Description: add readme files
Team:OTHERS Feature or Bugfix:Feature Binary Source:en/readme/figures/driver-installation.png PrivateCode(Yes/No):No Change-Id: I795fd40e8569fb6148cc3aed0accad5e4e66085b ChangeID:13278305
Showing
en/readme/ai.md
0 → 100755
en/readme/application-framework.md
100644 → 100755
此差异已折叠。
en/readme/compilation-and-building-subsystem.md
100644 → 100755
en/readme/dfx.md
100644 → 100755
此差异已折叠。
en/readme/distributed-scheduler.md
100644 → 100755
en/readme/driver-subsystem.md
100644 → 100755
en/readme/figures/1.png
已删除
100644 → 0
208.0 KB
en/readme/figures/2.png
已删除
100644 → 0
140.1 KB
37.3 KB
21.3 KB
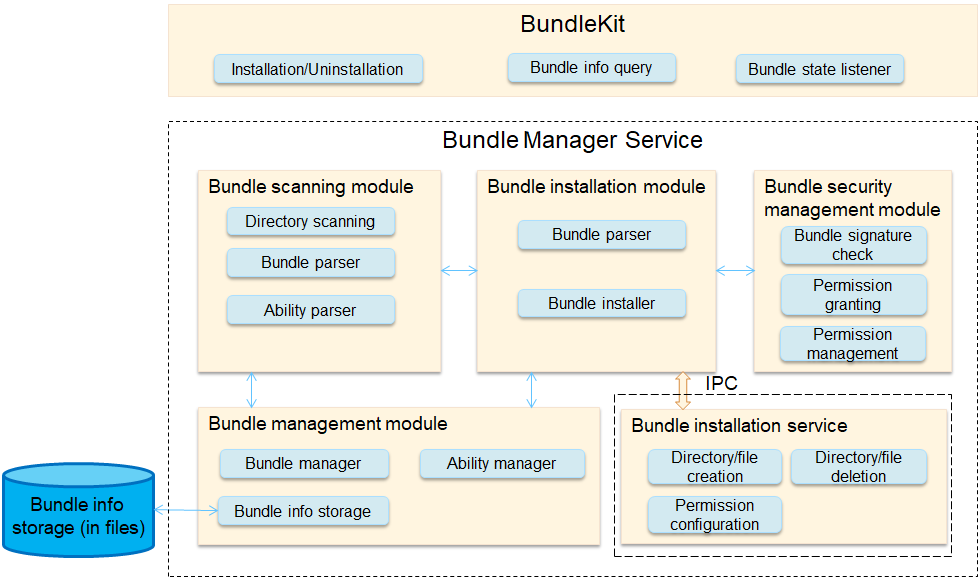
| W: | H:
| W: | H:
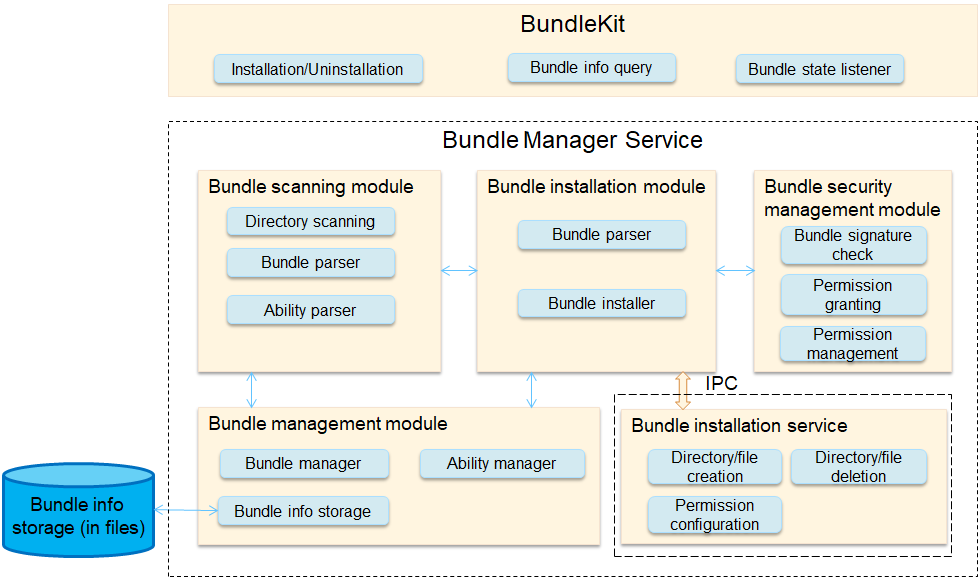
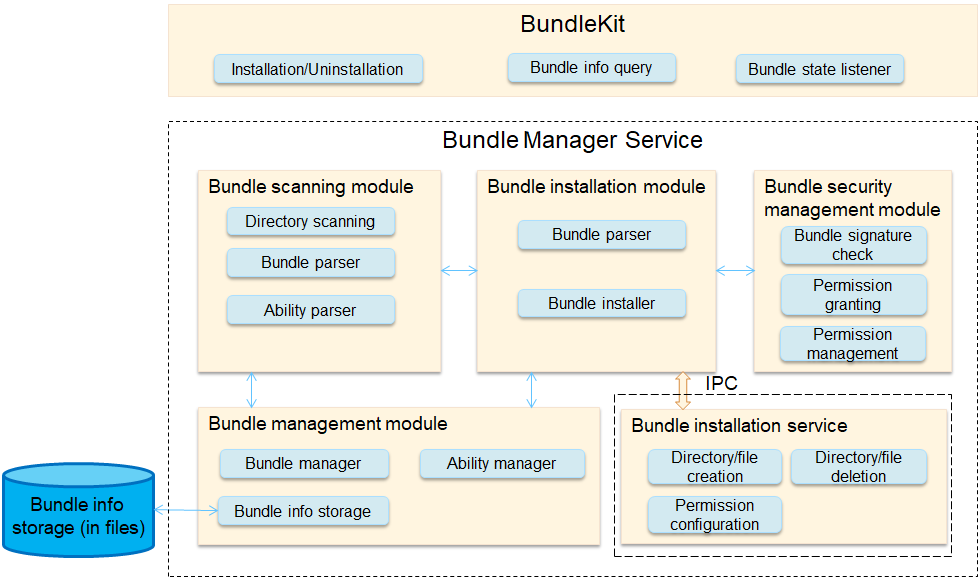
24.2 KB
en/readme/figures/bms策略举例.png
100644 → 100755
文件模式从 100644 更改为 100755
52.6 KB
73.8 KB
96.8 KB
56.3 KB
141.1 KB
221.7 KB
58.3 KB
en/readme/figures/en-us_image_0000001052584330.png
100644 → 100755
文件模式从 100644 更改为 100755
69.9 KB
34.7 KB
67.0 KB
26.9 KB
73.4 KB
en/readme/figures/en-us_image_0000001054941316.png
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/figures/en-us_image_0000001055103250.png
100644 → 100755
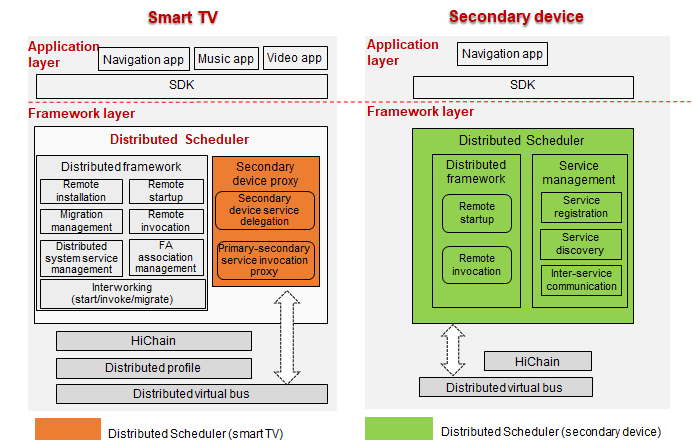
| W: | H:
| W: | H:
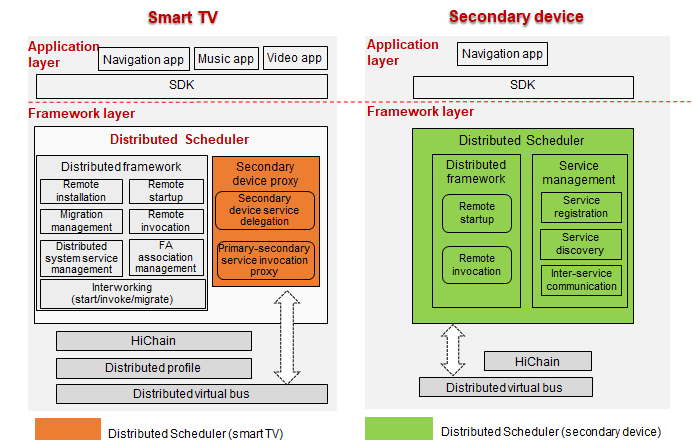
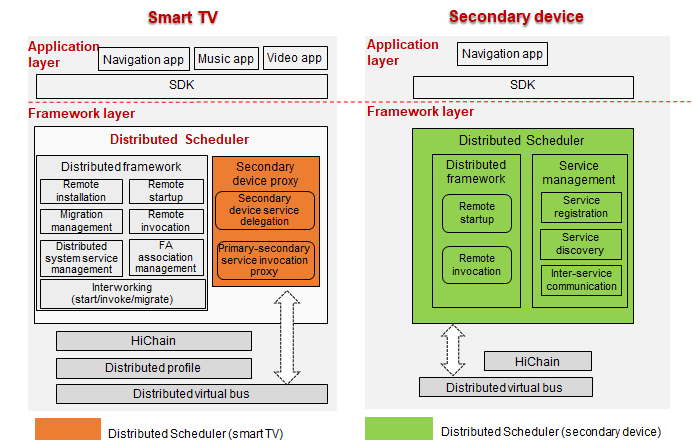
45.3 KB
69.3 KB
en/readme/figures/en-us_image_0000001055267336.png
100644 → 100755
文件模式从 100644 更改为 100755
6.2 KB
15.2 KB
14.2 KB
15.7 KB
156.6 KB
80.7 KB
67.1 KB
文件模式从 100644 更改为 100755
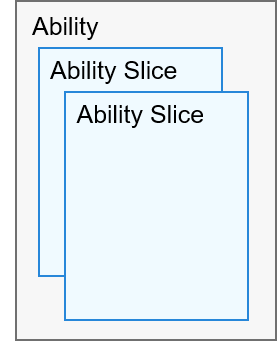
| W: | H:
| W: | H:
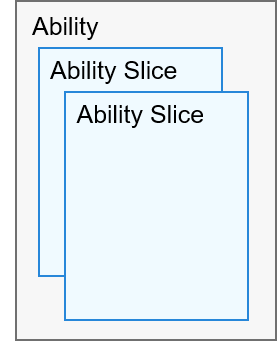
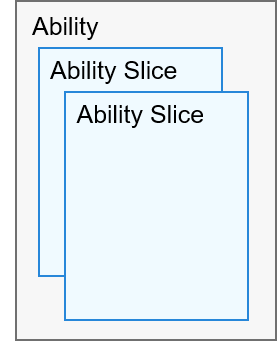
49.5 KB
40.0 KB
en/readme/figures/全局策略2.png
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/figures/策略类型2.png
100644 → 100755
文件模式从 100644 更改为 100755
31.9 KB
en/readme/globalization.md
0 → 100755
en/readme/graphics-subsystem.md
100644 → 100755
en/readme/intelligent-soft-bus.md
0 → 100755
en/readme/js-application-framework.md
100644 → 100755
en/readme/kernel-subsystem.md
已删除
100644 → 0
en/readme/kernel.md
0 → 100755
en/readme/liteipc_driver.md
已删除
100644 → 0
此差异已折叠。
en/readme/overview.md
已删除
100644 → 0
en/readme/pan-sensor.md
0 → 100755
en/readme/public_sys-resources/icon-caution.gif
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/public_sys-resources/icon-danger.gif
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/public_sys-resources/icon-note.gif
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/public_sys-resources/icon-notice.gif
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/public_sys-resources/icon-tip.gif
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/public_sys-resources/icon-warning.gif
100644 → 100755
文件模式从 100644 更改为 100755
en/readme/security-subsystem.md
100644 → 100755
此差异已折叠。
en/readme/startup.md
100644 → 100755
此差异已折叠。
en/readme/testing-subsystem.md
100644 → 100755
此差异已折叠。
en/readme/update.md
0 → 100755
en/readme/utils-library.md
已删除
100644 → 0
en/readme/utils.md
0 → 100755
此差异已折叠。
此差异已折叠。
en/readme/x-test-suite.md
0 → 100755
此差异已折叠。