Merge branch 'PaddlePaddle:dygraph' into dygraph
Showing
55.4 KB
doc/table/layout.jpg
0 → 100644
671.5 KB
doc/table/ppstructure.GIF
0 → 100644
2.5 MB

| W: | H:
| W: | H:



| W: | H:
| W: | H:


doc/table/table.jpg
0 → 100644
24.1 KB
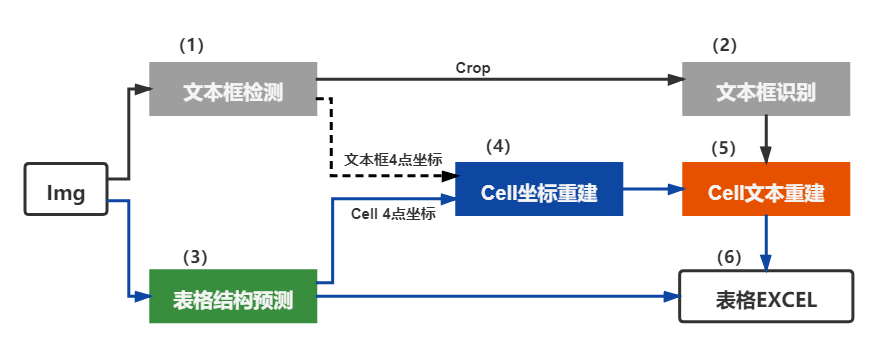
| W: | H:
| W: | H:
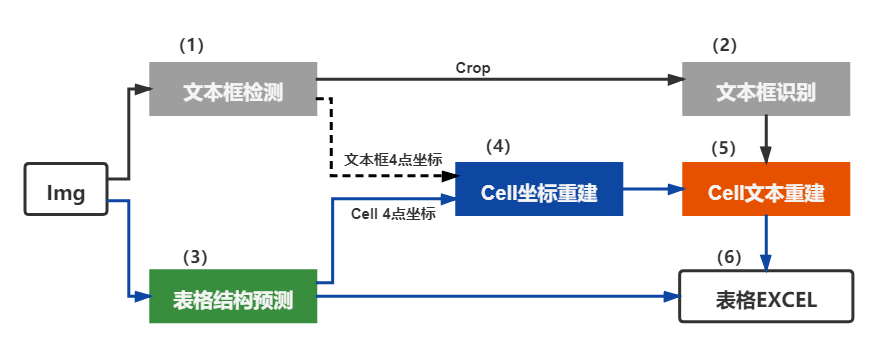
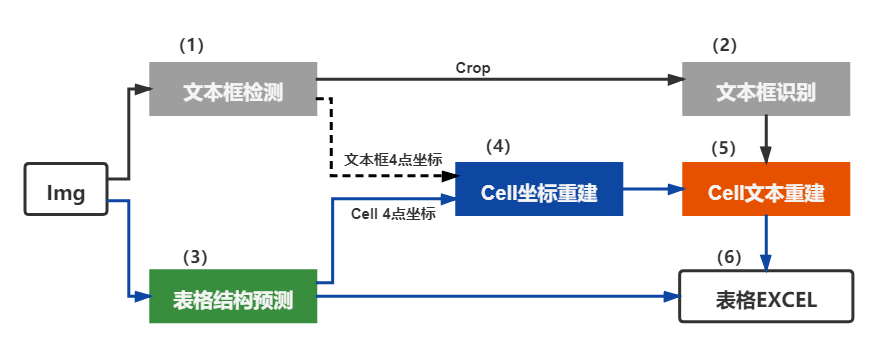
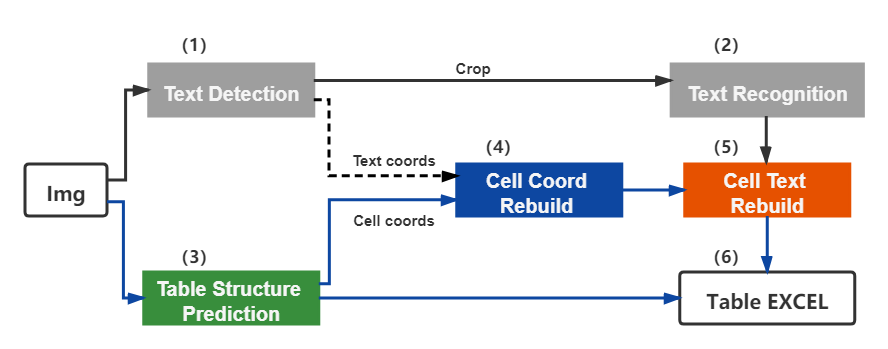
| W: | H:
| W: | H:
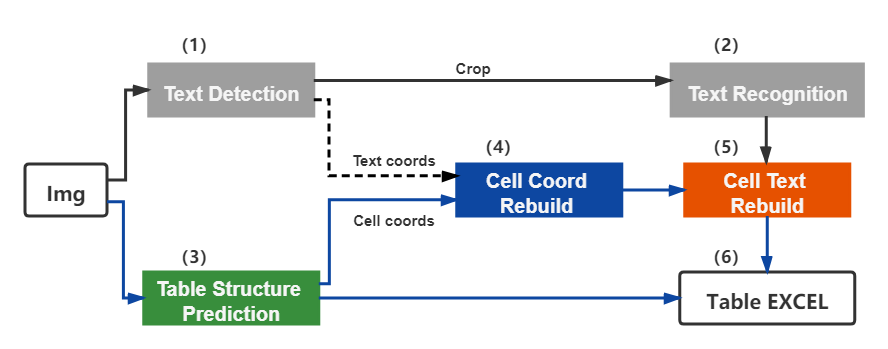
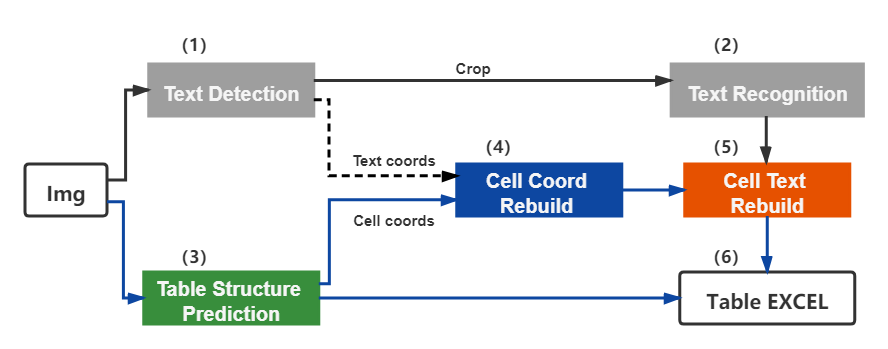
ppstructure/MANIFEST.in
已删除
100644 → 0
ppstructure/layout/README_ch.md
0 → 100644
ppstructure/setup.py
已删除
100644 → 0
test/ocr_rec_params.txt
已删除
100644 → 0
test/prepare.sh
已删除
100644 → 0
test/test.sh
已删除
100644 → 0
tests/prepare.sh
0 → 100644
tests/test.sh
0 → 100644