- 27 3月, 2023 4 次提交
-
-
由 PMazarovich 提交于
Currently when user try to open the task with git repository connected he will see this (screenshot) 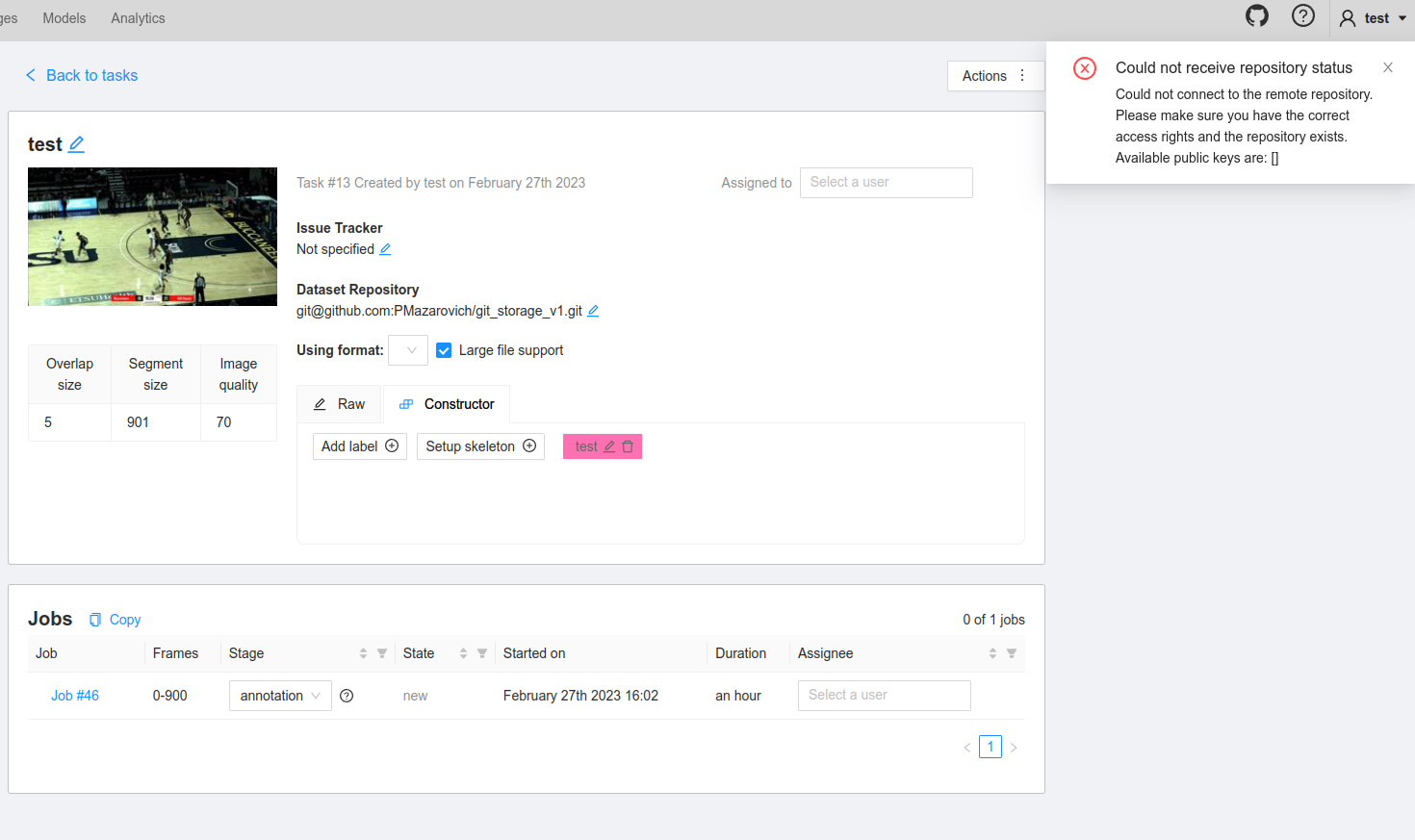 This happens after first synchronization and reloading the page. The cause of this is ssh-agent, that is not running in cvat_server container. This PR will enable ssh-agent on cvat_server so this issue disappears.
-
由 Roman Donchenko 提交于
v3 uses the `set-output` command, which will stop working on June 1st.
-
由 Roman Donchenko 提交于
Currently, if you try to generate the API schema with the VS Code launch configuration, it'll remove the `minimum` and `maximum` attributes from the definition of some of the integer fields. This is because those attributes come from the configured database engine in use. The "canonical" version of the schema is generated from the Docker image, which uses the production configuration, and thus PostgreSQL; while using the VS Code launch configuration will default to the development configuration, and thus SQLite. Fix it by aligning the configurations. Since ultimately, the schema is for the production version of the server, override the configuration to production for the VS Code launch configuration. Note that there's one more discrepancy that remains; that of the API version number. I haven't figured out the best way to fix that yet.
-
- 25 3月, 2023 5 次提交
-
-
由 Maxim Zhiltsov 提交于
Fixes https://github.com/opencv/cvat/issues/5698 It seems like `v` is missing in the version string for the last command. This PR fixes the problem.
-
由 Roman Donchenko 提交于
This version supports Python 3.10+.
-
由 Maxim Zhiltsov 提交于
This PR adds a script to autoformat Python code.
-
由 Maxim Zhiltsov 提交于
Fixes #5683 - Added a repo structure description page in docs
-
由 dependabot[bot] 提交于
Bumps [tensorflow](https://github.com/tensorflow/tensorflow) from 2.10.1 to 2.11.1. <details> <summary>Release notes</summary> <p><em>Sourced from <a href="https://github.com/tensorflow/tensorflow/releases">tensorflow's releases</a>.</em></p> <blockquote> <h2>TensorFlow 2.11.1</h2> <h1>Release 2.11.1</h1> <p><strong>Note</strong>: TensorFlow 2.10 was the last TensorFlow release that supported GPU on native-Windows. Starting with TensorFlow 2.11, you will need to install TensorFlow in WSL2, or install tensorflow-cpu and, optionally, try the TensorFlow-DirectML-Plugin.</p> <ul> <li>Security vulnerability fixes will no longer be patched to this Tensorflow version. The latest Tensorflow version includes the security vulnerability fixes. You can update to the latest version (recommended) or patch security vulnerabilities yourself <a href="https://github.com/tensorflow/tensorflow#patching-guidelines">steps</a>. You can refer to the <a href="https://github.com/tensorflow/tensorflow/releases">release notes</a> of the latest Tensorflow version for a list of newly fixed vulnerabilities. If you have any questions, please create a GitHub issue to let us know.</li> </ul> <p>This release also introduces several vulnerability fixes:</p> <ul> <li>Fixes an FPE in TFLite in conv kernel <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-27579">CVE-2023-27579</a></li> <li>Fixes a double free in Fractional(Max/Avg)Pool <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25801">CVE-2023-25801</a></li> <li>Fixes a null dereference on ParallelConcat with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25676">CVE-2023-25676</a></li> <li>Fixes a segfault in Bincount with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25675">CVE-2023-25675</a></li> <li>Fixes an NPE in RandomShuffle with XLA enable <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25674">CVE-2023-25674</a></li> <li>Fixes an FPE in TensorListSplit with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25673">CVE-2023-25673</a></li> <li>Fixes segmentation fault in tfg-translate <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25671">CVE-2023-25671</a></li> <li>Fixes an NPE in QuantizedMatMulWithBiasAndDequantize <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25670">CVE-2023-25670</a></li> <li>Fixes an FPE in AvgPoolGrad with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25669">CVE-2023-25669</a></li> <li>Fixes a heap out-of-buffer read vulnerability in the QuantizeAndDequantize operation <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25668">CVE-2023-25668</a></li> <li>Fixes a segfault when opening multiframe gif <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25667">CVE-2023-25667</a></li> <li>Fixes an NPE in SparseSparseMaximum <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25665">CVE-2023-25665</a></li> <li>Fixes an FPE in AudioSpectrogram <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25666">CVE-2023-25666</a></li> <li>Fixes a heap-buffer-overflow in AvgPoolGrad <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25664">CVE-2023-25664</a></li> <li>Fixes a NPE in TensorArrayConcatV2 <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25663">CVE-2023-25663</a></li> <li>Fixes a Integer overflow in EditDistance <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25662">CVE-2023-25662</a></li> <li>Fixes a Seg fault in <code>tf.raw_ops.Print</code> <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25660">CVE-2023-25660</a></li> <li>Fixes a OOB read in DynamicStitch <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25659">CVE-2023-25659</a></li> <li>Fixes a OOB Read in GRUBlockCellGrad <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25658">CVE-2023-25658</a></li> </ul> <h2>TensorFlow 2.11.0</h2> <h1>Release 2.11.0</h1> <h2>Breaking Changes</h2> <ul> <li> <p>The <code>tf.keras.optimizers.Optimizer</code> base class now points to the new Keras optimizer, while the old optimizers have been moved to the <code>tf.keras.optimizers.legacy</code> namespace.</p> <p>If you find your workflow failing due to this change, you may be facing one of the following issues:</p> <ul> <li><strong>Checkpoint loading failure.</strong> The new optimizer handles optimizer state differently from the old optimizer, which simplifies the logic of checkpoint saving/loading, but at the cost of breaking checkpoint backward compatibility in some cases. If you want to keep using an old checkpoint, please change your optimizer to <code>tf.keras.optimizer.legacy.XXX</code> (e.g. <code>tf.keras.optimizer.legacy.Adam</code>).</li> <li><strong>TF1 compatibility.</strong> The new optimizer, <code>tf.keras.optimizers.Optimizer</code>, does not support TF1 any more, so please use the legacy optimizer <code>tf.keras.optimizer.legacy.XXX</code>. We highly recommend <a href="https://www.tensorflow.org/guide/migrate">migrating your workflow to TF2</a> for stable support and new features.</li> <li><strong>Old optimizer API not found.</strong> The new optimizer, <code>tf.keras.optimizers.Optimizer</code>, has a different set of public APIs from the old optimizer. These API changes are mostly related to getting rid of slot variables and TF1 support. Please check the API documentation to find alternatives to the missing API. If you must call the deprecated API, please change your optimizer to the legacy optimizer.</li> <li><strong>Learning rate schedule access.</strong> When using a <code>tf.keras.optimizers.schedules.LearningRateSchedule</code>, the new optimizer's <code>learning_rate</code> property returns the current learning rate value instead of a <code>LearningRateSchedule</code> object as before. If you need to access the <code>LearningRateSchedule</code> object, please use <code>optimizer._learning_rate</code>.</li> <li><strong>If you implemented a custom optimizer based on the old optimizer.</strong> Please set your optimizer to subclass <code>tf.keras.optimizer.legacy.XXX</code>. If you want to migrate to the new optimizer and find it does not support your optimizer, please file an issue in the <a href="https://github.com/keras-team/keras/issues">Keras GitHub repo</a>.</li> <li><strong>Errors, such as <code>Cannot recognize variable...</code>.</strong> The new optimizer requires all optimizer variables to be created at the first <code>apply_gradients()</code> or <code>minimize()</code> call. If your workflow calls the optimizer to update different parts of the model in multiple stages, please call <code>optimizer.build(model.trainable_variables)</code> before the training loop.</li> <li><strong>Timeout or performance loss.</strong> We don't anticipate this to happen, but if you see such issues, please use the legacy optimizer, and file an issue in the Keras GitHub repo.</li> </ul> <p>The old Keras optimizer will never be deleted, but will not see any new feature additions. New optimizers (for example, <code>tf.keras.optimizers.Adafactor</code>) will only be implemented based on the new <code>tf.keras.optimizers.Optimizer</code> base class.</p> </li> <li> <p><code>tensorflow/python/keras</code> code is a legacy copy of Keras since the TensorFlow v2.7 release, and will be deleted in the v2.12 release. Please remove any import of <code>tensorflow.python.keras</code> and use the public API with <code>from tensorflow import keras</code> or <code>import tensorflow as tf; tf.keras</code>.</p> </li> </ul> <h2>Major Features and Improvements</h2> <!-- raw HTML omitted --> </blockquote> <p>... (truncated)</p> </details> <details> <summary>Changelog</summary> <p><em>Sourced from <a href="https://github.com/tensorflow/tensorflow/blob/master/RELEASE.md">tensorflow's changelog</a>.</em></p> <blockquote> <h1>Release 2.11.1</h1> <p><strong>Note</strong>: TensorFlow 2.10 was the last TensorFlow release that supported GPU on native-Windows. Starting with TensorFlow 2.11, you will need to install TensorFlow in WSL2, or install tensorflow-cpu and, optionally, try the TensorFlow-DirectML-Plugin.</p> <ul> <li>Security vulnerability fixes will no longer be patched to this Tensorflow version. The latest Tensorflow version includes the security vulnerability fixes. You can update to the latest version (recommended) or patch security vulnerabilities yourself <a href="https://github.com/tensorflow/tensorflow#patching-guidelines">steps</a>. You can refer to the <a href="https://github.com/tensorflow/tensorflow/releases">release notes</a> of the latest Tensorflow version for a list of newly fixed vulnerabilities. If you have any questions, please create a GitHub issue to let us know.</li> </ul> <p>This release also introduces several vulnerability fixes:</p> <ul> <li>Fixes an FPE in TFLite in conv kernel <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-27579">CVE-2023-27579</a></li> <li>Fixes a double free in Fractional(Max/Avg)Pool <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25801">CVE-2023-25801</a></li> <li>Fixes a null dereference on ParallelConcat with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25676">CVE-2023-25676</a></li> <li>Fixes a segfault in Bincount with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25675">CVE-2023-25675</a></li> <li>Fixes an NPE in RandomShuffle with XLA enable <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25674">CVE-2023-25674</a></li> <li>Fixes an FPE in TensorListSplit with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25673">CVE-2023-25673</a></li> <li>Fixes segmentation fault in tfg-translate <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25671">CVE-2023-25671</a></li> <li>Fixes an NPE in QuantizedMatMulWithBiasAndDequantize <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25670">CVE-2023-25670</a></li> <li>Fixes an FPE in AvgPoolGrad with XLA <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25669">CVE-2023-25669</a></li> <li>Fixes a heap out-of-buffer read vulnerability in the QuantizeAndDequantize operation <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25668">CVE-2023-25668</a></li> <li>Fixes a segfault when opening multiframe gif <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25667">CVE-2023-25667</a></li> <li>Fixes an NPE in SparseSparseMaximum <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25665">CVE-2023-25665</a></li> <li>Fixes an FPE in AudioSpectrogram <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25666">CVE-2023-25666</a></li> <li>Fixes a heap-buffer-overflow in AvgPoolGrad <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25664">CVE-2023-25664</a></li> <li>Fixes a NPE in TensorArrayConcatV2 <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25663">CVE-2023-25663</a></li> <li>Fixes a Integer overflow in EditDistance <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25662">CVE-2023-25662</a></li> <li>Fixes a Seg fault in <code>tf.raw_ops.Print</code> <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25660">CVE-2023-25660</a></li> <li>Fixes a OOB read in DynamicStitch <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25659">CVE-2023-25659</a></li> <li>Fixes a OOB Read in GRUBlockCellGrad <a href="https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2023-25658">CVE-2023-25658</a></li> </ul> <h1>Release 2.11.0</h1> <h2>Breaking Changes</h2> <ul> <li> <p><code>tf.keras.optimizers.Optimizer</code> now points to the new Keras optimizer, and old optimizers have moved to the <code>tf.keras.optimizers.legacy</code> namespace. If you find your workflow failing due to this change, you may be facing one of the following issues:</p> <ul> <li><strong>Checkpoint loading failure.</strong> The new optimizer handles optimizer state differently from the old optimizer, which simplies the logic of checkpoint saving/loading, but at the cost of breaking checkpoint backward compatibility in some cases. If you want to keep using an old checkpoint, please change your optimizer to <code>tf.keras.optimizers.legacy.XXX</code> (e.g. <code>tf.keras.optimizers.legacy.Adam</code>).</li> <li><strong>TF1 compatibility.</strong> The new optimizer does not support TF1 any more, so please use the legacy optimizer <code>tf.keras.optimizer.legacy.XXX</code>. We highly recommend to migrate your workflow to TF2 for stable support and new features.</li> <li><strong>API not found.</strong> The new optimizer has a different set of public APIs from the old optimizer. These API changes are mostly related to getting rid of slot variables and TF1 support. Please check the API</li> </ul> </li> </ul> <!-- raw HTML omitted --> </blockquote> <p>... (truncated)</p> </details> <details> <summary>Commits</summary> <ul> <li><a href="https://github.com/tensorflow/tensorflow/commit/a3e2c692c18649329c4210cf8df2487d2028e267"><code>a3e2c69</code></a> Merge pull request <a href="https://redirect.github.com/tensorflow/tensorflow/issues/60016">#60016</a> from tensorflow/fix-relnotes</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/13b85dcf966d0c94b2e5c21291be039db2dec7b9"><code>13b85dc</code></a> Fix release notes</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/48b18dbf1301f24be9f2f41189d318ce5398540a"><code>48b18db</code></a> Merge pull request <a href="https://redirect.github.com/tensorflow/tensorflow/issues/60014">#60014</a> from tensorflow/disable-test-that-ooms</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/eea48f50d6982879909bf8e0d0151bbce3f9bf4a"><code>eea48f5</code></a> Disable a test that results in OOM+segfault</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/a63258434247784605986cfc2b43cb3be846cf8a"><code>a632584</code></a> Merge pull request <a href="https://redirect.github.com/tensorflow/tensorflow/issues/60000">#60000</a> from tensorflow/venkat-patch-3</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/93dea7a67df44bde557e580dfdcde5ba0a7a344d"><code>93dea7a</code></a> Update RELEASE.md</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/a2ba9f16f0154bf93f21132878b154238d89fad6"><code>a2ba9f1</code></a> Updating Release.md with Legal Language for Release Notes</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/fae41c76bdc760454b3e5c1d3af9b8d5a5c6c548"><code>fae41c7</code></a> Merge pull request <a href="https://redirect.github.com/tensorflow/tensorflow/issues/59998">#59998</a> from tensorflow/fix-bad-cherrypick-again</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/2757416dcd4a2d00ea36512c2ffd347030c1196b"><code>2757416</code></a> Fix bad cherrypick</li> <li><a href="https://github.com/tensorflow/tensorflow/commit/c78616f4b00125c8a563e10ce6b76bea8070bdd0"><code>c78616f</code></a> Merge pull request <a href="https://redirect.github.com/tensorflow/tensorflow/issues/59992">#59992</a> from tensorflow/fix-2.11-build</li> <li>Additional commits viewable in <a href="https://github.com/tensorflow/tensorflow/compare/v2.10.1...v2.11.1">compare view</a></li> </ul> </details> <br /> [](https://docs.github.com/en/github/managing-security-vulnerabilities/about-dependabot-security-updates#about-compatibility-scores) Dependabot will resolve any conflicts with this PR as long as you don't alter it yourself. You can also trigger a rebase manually by commenting `@dependabot rebase`. [//]: # (dependabot-automerge-start) [//]: # (dependabot-automerge-end) --- <details> <summary>Dependabot commands and options</summary> <br /> You can trigger Dependabot actions by commenting on this PR: - `@dependabot rebase` will rebase this PR - `@dependabot recreate` will recreate this PR, overwriting any edits that have been made to it - `@dependabot merge` will merge this PR after your CI passes on it - `@dependabot squash and merge` will squash and merge this PR after your CI passes on it - `@dependabot cancel merge` will cancel a previously requested merge and block automerging - `@dependabot reopen` will reopen this PR if it is closed - `@dependabot close` will close this PR and stop Dependabot recreating it. You can achieve the same result by closing it manually - `@dependabot ignore this major version` will close this PR and stop Dependabot creating any more for this major version (unless you reopen the PR or upgrade to it yourself) - `@dependabot ignore this minor version` will close this PR and stop Dependabot creating any more for this minor version (unless you reopen the PR or upgrade to it yourself) - `@dependabot ignore this dependency` will close this PR and stop Dependabot creating any more for this dependency (unless you reopen the PR or upgrade to it yourself) You can disable automated security fix PRs for this repo from the [Security Alerts page](https://github.com/opencv/cvat/network/alerts). </details> Signed-off-by: Ndependabot[bot] <support@github.com> Co-authored-by: Ndependabot[bot] <49699333+dependabot[bot]@users.noreply.github.com>
-
- 24 3月, 2023 8 次提交
-
-
由 Roman Donchenko 提交于
The `CVAT_NUCLIO_FUNCTION_NAMESPACE` needs to be defined consistently in order for Nuclio integration to work. Currently, it's set to `cvat` for the main CVAT server process, but not for any other CVAT process (which means it defaults to `nuclio` in those processes). Since it's the annotation worker process that actually invokes the Nuclio functions, the invocation fails. Fix it by synchronizing the Nuclio environment variables across all backend deployments. Technically, I think only the server and annotation worker deployments need these variables, but since they're accessed by `cvat/settings/base.py` in every process that loads Django, define them everywhere to be sure. Fixes #5626.
-
由 Kirill Sizov 提交于
-
由 Boris Sekachev 提交于
Resolved #5564
-
由 Boris Sekachev 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context Resolved #5332 Related #5904 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [x] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [x] I have added tests to cover my changes - [x] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
由 Kirill Lakhov 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> Resolves #5615 - [x] Fix default preview resizing for all resources - [x] Fix loding preview ux 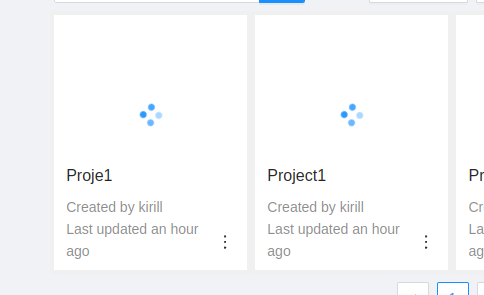 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [x] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - ~~[ ] I have updated the documentation accordingly~~ - ~~[ ] I have added tests to cover my changes~~ - [x] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
由 Boris Sekachev 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context Resolved #5845 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [x] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
由 Boris Sekachev 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context Helpful materials: https://github.com/jaredpalmer/cypress-image-snapshot/issues/143 https://www.cypress.io/blog/2021/03/01/generate-high-resolution-videos-and-screenshots ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [ ] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [ ] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
由 Rodrigo Berriel 提交于
HRNet uses `np.int` which throws an error for NumPy >= 1.24, because its deprecation was finally expired. This PR creates an additional step during HRNet function image build, downgrading NumPy for a version < 1.24. ### Motivation and context Closes #5571.
-
- 23 3月, 2023 1 次提交
-
-
由 Boris Sekachev 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context Resolved #4289 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [x] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [x] I have added tests to cover my changes - [x] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
- 22 3月, 2023 4 次提交
-
-
由 Kirill Lakhov 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> - [x] Resolved #5381 - [x] Fixed warining occured when opening export modal 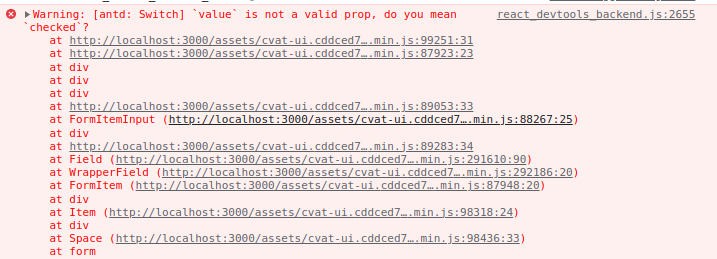 - [x] Fixed ui issue  ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - ~~[ ] I have updated the documentation accordingly~~ - [ ] I have added tests to cover my changes - [x] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [ ] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern. --------- Co-authored-by: NBoris Sekachev <boris.sekachev@yandex.ru>
-
由 Anastasia Yasakova 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> Fixed #5828 - Fixed mask annotations in CVAT format - Updated documentation ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [ ] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [ ] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern. --------- Co-authored-by: NBoris Sekachev <boris.sekachev@yandex.ru>
-
由 Boris Sekachev 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context Related #5769 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [x] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
由 Anastasia Yasakova 提交于
Fixed #5877
-
- 21 3月, 2023 5 次提交
-
-
由 Kirill Sizov 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context Fixed #5767 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [ ] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [ ] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [ ] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern. --------- Co-authored-by: NBoris Sekachev <sekachev.bs@gmail.com>
-
由 Andrey Zhavoronkov 提交于
-
由 suzu 提交于
This is a change to support Connection String authentication for Azure Blob Storage. For example, Connection String Authentication allows for Azure Blob Storage running on the IoT Edge of an edge device. ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> I tested it by actually running it as shown in the next image, I could not find where the E2E test for cloudstorage was done. <img width="1001" alt="cvat_blob_connection_string" src="https://user-images.githubusercontent.com/10334593/169458508-cfa4030a-578f-4aad-bfd9-fa01c9ca8230.png">
-
由 Andrey Zhavoronkov 提交于
-
由 Boris Sekachev 提交于
-
- 20 3月, 2023 1 次提交
-
-
由 Roman Donchenko 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> This is mostly just refactoring, but there are a couple of bugfixes: * `deleteTask` now correctly sets the `org` parameter (although I don't know why this parameter is needed to begin with); * `getCloudStorageContent` now works correctly when the manifest name contains special characters. ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> Manual testing. ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - ~~[ ] I have updated the documentation accordingly~~ - ~~[ ] I have added tests to cover my changes~~ - ~~[ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword))~~ - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern. --------- Co-authored-by: NBoris Sekachev <boris.sekachev@yandex.ru>
-
- 19 3月, 2023 2 次提交
-
-
由 Snyk bot 提交于
<h3>Snyk has created this PR to fix one or more vulnerable packages in the `pip` dependencies of this project.</h3> #### Changes included in this PR - Changes to the following files to upgrade the vulnerable dependencies to a fixed version: - cvat/requirements/base.txt #### Vulnerabilities that will be fixed ##### By pinning: Severity | Priority Score (*) | Issue | Upgrade | Breaking Change | Exploit Maturity :-------------------------:|-------------------------|:-------------------------|:-------------------------|:-------------------------|:-------------------------  | **506/1000** <br/> **Why?** Proof of Concept exploit, Has a fix available, CVSS 3.7 | NULL Pointer Dereference <br/>[SNYK-PYTHON-NUMPY-2321964](https://snyk.io/vuln/SNYK-PYTHON-NUMPY-2321964) | `numpy:` <br> `1.21.3 -> 1.22.2` <br> | No | Proof of Concept  | **399/1000** <br/> **Why?** Has a fix available, CVSS 3.7 | Buffer Overflow <br/>[SNYK-PYTHON-NUMPY-2321966](https://snyk.io/vuln/SNYK-PYTHON-NUMPY-2321966) | `numpy:` <br> `1.21.3 -> 1.22.2` <br> | No | No Known Exploit  | **506/1000** <br/> **Why?** Proof of Concept exploit, Has a fix available, CVSS 3.7 | Denial of Service (DoS) <br/>[SNYK-PYTHON-NUMPY-2321970](https://snyk.io/vuln/SNYK-PYTHON-NUMPY-2321970) | `numpy:` <br> `1.21.3 -> 1.22.2` <br> | No | Proof of Concept  | **/1000** <br/> **Why?** | Out-of-Bounds <br/>[SNYK-PYTHON-TENSORFLOW-3136280](https://snyk.io/vuln/SNYK-PYTHON-TENSORFLOW-3136280) | `tensorflow:` <br> `2.9.3 -> 2.10.1` <br> | No | No Known Exploit  | **/1000** <br/> **Why?** | Regular Expression Denial of Service (ReDoS) <br/>[SNYK-PYTHON-WHEEL-3180413](https://snyk.io/vuln/SNYK-PYTHON-WHEEL-3180413) | `wheel:` <br> `0.30.0 -> 0.38.0` <br> | No | No Known Exploit (*) Note that the real score may have changed since the PR was raised. Some vulnerabilities couldn't be fully fixed and so Snyk will still find them when the project is tested again. This may be because the vulnerability existed within more than one direct dependency, but not all of the affected dependencies could be upgraded. Check the changes in this PR to ensure they won't cause issues with your project. ------------ **Note:** *You are seeing this because you or someone else with access to this repository has authorized Snyk to open fix PRs.* For more information: <img src="https://api.segment.io/v1/pixel/track?data=eyJ3cml0ZUtleSI6InJyWmxZcEdHY2RyTHZsb0lYd0dUcVg4WkFRTnNCOUEwIiwiYW5vbnltb3VzSWQiOiJkNWY4N2RkOS1kOTIzLTRhMDYtODdkMS0zNjU0NjNkMmUyZDciLCJldmVudCI6IlBSIHZpZXdlZCIsInByb3BlcnRpZXMiOnsicHJJZCI6ImQ1Zjg3ZGQ5LWQ5MjMtNGEwNi04N2QxLTM2NTQ2M2QyZTJkNyJ9fQ==" width="0" height="0"/> 🧐 [View latest project report](https://app.snyk.io/org/cvat/project/4bbc4b80-3fb9-4009-a7bb-51016d44946b?utm_source=github&utm_medium=referral&page=fix-pr)
🛠 [Adjust project settings](https://app.snyk.io/org/cvat/project/4bbc4b80-3fb9-4009-a7bb-51016d44946b?utm_source=github&utm_medium=referral&page=fix-pr/settings)📚 [Read more about Snyk's upgrade and patch logic](https://support.snyk.io/hc/en-us/articles/360003891078-Snyk-patches-to-fix-vulnerabilities) [//]: # (snyk:metadata:{"prId":"d5f87dd9-d923-4a06-87d1-365463d2e2d7","prPublicId":"d5f87dd9-d923-4a06-87d1-365463d2e2d7","dependencies":[{"name":"numpy","from":"1.21.3","to":"1.22.2"},{"name":"tensorflow","from":"2.9.3","to":"2.10.1"},{"name":"wheel","from":"0.30.0","to":"0.38.0"}],"packageManager":"pip","projectPublicId":"4bbc4b80-3fb9-4009-a7bb-51016d44946b","projectUrl":"https://app.snyk.io/org/cvat/project/4bbc4b80-3fb9-4009-a7bb-51016d44946b?utm_source=github&utm_medium=referral&page=fix-pr","type":"auto","patch":[],"vulns":["SNYK-PYTHON-NUMPY-2321964","SNYK-PYTHON-NUMPY-2321966","SNYK-PYTHON-NUMPY-2321970","SNYK-PYTHON-TENSORFLOW-3136280","SNYK-PYTHON-WHEEL-3180413"],"upgrade":[],"isBreakingChange":false,"env":"prod","prType":"fix","templateVariants":["priorityScore"],"priorityScoreList":[506,399,506,null,null],"remediationStrategy":"vuln"}) --- **Learn how to fix vulnerabilities with free interactive lessons:**🦉 [NULL Pointer Dereference](https://learn.snyk.io/lessons/null-dereference/cpp/?loc=fix-pr)🦉 [Denial of Service (DoS)](https://learn.snyk.io/lessons/redos/javascript/?loc=fix-pr) -
由 Roman Donchenko 提交于
-
- 18 3月, 2023 2 次提交
-
-
由 Roman Donchenko 提交于
The full workflow is broken, because some changes from the main workflow weren't applied to it. Update them both to minimize differences between them.
-
由 Andrey Zhavoronkov 提交于
-
- 17 3月, 2023 2 次提交
-
-
由 Roman Donchenko 提交于
-
由 Kirill Lakhov 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> 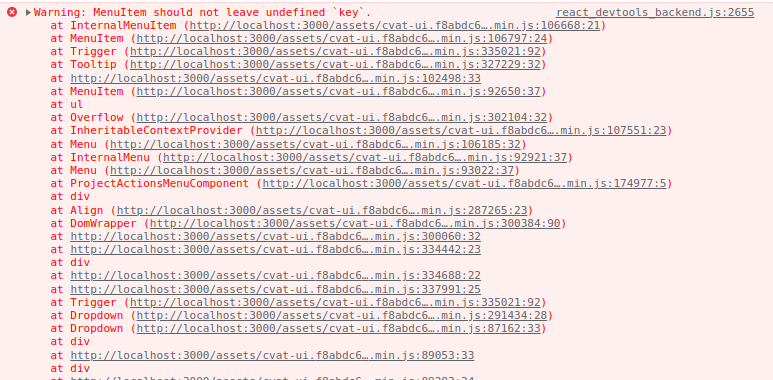 ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [x] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - ~~[ ] I have updated the documentation accordingly~~ - ~~[ ] I have added tests to cover my changes~~ - ~~[ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword))~~ - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern.
-
- 16 3月, 2023 6 次提交
-
-
由 Maria Khrustaleva 提交于
-
由 Snyk bot 提交于
-
由 Maxim Zhiltsov 提交于
-
由 Boris Sekachev 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [x] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [x] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [x] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern. # How to add your own plugin **1. Write a ``PluginBuilder``** ``PluginBuilder`` is a function that accepts the following object as an argument: ```js { dispatch, REGISTER_ACTION, REMOVE_ACTION, core, } ``` This object is passed from the core application. Where: - ``dispatch`` is a redux function that can be used to add any React components - ``REGISTER_ACTION`` is action name to append component - ``REMOVE_ACTION`` is action name to remove component - ``core`` core library to access to server and interaction with any available API, e.g. ``core.tasks.get()``, ``core.server.request('dummy/url/...', { method: 'POST', data: { param1: 'value1', param2: 'value2' } })`` If you want to include authorization headers to the request, you must do the request via the core library. ``PluginBuilder`` must return the following object ``` { name: string; destructor: CallableFunction; } ``` - ``name`` is a plugin name - ``destructor`` is a function that removes plugin from storage and does any destructive actions ``PluginBuilder`` might register additional components this way: ```tsx const Component = () => <Button> Press me </Button>; dispatch({ type: REGISTER_ACTION, payload: { path: 'loginPage.loginForm', // path must be supported by the core application component: Component, data: { // optional method, define if necessary to render component conditionally, based on props, state of a target component shouldBeRendered: (targetComponentProps, targetComponentState) => { return true; } // optional field, define if necessary to affect rendering order weight: 5, } } }); ``` Destructor callback of a ``PluginBuilder`` must remove components this way: ```ts dispatch({ type: REMOVE_ACTION, payload: { path: 'loginPage.loginForm', // the same path as when register component: Component, // the same component as when register } }); ``` **2. Define plugin entrypoint** It must be in ``<plugin_directory>/src/ts/index.tsx``. Plugin entrypoint might register plugin in the core application when ``plugins.ready`` event is triggered. To achieve that, pass ``PluginBuilder`` to the exposed method: ``window.cvatUI.registerComponent(PluginBuilder)``. In general plugin can register itself anytime, but the above method must be available. Example code is below: ```ts function register() { if (Object.prototype.hasOwnProperty.call(window, 'cvatUI')) { (window as any as { cvatUI: { registerComponent: PluginEntryPoint } }) .cvatUI.registerComponent(PluginBuilder); } }; window.addEventListener('plugins.ready', register, { once: true }); ``` **3. Build/run core application together with plugins:** Just pass ``CLIENT_PLUGINS`` env variable to webpack. It can include multiple plugins: ```sh CLIENT_PLUGINS="path/to/plugin1:path/to/plugin2:path/to/plugin3" yarn start:cvat-ui CLIENT_PLUGINS="path/to/plugin1:path/to/plugin2:path/to/plugin3" yarn build:cvat-ui ``` Path may be defined in two formats: - relative to ``cvat-ui`` directory: ``plugins/plugin1``, ``../../another_place/plugin2`` - absolute, including entrypoint file: ``/home/user/some_path/plugin/src/ts/index.tsx`` **Webpack defines two aliases:** ``@modules`` - to use dependencies of the core application For example React may be imported this way: ```ts import React from '@modules/react'; ``` ``@root`` - to import something from the core application ```ts import { CombinedState } from '@root/reducers'; ``` You can install other dependencies to plugin directory if necessary. To avoid typescript errors in IDE and working with types, you can add ``tsconfig.json``. ```json { "compilerOptions": { "target": "es2020", "baseUrl": ".", "paths": { "@modules/*": ["/path/to/cvat/node_modules/*"], "@root/*": ["path/to/cvat/cvat-ui/src/*"] }, "moduleResolution": "node", "lib": ["dom", "dom.iterable", "esnext"], "jsx": "react", } } ```
-
由 Mariia Acoca 提交于
<!-- Raise an issue to propose your change (https://github.com/opencv/cvat/issues). It helps to avoid duplication of efforts from multiple independent contributors. Discuss your ideas with maintainers to be sure that changes will be approved and merged. Read the [Contribution guide](https://opencv.github.io/cvat/docs/contributing/). --> <!-- Provide a general summary of your changes in the Title above --> ### Motivation and context <!-- Why is this change required? What problem does it solve? If it fixes an open issue, please link to the issue here. Describe your changes in detail, add screenshots. --> ### How has this been tested? <!-- Please describe in detail how you tested your changes. Include details of your testing environment, and the tests you ran to see how your change affects other areas of the code, etc. --> ### Checklist <!-- Go over all the following points, and put an `x` in all the boxes that apply. If an item isn't applicable for some reason, then ~~explicitly strikethrough~~ the whole line. If you don't do that, GitHub will show incorrect progress for the pull request. If you're unsure about any of these, don't hesitate to ask. We're here to help! --> - [ ] I submit my changes into the `develop` branch - [ ] I have added a description of my changes into the [CHANGELOG](https://github.com/opencv/cvat/blob/develop/CHANGELOG.md) file - [ ] I have updated the documentation accordingly - [ ] I have added tests to cover my changes - [ ] I have linked related issues (see [GitHub docs]( https://help.github.com/en/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword)) - [ ] I have increased versions of npm packages if it is necessary ([cvat-canvas](https://github.com/opencv/cvat/tree/develop/cvat-canvas#versioning), [cvat-core](https://github.com/opencv/cvat/tree/develop/cvat-core#versioning), [cvat-data](https://github.com/opencv/cvat/tree/develop/cvat-data#versioning) and [cvat-ui](https://github.com/opencv/cvat/tree/develop/cvat-ui#versioning)) ### License - [ ] I submit _my code changes_ under the same [MIT License]( https://github.com/opencv/cvat/blob/develop/LICENSE) that covers the project. Feel free to contact the maintainers if that's a concern. --------- Co-authored-by: NBoris Sekachev <boris.sekachev@yandex.ru>
-
由 Andrey Zhavoronkov 提交于
-