Merge branch 'master' of gitee.com:openharmony/docs into master
Signed-off-by: N赵淦 <zhaogan2@huawei.com>
Showing
489.5 KB
68.9 KB
99.5 KB
50.6 KB
此差异已折叠。
77.2 KB
29.9 KB
33.3 KB
140.2 KB
文件已移动
文件已移动
文件已移动
文件已移动
文件已移动
文件已移动
文件已移动
文件已移动
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
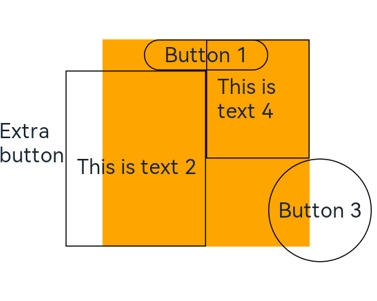
| W: | H:
| W: | H:
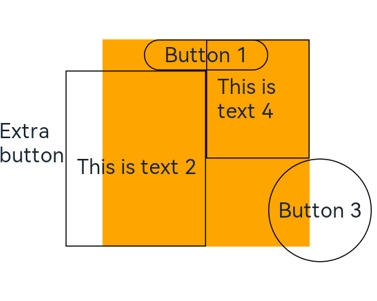
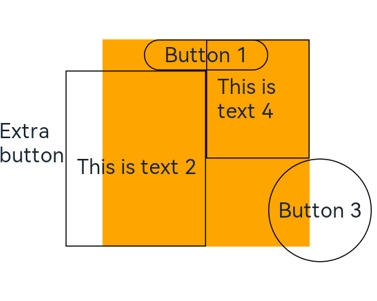
此差异已折叠。
219.7 KB
此差异已折叠。
此差异已折叠。
此差异已折叠。