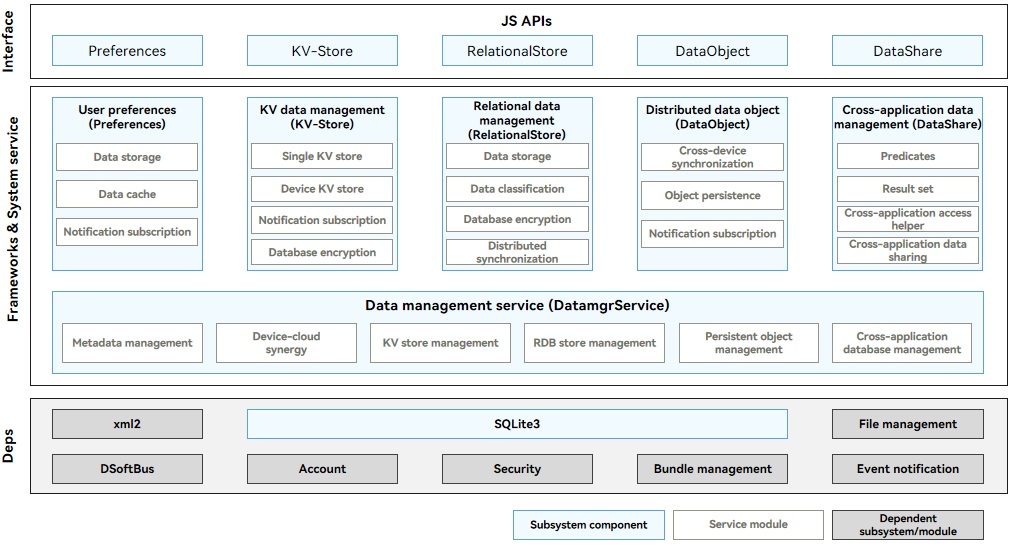
| W: | H:
| W: | H:
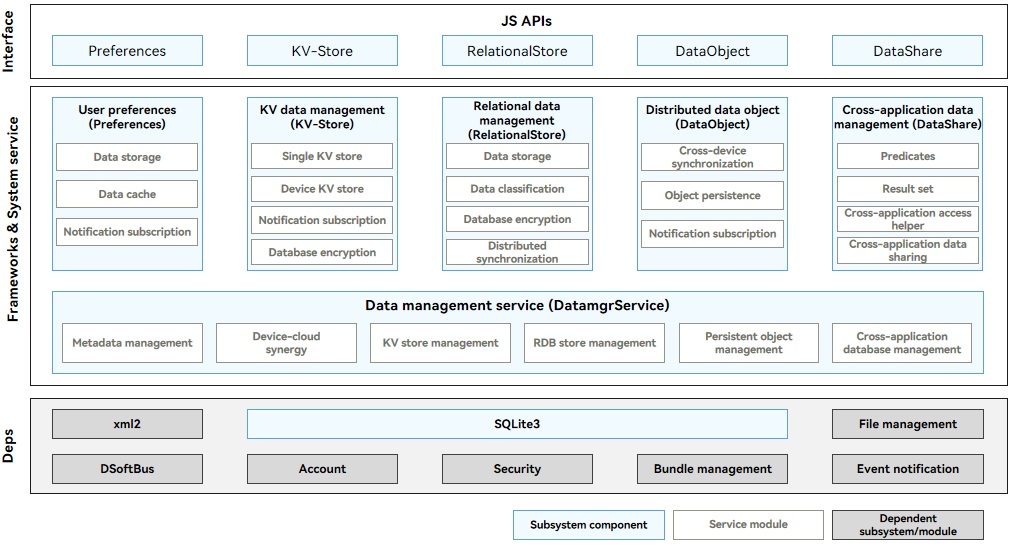
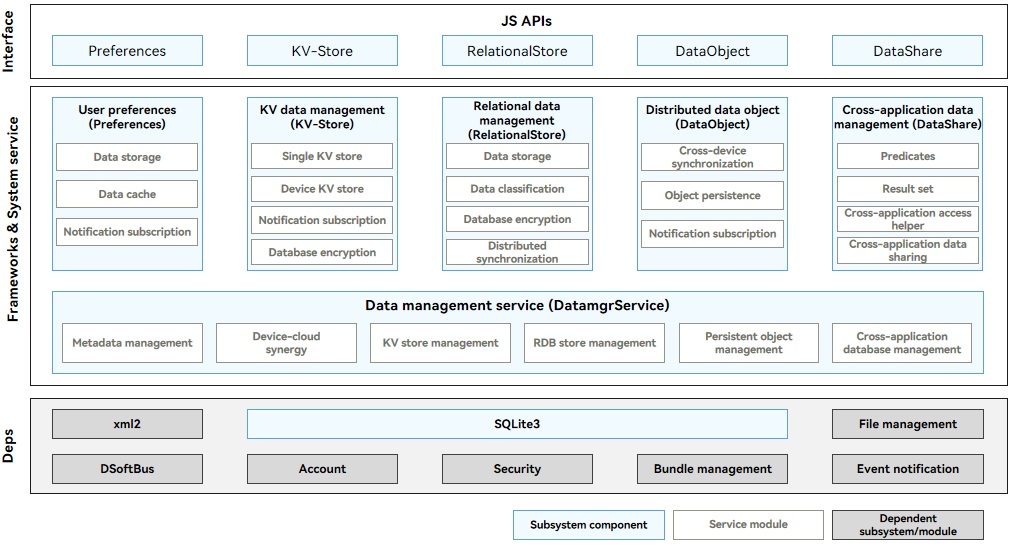
5.9 KB
19.1 KB
9.9 KB
7.4 KB
16.9 KB
此差异已折叠。

| W: | H:
| W: | H:



| W: | H:
| W: | H:



| W: | H:
| W: | H:



| W: | H:
| W: | H:


209.0 KB
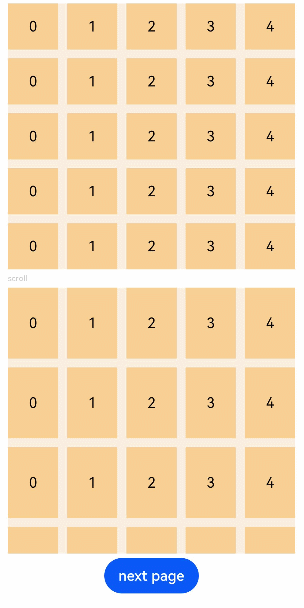
| W: | H:
| W: | H:
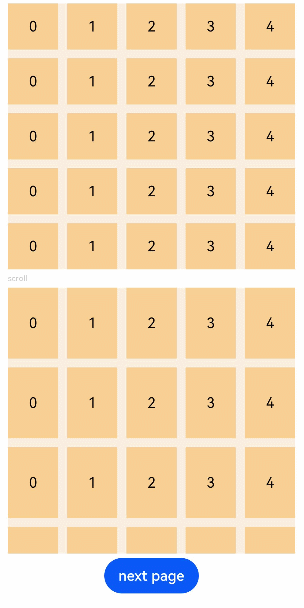
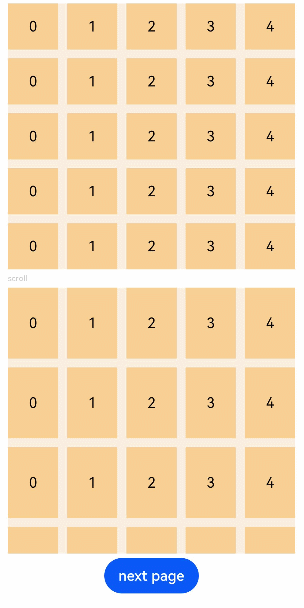
58.3 KB
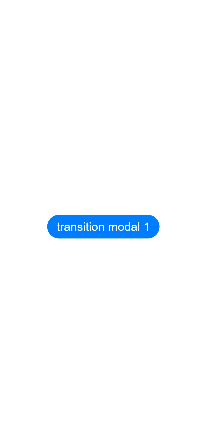
| W: | H:
| W: | H:
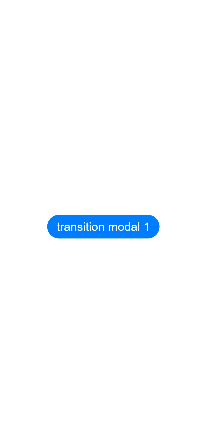
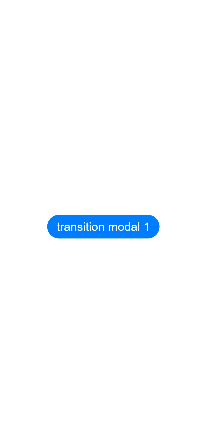
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
50.2 KB
54.8 KB
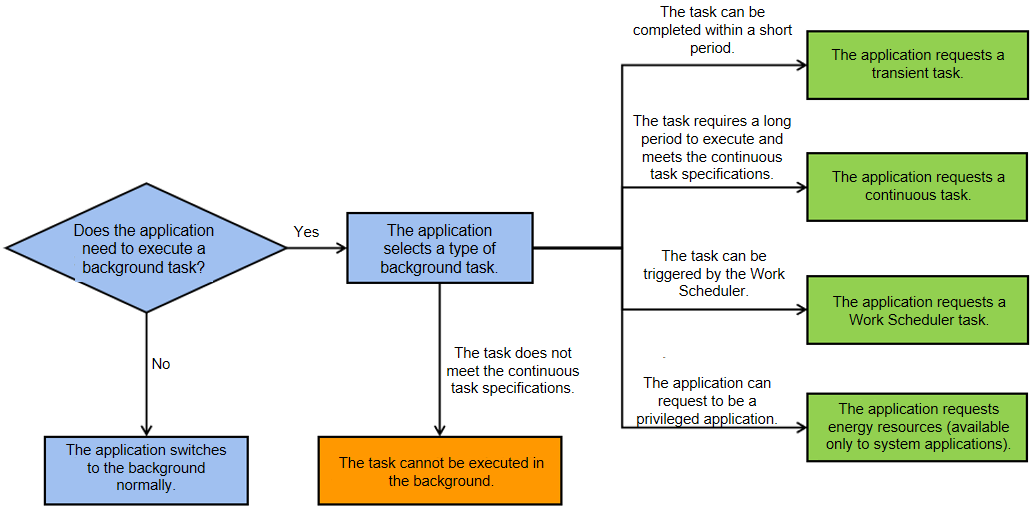
| W: | H:
| W: | H:
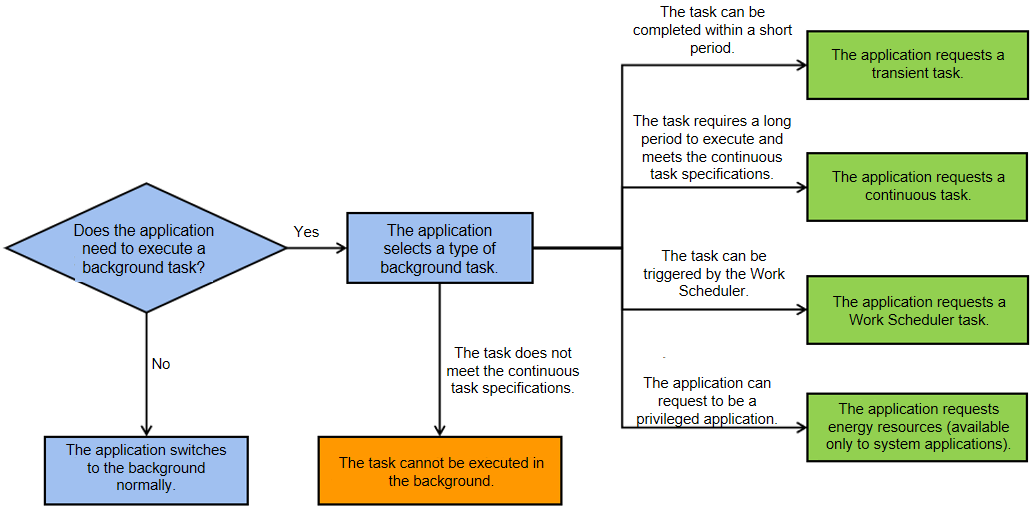
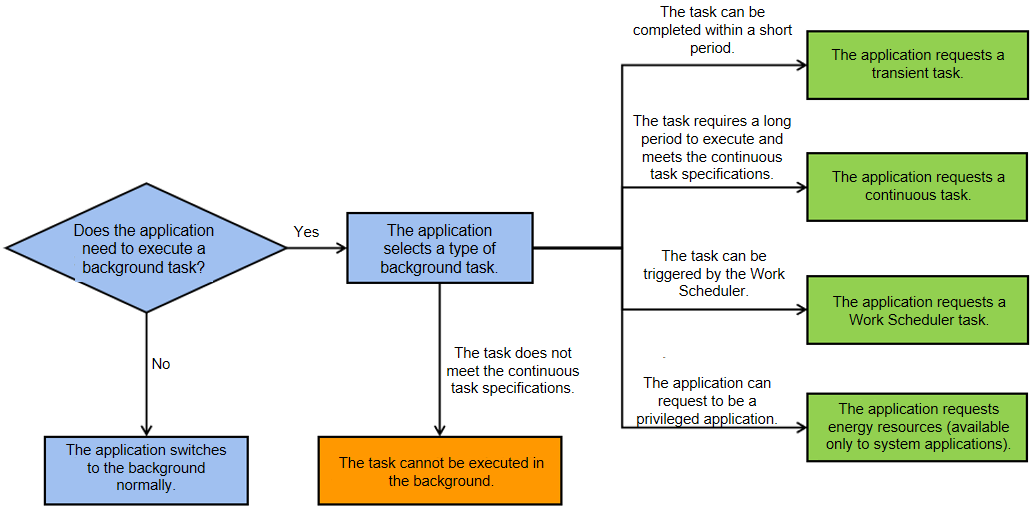
243.7 KB
25.5 KB
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。