Skip to content
体验新版
项目
组织
正在加载...
登录
切换导航
打开侧边栏
CSDN 技术社区
skill_tree_dailycode
提交
12dafb7c
S
skill_tree_dailycode
项目概览
CSDN 技术社区
/
skill_tree_dailycode
通知
11
Star
4
Fork
1
代码
文件
提交
分支
Tags
贡献者
分支图
Diff
Issue
2
列表
看板
标记
里程碑
合并请求
0
DevOps
流水线
流水线任务
计划
Wiki
0
Wiki
分析
仓库
DevOps
项目成员
Pages
S
skill_tree_dailycode
项目概览
项目概览
详情
发布
仓库
仓库
文件
提交
分支
标签
贡献者
分支图
比较
Issue
2
Issue
2
列表
看板
标记
里程碑
合并请求
0
合并请求
0
Pages
DevOps
DevOps
流水线
流水线任务
计划
分析
分析
仓库分析
DevOps
Wiki
0
Wiki
成员
成员
收起侧边栏
关闭侧边栏
动态
分支图
创建新Issue
流水线任务
提交
Issue看板
提交
12dafb7c
编写于
12月 13, 2021
作者:
每日一练社区
浏览文件
操作
浏览文件
下载
电子邮件补丁
差异文件
update 17 exercises
上级
851f5ccf
变更
19
隐藏空白更改
内联
并排
Showing
19 changed file
with
1113 addition
and
247 deletion
+1113
-247
.gitignore
.gitignore
+2
-1
data/1.dailycode初阶/3.python/1.exercises/solution.md
data/1.dailycode初阶/3.python/1.exercises/solution.md
+51
-5
data/1.dailycode初阶/3.python/2.exercises/solution.md
data/1.dailycode初阶/3.python/2.exercises/solution.md
+38
-4
data/1.dailycode初阶/3.python/3.exercises/solution.md
data/1.dailycode初阶/3.python/3.exercises/solution.md
+24
-4
data/1.dailycode初阶/3.python/4.exercises/solution.md
data/1.dailycode初阶/3.python/4.exercises/solution.md
+50
-4
data/2.dailycode中阶/3.python/1.exercises/solution.md
data/2.dailycode中阶/3.python/1.exercises/solution.md
+37
-14
data/2.dailycode中阶/3.python/2.exercises/solution.md
data/2.dailycode中阶/3.python/2.exercises/solution.md
+47
-12
data/3.dailycode高阶/3.python/10.exercises/solution.md
data/3.dailycode高阶/3.python/10.exercises/solution.md
+41
-4
data/3.dailycode高阶/3.python/11.exercises/solution.md
data/3.dailycode高阶/3.python/11.exercises/solution.md
+60
-4
data/3.dailycode高阶/3.python/12.exercises/solution.md
data/3.dailycode高阶/3.python/12.exercises/solution.md
+48
-4
data/3.dailycode高阶/3.python/13.exercises/solution.md
data/3.dailycode高阶/3.python/13.exercises/solution.md
+89
-4
data/3.dailycode高阶/3.python/14.exercises/solution.md
data/3.dailycode高阶/3.python/14.exercises/solution.md
+53
-4
data/3.dailycode高阶/3.python/15.exercises/solution.md
data/3.dailycode高阶/3.python/15.exercises/solution.md
+42
-4
data/3.dailycode高阶/3.python/16.exercises/solution.md
data/3.dailycode高阶/3.python/16.exercises/solution.md
+85
-34
data/3.dailycode高阶/3.python/17.exercises/solution.md
data/3.dailycode高阶/3.python/17.exercises/solution.md
+104
-39
data/3.dailycode高阶/3.python/18.exercises/solution.md
data/3.dailycode高阶/3.python/18.exercises/solution.md
+178
-65
data/3.dailycode高阶/3.python/19.exercises/solution.md
data/3.dailycode高阶/3.python/19.exercises/solution.md
+83
-33
data/3.dailycode高阶/3.python/20.exercises/solution.md
data/3.dailycode高阶/3.python/20.exercises/solution.md
+49
-4
data/3.dailycode高阶/3.python/21.exercises/solution.md
data/3.dailycode高阶/3.python/21.exercises/solution.md
+32
-4
未找到文件。
.gitignore
浏览文件 @
12dafb7c
...
...
@@ -8,4 +8,5 @@ __pycache__
helper.py
test.md
data_source/dailycode
test.cpp
\ No newline at end of file
test.cpp
test.py
\ No newline at end of file
data/1.dailycode初阶/3.python/1.exercises/solution.md
浏览文件 @
12dafb7c
# 找出素数对
任意输入一个大于10的偶数编程找出所有和等于该偶数的素数对
任意输入一个大于10的偶数,编程找出所有和等于该偶数的素数对
以下程序实现了这一功能,请你填补空白处内容:
```
python
h
=
0
def
a
(
h
):
x
=
0
for
j
in
range
(
2
,
h
):
if
h
%
j
==
0
:
x
=
1
break
if
x
==
0
:
return
1
n
=
int
(
input
(
"输入任意大于10的偶数:"
))
for
i
in
range
(
n
,
n
+
1
):
h
=
0
if
i
%
2
==
0
:
for
k
in
range
(
2
,
i
):
if
a
(
k
)
==
1
and
a
(
i
-
k
)
==
1
:
________________
```
## template
...
...
@@ -32,7 +53,13 @@ for i in range(n,n+1):
## 答案
```
python
h
=
1
if
h
==
0
:
print
(
"%d can't"
%
i
)
break
else
:
print
(
"%d=%d+%d"
%
(
i
,
k
,
i
-
k
))
break
```
## 选项
...
...
@@ -40,17 +67,35 @@ for i in range(n,n+1):
### A
```
python
h
=
1
if
h
==
1
:
print
(
"%d can't"
%
i
)
break
else
:
print
(
"%d=%d+%d"
%
(
i
,
k
,
i
-
k
))
break
```
### B
```
python
h
=
1
if
h
==
1
:
print
(
"%d can't"
%
i
)
continue
else
:
print
(
"%d=%d+%d"
%
(
i
,
k
,
i
-
k
))
continue
```
### C
```
python
h
=
1
if
h
==
0
:
print
(
"%d can't"
%
i
)
continue
else
:
print
(
"%d=%d+%d"
%
(
i
,
k
,
i
-
k
))
continue
```
\ No newline at end of file
data/1.dailycode初阶/3.python/2.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,27 @@
<p>
输入一个正数n
,
计算出因子里面分别有几个4和7
,
输出因子中4和7的个位数
</p>
以下程序实现了这一功能,请你填补空白处内容:
```
python
n
=
int
(
input
(
"输入数字:"
))
factor
=
[
n
]
num
=
1
__________________
print
(
factor
)
m
=
[
str
(
i
)
for
i
in
factor
]
count4
=
0
count7
=
0
for
i
in
m
:
if
'4'
in
i
:
count4
+=
1
print
(
'以4结尾的因子的个位数:'
,
int
(
i
)
%
10
)
if
'7'
in
i
:
count7
+=
1
print
(
'以7结尾的因子的个位数:'
,
int
(
i
)
%
10
)
print
(
'因子里面分别有{0}个4和{1}个7'
.
format
(
count4
,
count7
))
```
## template
```
python
...
...
@@ -29,7 +50,10 @@ print('因子里面分别有{0}个4和{1}个7'.format(count4,count7))
## 答案
```
python
while
num
<=
n
/
2
+
1
:
if
n
%
num
==
0
:
factor
.
append
(
num
)
num
=
num
+
1
```
## 选项
...
...
@@ -37,17 +61,26 @@ print('因子里面分别有{0}个4和{1}个7'.format(count4,count7))
### A
```
python
while
num
<=
n
+
1
:
if
n
%
num
==
0
:
factor
.
append
(
num
)
num
=
num
+
1
```
### B
```
python
while
num
<=
n
+
1
:
if
n
%
num
==
1
:
factor
.
append
(
num
)
num
=
num
+
1
```
### C
```
python
while
num
<=
n
/
2
+
1
:
if
n
%
num
==
1
:
factor
.
append
(
num
)
num
=
num
+
1
```
\ No newline at end of file
data/1.dailycode初阶/3.python/3.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,21 @@
<p>
计算圆周率。存在圆心在直角坐标系原点且半径为 1 的圆及其外切正方形。为计算方便
,
仅考虑位于第一象限的四分之一正方形和四分之一圆。随机生成该四分之一正方形中一系列点
,
散布于四分之一圆内比例即为圆周率四分之一。散步点越多
,
结果越精确
,
耗时也越长。
</p>
以下程序实现了这一功能,请你填补空白处内容:
```
python
from
random
import
random
from
math
import
sqrt
N
=
eval
(
input
(
"请输入次数:"
))
K
=
0
for
i
in
range
(
1
,
N
+
1
):
x
,
y
=
random
(),
random
()
dist
=
sqrt
(
x
**
2
+
y
**
2
)
_____________________
pi
=
4
*
(
K
/
N
)
print
(
"圆周率值:{}"
.
format
(
pi
))
```
## template
```
python
...
...
@@ -21,7 +36,8 @@ print("圆周率值:{}".format(pi))
## 答案
```
python
if
dist
<=
1.0
:
K
=
K
+
1
```
## 选项
...
...
@@ -29,17 +45,20 @@ print("圆周率值:{}".format(pi))
### A
```
python
if
dist
>=
1.0
:
K
=
K
+
1
```
### B
```
python
if
dist
>
1.0
:
K
=
K
+
1
```
### C
```
python
if
dist
==
1.0
:
K
=
K
+
1
```
\ No newline at end of file
data/1.dailycode初阶/3.python/4.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,23 +2,56 @@
<p>
【问题描述】
输入一个列表
,
包含若干个整数
(
允许为空
),
然后将其中的奇数和偶数单独放置在一个列表中
,
保持原有顺序
【输入形式】
【输出形式】
分两行输出
,
第一行输出偶数序列
,
第二行输出奇数序列
【样例输入1】
[48,82,47,54,55,57,27,73,86,14]
【样例输出1】
48, 82, 54, 86, 14
47, 55, 57, 27, 73
【样例输入2】
[10, 22, 40]
【样例输出2】
10, 22, 40
NONE
【样例说明】
如果奇偶拆分后
,
奇数列表
,
或者偶数列表为空
,
请直接输出NONE表示
</p>
以下程序实现了这一功能,请你填补空白处内容:
```
python
x
=
input
()
x1
=
x
.
strip
(
'[]'
)
x2
=
x1
.
split
(
","
)
a
=
[]
b
=
[]
for
i
in
x2
:
______________
if
a
==
[]:
print
(
"NONE"
)
else
:
print
(
a
)
if
b
==
[]:
print
(
"NONE"
)
else
:
print
(
b
)
```
## template
```
python
...
...
@@ -45,7 +78,10 @@ else:
## 答案
```
python
if
int
(
i
)
%
2
==
0
:
a
.
append
(
i
)
else
:
b
.
append
(
i
)
```
## 选项
...
...
@@ -53,17 +89,26 @@ else:
### A
```
python
if
i
%
2
==
0
:
a
.
append
(
i
)
else
:
b
.
append
(
i
)
```
### B
```
python
if
int
(
i
)
%
2
==
1
:
a
.
append
(
i
)
else
:
b
.
append
(
i
)
```
### C
```
python
if
i
%
2
==
1
:
a
.
append
(
i
)
else
:
b
.
append
(
i
)
```
\ No newline at end of file
data/2.dailycode中阶/3.python/1.exercises/solution.md
浏览文件 @
12dafb7c
# 将一组数尽可能均匀地分成两堆,使两个堆中的数的和尽可能相等
<p>
麦克叔叔去世了,他在遗嘱中给他的两个孙子阿贝和鲍勃留下了一堆珍贵的口袋妖怪卡片。遗嘱中唯一的方向是“尽可能均匀地分配纸牌的价值”。作为Mike遗嘱的执行人,你已经为每一张口袋妖怪卡片定价,以获得准确的货币价值。你要决定如何将口袋妖怪卡片分成两堆,以尽量减少每一堆卡片的价值总和的差异。
例如,你有下列n=8 个口袋妖怪卡片:

经过大量的工作,你发现你可以用下面的方法来划分卡片:
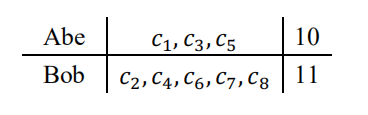
这给了安倍10美元的牌给了鲍勃11美元的牌。这是最好的除法吗?
你要做的是解决n张牌的问题其中每张牌ci都有一个正整数值vi.你的解决方法是计算牌应该如何被分割以及每摞牌的价值。
输入输出示例如下:
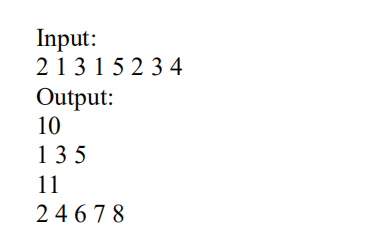
1.
通过检查所有可能的桩以蛮力解决此问题。 对这种蛮力算法的时间复杂度进行分析,并通过实施和实验验证您的分析结果(既写出来算法的设计思路等),并用python算法实现编程
<p>
麦克叔叔去世了,他在遗嘱中给他的两个孙子阿贝和鲍勃留下了一堆珍贵的口袋妖怪卡片。遗嘱中唯一的方向是“尽可能均匀地分配纸牌的价值”。作为Mike遗嘱的执行人,你已经为每一张口袋妖怪卡片定价,以获得准确的货币价值。你要决定如何将口袋妖怪卡片分成两堆,以尽量减少每一堆卡片的价值总和的差异。
<br
/>
例如,你有下列n=8 个口袋妖怪卡片:
<br
/>

<br
/>
经过大量的工作,你发现你可以用下面的方法来划分卡片:
<br
/>
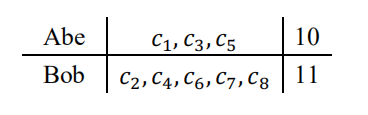
<br
/>
这给了安倍10美元的牌给了鲍勃11美元的牌。这是最好的除法吗?
<br
/>
你要做的是解决n张牌的问题其中每张牌ci都有一个正整数值vi.你的解决方法是计算牌应该如何被分割以及每摞牌的价值。
<br
/>
输入输出示例如下:
<br
/>
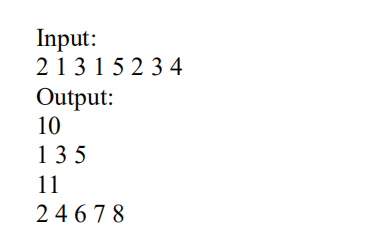
<br
/>
1.
通过检查所有可能的桩以蛮力解决此问题。 对这种蛮力算法的时间复杂度进行分析,并通过实施和实验验证您的分析结果(既写出来算法的设计思路等),并用python算法实现编程
<br
/>
2.
通过动态编程开发更有效的算法。 您应该首先通过动态编程的思想来分析此问题,并编写相应的递归属性。 对这种算法的时间复杂度进行分析,并通过实施和实验验证您的分析结果。并用python代码实现动态编程
</p>
以下程序实现了这一功能,请你填补空白处内容:
```
python
def
deal
(
data
,
flag
):
a
=
[]
for
i
in
data
:
if
i
>=
flag
:
return
[
i
]
elif
a
==
[]:
a
.
append
([
i
])
else
:
_______________________
a
.
append
([
i
])
target
=
sum
(
max
(
a
,
key
=
sum
))
return
list
(
filter
(
lambda
x
:
sum
(
x
)
==
target
,
a
))
if
__name__
==
'__main__'
:
c
=
[
2
,
1
,
3
,
1
,
5
,
2
,
3
,
4
]
flag
=
sum
(
c
)
//
2
res
=
deal
(
c
,
flag
)
print
(
res
)
```
## template
```
python
...
...
@@ -37,7 +59,7 @@ if __name__=='__main__':
## 答案
```
python
a
=
a
+
[
k
+
[
i
]
for
k
in
a
if
sum
(
k
)
+
i
<=
flag
]
```
## 选项
...
...
@@ -45,17 +67,17 @@ if __name__=='__main__':
### A
```
python
a
=
a
+
[
k
+
[
i
]
for
k
in
a
if
sum
(
k
)
+
i
>=
flag
]
```
### B
```
python
a
=
a
+
[
k
+
[
i
]
for
k
in
a
if
sum
(
k
)
+
i
>
flag
]
```
### C
```
python
a
=
a
+
[
k
+
[
i
]
for
k
in
a
if
k
+
i
<=
flag
]
```
\ No newline at end of file
data/2.dailycode中阶/3.python/2.exercises/solution.md
浏览文件 @
12dafb7c
# 求两个给定正整数的最大公约数和最小公倍数
<pre><p>
本题要求两个给定正整数的最大公约数和最小公倍数。
输入格式:
输入在两行中分别输入正整数x和y。
输出格式:
在一行中输出最大公约数和最小公倍数的值。
</pre><pre>
输入样例1:
在这里给出一组输入。
输入格式:
<br
/>
输入在两行中分别输入正整数x和y。
<br
/>
输出格式:
<br
/>
在一行中输出最大公约数和最小公倍数的值。
<br
/>
</pre><pre>
输入样例1:
<br
/>
在这里给出一组输入。
<br
/>
例如
:
</p><pre><code>
100
1520
</code></pre><p>
输出样例1:
在这里给出相应的输出。
1520
</code></pre><p>
输出样例1:
<br
/>
在这里给出相应的输出。
<br
/>
例如
:
</p><pre><code>
20 7600
</code></pre></pre>
以下程序实现了这一功能,请你填补空白处内容:
```
python
def
hcf
(
x
,
y
):
if
x
>
y
:
smaller
=
y
else
:
smaller
=
x
for
i
in
range
(
1
,
smaller
+
1
):
if
((
x
%
i
==
0
)
and
(
y
%
i
==
0
)):
hcf
=
i
return
hcf
def
lcm
(
x
,
y
):
if
x
>
y
:
greater
=
x
else
:
greater
=
y
while
(
True
):
____________________
greater
+=
1
return
lcm
num1
=
int
(
input
(
"输入第一个数字: "
))
num2
=
int
(
input
(
"输入第二个数字: "
))
print
(
"最大公约数为"
,
hcf
(
num1
,
num2
),
"最小公倍数为"
,
lcm
(
num1
,
num2
))
```
## template
```
python
...
...
@@ -43,7 +69,9 @@ print("最大公约数为",hcf(num1, num2),"最小公倍数为",lcm(num1,num2))
## 答案
```
python
if
((
greater
%
x
==
0
)
and
(
greater
%
y
==
0
)):
lcm
=
greater
break
```
## 选项
...
...
@@ -51,17 +79,23 @@ print("最大公约数为",hcf(num1, num2),"最小公倍数为",lcm(num1,num2))
### A
```
python
if
((
greater
%
x
==
1
)
and
(
greater
%
y
==
1
)):
lcm
=
greater
break
```
### B
```
python
if
((
greater
%
x
==
0
)
or
(
greater
%
y
==
0
)):
lcm
=
greater
break
```
### C
```
python
if
((
greater
%
x
==
1
)
or
(
greater
%
y
==
1
)):
lcm
=
greater
break
```
\ No newline at end of file
data/3.dailycode高阶/3.python/10.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,34 @@
<p>
给你一个只包含
<code>
'('
</code>
和
<code>
')'
</code>
的字符串,找出最长有效(格式正确且连续)括号子串的长度。
</p><p>
</p><div
class=
"original__bRMd"
><div><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
s = "(()"
<strong><br
/>
输出:
</strong>
2
<strong><br
/>
解释:
</strong>
最长有效括号子串是 "()"
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
s = ")()())"
<strong><br
/>
输出:
</strong>
4
<strong><br
/>
解释:
</strong>
最长有效括号子串是 "()()"
</pre><p><strong>
示例 3:
</strong></p><pre><strong>
输入:
</strong>
s = ""
<strong><br
/>
输出:
</strong>
0
</pre><p>
</p><p><strong>
提示:
</strong></p><ul>
<li><code>
0
<
=
s.length
<=
3
*
10<
sup
>
4
</sup></code></li>
<li><code>
s[i]
</code>
为
<code>
'('
</code>
或
<code>
')'
</code></li></ul></div></div>
以下程序实现了这一功能,请你填补空白处内容:
```
python
import
pdb
class
Solution
(
object
):
def
longestValidParentheses
(
self
,
s
):
ls
=
len
(
s
)
stack
=
[]
data
=
[
0
]
*
ls
for
i
in
range
(
ls
):
curr
=
s
[
i
]
if
curr
==
'('
:
stack
.
append
(
i
)
else
:
__________________
tep
,
res
=
0
,
0
for
t
in
data
:
if
t
==
1
:
tep
+=
1
else
:
res
=
max
(
tep
,
res
)
tep
=
0
return
max
(
tep
,
res
)
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
longestValidParentheses
(
')()())'
))
```
## template
```
python
...
...
@@ -35,7 +63,9 @@ if __name__ == '__main__':
## 答案
```
python
if
len
(
stack
)
>
0
:
data
[
i
]
=
1
data
[
stack
.
pop
(
-
1
)]
=
1
```
## 选项
...
...
@@ -43,17 +73,23 @@ if __name__ == '__main__':
### A
```
python
if
len
(
stack
)
>
0
:
data
[
i
]
=
1
data
[
stack
.
pop
(
1
)]
=
1
```
### B
```
python
if
len
(
stack
)
<
0
:
data
[
i
]
=
1
data
[
stack
.
pop
(
-
1
)]
=
1
```
### C
```
python
if
len
(
stack
)
<
0
:
data
[
i
]
=
1
data
[
stack
.
pop
(
1
)]
=
1
```
\ No newline at end of file
data/3.dailycode高阶/3.python/11.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,38 @@
<p><strong>
n 皇后问题
</strong>
研究的是如何将
<code>
n
</code>
个皇后放置在
<code>
n×n
</code>
的棋盘上,并且使皇后彼此之间不能相互攻击。
</p><p>
给你一个整数
<code>
n
</code>
,返回所有不同的
<strong>
n
<em>
</em>
皇后问题
</strong>
的解决方案。
</p><div
class=
"original__bRMd"
><div><p>
每一种解法包含一个不同的
<strong>
n 皇后问题
</strong>
的棋子放置方案,该方案中
<code>
'Q'
</code>
和
<code>
'.'
</code>
分别代表了皇后和空位。
</p><p>
</p><p><strong>
示例 1:
</strong></p><img
alt=
""
src=
"https://assets.leetcode.com/uploads/2020/11/13/queens.jpg"
style=
"width: 600px; height: 268px;"
/><pre><strong>
输入:
</strong>
n = 4
<strong><br
/>
输出:
</strong>
[[".Q..","...Q","Q...","..Q."],["..Q.","Q...","...Q",".Q.."]]
<strong><br
/>
解释:
</strong>
如上图所示,4 皇后问题存在两个不同的解法。
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
n = 1
<strong><br
/>
输出:
</strong>
[["Q"]]
</pre><p>
</p><p><strong>
提示:
</strong></p><ul>
<li><code>
1
<
=
n
<=
9</
code
></li>
<li>
皇后彼此不能相互攻击,也就是说:任何两个皇后都不能处于同一条横行、纵行或斜线上。
</li></ul></div></div>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
solveNQueens
(
self
,
n
):
if
n
==
0
:
return
0
res
=
[]
board
=
[[
'.'
]
*
n
for
t
in
range
(
n
)]
self
.
do_solveNQueens
(
res
,
board
,
n
)
return
res
def
do_solveNQueens
(
self
,
res
,
board
,
num
):
if
num
==
0
:
res
.
append
([
''
.
join
(
t
)
for
t
in
board
])
return
ls
=
len
(
board
)
pos
=
ls
-
num
check
=
[
True
]
*
ls
for
i
in
range
(
pos
):
for
j
in
range
(
ls
):
if
board
[
i
][
j
]
==
'Q'
:
______________________
for
j
in
range
(
ls
):
if
check
[
j
]:
board
[
pos
][
j
]
=
'Q'
self
.
do_solveNQueens
(
res
,
board
,
num
-
1
)
board
[
pos
][
j
]
=
'.'
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
solveNQueens
(
4
))
```
## template
```
python
...
...
@@ -43,7 +75,13 @@ if __name__ == '__main__':
## 答案
```
python
check
[
j
]
=
False
step
=
pos
-
i
if
j
+
step
<
ls
:
check
[
j
+
step
]
=
False
if
j
-
step
>=
0
:
check
[
j
-
step
]
=
False
break
```
## 选项
...
...
@@ -51,17 +89,34 @@ if __name__ == '__main__':
### A
```
python
check
[
j
]
=
False
step
=
pos
-
i
if
j
+
step
<
ls
:
check
[
j
+
step
]
=
False
if
j
-
step
>=
0
:
check
[
j
-
step
]
=
False
```
### B
```
python
check
[
j
]
=
False
step
=
pos
-
i
if
j
+
step
<
ls
:
check
[
j
+
step
]
=
False
if
j
-
step
<=
0
:
check
[
j
-
step
]
=
False
break
```
### C
```
python
check
[
j
]
=
False
step
=
pos
-
i
if
j
+
step
>
ls
:
check
[
j
+
step
]
=
False
if
j
-
step
<=
0
:
check
[
j
-
step
]
=
False
break
```
\ No newline at end of file
data/3.dailycode高阶/3.python/12.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -60,6 +60,29 @@
</ul>
</div>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
isScramble
(
self
,
s1
,
s2
,
memo
=
{}):
if
len
(
s1
)
!=
len
(
s2
)
or
sorted
(
s1
)
!=
sorted
(
s2
):
return
False
if
len
(
s1
)
<=
len
(
s2
)
<=
1
:
return
s1
==
s2
if
s1
==
s2
:
return
True
if
(
s1
,
s2
)
in
memo
:
return
memo
[
s1
,
s2
]
n
=
len
(
s1
)
for
i
in
range
(
1
,
n
):
___________________________
memo
[
s1
,
s2
]
=
False
return
False
# %%
s
=
Solution
()
print
(
s
.
isScramble
(
s1
=
"great"
,
s2
=
"rgeat"
))
```
## template
```
python
...
...
@@ -91,7 +114,12 @@ print(s.isScramble(s1 = "great", s2 = "rgeat"))
## 答案
```
python
a
=
self
.
isScramble
(
s1
[:
i
],
s2
[:
i
],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[
i
:],
memo
)
if
not
a
:
b
=
self
.
isScramble
(
s1
[:
i
],
s2
[
-
i
:],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[:
-
i
],
memo
)
if
a
or
b
:
memo
[
s1
,
s2
]
=
True
return
True
```
## 选项
...
...
@@ -99,17 +127,32 @@ print(s.isScramble(s1 = "great", s2 = "rgeat"))
### A
```
python
a
=
self
.
isScramble
(
s1
[:
i
],
s2
[:
i
],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[
i
:],
memo
)
if
not
a
:
b
=
self
.
isScramble
(
s1
[:
i
],
s2
[
i
:],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[:
i
],
memo
)
if
a
or
b
:
memo
[
s1
,
s2
]
=
True
return
True
```
### B
```
python
a
=
self
.
isScramble
(
s1
[:
i
],
s2
[:
i
-
1
],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[
i
+
1
:],
memo
)
if
not
a
:
b
=
self
.
isScramble
(
s1
[:
i
],
s2
[
-
i
:],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[:
-
i
],
memo
)
if
a
or
b
:
memo
[
s1
,
s2
]
=
True
return
True
```
### C
```
python
a
=
self
.
isScramble
(
s1
[:
i
-
1
],
s2
[:
i
],
memo
)
and
self
.
isScramble
(
s1
[
i
+
1
:],
s2
[
i
:],
memo
)
if
not
a
:
b
=
self
.
isScramble
(
s1
[:
i
],
s2
[
-
i
:],
memo
)
and
self
.
isScramble
(
s1
[
i
:],
s2
[:
-
i
],
memo
)
if
a
or
b
:
memo
[
s1
,
s2
]
=
True
return
True
```
\ No newline at end of file
data/3.dailycode高阶/3.python/13.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,66 @@
<p>给你一个链表,每 <em>k </em>个节点一组进行翻转,请你返回翻转后的链表。</p><p><em>k </em>是一个正整数,它的值小于或等于链表的长度。</p><p>如果节点总数不是 <em>k </em>的整数倍,那么请将最后剩余的节点保持原有顺序。</p><p><strong>进阶:</strong></p><ul> <li>你可以设计一个只使用常数额外空间的算法来解决此问题吗?</li> <li><strong>你不能只是单纯的改变节点内部的值</strong>,而是需要实际进行节点交换。</li></ul><p> </p><p><strong>示例 1:</strong></p><img alt="" src="https://cdn.jsdelivr.net/gh/doocs/leetcode@main/solution/0000-0099/0025.Reverse%20Nodes%20in%20k-Group/images/reverse_ex1.jpg" style="width: 542px; height: 222px;" /><pre><strong>输入:</strong>head = [1,2,3,4,5], k = 2<strong><br />输出:</strong>[2,1,4,3,5]</pre><p><strong>示例 2:</strong></p><img alt="" src="https://cdn.jsdelivr.net/gh/doocs/leetcode@main/solution/0000-0099/0025.Reverse%20Nodes%20in%20k-Group/images/reverse_ex2.jpg" style="width: 542px; height: 222px;" /><pre><strong>输入:</strong>head = [1,2,3,4,5], k = 3<strong><br />输出:</strong>[3,2,1,4,5]</pre><p><strong>示例 3:</strong></p><pre><strong>输入:</strong>head = [1,2,3,4,5], k = 1<strong><br />输出:</strong>[1,2,3,4,5]</pre><p><strong>示例 4:</strong></p><pre><strong>输入:</strong>head = [1], k = 1<strong><br />输出:</strong>[1]</pre><ul></ul><p><strong>提示:</strong></p><ul> <li>列表中节点的数量在范围 <code>sz</code> 内</li> <li><code>1 <= sz <= 5000</code></li> <li><code>0 <= Node.val <= 1000</code></li> <li><code>1 <= k <= sz</code></li></ul>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
ListNode
(
object
):
def
__init__
(
self
,
x
):
self
.
val
=
x
self
.
next
=
None
class
LinkList
:
def
__init__
(
self
):
self
.
head
=
None
def
initList
(
self
,
data
):
self
.
head
=
ListNode
(
data
[
0
])
r
=
self
.
head
p
=
self
.
head
for
i
in
data
[
1
:]:
node
=
ListNode
(
i
)
p
.
next
=
node
p
=
p
.
next
return
r
def
convert_list
(
self
,
head
):
ret
=
[]
if
head
==
None
:
return
node
=
head
while
node
!=
None
:
ret
.
append
(
node
.
val
)
node
=
node
.
next
return
ret
class
Solution
(
object
):
def
reverseKGroup
(
self
,
head
,
k
):
if
head
is
None
:
return
None
index
=
0
lead
,
last
=
0
,
0
pos
=
head
temp
=
ListNode
(
-
1
)
temp
.
next
=
head
head
=
temp
start
=
head
_________________
return
head
.
next
def
reverseList
(
self
,
head
,
end
):
pos
=
head
.
next
last
=
end
next_start
=
pos
while
pos
!=
end
:
head
.
next
=
pos
last_pos
=
pos
pos
=
pos
.
next
last_pos
.
next
=
last
last
=
last_pos
return
next_start
# %%
l
=
LinkList
()
head
=
[
1
,
2
,
3
,
4
,
5
]
l1
=
l
.
initList
(
head
)
s
=
Solution
()
print
(
l
.
convert_list
(
s
.
reverseKGroup
(
l1
,
k
=
2
)))
```
## template
```
python
...
...
@@ -71,7 +131,13 @@ print(l.convert_list(s.reverseKGroup(l1, k = 2)))
## 答案
```
python
while
pos
is
not
None
:
if
index
%
k
==
k
-
1
:
last
=
pos
.
next
start
=
self
.
reverseList
(
start
,
last
)
pos
=
start
pos
=
pos
.
next
index
+=
1
```
## 选项
...
...
@@ -79,17 +145,35 @@ print(l.convert_list(s.reverseKGroup(l1, k = 2)))
### A
```
python
while
pos
is
not
None
:
if
index
%
k
==
k
+
1
:
last
=
pos
.
next
start
=
self
.
reverseList
(
start
,
last
)
pos
=
start
pos
=
pos
.
next
index
+=
1
```
### B
```
python
while
pos
is
not
None
:
if
index
%
k
==
k
:
last
=
pos
.
next
start
=
self
.
reverseList
(
start
,
last
)
pos
=
start
pos
=
pos
.
next
index
+=
1
```
### C
```
python
while
pos
is
not
None
:
if
index
//
k
==
k
+
1
:
last
=
pos
.
next
start
=
self
.
reverseList
(
start
,
last
)
pos
=
start
pos
=
pos
.
next
index
+=
1
```
\ No newline at end of file
data/3.dailycode高阶/3.python/14.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,38 @@
<p><strong>
有效数字
</strong>
(按顺序)可以分成以下几个部分:
</p><ol>
<li>
一个
<strong>
小数
</strong>
或者
<strong>
整数
</strong></li>
<li>
(可选)一个
<code>
'e'
</code>
或
<code>
'E'
</code>
,后面跟着一个
<strong>
整数
</strong></li></ol><p><strong>
小数
</strong>
(按顺序)可以分成以下几个部分:
</p><ol>
<li>
(可选)一个符号字符(
<code>
'+'
</code>
或
<code>
'-'
</code>
)
</li>
<li>
下述格式之一:
<ol>
<li>
至少一位数字,后面跟着一个点
<code>
'.'
</code></li>
<li>
至少一位数字,后面跟着一个点
<code>
'.'
</code>
,后面再跟着至少一位数字
</li>
<li>
一个点
<code>
'.'
</code>
,后面跟着至少一位数字
</li>
</ol>
</li></ol><p><strong>
整数
</strong>
(按顺序)可以分成以下几个部分:
</p><ol>
<li>
(可选)一个符号字符(
<code>
'+'
</code>
或
<code>
'-'
</code>
)
</li>
<li>
至少一位数字
</li></ol><p>
部分有效数字列举如下:
</p><ul>
<li><code>
["2", "0089", "-0.1", "+3.14", "4.", "-.9", "2e10", "-90E3", "3e+7", "+6e-1", "53.5e93", "-123.456e789"]
</code></li></ul><p>
部分无效数字列举如下:
</p><ul>
<li><code>
["abc", "1a", "1e", "e3", "99e2.5", "--6", "-+3", "95a54e53"]
</code></li></ul><p>
给你一个字符串
<code>
s
</code>
,如果
<code>
s
</code>
是一个
<strong>
有效数字
</strong>
,请返回
<code>
true
</code>
。
</p><p>
</p><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
s = "0"
<strong><br
/>
输出:
</strong>
true
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
s = "e"
<strong><br
/>
输出:
</strong>
false
</pre><p><strong>
示例 3:
</strong></p><pre><strong>
输入:
</strong>
s = "."
<strong><br
/>
输出:
</strong>
false
</pre><p><strong>
示例 4:
</strong></p><pre><strong>
输入:
</strong>
s = ".1"
<strong><br
/>
输出:
</strong>
true
</pre><p>
</p><p><strong>
提示:
</strong></p><ul>
<li><code>
1
<
=
s.length
<=
20</
code
></li>
<li><code>
s
</code>
仅含英文字母(大写和小写),数字(
<code>
0-9
</code>
),加号
<code>
'+'
</code>
,减号
<code>
'-'
</code>
,或者点
<code>
'.'
</code>
。
</li></ul>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
isNumber
(
self
,
s
):
s
=
s
.
strip
()
ls
,
pos
=
len
(
s
),
0
if
ls
==
0
:
return
False
if
s
[
pos
]
==
'+'
or
s
[
pos
]
==
'-'
:
pos
+=
1
isNumeric
=
False
while
pos
<
ls
and
s
[
pos
].
isdigit
():
pos
+=
1
isNumeric
=
True
_____________________________
elif
pos
<
ls
and
s
[
pos
]
==
'e'
and
isNumeric
:
isNumeric
=
False
pos
+=
1
if
pos
<
ls
and
(
s
[
pos
]
==
'+'
or
s
[
pos
]
==
'-'
):
pos
+=
1
while
pos
<
ls
and
s
[
pos
].
isdigit
():
pos
+=
1
isNumeric
=
True
if
pos
==
ls
and
isNumeric
:
return
True
return
False
# %%
s
=
Solution
()
print
(
s
.
isNumber
(
s
=
"0"
))
```
## template
```
python
...
...
@@ -41,7 +73,11 @@ print(s.isNumber(s = "0"))
## 答案
```
python
if
pos
<
ls
and
s
[
pos
]
==
'.'
:
pos
+=
1
while
pos
<
ls
and
s
[
pos
].
isdigit
():
pos
+=
1
isNumeric
=
True
```
## 选项
...
...
@@ -49,17 +85,29 @@ print(s.isNumber(s = "0"))
### A
```
python
if
pos
<
ls
and
s
[
pos
]
==
'.'
:
pos
+=
1
while
pos
<
ls
:
pos
+=
1
isNumeric
=
True
```
### B
```
python
if
pos
<
ls
and
s
[
pos
]
==
'.'
:
pos
+=
1
while
pos
>
ls
:
pos
+=
1
isNumeric
=
True
```
### C
```
python
if
pos
<
ls
and
s
[
pos
]
==
'.'
:
pos
+=
1
while
pos
>
ls
and
s
[
pos
].
isdigit
():
pos
+=
1
isNumeric
=
True
```
\ No newline at end of file
data/3.dailycode高阶/3.python/15.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -9,6 +9,43 @@
<p><strong>
示例 2:
</strong></p>
<pre><strong>
输入: s =
</strong>
"
wordgoodgoodgoodbestword
"
,
<strong>
words =
</strong>
[
"
word
"
,
"
good
"
,
"
best
"
,
"
word
"
]
<strong><br
/>
输出:
</strong>
[]
</pre>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
findSubstring
(
self
,
s
,
words
):
"""
:type s: str
:type words: List[str]
:rtype: List[int]
"""
ls
=
len
(
s
)
word_ls
=
len
(
words
[
0
])
target_dict
=
{}
for
word
in
words
:
try
:
target_dict
[
word
]
+=
1
except
KeyError
:
target_dict
[
word
]
=
1
res
=
[]
___________________________________
curr_dict
=
target_dict
.
copy
()
for
pos
in
range
(
start
,
start
+
word_ls
*
len
(
words
),
word_ls
):
curr
=
s
[
pos
:
pos
+
word_ls
]
try
:
curr_dict
[
curr
]
-=
1
if
curr_dict
[
curr
]
<
0
:
break
except
KeyError
:
break
else
:
res
.
append
(
start
)
return
res
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
findSubstring
(
'wordgoodgoodgoodbestword'
,
[
"word"
,
"good"
,
"best"
,
"good"
]))
```
## template
```
python
...
...
@@ -49,7 +86,7 @@ if __name__ == '__main__':
## 答案
```
python
for
start
in
range
(
ls
-
word_ls
*
len
(
words
)
+
1
):
```
## 选项
...
...
@@ -57,17 +94,17 @@ if __name__ == '__main__':
### A
```
python
for
start
in
range
(
ls
-
word_ls
*
len
(
words
)):
```
### B
```
python
for
start
in
range
(
ls
+
word_ls
*
len
(
words
)):
```
### C
```
python
for
start
in
range
(
ls
+
word_ls
*
len
(
words
)
+
1
):
```
\ No newline at end of file
data/3.dailycode高阶/3.python/16.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,50 +2,100 @@
<p>
给定两个大小分别为
<code>
m
</code>
和
<code>
n
</code>
的正序(从小到大)数组
<code>
nums1
</code>
和
<code>
nums2
</code>
。请你找出并返回这两个正序数组的
<strong>
中位数
</strong>
。
</p><p>
</p><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
nums1 = [1,3], nums2 = [2]
<strong><br
/>
输出:
</strong>
2.00000
<strong><br
/>
解释:
</strong>
合并数组 = [1,2,3] ,中位数 2
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
nums1 = [1,2], nums2 = [3,4]
<strong><br
/>
输出:
</strong>
2.50000
<strong><br
/>
解释:
</strong>
合并数组 = [1,2,3,4] ,中位数 (2 + 3) / 2 = 2.5
</pre><p><strong>
示例 3:
</strong></p><pre><strong>
输入:
</strong>
nums1 = [0,0], nums2 = [0,0]
<strong><br
/>
输出:
</strong>
0.00000
</pre><p><strong>
示例 4:
</strong></p><pre><strong>
输入:
</strong>
nums1 = [], nums2 = [1]
<strong><br
/>
输出:
</strong>
1.00000
</pre><p><strong>
示例 5:
</strong></p><pre><strong>
输入:
</strong>
nums1 = [2], nums2 = []
<strong><br
/>
输出:
</strong>
2.00000
</pre><p>
</p><p><strong>
提示:
</strong></p><ul>
<li><code>
nums1.length == m
</code></li>
<li><code>
nums2.length == n
</code></li>
<li><code>
0
<
=
m
<=
1000</
code
></li>
<li><code>
0
<
=
n
<=
1000</
code
></li>
<li><code>
1
<
=
m
+
n
<=
2000</
code
></li>
<li><code>
-10
<sup>
6
</sup>
<
=
nums1
[
i
],
nums2
[
i
]
<=
10<
sup
>
6
</sup></code></li></ul><p>
</p><p><strong>
进阶:
</strong>
你能设计一个时间复杂度为
<code>
O(log (m+n))
</code>
的算法解决此问题吗?
</p>
以下程序实现了这一功能,请你填补空白处内容:
```
python
import
math
from
typing
import
List
class
Solution
:
def
findMedianSortedArrays
(
self
,
nums1
:
List
[
int
],
nums2
:
List
[
int
])
->
float
:
nums1Size
=
len
(
nums1
)
nums2Size
=
len
(
nums2
)
na
=
nums1Size
+
nums2Size
ns
=
[]
i
=
0
j
=
0
m
=
int
(
math
.
floor
(
na
/
2
+
1
))
while
len
(
ns
)
<
m
:
n
=
None
if
i
<
nums1Size
and
j
<
nums2Size
:
if
nums1
[
i
]
<
nums2
[
j
]:
n
=
nums1
[
i
]
i
+=
1
else
:
n
=
nums2
[
j
]
j
+=
1
elif
i
<
nums1Size
:
n
=
nums1
[
i
]
i
+=
1
elif
j
<
nums2Size
:
n
=
nums2
[
j
]
j
+=
1
ns
.
append
(
n
)
d
=
len
(
ns
)
if
na
%
2
==
1
:
return
ns
[
d
-
1
]
else
:
return
_____________________
# %%
s
=
Solution
()
print
(
s
.
findMedianSortedArrays
([
1
,
3
],
[
2
]))
```
## template
```
python
import
math
from
typing
import
List
class
Solution
:
def
findMedianSortedArrays
(
self
,
nums1
:
List
[
int
],
nums2
:
List
[
int
])
->
float
:
nums1Size
=
len
(
nums1
)
nums2Size
=
len
(
nums2
)
na
=
nums1Size
+
nums2Size
ns
=
[]
i
=
0
j
=
0
m
=
int
(
math
.
floor
(
na
/
2
+
1
))
while
len
(
ns
)
<
m
:
n
=
None
if
i
<
nums1Size
and
j
<
nums2Size
:
if
nums1
[
i
]
<
nums2
[
j
]:
n
=
nums1
[
i
]
i
+=
1
else
:
n
=
nums2
[
j
]
j
+=
1
elif
i
<
nums1Size
:
n
=
nums1
[
i
]
i
+=
1
elif
j
<
nums2Size
:
n
=
nums2
[
j
]
j
+=
1
ns
.
append
(
n
)
d
=
len
(
ns
)
if
na
%
2
==
1
:
return
ns
[
d
-
1
]
else
:
return
(
ns
[
d
-
1
]
+
ns
[
d
-
2
])
/
2.0
def
findMedianSortedArrays
(
self
,
nums1
:
List
[
int
],
nums2
:
List
[
int
])
->
float
:
nums1Size
=
len
(
nums1
)
nums2Size
=
len
(
nums2
)
na
=
nums1Size
+
nums2Size
ns
=
[]
i
=
0
j
=
0
m
=
int
(
math
.
floor
(
na
/
2
+
1
))
while
len
(
ns
)
<
m
:
n
=
None
if
i
<
nums1Size
and
j
<
nums2Size
:
if
nums1
[
i
]
<
nums2
[
j
]:
n
=
nums1
[
i
]
i
+=
1
else
:
n
=
nums2
[
j
]
j
+=
1
elif
i
<
nums1Size
:
n
=
nums1
[
i
]
i
+=
1
elif
j
<
nums2Size
:
n
=
nums2
[
j
]
j
+=
1
ns
.
append
(
n
)
d
=
len
(
ns
)
if
na
%
2
==
1
:
return
ns
[
d
-
1
]
else
:
return
(
ns
[
d
-
1
]
+
ns
[
d
-
2
])
/
2.0
# %%
s
=
Solution
()
print
(
s
.
findMedianSortedArrays
([
1
,
3
],
[
2
]))
print
(
s
.
findMedianSortedArrays
([
1
,
3
],
[
2
]))
```
## 答案
```
python
(
ns
[
d
-
1
]
+
ns
[
d
-
2
])
/
2.0
```
## 选项
...
...
@@ -53,17 +103,17 @@ print(s.findMedianSortedArrays([1,3], [2]))
### A
```
python
(
ns
[
d
+
1
]
+
ns
[
d
+
2
])
/
2.0
```
### B
```
python
(
ns
[
d
-
1
]
-
ns
[
d
-
2
])
/
2.0
```
### C
```
python
(
ns
[
d
+
1
]
-
ns
[
d
+
2
])
/
2.0
```
\ No newline at end of file
data/3.dailycode高阶/3.python/17.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,52 +2,98 @@
<p>
给你一个字符串
<code>
s
</code>
、一个字符串
<code>
t
</code>
。返回
<code>
s
</code>
中涵盖
<code>
t
</code>
所有字符的最小子串。如果
<code>
s
</code>
中不存在涵盖
<code>
t
</code>
所有字符的子串,则返回空字符串
<code>
""
</code>
。
</p><p><strong>
注意:
</strong>
如果
<code>
s
</code>
中存在这样的子串,我们保证它是唯一的答案。
</p><p>
</p><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
s = "ADOBECODEBANC", t = "ABC"
<strong><br
/>
输出:
</strong>
"BANC"
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
s = "a", t = "a"
<strong><br
/>
输出:
</strong>
"a"
</pre><p>
</p><p><strong>
提示:
</strong></p><ul>
<li><code>
1
<
=
s.length
,
t.length
<=
10<
sup
>
5
</sup></code></li>
<li><code>
s
</code>
和
<code>
t
</code>
由英文字母组成
</li></ul><p>
</p><strong>
进阶:
</strong>
你能设计一个在
<code>
o(n)
</code>
时间内解决此问题的算法吗?
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
minWindow
(
self
,
s
,
t
):
ls_s
,
ls_t
=
len
(
s
),
len
(
t
)
need_to_find
=
[
0
]
*
256
has_found
=
[
0
]
*
256
min_begin
,
min_end
=
0
,
-
1
min_window
=
100000000000000
for
index
in
range
(
ls_t
):
need_to_find
[
ord
(
t
[
index
])]
+=
1
count
,
begin
=
0
,
0
for
end
in
range
(
ls_s
):
end_index
=
ord
(
s
[
end
])
if
need_to_find
[
end_index
]
==
0
:
continue
has_found
[
end_index
]
+=
1
if
has_found
[
end_index
]
<=
need_to_find
[
end_index
]:
count
+=
1
if
count
==
ls_t
:
begin_index
=
ord
(
s
[
begin
])
____________________________
if
window_ls
<
min_window
:
min_begin
=
begin
min_end
=
end
min_window
=
window_ls
if
count
==
ls_t
:
return
s
[
min_begin
:
min_end
+
1
]
else
:
return
''
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
minWindow
(
'a'
,
'a'
))
```
## template
```
python
class
Solution
(
object
):
def
minWindow
(
self
,
s
,
t
):
ls_s
,
ls_t
=
len
(
s
),
len
(
t
)
need_to_find
=
[
0
]
*
256
has_found
=
[
0
]
*
256
min_begin
,
min_end
=
0
,
-
1
min_window
=
100000000000000
for
index
in
range
(
ls_t
):
need_to_find
[
ord
(
t
[
index
])]
+=
1
count
,
begin
=
0
,
0
for
end
in
range
(
ls_s
):
end_index
=
ord
(
s
[
end
])
if
need_to_find
[
end_index
]
==
0
:
continue
has_found
[
end_index
]
+=
1
if
has_found
[
end_index
]
<=
need_to_find
[
end_index
]:
count
+=
1
if
count
==
ls_t
:
begin_index
=
ord
(
s
[
begin
])
while
need_to_find
[
begin_index
]
==
0
or
\
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
if
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
has_found
[
begin_index
]
-=
1
begin
+=
1
begin_index
=
ord
(
s
[
begin
])
window_ls
=
end
-
begin
+
1
if
window_ls
<
min_window
:
min_begin
=
begin
min_end
=
end
min_window
=
window_ls
if
count
==
ls_t
:
return
s
[
min_begin
:
min_end
+
1
]
else
:
return
''
def
minWindow
(
self
,
s
,
t
):
ls_s
,
ls_t
=
len
(
s
),
len
(
t
)
need_to_find
=
[
0
]
*
256
has_found
=
[
0
]
*
256
min_begin
,
min_end
=
0
,
-
1
min_window
=
100000000000000
for
index
in
range
(
ls_t
):
need_to_find
[
ord
(
t
[
index
])]
+=
1
count
,
begin
=
0
,
0
for
end
in
range
(
ls_s
):
end_index
=
ord
(
s
[
end
])
if
need_to_find
[
end_index
]
==
0
:
continue
has_found
[
end_index
]
+=
1
if
has_found
[
end_index
]
<=
need_to_find
[
end_index
]:
count
+=
1
if
count
==
ls_t
:
begin_index
=
ord
(
s
[
begin
])
while
need_to_find
[
begin_index
]
==
0
or
\
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
if
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
has_found
[
begin_index
]
-=
1
begin
+=
1
begin_index
=
ord
(
s
[
begin
])
window_ls
=
end
-
begin
+
1
if
window_ls
<
min_window
:
min_begin
=
begin
min_end
=
end
min_window
=
window_ls
if
count
==
ls_t
:
return
s
[
min_begin
:
min_end
+
1
]
else
:
return
''
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
minWindow
(
'a'
,
'a'
))
s
=
Solution
()
print
(
s
.
minWindow
(
'a'
,
'a'
))
```
## 答案
```
python
while
need_to_find
[
begin_index
]
==
0
or
\
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
if
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
has_found
[
begin_index
]
-=
1
begin
+=
1
begin_index
=
ord
(
s
[
begin
])
window_ls
=
end
-
begin
+
1
```
## 选项
...
...
@@ -55,17 +101,35 @@ if __name__ == '__main__':
### A
```
python
while
need_to_find
[
begin_index
]
==
0
or
\
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
if
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
has_found
[
begin_index
]
-=
1
begin
+=
1
begin_index
=
ord
(
s
[
begin
])
window_ls
=
end
-
begin
```
### B
```
python
while
need_to_find
[
begin_index
]
==
0
or
\
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
if
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
has_found
[
begin_index
]
+=
1
begin
+=
1
begin_index
=
ord
(
s
[
begin
])
window_ls
=
end
-
begin
```
### C
```
python
while
need_to_find
[
begin_index
]
==
0
or
\
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
if
has_found
[
begin_index
]
>
need_to_find
[
begin_index
]:
has_found
[
begin_index
]
+=
1
begin
+=
1
begin_index
=
ord
(
s
[
begin
])
window_ls
=
end
-
begin
+
1
```
\ No newline at end of file
data/3.dailycode高阶/3.python/18.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,76 +2,168 @@
<p>
给你一个链表数组,每个链表都已经按升序排列。
</p><p>
请你将所有链表合并到一个升序链表中,返回合并后的链表。
</p><p>
</p><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
lists =
[
[1,4,5],[1,3,4],[2,6]]<strong><br />输出:</strong>[1,1,2,3,4,4,5,6]<strong><br />解释:</strong>链表数组如下:[ 1->4->5, 1->3->4, 2->6]将它们合并到一个有序链表中得到。1->1->2->3->4->4->5->6</pre><p><strong>示例 2:</strong></p><pre><strong>输入:</strong>lists = []<strong><br />输出:</strong>[]</pre><p><strong>示例 3:</strong></p><pre><strong>输入:</strong>lists = [[]]<strong><br />输出:</strong>[]</pre><p> </p><p><strong>提示:</strong></p><ul> <li><code>k == lists.length</code></li> <li><code>0 <= k <= 10^4</code></li> <li><code>0 <= lists[i].length <= 500</code></li> <li><code>-10^4 <= lists[i
][
j
]
<
= 10^4
</code></li>
<li><code>
lists[i]
</code>
按
<strong>
升序
</strong>
排列
</li>
<li><code>
lists[i].length
</code>
的总和不超过
<code>
10^4
</code></li></ul>
以下程序实现了这一功能,请你填补空白处内容:
```
python
from
typing
import
List
class
ListNode
(
object
):
def
__init__
(
self
,
x
):
self
.
val
=
x
self
.
next
=
None
class
LinkList
:
def
__init__
(
self
):
self
.
head
=
None
def
initList
(
self
,
data
):
self
.
head
=
ListNode
(
data
[
0
])
r
=
self
.
head
p
=
self
.
head
for
i
in
data
[
1
:]:
node
=
ListNode
(
i
)
p
.
next
=
node
p
=
p
.
next
return
r
def
convert_list
(
self
,
head
):
ret
=
[]
if
head
==
None
:
return
node
=
head
while
node
!=
None
:
ret
.
append
(
node
.
val
)
node
=
node
.
next
return
ret
class
Solution
(
object
):
def
mergeKLists
(
self
,
lists
):
if
lists
is
None
:
return
None
elif
len
(
lists
)
==
0
:
return
None
return
self
.
mergeK
(
lists
,
0
,
len
(
lists
)
-
1
)
def
mergeK
(
self
,
lists
,
low
,
high
):
if
low
==
high
:
return
lists
[
int
(
low
)]
elif
low
+
1
==
high
:
return
self
.
mergeTwolists
(
lists
[
int
(
low
)],
lists
[
int
(
high
)])
mid
=
(
low
+
high
)
/
2
return
self
.
mergeTwolists
(
self
.
mergeK
(
lists
,
low
,
mid
),
self
.
mergeK
(
lists
,
mid
+
1
,
high
))
def
mergeTwolists
(
self
,
l1
,
l2
):
l
=
LinkList
()
if
type
(
l1
)
==
list
:
l1
=
l
.
initList
(
l1
)
if
type
(
l2
)
==
list
:
l2
=
l
.
initList
(
l2
)
if
l1
is
None
:
return
l2
if
l2
is
None
:
return
l1
head
=
curr
=
ListNode
(
-
1
)
while
l1
is
not
None
and
l2
is
not
None
:
____________________
curr
=
curr
.
next
if
l1
is
not
None
:
curr
.
next
=
l1
if
l2
is
not
None
:
curr
.
next
=
l2
return
head
.
next
# %%
l
=
LinkList
()
list1
=
[[
1
,
4
,
5
],
[
1
,
3
,
4
],
[
2
,
6
]]
s
=
Solution
()
print
(
l
.
convert_list
(
s
.
mergeKLists
(
list1
)))
```
## template
```
python
from
typing
import
List
class
ListNode
(
object
):
def
__init__
(
self
,
x
):
self
.
val
=
x
self
.
next
=
None
def
__init__
(
self
,
x
):
self
.
val
=
x
self
.
next
=
None
class
LinkList
:
def
__init__
(
self
):
self
.
head
=
None
def
initList
(
self
,
data
):
self
.
head
=
ListNode
(
data
[
0
])
r
=
self
.
head
p
=
self
.
head
for
i
in
data
[
1
:]:
node
=
ListNode
(
i
)
p
.
next
=
node
p
=
p
.
next
return
r
def
convert_list
(
self
,
head
):
ret
=
[]
if
head
==
None
:
return
node
=
head
while
node
!=
None
:
ret
.
append
(
node
.
val
)
node
=
node
.
next
return
ret
def
__init__
(
self
):
self
.
head
=
None
def
initList
(
self
,
data
):
self
.
head
=
ListNode
(
data
[
0
])
r
=
self
.
head
p
=
self
.
head
for
i
in
data
[
1
:]:
node
=
ListNode
(
i
)
p
.
next
=
node
p
=
p
.
next
return
r
def
convert_list
(
self
,
head
):
ret
=
[]
if
head
==
None
:
return
node
=
head
while
node
!=
None
:
ret
.
append
(
node
.
val
)
node
=
node
.
next
return
ret
class
Solution
(
object
):
def
mergeKLists
(
self
,
lists
):
if
lists
is
None
:
return
None
elif
len
(
lists
)
==
0
:
return
None
return
self
.
mergeK
(
lists
,
0
,
len
(
lists
)
-
1
)
def
mergeK
(
self
,
lists
,
low
,
high
):
if
low
==
high
:
return
lists
[
int
(
low
)]
elif
low
+
1
==
high
:
return
self
.
mergeTwolists
(
lists
[
int
(
low
)],
lists
[
int
(
high
)])
mid
=
(
low
+
high
)
/
2
return
self
.
mergeTwolists
(
self
.
mergeK
(
lists
,
low
,
mid
),
self
.
mergeK
(
lists
,
mid
+
1
,
high
))
def
mergeTwolists
(
self
,
l1
,
l2
):
l
=
LinkList
()
if
type
(
l1
)
==
list
:
l1
=
l
.
initList
(
l1
)
if
type
(
l2
)
==
list
:
l2
=
l
.
initList
(
l2
)
if
l1
is
None
:
return
l2
if
l2
is
None
:
return
l1
head
=
curr
=
ListNode
(
-
1
)
while
l1
is
not
None
and
l2
is
not
None
:
if
l1
.
val
<=
l2
.
val
:
curr
.
next
=
l1
l1
=
l1
.
next
else
:
curr
.
next
=
l2
l2
=
l2
.
next
curr
=
curr
.
next
if
l1
is
not
None
:
curr
.
next
=
l1
if
l2
is
not
None
:
curr
.
next
=
l2
return
head
.
next
def
mergeKLists
(
self
,
lists
):
if
lists
is
None
:
return
None
elif
len
(
lists
)
==
0
:
return
None
return
self
.
mergeK
(
lists
,
0
,
len
(
lists
)
-
1
)
def
mergeK
(
self
,
lists
,
low
,
high
):
if
low
==
high
:
return
lists
[
int
(
low
)]
elif
low
+
1
==
high
:
return
self
.
mergeTwolists
(
lists
[
int
(
low
)],
lists
[
int
(
high
)])
mid
=
(
low
+
high
)
/
2
return
self
.
mergeTwolists
(
self
.
mergeK
(
lists
,
low
,
mid
),
self
.
mergeK
(
lists
,
mid
+
1
,
high
))
def
mergeTwolists
(
self
,
l1
,
l2
):
l
=
LinkList
()
if
type
(
l1
)
==
list
:
l1
=
l
.
initList
(
l1
)
if
type
(
l2
)
==
list
:
l2
=
l
.
initList
(
l2
)
if
l1
is
None
:
return
l2
if
l2
is
None
:
return
l1
head
=
curr
=
ListNode
(
-
1
)
while
l1
is
not
None
and
l2
is
not
None
:
list1
=
[[
1
,
4
,
5
],
[
1
,
3
,
4
],
[
2
,
6
]]
s
=
Solution
()
print
(
l
.
convert_list
(
s
.
mergeKLists
(
list1
)))
curr
=
curr
.
next
if
l1
is
not
None
:
curr
.
next
=
l1
if
l2
is
not
None
:
curr
.
next
=
l2
return
head
.
next
# %%
l
=
LinkList
()
list1
=
[[
1
,
4
,
5
],[
1
,
3
,
4
],[
2
,
6
]]
list1
=
[[
1
,
4
,
5
],
[
1
,
3
,
4
],
[
2
,
6
]]
s
=
Solution
()
print
(
l
.
convert_list
(
s
.
mergeKLists
(
list1
)))
```
...
...
@@ -79,7 +171,12 @@ print(l.convert_list(s.mergeKLists(list1)))
## 答案
```
python
if
l1
.
val
<=
l2
.
val
:
curr
.
next
=
l1
l1
=
l1
.
next
else
:
curr
.
next
=
l2
l2
=
l2
.
next
```
## 选项
...
...
@@ -87,17 +184,32 @@ print(l.convert_list(s.mergeKLists(list1)))
### A
```
python
if
l1
.
val
>=
l2
.
val
:
curr
.
next
=
l1
l1
=
l1
.
next
else
:
curr
.
next
=
l2
l2
=
l2
.
next
```
### B
```
python
if
l1
.
val
<=
l2
.
val
:
curr
.
next
=
l2
l2
=
l2
.
next
else
:
curr
.
next
=
l1
l1
=
l1
.
next
```
### C
```
python
if
l1
.
val
>
l2
.
val
:
curr
.
next
=
l1
l1
=
l1
.
next
else
:
curr
.
next
=
l2
l2
=
l2
.
next
```
\ No newline at end of file
data/3.dailycode高阶/3.python/19.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,50 +2,99 @@
<p>
给出集合
<code>
[1,2,3,...,n]
</code>
,其所有元素共有
<code>
n!
</code>
种排列。
</p><p>
按大小顺序列出所有排列情况,并一一标记,当
<code>
n = 3
</code>
时, 所有排列如下:
</p><ol>
<li><code>
"123"
</code></li>
<li><code>
"132"
</code></li>
<li><code>
"213"
</code></li>
<li><code>
"231"
</code></li>
<li><code>
"312"
</code></li>
<li><code>
"321"
</code></li></ol><p>
给定
<code>
n
</code>
和
<code>
k
</code>
,返回第
<code>
k
</code>
个排列。
</p><p>
</p><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
n = 3, k = 3
<strong><br
/>
输出:
</strong>
"213"
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
n = 4, k = 9
<strong><br
/>
输出:
</strong>
"2314"
</pre><p><strong>
示例 3:
</strong></p><pre><strong>
输入:
</strong>
n = 3, k = 1
<strong><br
/>
输出:
</strong>
"123"
</pre><p>
</p><p><strong>
提示:
</strong></p><ul>
<li><code>
1
<
=
n
<=
9</
code
></li>
<li><code>
1
<
=
k
<=
n
!</
code
></li></ul>
## template
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
getPermutation
(
self
,
n
,
k
):
def
getPermutation
(
self
,
n
,
k
):
"""
:type n: int
:type k: int
:rtype: str
"""
import
math
res
=
[
""
]
def
generate
(
s
,
k
):
n
=
len
(
s
)
if
n
<=
2
:
if
k
==
2
:
res
[
0
]
+=
s
[::
-
1
]
else
:
res
[
0
]
+=
s
return
step
=
math
.
factorial
(
n
-
1
)
yu
=
k
%
step
if
yu
==
0
:
yu
=
step
c
=
k
//
step
-
1
else
:
c
=
k
//
step
res
[
0
]
+=
s
[
c
]
____________________
return
s
=
""
for
i
in
range
(
1
,
n
+
1
):
s
+=
str
(
i
)
generate
(
s
,
k
)
return
res
[
0
]
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
getPermutation
(
3
,
2
))
```
## template
```
python
class
Solution
(
object
):
def
getPermutation
(
self
,
n
,
k
):
"""
:type n: int
:type k: int
:rtype: str
"""
import
math
res
=
[
""
]
def
generate
(
s
,
k
):
n
=
len
(
s
)
if
n
<=
2
:
if
k
==
2
:
res
[
0
]
+=
s
[::
-
1
]
else
:
res
[
0
]
+=
s
return
step
=
math
.
factorial
(
n
-
1
)
yu
=
k
%
step
if
yu
==
0
:
yu
=
step
c
=
k
//
step
-
1
else
:
c
=
k
//
step
res
[
0
]
+=
s
[
c
]
generate
(
s
[:
c
]
+
s
[
c
+
1
:],
yu
)
return
s
=
""
for
i
in
range
(
1
,
n
+
1
):
s
+=
str
(
i
)
generate
(
s
,
k
)
return
res
[
0
]
import
math
res
=
[
""
]
def
generate
(
s
,
k
):
n
=
len
(
s
)
if
n
<=
2
:
if
k
==
2
:
res
[
0
]
+=
s
[::
-
1
]
else
:
res
[
0
]
+=
s
return
step
=
math
.
factorial
(
n
-
1
)
yu
=
k
%
step
if
yu
==
0
:
yu
=
step
c
=
k
//
step
-
1
else
:
c
=
k
//
step
res
[
0
]
+=
s
[
c
]
generate
(
s
[:
c
]
+
s
[
c
+
1
:],
yu
)
return
s
=
""
for
i
in
range
(
1
,
n
+
1
):
s
+=
str
(
i
)
generate
(
s
,
k
)
return
res
[
0
]
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
getPermutation
(
3
,
2
))
s
=
Solution
()
print
(
s
.
getPermutation
(
3
,
2
))
```
## 答案
```
python
generate
(
s
[:
c
]
+
s
[
c
+
1
:],
yu
)
```
## 选项
...
...
@@ -53,17 +102,17 @@ if __name__ == '__main__':
### A
```
python
generate
(
s
[:
c
-
1
]
+
s
[
c
+
1
:],
yu
)
```
### B
```
python
generate
(
s
[:
c
+
1
]
+
s
[
c
-
1
:],
yu
)
```
### C
```
python
generate
(
s
[:
c
]
+
s
[
c
:],
yu
)
```
\ No newline at end of file
data/3.dailycode高阶/3.python/20.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,38 @@
<p>
给定一个字符串
(
<code>
s
</code>
) 和一个字符模式
(
<code>
p
</code>
) ,实现一个支持
<code>
'
?
'
</code>
和
<code>
'
*
'
</code>
的通配符匹配。
</p><pre>
'
?
'
可以匹配任何单个字符。
'
*
'
可以匹配任意字符串(包括空字符串)。
</pre><p>
两个字符串
<strong>
完全匹配
</strong>
才算匹配成功。
</p><p><strong>
说明:
</strong></p><ul>
<li><code>
s
</code>
可能为空,且只包含从
<code>
a-z
</code>
的小写字母。
</li>
<li><code>
p
</code>
可能为空,且只包含从
<code>
a-z
</code>
的小写字母,以及字符
<code>
?
</code>
和
<code>
*
</code>
。
</li></ul><p><strong>
示例
1:
</strong></p><pre><strong>
输入:
</strong>
s =
"
aa
"
p =
"
a
"
<strong><br
/>
输出:
</strong>
false
<strong><br
/>
解释:
</strong>
"
a
"
无法匹配
"
aa
"
整个字符串。
</pre><p><strong>
示例
2:
</strong></p><pre><strong>
输入:
</strong>
s =
"
aa
"
p =
"
*
"
<strong><br
/>
输出:
</strong>
true
<strong><br
/>
解释:
</strong>
'
*
'
可以匹配任意字符串。
</pre><p><strong>
示例
3:
</strong></p><pre><strong>
输入:
</strong>
s =
"
cb
"
p =
"
?a
"
<strong><br
/>
输出:
</strong>
false
<strong><br
/>
解释:
</strong>
'
?
'
可以匹配
'
c
'
, 但第二个
'
a
'
无法匹配
'
b
'
。
</pre><p><strong>
示例
4:
</strong></p><pre><strong>
输入:
</strong>
s =
"
adceb
"
p =
"
*a*b
"
<strong><br
/>
输出:
</strong>
true
<strong><br
/>
解释:
</strong>
第一个
'
*
'
可以匹配空字符串, 第二个
'
*
'
可以匹配字符串
"
dce
"
.
</pre><p><strong>
示例
5:
</strong></p><pre><strong>
输入:
</strong>
s =
"
acdcb
"
p =
"
a*c?b
"
<strong><br
/>
输出:
</strong>
false
</pre>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
(
object
):
def
isMatch
(
self
,
s
,
p
):
"""
:type s: str
:type p: str
:rtype: bool
"""
s_index
,
p_index
=
0
,
0
star
,
s_star
=
-
1
,
0
s_len
,
p_len
=
len
(
s
),
len
(
p
)
while
s_index
<
s_len
:
if
p_index
<
p_len
and
(
s
[
s_index
]
==
p
[
p_index
]
or
p
[
p_index
]
==
'?'
):
s_index
+=
1
p_index
+=
1
______________________
elif
star
!=
-
1
:
p_index
=
star
+
1
s_star
+=
1
s_index
=
s_star
else
:
return
False
while
p_index
<
p_len
and
p
[
p_index
]
==
'*'
:
p_index
+=
1
return
p_index
==
p_len
if
__name__
==
'__main__'
:
s
=
Solution
()
print
(
s
.
isMatch
(
s
=
"aa"
,
p
=
"a"
))
```
## template
```
python
...
...
@@ -40,7 +72,10 @@ if __name__ == '__main__':
## 答案
```
python
elif
p_index
<
p_len
and
p
[
p_index
]
==
'*'
:
star
=
p_index
s_star
=
s_index
p_index
+=
1
```
## 选项
...
...
@@ -48,17 +83,26 @@ if __name__ == '__main__':
### A
```
python
elif
p_index
>
p_len
and
p
[
p_index
]
==
'*'
:
star
=
p_index
s_star
=
s_index
p_index
+=
1
```
### B
```
python
elif
p_index
<
p_len
and
p
[
p_index
]
==
'*'
:
star
=
p_index
s_star
=
s_index
p_index
+=
s_star
```
### C
```
python
elif
p_index
>
p_len
and
p
[
p_index
]
==
'*'
:
star
=
p_index
s_star
=
s_index
p_index
+=
s_star
```
\ No newline at end of file
data/3.dailycode高阶/3.python/21.exercises/solution.md
浏览文件 @
12dafb7c
...
...
@@ -2,6 +2,25 @@
<p>
给你一个字符串
<code>
s
</code>
和一个字符规律
<code>
p
</code>
,请你来实现一个支持
<code>
'.'
</code>
和
<code>
'
*'</code> 的正则表达式匹配。</p><ul> <li><code>'.'</code> 匹配任意单个字符</li> <li><code>'*
'
</code>
匹配零个或多个前面的那一个元素
</li></ul><p>
所谓匹配,是要涵盖
<strong>
整个
</strong>
字符串
<code>
s
</code>
的,而不是部分字符串。
</p><p><strong>
示例 1:
</strong></p><pre><strong>
输入:
</strong>
s = "aa" p = "a"
<strong><br
/>
输出:
</strong>
false
<strong><br
/>
解释:
</strong>
"a" 无法匹配 "aa" 整个字符串。
</pre><p><strong>
示例 2:
</strong></p><pre><strong>
输入:
</strong>
s = "aa" p = "a
*"<strong><br />输出:</strong>true<strong><br />解释:</strong>因为 '*
' 代表可以匹配零个或多个前面的那一个元素, 在这里前面的元素就是 'a'。因此,字符串 "aa" 可被视为 'a' 重复了一次。
</pre><p><strong>
示例 3:
</strong></p><pre><strong>
输入:
</strong>
s = "ab" p = ".
*"<strong><br />输出:</strong>true<strong><br />解释:</strong>".*
" 表示可匹配零个或多个('
*')任意字符('.')。</pre><p><strong>示例 4:</strong></p><pre><strong>输入:</strong>s = "aab" p = "c*
a
*b"<strong><br />输出:</strong>true<strong><br />解释:</strong>因为 '*
' 表示零个或多个,这里 'c' 为 0 个, 'a' 被重复一次。因此可以匹配字符串 "aab"。
</pre><p><strong>
示例 5:
</strong></p><pre><strong>
输入:
</strong>
s = "mississippi" p = "mis
*is*
p
*."<strong><br />输出:</strong>false</pre><p> </p><p><strong>提示:</strong></p><ul> <li><code>0 <= s.length <= 20</code></li> <li><code>0 <= p.length <= 30</code></li> <li><code>s</code> 可能为空,且只包含从 <code>a-z</code> 的小写字母。</li> <li><code>p</code> 可能为空,且只包含从 <code>a-z</code> 的小写字母,以及字符 <code>.</code> 和 <code>*
</code>
。
</li>
<li>
保证每次出现字符
<code>
*
</code>
时,前面都匹配到有效的字符
</li></ul>
以下程序实现了这一功能,请你填补空白处内容:
```
python
class
Solution
:
def
isMatch
(
self
,
s
:
str
,
p
:
str
)
->
bool
:
if
len
(
p
)
==
0
:
return
len
(
s
)
==
0
head_match
=
len
(
s
)
>
0
and
(
s
[
0
]
==
p
[
0
]
or
p
[
0
]
==
'.'
)
if
len
(
p
)
>
1
and
p
[
1
]
==
'*'
:
__________________________
else
:
if
not
head_match
:
return
False
return
self
.
isMatch
(
s
[
1
:],
p
[
1
:])
# %%
s
=
Solution
()
print
(
s
.
isMatch
(
s
=
"aa"
,
p
=
"a"
))
```
## template
```
python
...
...
@@ -26,7 +45,9 @@ print(s.isMatch(s = "aa" , p = "a"))
## 答案
```
python
if
head_match
and
self
.
isMatch
(
s
[
1
:],
p
):
return
True
return
self
.
isMatch
(
s
,
p
[
2
:])
```
## 选项
...
...
@@ -34,17 +55,23 @@ print(s.isMatch(s = "aa" , p = "a"))
### A
```
python
if
self
.
isMatch
(
s
[
1
:],
p
):
return
True
return
self
.
isMatch
(
s
,
p
[
2
:])
```
### B
```
python
if
self
.
isMatch
(
s
[
2
:],
p
):
return
True
return
self
.
isMatch
(
s
,
p
[
1
:])
```
### C
```
python
if
head_match
and
self
.
isMatch
(
s
[
2
:],
p
):
return
False
return
self
.
isMatch
(
s
,
p
[
1
:])
```
\ No newline at end of file
编辑
预览
Markdown
is supported
0%
请重试
或
添加新附件
.
添加附件
取消
You are about to add
0
people
to the discussion. Proceed with caution.
先完成此消息的编辑!
取消
想要评论请
注册
或
登录