Skip to content
体验新版
项目
组织
正在加载...
登录
切换导航
打开侧边栏
bluewinestar
spring-boot-examples
提交
4bd47ecd
S
spring-boot-examples
项目概览
bluewinestar
/
spring-boot-examples
与 Fork 源项目一致
从无法访问的项目Fork
通知
1
Star
0
Fork
0
代码
文件
提交
分支
Tags
贡献者
分支图
Diff
Issue
0
列表
看板
标记
里程碑
合并请求
0
Wiki
0
Wiki
分析
仓库
DevOps
项目成员
Pages
S
spring-boot-examples
项目概览
项目概览
详情
发布
仓库
仓库
文件
提交
分支
标签
贡献者
分支图
比较
Issue
0
Issue
0
列表
看板
标记
里程碑
合并请求
0
合并请求
0
Pages
分析
分析
仓库分析
DevOps
Wiki
0
Wiki
成员
成员
收起侧边栏
关闭侧边栏
动态
分支图
创建新Issue
提交
Issue看板
前往新版Gitcode,体验更适合开发者的 AI 搜索 >>
提交
4bd47ecd
编写于
5月 06, 2017
作者:
微笑很纯洁
浏览文件
操作
浏览文件
下载
电子邮件补丁
差异文件
add spring-boot-multi-mongodb
上级
6c3e9832
变更
14
隐藏空白更改
内联
并排
Showing
14 changed file
with
333 addition
and
1 deletion
+333
-1
README.md
README.md
+3
-1
spring-boot-multi-mongodb/pom.xml
spring-boot-multi-mongodb/pom.xml
+60
-0
spring-boot-multi-mongodb/src/main/java/com/neo/Application.java
...boot-multi-mongodb/src/main/java/com/neo/Application.java
+18
-0
spring-boot-multi-mongodb/src/main/java/com/neo/config/MultipleMongoConfig.java
...odb/src/main/java/com/neo/config/MultipleMongoConfig.java
+48
-0
spring-boot-multi-mongodb/src/main/java/com/neo/config/PrimaryMongoConfig.java
...godb/src/main/java/com/neo/config/PrimaryMongoConfig.java
+15
-0
spring-boot-multi-mongodb/src/main/java/com/neo/config/SecondaryMongoConfig.java
...db/src/main/java/com/neo/config/SecondaryMongoConfig.java
+15
-0
spring-boot-multi-mongodb/src/main/java/com/neo/config/props/MultipleMongoProperties.java
...in/java/com/neo/config/props/MultipleMongoProperties.java
+16
-0
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/primary/PrimaryMongoObject.java
.../com/neo/model/repository/primary/PrimaryMongoObject.java
+28
-0
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/primary/PrimaryRepository.java
...a/com/neo/model/repository/primary/PrimaryRepository.java
+9
-0
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/secondary/SecondaryMongoObject.java
.../neo/model/repository/secondary/SecondaryMongoObject.java
+29
-0
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/secondary/SecondaryRepository.java
...m/neo/model/repository/secondary/SecondaryRepository.java
+9
-0
spring-boot-multi-mongodb/src/main/resources/application.yml
spring-boot-multi-mongodb/src/main/resources/application.yml
+9
-0
spring-boot-multi-mongodb/src/test/java/com/neo/ApplicationTests.java
...multi-mongodb/src/test/java/com/neo/ApplicationTests.java
+17
-0
spring-boot-multi-mongodb/src/test/java/com/neo/model/repository/MuliDatabaseTest.java
.../test/java/com/neo/model/repository/MuliDatabaseTest.java
+57
-0
未找到文件。
README.md
浏览文件 @
4bd47ecd
...
...
@@ -14,6 +14,7 @@ Spring boot使用的各种示例,以最简单、最实用为标准
-
[
spring-boot-web
](
https://github.com/ityouknow/spring-boot-starter/tree/master/spring-boot-web
)
:web开发综合使用案例
-
[
spring-boot-mail
](
https://github.com/ityouknow/spring-boot-starter/tree/master/spring-boot-mail
)
:spring boot和邮件服务
-
[
spring-boot-mongodb
](
https://github.com/ityouknow/spring-boot-starter/tree/master/spring-boot-mongodb
)
:spring boot和mongodb的使用
-
[
spring-boot-multi-mongodb
](
https://github.com/ityouknow/spring-boot-starter/tree/master/spring-boot-multi-mongodb
)
:spring boot和mongodb多数据源的使用
-
[
Favorites-web
](
https://github.com/cloudfavorites/favorites-web
)
:云收藏(springboot实战开源软件)
...
...
@@ -34,8 +35,9 @@ Spring boot使用的各种示例,以最简单、最实用为标准
-
[
springboot实战:我们的第一款开源软件
](
http://www.ityouknow.com/springboot/2016/09/26/springboot%E5%AE%9E%E6%88%98-%E6%88%91%E4%BB%AC%E7%9A%84%E7%AC%AC%E4%B8%80%E6%AC%BE%E5%BC%80%E6%BA%90%E8%BD%AF%E4%BB%B6.html
)
> 如果大家想了解关于springboot的其它方面应用,也可以以issues的形式反馈给我,我后续来完善。
关注公众号:纯洁的微笑,回复"springboot"进群交流
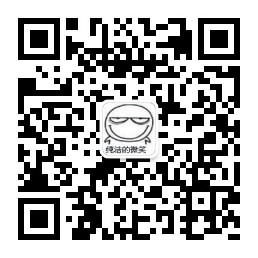
...
...
spring-boot-multi-mongodb/pom.xml
0 → 100644
浏览文件 @
4bd47ecd
<?xml version="1.0" encoding="UTF-8"?>
<project
xmlns=
"http://maven.apache.org/POM/4.0.0"
xmlns:xsi=
"http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation=
"http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"
>
<modelVersion>
4.0.0
</modelVersion>
<groupId>
com.neo
</groupId>
<artifactId>
spring-boot-multi-mongodb
</artifactId>
<version>
1.0.0
</version>
<packaging>
jar
</packaging>
<name>
spring-boot-multi-mongodb
</name>
<description>
Demo project for Spring Boot and multi mongodb
</description>
<parent>
<groupId>
org.springframework.boot
</groupId>
<artifactId>
spring-boot-starter-parent
</artifactId>
<version>
1.5.3.RELEASE
</version>
<relativePath/>
<!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>
UTF-8
</project.build.sourceEncoding>
<java.version>
1.8
</java.version>
</properties>
<dependencies>
<dependency>
<groupId>
org.springframework.boot
</groupId>
<artifactId>
spring-boot-starter-data-mongodb
</artifactId>
</dependency>
<dependency>
<groupId>
org.projectlombok
</groupId>
<artifactId>
lombok
</artifactId>
</dependency>
<dependency>
<groupId>
org.springframework.boot
</groupId>
<artifactId>
spring-boot-autoconfigure
</artifactId>
<version>
RELEASE
</version>
</dependency>
<dependency>
<groupId>
org.springframework.boot
</groupId>
<artifactId>
spring-boot-starter-test
</artifactId>
<scope>
test
</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>
org.springframework.boot
</groupId>
<artifactId>
spring-boot-maven-plugin
</artifactId>
<configuration>
<fork>
true
</fork>
</configuration>
</plugin>
</plugins>
</build>
</project>
spring-boot-multi-mongodb/src/main/java/com/neo/Application.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo
;
import
com.neo.config.props.MultipleMongoProperties
;
import
org.springframework.boot.SpringApplication
;
import
org.springframework.boot.autoconfigure.EnableAutoConfiguration
;
import
org.springframework.boot.autoconfigure.SpringBootApplication
;
import
org.springframework.boot.autoconfigure.mongo.MongoAutoConfiguration
;
import
org.springframework.boot.context.properties.EnableConfigurationProperties
;
import
org.springframework.scheduling.annotation.EnableScheduling
;
@EnableConfigurationProperties
(
MultipleMongoProperties
.
class
)
@SpringBootApplication
(
exclude
=
MongoAutoConfiguration
.
class
)
public
class
Application
{
public
static
void
main
(
String
[]
args
)
{
SpringApplication
.
run
(
Application
.
class
,
args
);
}
}
spring-boot-multi-mongodb/src/main/java/com/neo/config/MultipleMongoConfig.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.config
;
import
com.mongodb.MongoClient
;
import
com.neo.config.props.MultipleMongoProperties
;
import
org.springframework.beans.factory.annotation.Autowired
;
import
org.springframework.beans.factory.annotation.Qualifier
;
import
org.springframework.boot.autoconfigure.mongo.MongoProperties
;
import
org.springframework.context.annotation.Bean
;
import
org.springframework.context.annotation.Configuration
;
import
org.springframework.context.annotation.Primary
;
import
org.springframework.data.mongodb.MongoDbFactory
;
import
org.springframework.data.mongodb.core.MongoTemplate
;
import
org.springframework.data.mongodb.core.SimpleMongoDbFactory
;
/**
* @author neo
*/
@Configuration
public
class
MultipleMongoConfig
{
@Autowired
private
MultipleMongoProperties
mongoProperties
;
@Primary
@Bean
(
name
=
PrimaryMongoConfig
.
MONGO_TEMPLATE
)
public
MongoTemplate
primaryMongoTemplate
()
throws
Exception
{
return
new
MongoTemplate
(
primaryFactory
(
this
.
mongoProperties
.
getPrimary
()));
}
@Bean
@Qualifier
(
SecondaryMongoConfig
.
MONGO_TEMPLATE
)
public
MongoTemplate
secondaryMongoTemplate
()
throws
Exception
{
return
new
MongoTemplate
(
secondaryFactory
(
this
.
mongoProperties
.
getSecondary
()));
}
@Bean
@Primary
public
MongoDbFactory
primaryFactory
(
MongoProperties
mongo
)
throws
Exception
{
return
new
SimpleMongoDbFactory
(
new
MongoClient
(
mongo
.
getHost
(),
mongo
.
getPort
()),
mongo
.
getDatabase
());
}
@Bean
public
MongoDbFactory
secondaryFactory
(
MongoProperties
mongo
)
throws
Exception
{
return
new
SimpleMongoDbFactory
(
new
MongoClient
(
mongo
.
getHost
(),
mongo
.
getPort
()),
mongo
.
getDatabase
());
}
}
spring-boot-multi-mongodb/src/main/java/com/neo/config/PrimaryMongoConfig.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.config
;
import
org.springframework.context.annotation.Configuration
;
import
org.springframework.data.mongodb.repository.config.EnableMongoRepositories
;
/**
* @author neo
*/
@Configuration
@EnableMongoRepositories
(
basePackages
=
"com.neo.model.repository.primary"
,
mongoTemplateRef
=
PrimaryMongoConfig
.
MONGO_TEMPLATE
)
public
class
PrimaryMongoConfig
{
protected
static
final
String
MONGO_TEMPLATE
=
"primaryMongoTemplate"
;
}
spring-boot-multi-mongodb/src/main/java/com/neo/config/SecondaryMongoConfig.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.config
;
import
org.springframework.context.annotation.Configuration
;
import
org.springframework.data.mongodb.repository.config.EnableMongoRepositories
;
/**
* @author neo
*/
@Configuration
@EnableMongoRepositories
(
basePackages
=
"com.neo.model.repository.secondary"
,
mongoTemplateRef
=
SecondaryMongoConfig
.
MONGO_TEMPLATE
)
public
class
SecondaryMongoConfig
{
protected
static
final
String
MONGO_TEMPLATE
=
"secondaryMongoTemplate"
;
}
spring-boot-multi-mongodb/src/main/java/com/neo/config/props/MultipleMongoProperties.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.config.props
;
import
lombok.Data
;
import
org.springframework.boot.autoconfigure.mongo.MongoProperties
;
import
org.springframework.boot.context.properties.ConfigurationProperties
;
/**
* @author neo
*/
@Data
@ConfigurationProperties
(
prefix
=
"mongodb"
)
public
class
MultipleMongoProperties
{
private
MongoProperties
primary
=
new
MongoProperties
();
private
MongoProperties
secondary
=
new
MongoProperties
();
}
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/primary/PrimaryMongoObject.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.model.repository.primary
;
import
lombok.AllArgsConstructor
;
import
lombok.Data
;
import
lombok.NoArgsConstructor
;
import
org.springframework.data.annotation.Id
;
import
org.springframework.data.mongodb.core.mapping.Document
;
/**
* @author neo
*/
@Data
@AllArgsConstructor
@NoArgsConstructor
@Document
(
collection
=
"first_mongo"
)
public
class
PrimaryMongoObject
{
@Id
private
String
id
;
private
String
value
;
@Override
public
String
toString
()
{
return
"PrimaryMongoObject{"
+
"id='"
+
id
+
'\''
+
", value='"
+
value
+
'\''
+
'}'
;
}
}
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/primary/PrimaryRepository.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.model.repository.primary
;
import
org.springframework.data.mongodb.repository.MongoRepository
;
/**
* @author neo
*/
public
interface
PrimaryRepository
extends
MongoRepository
<
PrimaryMongoObject
,
String
>
{
}
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/secondary/SecondaryMongoObject.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.model.repository.secondary
;
import
lombok.AllArgsConstructor
;
import
lombok.Data
;
import
lombok.NoArgsConstructor
;
import
org.springframework.data.annotation.Id
;
import
org.springframework.data.mongodb.core.mapping.Document
;
/**
* @author neo
*/
@Data
@AllArgsConstructor
@NoArgsConstructor
@Document
(
collection
=
"second_mongo"
)
public
class
SecondaryMongoObject
{
@Id
private
String
id
;
private
String
value
;
@Override
public
String
toString
()
{
return
"SecondaryMongoObject{"
+
"id='"
+
id
+
'\''
+
", value='"
+
value
+
'\''
+
'}'
;
}
}
spring-boot-multi-mongodb/src/main/java/com/neo/model/repository/secondary/SecondaryRepository.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.model.repository.secondary
;
import
org.springframework.data.mongodb.repository.MongoRepository
;
/**
* @author neo
*/
public
interface
SecondaryRepository
extends
MongoRepository
<
SecondaryMongoObject
,
String
>
{
}
spring-boot-multi-mongodb/src/main/resources/application.yml
0 → 100644
浏览文件 @
4bd47ecd
mongodb
:
primary
:
host
:
192.168.9.60
port
:
20000
database
:
test
secondary
:
host
:
192.168.9.61
port
:
20000
database
:
test1
\ No newline at end of file
spring-boot-multi-mongodb/src/test/java/com/neo/ApplicationTests.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo
;
import
org.junit.Test
;
import
org.junit.runner.RunWith
;
import
org.springframework.boot.test.context.SpringBootTest
;
import
org.springframework.test.context.junit4.SpringRunner
;
@RunWith
(
SpringRunner
.
class
)
@SpringBootTest
public
class
ApplicationTests
{
@Test
public
void
contextLoads
()
{
System
.
out
.
println
(
"hello world"
);
}
}
spring-boot-multi-mongodb/src/test/java/com/neo/model/repository/MuliDatabaseTest.java
0 → 100644
浏览文件 @
4bd47ecd
package
com.neo.model.repository
;
import
com.neo.model.repository.primary.PrimaryMongoObject
;
import
com.neo.model.repository.primary.PrimaryRepository
;
import
com.neo.model.repository.secondary.SecondaryMongoObject
;
import
com.neo.model.repository.secondary.SecondaryRepository
;
import
org.junit.Test
;
import
org.junit.runner.RunWith
;
import
org.springframework.beans.factory.annotation.Autowired
;
import
org.springframework.boot.test.context.SpringBootTest
;
import
org.springframework.test.context.junit4.SpringRunner
;
import
java.util.List
;
/**
* Created by neo on 2017/5/6.
*/
@RunWith
(
SpringRunner
.
class
)
@SpringBootTest
public
class
MuliDatabaseTest
{
@Autowired
private
PrimaryRepository
primaryRepository
;
@Autowired
private
SecondaryRepository
secondaryRepository
;
@Test
public
void
TestSave
()
{
System
.
out
.
println
(
"************************************************************"
);
System
.
out
.
println
(
"测试开始"
);
System
.
out
.
println
(
"************************************************************"
);
this
.
primaryRepository
.
save
(
new
PrimaryMongoObject
(
null
,
"第一个库的对象"
));
this
.
secondaryRepository
.
save
(
new
SecondaryMongoObject
(
null
,
"第二个库的对象"
));
List
<
PrimaryMongoObject
>
primaries
=
this
.
primaryRepository
.
findAll
();
for
(
PrimaryMongoObject
primary
:
primaries
)
{
System
.
out
.
println
(
primary
.
toString
());
}
List
<
SecondaryMongoObject
>
secondaries
=
this
.
secondaryRepository
.
findAll
();
for
(
SecondaryMongoObject
secondary
:
secondaries
)
{
System
.
out
.
println
(
secondary
.
toString
());
}
System
.
out
.
println
(
"************************************************************"
);
System
.
out
.
println
(
"测试完成"
);
System
.
out
.
println
(
"************************************************************"
);
}
}
编辑
预览
Markdown
is supported
0%
请重试
或
添加新附件
.
添加附件
取消
You are about to add
0
people
to the discussion. Proceed with caution.
先完成此消息的编辑!
取消
想要评论请
注册
或
登录