!19116 翻译完成 17976
Merge pull request !19116 from ester.zhou/TR-17976
Showing
140.1 KB
40.5 KB
74.0 KB
85.2 KB
643.4 KB
328.6 KB
109.7 KB
243.3 KB
103.2 KB
393.0 KB
45.5 KB
233.9 KB
157.4 KB
110.3 KB
97.7 KB
19.5 KB
31.0 KB
29.1 KB
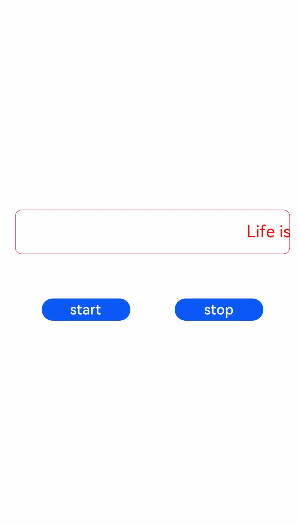
| W: | H:
| W: | H:
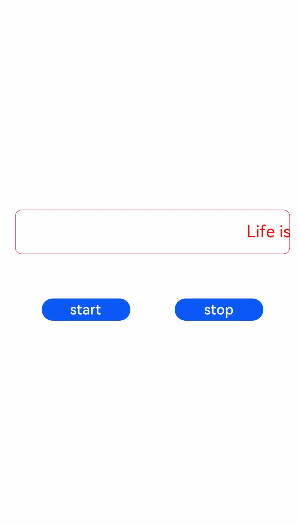
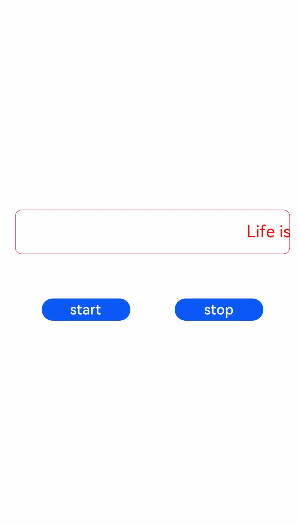
86.0 KB
58.9 KB
350.3 KB
53.0 KB
279.8 KB
97.9 KB
194.3 KB
171.1 KB
96.2 KB
665.6 KB
50.3 KB
413.6 KB
364.2 KB
72.5 KB