- 25 2月, 2022 1 次提交
-
-
由 Michael Woerister 提交于
-
- 21 2月, 2022 5 次提交
-
-
由 Michael Woerister 提交于
The previous implementation was written before types were properly normalized for code generation and had to assume a more complicated relationship between types and their debuginfo -- generating separate identifiers for debuginfo nodes that were based on normalized types. Since types are now already normalized, we can use them as identifiers for debuginfo nodes.
-
由 bors 提交于
Remove SimpleDefKind Now that rustc_query_system depends on rustc_hir, we can just directly make use of the regular DefKind.
-
由 bors 提交于
Improve `unused_unsafe` lint I’m going to add some motivation and explanation below, particularly pointing the changes in behavior from this PR. _Edit:_ Looking for existing issues, looks like this PR fixes #88260. _Edit2:_ Now also contains code that closes #90776.
-
由 Frank Steffahn 提交于
Main motivation: Fixes some issues with the current behavior. This PR is more-or-less completely re-implementing the unused_unsafe lint; it’s also only done in the MIR-version of the lint, the set of tests for the `-Zthir-unsafeck` version no longer succeeds (and is thus disabled, see `lint-unused-unsafe.rs`). On current nightly, ```rs unsafe fn unsf() {} fn inner_ignored() { unsafe { #[allow(unused_unsafe)] unsafe { unsf() } } } ``` doesn’t create any warnings. This situation is not unrealistic to come by, the inner `unsafe` block could e.g. come from a macro. Actually, this PR even includes removal of one unused `unsafe` in the standard library that was missed in a similar situation. (The inner `unsafe` coming from an external macro hides the warning, too.) The reason behind this problem is how the check currently works: * While generating MIR, it already skips nested unsafe blocks (i.e. unsafe nested in other unsafe) so that the inner one is always the one considered unused * To differentiate the cases of no unsafe operations inside the `unsafe` vs. a surrounding `unsafe` block, there’s some ad-hoc magic walking up the HIR to look for surrounding used `unsafe` blocks. There’s a lot of problems with this approach besides the one presented above. E.g. the MIR-building uses checks for `unsafe_op_in_unsafe_fn` lint to decide early whether or not `unsafe` blocks in an `unsafe fn` are redundant and ought to be removed. ```rs unsafe fn granular_disallow_op_in_unsafe_fn() { unsafe { #[deny(unsafe_op_in_unsafe_fn)] { unsf(); } } } ``` ``` error: call to unsafe function is unsafe and requires unsafe block (error E0133) --> src/main.rs:13:13 | 13 | unsf(); | ^^^^^^ call to unsafe function | note: the lint level is defined here --> src/main.rs:11:16 | 11 | #[deny(unsafe_op_in_unsafe_fn)] | ^^^^^^^^^^^^^^^^^^^^^^ = note: consult the function's documentation for information on how to avoid undefined behavior warning: unnecessary `unsafe` block --> src/main.rs:10:5 | 9 | unsafe fn granular_disallow_op_in_unsafe_fn() { | --------------------------------------------- because it's nested under this `unsafe` fn 10 | unsafe { | ^^^^^^ unnecessary `unsafe` block | = note: `#[warn(unused_unsafe)]` on by default ``` Here, the intermediate `unsafe` was ignored, even though it contains a unsafe operation that is not allowed to happen in an `unsafe fn` without an additional `unsafe` block. Also closures were problematic and the workaround/algorithms used on current nightly didn’t work properly. (I skipped trying to fully understand what it was supposed to do, because this PR uses a completely different approach.) ```rs fn nested() { unsafe { unsafe { unsf() } } } ``` ``` warning: unnecessary `unsafe` block --> src/main.rs:10:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block 10 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block | = note: `#[warn(unused_unsafe)]` on by default ``` vs ```rs fn nested() { let _ = || unsafe { let _ = || unsafe { unsf() }; }; } ``` ``` warning: unnecessary `unsafe` block --> src/main.rs:9:16 | 9 | let _ = || unsafe { | ^^^^^^ unnecessary `unsafe` block | = note: `#[warn(unused_unsafe)]` on by default warning: unnecessary `unsafe` block --> src/main.rs:10:20 | 10 | let _ = || unsafe { unsf() }; | ^^^^^^ unnecessary `unsafe` block ``` *note that this warning kind-of suggests that **both** unsafe blocks are redundant* -------------------------------------------------------------------------------- I also dislike the fact that it always suggests keeping the outermost `unsafe`. E.g. for ```rs fn granularity() { unsafe { unsafe { unsf() } unsafe { unsf() } unsafe { unsf() } } } ``` I prefer if `rustc` suggests removing the more-course outer-level `unsafe` instead of the fine-grained inner `unsafe` blocks, which it currently does on nightly: ``` warning: unnecessary `unsafe` block --> src/main.rs:10:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block 10 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block | = note: `#[warn(unused_unsafe)]` on by default warning: unnecessary `unsafe` block --> src/main.rs:11:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block 10 | unsafe { unsf() } 11 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block warning: unnecessary `unsafe` block --> src/main.rs:12:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block ... 12 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block ``` -------------------------------------------------------------------------------- Needless to say, this PR addresses all these points. For context, as far as my understanding goes, the main advantage of skipping inner unsafe blocks was that a test case like ```rs fn top_level_used() { unsafe { unsf(); unsafe { unsf() } unsafe { unsf() } unsafe { unsf() } } } ``` should generate some warning because there’s redundant nested `unsafe`, however every single `unsafe` block _does_ contain some statement that uses it. Of course this PR doesn’t aim change the warnings on this kind of code example, because the current behavior, warning on all the inner `unsafe` blocks, makes sense in this case. As mentioned, during MIR building all the unsafe blocks *are* kept now, and usage is attributed to them. The way to still generate a warning like ``` warning: unnecessary `unsafe` block --> src/main.rs:11:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block 10 | unsf(); 11 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block | = note: `#[warn(unused_unsafe)]` on by default warning: unnecessary `unsafe` block --> src/main.rs:12:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block ... 12 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block warning: unnecessary `unsafe` block --> src/main.rs:13:9 | 9 | unsafe { | ------ because it's nested under this `unsafe` block ... 13 | unsafe { unsf() } | ^^^^^^ unnecessary `unsafe` block ``` in this case is by emitting a `unused_unsafe` warning for all of the `unsafe` blocks that are _within a **used** unsafe block_. The previous code had a little HIR traversal already anyways to collect a set of all the unsafe blocks (in order to afterwards determine which ones are unused afterwards). This PR uses such a traversal to do additional things including logic like _always_ warn for an `unsafe` block that’s inside of another **used** unsafe block. The traversal is expanded to include nested closures in the same go, this simplifies a lot of things. The whole logic around `unsafe_op_in_unsafe_fn` is a little complicated, there’s some test cases of corner-cases in this PR. (The implementation involves differentiating between whether a used unsafe block was used exclusively by operations where `allow(unsafe_op_in_unsafe_fn)` was active.) The main goal was to make sure that code should compile successfully if all the `unused_unsafe`-warnings are addressed _simultaneously_ (by removing the respective `unsafe` blocks) no matter how complicated the patterns of `unsafe_op_in_unsafe_fn` being disallowed and allowed throughout the function are. -------------------------------------------------------------------------------- One noteworthy design decision I took here: An `unsafe` block with `allow(unused_unsafe)` **is considered used** for the purposes of linting about redundant contained unsafe blocks. So while ```rs fn granularity() { unsafe { //~ ERROR: unnecessary `unsafe` block unsafe { unsf() } unsafe { unsf() } unsafe { unsf() } } } ``` warns for the outer `unsafe` block, ```rs fn top_level_ignored() { #[allow(unused_unsafe)] unsafe { #[deny(unused_unsafe)] { unsafe { unsf() } //~ ERROR: unnecessary `unsafe` block unsafe { unsf() } //~ ERROR: unnecessary `unsafe` block unsafe { unsf() } //~ ERROR: unnecessary `unsafe` block } } } ``` warns on the inner ones.
-
由 bors 提交于
Move ty::print methods to Drop-based scope guards Primary goal is reducing codegen of the TLS access for each closure, which shaves ~3 seconds of bootstrap time over rustc as a whole.
-
- 20 2月, 2022 26 次提交
-
-
由 bors 提交于
Allow inlining of `ensure_sufficient_stack()` This functions is monomorphized a lot and allowing the compiler to inline it improves instructions count and max RSS significantly in my local tests.
-
由 bors 提交于
Put crate metadata first in the rlib This should make metadata lookup faster Fixes https://github.com/rust-lang/rust/issues/93806
-
由 bors 提交于
rustdoc: resolve intra-doc links when checking HTML Similar to #86451 CC #67799 Given this test case: ```rust #![warn(rustdoc::invalid_html_tags)] #![warn(rustdoc::broken_intra_doc_links)] pub struct ExistentStruct<T>(T); /// This [test][ExistentStruct<i32>] thing! pub struct NoError; ``` This pull request silences the following, spurious warning: ```text warning: unclosed HTML tag `i32` --> test.rs:6:31 | 6 | /// This [test][ExistentStruct<i32>] thing! | ^^^^^ | note: the lint level is defined here --> test.rs:1:9 | 1 | #![warn(rustdoc::invalid_html_tags)] | ^^^^^^^^^^^^^^^^^^^^^^^^^^ help: try marking as source code | 6 | /// This [test][`ExistentStruct<i32>`] thing! | + + warning: 1 warning emitted ```
-
由 bors 提交于
Extend uninhabited enum variant branch elimination to also affect fallthrough The `uninhabited_enum_branching` mir opt eliminates branches on variants where the data is uninhabited. This change extends this pass to also ensure that the `otherwise` case points to a trivially unreachable bb if all inhabited variants are present in the non-otherwise branches. I believe it was `@scottmcm` who said that LLVM eliminates some of this information in its SimplifyCFG pass. This is unfortunate, but this change should still be at least a small improvement in principle (I don't think it will show up on any benchmarks)
-
由 bors 提交于
Rollup of 14 pull requests Successful merges: - #93580 (Stabilize pin_static_ref.) - #93639 (Release notes for 1.59) - #93686 (core: Implement ASCII trim functions on byte slices) - #94002 (rustdoc: Avoid duplicating macros in sidebar) - #94019 (removing architecture requirements for RustyHermit) - #94023 (adapt static-nobundle test to use llvm-nm) - #94091 (Fix rustdoc const computed value) - #94093 (Fix pretty printing of enums without variants) - #94097 (Add module-level docs for `rustc_middle::query`) - #94112 (Optimize char_try_from_u32) - #94113 (document rustc_middle::mir::Field) - #94122 (Fix miniz_oxide types showing up in std docs) - #94142 (rustc_typeck: adopt let else in more places) - #94146 (Adopt let else in more places) Failed merges: r? `@ghost` `@rustbot` modify labels: rollup
-
由 Matthias Krüger 提交于
Adopt let else in more places Continuation of #89933, #91018, #91481, #93046, #93590, #94011. I have extended my clippy lint to also recognize tuple passing and match statements. The diff caused by fixing it is way above 1 thousand lines. Thus, I split it up into multiple pull requests to make reviewing easier. This is the biggest of these PRs and handles the changes outside of rustdoc, rustc_typeck, rustc_const_eval, rustc_trait_selection, which were handled in PRs #94139, #94142, #94143, #94144.
-
由 Matthias Krüger 提交于
rustc_typeck: adopt let else in more places Continuation of https://github.com/rust-lang/rust/pull/89933, https://github.com/rust-lang/rust/pull/91018, https://github.com/rust-lang/rust/pull/91481, https://github.com/rust-lang/rust/pull/93046, https://github.com/rust-lang/rust/pull/93590, https://github.com/rust-lang/rust/pull/94011. I have extended my clippy lint to also recognize tuple passing and match statements. The diff caused by fixing it is way above 1 thousand lines. Thus, I split it up into multiple pull requests to make reviewing easier. This PR handles rustc_typeck.
-
由 Matthias Krüger 提交于
Fix miniz_oxide types showing up in std docs Fixes #90526. Thanks to ```````@camelid,``````` I rediscovered `doc(masked)`, allowing us to prevent `miniz_oxide` type to show up in std docs. r? ```````@notriddle```````
-
由 Matthias Krüger 提交于
document rustc_middle::mir::Field cc #94025
-
由 Matthias Krüger 提交于
Optimize char_try_from_u32 The optimization was proposed by ```````@falk-hueffner``````` in https://rust-lang.zulipchat.com/#narrow/stream/219381-t-libs/topic/Micro-optimizing.20char.3A.3Afrom_u32/near/272146171, and I simplified it a bit and added an explanation of why the optimization is correct. The generated code is 2 instructions shorter and uses 2 registers instead of 4 on x86.
-
由 Matthias Krüger 提交于
Add module-level docs for `rustc_middle::query`
-
由 Matthias Krüger 提交于
Fix pretty printing of enums without variants 92d20c4a removed no-variants special case from `try_destructure_const` with expectation that this case would be handled gracefully when `read_discriminant` returns an error. Alas in that case `read_discriminant` succeeds while returning a non-existing variant, so the special case is still necessary. Fixes #94073. r? ````@oli-obk````
-
由 Matthias Krüger 提交于
Fix rustdoc const computed value Fixes #85088. It looks like this now (instead of hexadecimal): 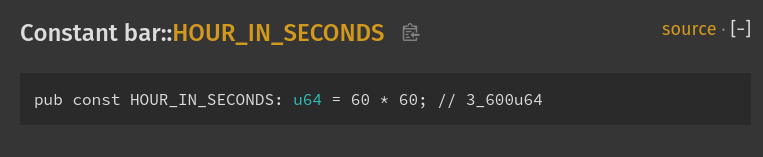 r? ````@oli-obk````
-
由 Matthias Krüger 提交于
adapt static-nobundle test to use llvm-nm No functional changes intended. This updates the test case to use llvm-nm instead of the system nm. This fixes an instance over at the experimental build of rustc with HEAD LLVM: https://buildkite.com/llvm-project/rust-llvm-integrate-prototype/builds/8380#ef6f41b5-8595-49a6-be37-0eff80e0ccb5 It is related to https://github.com/rust-lang/rust/pull/94001. The issue is that this test uses the system nm, which may not be recent enough to understand the update to uwtable. This replaces the test to use the llvm-nm that should be recent enough (consistent with the LLVM sources we use to build rustc).
-
由 Matthias Krüger 提交于
removing architecture requirements for RustyHermit RustHermit and HermitCore is able to run on aarch64 and x86_64. In the future these operating systems will also support RISC-V. Consequently, the dependency to a specific target should be removed. The build process of `hermit-abi` fails if the architecture isn't supported.
-
由 Matthias Krüger 提交于
rustdoc: Avoid duplicating macros in sidebar Fixes #93912. cc ``````@jsha`````` (for the GUI test) r? ``````@camelid``````
-
由 Matthias Krüger 提交于
core: Implement ASCII trim functions on byte slices Hi ````````@rust-lang/libs!```````` This is a feature that I wished for when implementing serial protocols with microcontrollers. Often these protocols may contain leading or trailing whitespace, which needs to be removed. Because oftentimes drivers will operate on the byte level, decoding to unicode and checking for unicode whitespace is unnecessary overhead. This PR adds three new methods to byte slices: - `trim_ascii_start` - `trim_ascii_end` - `trim_ascii` I did not find any pre-existing discussions about this, which surprises me a bit. Maybe I'm missing something, and this functionality is already possible through other means? There's https://github.com/rust-lang/rfcs/issues/2547 ("Trim methods on slices"), but that has a different purpose. As per the [std dev guide](https://std-dev-guide.rust-lang.org/feature-lifecycle/new-unstable-features.html), this is a proposed implementation without any issue / RFC. If this is the wrong process, please let me know. However, I thought discussing code is easier than discussing a mere idea, and hacking on the stdlib was fun. Tracking issue: https://github.com/rust-lang/rust/issues/94035
-
由 Matthias Krüger 提交于
Release notes for 1.59 cc `@rust-lang/release` r? `@cuviper`
-
由 Matthias Krüger 提交于
Stabilize pin_static_ref. FCP finished here: https://github.com/rust-lang/rust/issues/78186#issuecomment-1024987221 Closes #78186
-
由 bors 提交于
Guard against unwinding in cleanup code Currently the only safe guard we have against double unwind is the panic count (which is local to Rust). When double unwinds indeed happen (e.g. C++ exception + Rust panic, or two C++ exceptions), then the second unwind actually goes through and the first unwind is leaked. This can cause UB. cc rust-lang/project-ffi-unwind#6 E.g. given the following C++ code: ```c++ extern "C" void foo() { throw "A"; } extern "C" void execute(void (*fn)()) { try { fn(); } catch(...) { } } ``` This program is well-defined to terminate: ```c++ struct dtor { ~dtor() noexcept(false) { foo(); } }; void a() { dtor a; dtor b; } int main() { execute(a); return 0; } ``` But this Rust code doesn't catch the double unwind: ```rust extern "C-unwind" { fn foo(); fn execute(f: unsafe extern "C-unwind" fn()); } struct Dtor; impl Drop for Dtor { fn drop(&mut self) { unsafe { foo(); } } } extern "C-unwind" fn a() { let _a = Dtor; let _b = Dtor; } fn main() { unsafe { execute(a) }; } ``` To address this issue, this PR adds an unwind edge to an abort block, so that the Rust example aborts. This is similar to how clang guards against double unwind (except clang calls terminate per C++ spec and we abort). The cost should be very small; it's an additional trap instruction (well, two for now, since we use TrapUnreachable, but that's a different issue) for each function with landing pads; if LLVM gains support to encode "abort/terminate" info directly in LSDA like GCC does, then it'll be free. It's an additional basic block though so compile time may be worse, so I'd like a perf run. r? `@ghost` `@rustbot` label: F-c_unwind
-
由 bors 提交于
Bump version to 1.61 r? `@Mark-Simulacrum`
-
由 Mark Rousskov 提交于
-
由 Gary Guo 提交于
-
由 est31 提交于
-
由 est31 提交于
-
由 Tomasz Miąsko 提交于
92d20c4a removed no-variants special case from try_destructure_const with expectation that this case would be handled gracefully when read_discriminant returns an error. Alas in that case read_discriminant succeeds while returning a non-existing variant, so the special case is still necessary.
-
- 19 2月, 2022 8 次提交
-
-
由 Guillaume Gomez 提交于
-
由 Guillaume Gomez 提交于
-
由 bors 提交于
Rollup of 7 pull requests Successful merges: - #92902 (Improve the documentation of drain members) - #93658 (Stabilize `#[cfg(panic = "...")]`) - #93954 (rustdoc-json: buffer output) - #93979 (Add debug assertions to validate NUL terminator in c strings) - #93990 (pre #89862 cleanup) - #94006 (Use a `Field` in `ConstraintCategory::ClosureUpvar`) - #94086 (Fix ScalarInt to char conversion) Failed merges: r? `@ghost` `@rustbot` modify labels: rollup
-
由 Matthias Krüger 提交于
Fix ScalarInt to char conversion to avoid panic for invalid Unicode scalar values
-
由 Matthias Krüger 提交于
Use a `Field` in `ConstraintCategory::ClosureUpvar` As part of #90317, we do not want `HirId` to implement `Ord`, `PartialOrd`. This line of code has made that difficult https://github.com/rust-lang/rust/blob/1b27144afc77031ba9c05d86c06c64485589775a/compiler/rustc_borrowck/src/region_infer/mod.rs#L2184 since it sorts a [`ConstraintCategory::ClosureUpvar(HirId)`](https://doc.rust-lang.org/nightly/nightly-rustc/rustc_middle/mir/enum.ConstraintCategory.html#variant.ClosureUpvar). This PR makes that variant take a [`Field`](https://doc.rust-lang.org/nightly/nightly-rustc/rustc_middle/mir/struct.Field.html) instead. r? `@nikomatsakis`
-
由 Matthias Krüger 提交于
pre #89862 cleanup changes used in #89862 which can be landed without the rest of this PR being finished. r? `@estebank`
-
由 Matthias Krüger 提交于
Add debug assertions to validate NUL terminator in c strings The `unchecked` variants from the stdlib usually perform the check anyway if debug assertions are on (for example, `unwrap_unchecked`). This PR does the same thing for `CStr` and `CString`, validating the correctness for the NUL byte in debug mode.
-
由 Matthias Krüger 提交于
rustdoc-json: buffer output It turns out we were doing syscalls for each part of the json syntax Before: ``` ... [pid 1801267] write(5, "\"", 1) = 1 [pid 1801267] write(5, ",", 1) = 1 [pid 1801267] write(5, "\"", 1) = 1 ... ``` After: ``` [pid 1974821] write(5, "{\"root\":\"0:0\",\"crate_version\":nu"..., 1575) = 1575 ``` In one benchmark (one struct, almost all time in `std`), this gives ~2x perf r? `@CraftSpider` `@rustbot` modify labels: +A-rustdoc-json +T-rustdoc -A-testsuite
-