diff --git a/JSReverse/www_gm99_com/README.md b/JSReverse/www_gm99_com/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..4a6d403d92c0bdd486ebd2a84b9b3fa1c7ffaf51
--- /dev/null
+++ b/JSReverse/www_gm99_com/README.md
@@ -0,0 +1,456 @@
+## 【JS 逆向百例】webpack 改写实战,G 某游戏 RSA 加密
+
+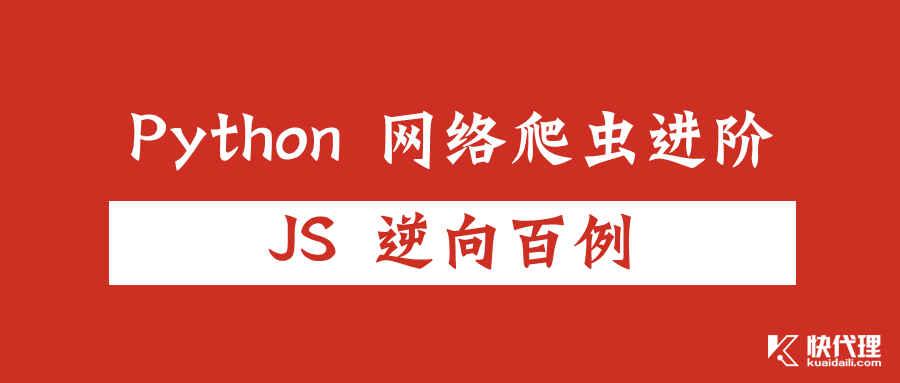
+
+> 关注微信公众号:K哥爬虫,QQ交流群:808574309,持续分享爬虫进阶、JS/安卓逆向等技术干货!
+
+## 声明
+
+**本文章中所有内容仅供学习交流,抓包内容、敏感网址、数据接口均已做脱敏处理,严禁用于商业用途和非法用途,否则由此产生的一切后果均与作者无关,若有侵权,请联系我立即删除!**
+
+## 逆向目标
+
+- 目标:G某游戏登录
+- 主页:`aHR0cHM6Ly93d3cuZ205OS5jb20v`
+- 接口:`aHR0cHM6Ly9wYXNzcG9ydC5nbTk5LmNvbS9sb2dpbi9sb2dpbjM=`
+- 逆向参数:
+ Query String Parameters:
+ ```text
+ password: kRtqfg41ogc8btwGlEw6nWLg8cHcCW6R8JaeM......
+ ```
+
+## 逆向过程
+
+### 抓包分析
+
+来到首页,随便输入一个账号密码,点击登陆,抓包定位到登录接口为 `aHR0cHM6Ly9wYXNzcG9ydC5nbTk5LmNvbS9sb2dpbi9sb2dpbjM=`,GET 请求,Query String Parameters 里,密码 password 被加密处理了。
+
+
+
+### 加密入口
+
+直接搜索关键字 password 会发现结果太多不好定位,使用 XHR 断点比较容易定位到加密入口,有关 XHR 断点调试可以查看 K 哥往期的教程:[【JS 逆向百例】XHR 断点调试,Steam 登录逆向](https://mp.weixin.qq.com/s/DPNtkF9e1pvFVa1m-DsyJw),如下图所示,在 home.min.js 里可以看到关键语句 `a.encode(t.password, s)`,`t.password` 是明文密码,`s` 是时间戳。
+
+
+
+跟进 `a.encode()` 函数,此函数仍然在 home.min.js 里,观察这部分代码,可以发现使用了 JSEncrypt,并且有 setPublicKey 设置公钥方法,由此可以看出应该是 RSA 加密,具体步骤是将明文密码和时间戳组合成用 | 组合,经过 RSA 加密后再进行 URL 编码得到最终结果,如下图所示:
+
+
+
+RSA 加密找到了公钥,其实就可以直接使用 Python 的 Cryptodome 模块来实现加密过程了,代码如下所示:
+
+```python
+import time
+import base64
+from urllib import parse
+from Cryptodome.PublicKey import RSA
+from Cryptodome.Cipher import PKCS1_v1_5
+
+
+password = "12345678"
+timestamp = str(int(time.time() * 1000))
+encrypted_object = timestamp + "|" + password
+public_key = "MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDq04c6My441Gj0UFKgrqUhAUg+kQZeUeWSPlAU9fr4HBPDldAeqzx1UR92KJHuQh/zs1HOamE2dgX9z/2oXcJaqoRIA/FXysx+z2YlJkSk8XQLcQ8EBOkp//MZrixam7lCYpNOjadQBb2Ot0U/Ky+jF2p+Ie8gSZ7/u+Wnr5grywIDAQAB"
+rsa_key = RSA.import_key(base64.b64decode(public_key)) # 导入读取到的公钥
+cipher = PKCS1_v1_5.new(rsa_key) # 生成对象
+encrypted_password = base64.b64encode(cipher.encrypt(encrypted_object.encode(encoding="utf-8")))
+encrypted_password = parse.quote(encrypted_password)
+print(encrypted_password)
+```
+
+即便是不使用 Python,我们同样可以自己引用 JSEncrypt 模块来实现这个加密过程(该模块使用方法可参考 [JSEncrypt GitHub](https://github.com/travist/jsencrypt)),如下所示:
+
+```javascript
+/*
+引用 jsencrypt 加密模块,如果在 PyCharm 里直接使用 require 引用最新版 jsencrypt,
+运行可能会提示 jsencrypt.js 里 window 未定义,直接在该文件定义 var window = this; 即可,
+也可以使用和网站用的一样的 2.3.1 版本:https://npmcdn.com/jsencrypt@2.3.1/bin/jsencrypt.js
+也可以将 jsencrypt.js 直接粘贴到此脚本中使用,如果提示未定义,直接在该脚本中定义即可。
+*/
+
+JSEncrypt = require("jsencrypt")
+
+function getEncryptedPassword(t, e) {
+ var jsEncrypt = new JSEncrypt();
+ jsEncrypt.setPublicKey('MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDq04c6My441Gj0UFKgrqUhAUg+kQZeUeWSPlAU9fr4HBPDldAeqzx1UR92KJHuQh/zs1HOamE2dgX9z/2oXcJaqoRIA/FXysx+z2YlJkSk8XQLcQ8EBOkp//MZrixam7lCYpNOjadQBb2Ot0U/Ky+jF2p+Ie8gSZ7/u+Wnr5grywIDAQAB');
+ var i = e ? e + "|" + t : t;
+ return encodeURIComponent(jsEncrypt.encrypt(i));
+}
+
+var password = "12345678";
+var timestamp = (new Date).getTime();
+console.log(getEncryptedPassword(password, timestamp));
+```
+
+### webpack 改写
+
+本文的标题是 webpack 改写实战,所以很显然本文的目的是为了练习 JavaScript 模块化编程 webpack 代码的改写,现在大多数站点都使用了这种写法,然而并不是所有站点都像本文遇到的站点一样,可以很容易使用其他方法来实现的,往往大多数站点需要你自己扒下他的源码来还原加密过程,有关 JavaScript 模块化编程,即 webpack,在 K 哥往期的文章中有过详细的介绍:xxxxxxxxx
+
+一个标准的 webpack 整体是一个 IIFE 立即调用函数表达式,其中有一个模块加载器,也就是调用模块的函数,该函数中一般具有 `function.call()` 或者 `function.apply()` 方法,IIFE 传递的参数是一个列表或者字典,里面是一些需要调用的模块,写法类似于:
+
+```javascript
+!function (allModule) {
+ function useModule(whichModule) {
+ allModule[whichModule].call(null, "hello world!");
+ }
+}([
+ function module0(param) {console.log("module0: " + param)},
+ function module1(param) {console.log("module1: " + param)},
+ function module2(param) {console.log("module2: " + param)},
+]);
+```
+
+观察这次站点的加密代码,会发现所有加密方法都在 home.min.js 里面,在此文件开头可以看到整个是一个 IIFE 立即调用函数表达式,`function e` 里面有关键方法 `.call()`,由此可以判断该函数为模块加载器,后面传递的参数是一个字典,里面是一个个的对象方法,也就是需要调用的模块函数,这就是一个典型的 webpack 写法,如下图所示:
+
+
+
+接下来我们通过 4 步完成对 webpack 代码的改写,将原始代码扒下来实现加密的过程。
+
+#### 1、找到 IIFE
+
+IIFE 立即调用函数表达式,也称为立即执行函数,自执行函数,将源码中的 IIFE 框架抠出来,后续将有用的代码再往里面放:
+
+```javascript
+!function (t) {
+
+}({
+
+})
+```
+
+#### 2、找到模块加载器
+
+前面我们已经讲过,带有 `function.call()` 或者 `function.apply()` 方法的就是模块加载器,也就是调用模块的方法,在本例中,`function e` 就是模块加载器,将其抠下来即可,其他多余的代码可以直接删除,注意里面用到了 `i`,所以定义 `i` 的语句也要抠下来:
+
+```javascript
+!function (t) {
+ function e(s) {
+ if (i[s])
+ return i[s].exports;
+ var n = i[s] = {
+ exports: {},
+ id: s,
+ loaded: !1
+ };
+ return t[s].call(n.exports, n, n.exports, e),
+ n.loaded = !0,
+ n.exports
+ }
+ var i = {};
+}({
+
+})
+```
+
+#### 3、找到调用的模块
+
+重新来到加密的地方,第一个模块是 3,`n` 里面的 `encode` 方法最终返回的就是加密后的结果,如下图所示:
+
+
+
+第二个模块是 4,可以看到模块 3 里面的 `this.jsencrypt.encrypt(i)` 方法实际上是调用的第 3340 行的方法,该方法在模块 4 里面,这里定位在模块 4 的方法,可以在浏览器开发者工具 source 页面,将鼠标光标放到该函数前面,一直往上滑动,直到模块开头,也可以使用 VS Code 等编辑器,将整个 home.min.js 代码粘贴过去,然后选择折叠所有代码,再搜索这个函数,即可快速定位在哪个模块。
+
+
+
+确定使用了 3 和 4 模块后,将这两个模块的所有代码扣下来即可,大致代码架构如下(模块 4 具体的代码太长,已删除):
+
+```javascript
+!function (t) {
+ function e(s) {
+ if (i[s])
+ return i[s].exports;
+ var n = i[s] = {
+ exports: {},
+ id: s,
+ loaded: !1
+ };
+ return t[s].call(n.exports, n, n.exports, e),
+ n.loaded = !0,
+ n.exports
+ }
+ var i = {};
+}(
+ {
+ 4: function (t, e, i) {},
+ 3: function (t, e, i) {
+ var s;
+ s = function (t, e, s) {
+ function n() {
+ "undefined" != typeof r && (this.jsencrypt = new r.JSEncrypt,
+ this.jsencrypt.setPublicKey("-----BEGIN PUBLIC KEY-----略-----END PUBLIC KEY-----"))
+ }
+
+ var r = i(4);
+ n.prototype.encode = function (t, e) {
+ var i = e ? e + "|" + t : t;
+ return encodeURIComponent(this.jsencrypt.encrypt(i))
+ },
+ s.exports = n
+ }.call(e, i, e, t),
+ !(void 0 !== s && (t.exports = s))
+ }
+ }
+)
+```
+
+这里需要我们理解一个地方,那就是模块 3 的代码里有一行 `var r = i(4);`,这里的 `i` 是 `3: function (t, e, i) {}`,传递过来的 `i`,而模块 3 又是由模块加载器调用的,即 `.call(n.exports, n, n.exports, e)` 里面的某个参数就是 `i`,观察代码,`i` 是一个函数,`.call(n.exports, n, n.exports, e)` 里面只有 `e` 是函数,所以 `var r = i(4);` 实际上就是模块加载器 `function e` 调用了模块 4,由于这里模块 4 是个对象,所以这里最好写成 `var r = i("4");`,这里是数字,所以可以成功运行,如果模块 4 名字变成 func4 或者其他名字,那么调用时就必须要加引号了。
+
+#### 4、导出加密函数
+
+目前关键的加密代码已经剥离完毕了,最后一步就是需要把加密函数导出来供我们调用了,首先定义一个全局变量,如 eFunc,然后在模块加载器后面使用语句 `eFunc = e`,把模块加载器导出来:
+
+```javascript
+var eFunc;
+
+!function (t) {
+ function e(s) {
+ if (i[s])
+ return i[s].exports;
+ var n = i[s] = {
+ exports: {},
+ id: s,
+ loaded: !1
+ };
+ return t[s].call(n.exports, n, n.exports, e),
+ n.loaded = !0,
+ n.exports
+ }
+ var i = {};
+ eFunc = e
+}(
+ {
+ 4: function (t, e, i) {},
+ 3: function (t, e, i) {}
+ }
+)
+```
+
+然后定义一个函数,传入明文密码,返回加密后的密码:
+
+```javascript
+function getEncryptedPassword(password) {
+ var timestamp = (new Date).getTime();
+ var encryptFunc = eFunc("3");
+ var encrypt = new encryptFunc;
+ return encrypt.encode(password, timestamp)
+}
+```
+
+其中 timestamp 为时间戳,因为我们最终需要调用的是模块 3 里面的 `n.prototype.encode` 这个方法,所以首先调用模块 3,返回的是模块 3 里面的 n 函数(可以在浏览器运行代码,一步一步查看结果),然后将其 new 出来,调用 n 的 encode 方法,返回加密后的结果。
+
+自此,webpack 的加密代码就剥离完毕了,最后调试会发现 navigator 和 window 未定义,定义一下即可:
+
+```javascript
+var navigator = {};
+var window = global;
+```
+
+这里扩展一下,在浏览器里面 window 其实就是 global,在 nodejs 里没有 window,但是有个 global,与浏览器的 window 对象类型相似,是全局可访问的对象,因此在 nodejs 环境中可以将 window 定义为 global,如果定义为空,可能会引起其他错误。
+
+## 完整代码
+
+GitHub 关注 K 哥爬虫,持续分享爬虫相关代码!欢迎 star !https://github.com/kgepachong/
+
+**以下只演示部分关键代码,不能直接运行!**完整代码仓库地址:https://github.com/kgepachong/crawler/
+
+### JavaScript 加密关键代码架构
+
+方法一:webpack 改写源码实现 RSA 加密:
+
+```javascript
+var navigator = {};
+var window = global;
+var eFunc;
+
+!function (t) {
+ function e(s) {
+ if (i[s])
+ return i[s].exports;
+ var n = i[s] = {
+ exports: {},
+ id: s,
+ loaded: !1
+ };
+ return t[s].call(n.exports, n, n.exports, e),
+ n.loaded = !0,
+ n.exports
+ }
+
+ var i = {};
+ eFunc = e;
+}(
+ {
+ 4: function (t, e, i) {},
+ 3: function (t, e, i) {}
+ }
+)
+
+function getEncryptedPassword(password) {
+ var timestamp = (new Date).getTime();
+ var encryptFunc = eFunc("3");
+ var encrypt = new encryptFunc;
+ return encrypt.encode(password, timestamp)
+}
+
+// 测试样例
+// console.log(getEncryptedPassword("12345678"))
+```
+
+方法二:直接使用 JSEncrypt 模块实现 RSA 加密:
+
+```javascript
+/*
+引用 jsencrypt 加密模块,此脚适合在 nodejs 环境下运行。
+1、使用 require 语句引用,前提是使用 npm 安装过;
+2、将 jsencrypt.js 直接粘贴到此脚本中使用,同时要将结尾 exports.JSEncrypt = JSEncrypt; 改为 je = JSEncrypt 导出方法。
+PS:需要定义 var navigator = {}; var window = global;,否则提示未定义。
+*/
+
+// ========================= 1、require 方式引用 =========================
+// var je = require("jsencrypt")
+
+// =================== 2、直接将 jsencrypt.js 复制过来 ===================
+/*! JSEncrypt v2.3.1 | https://npmcdn.com/jsencrypt@2.3.1/LICENSE.txt */
+var navigator = {};
+var window = global;
+
+// 这里是 jsencrypt.js 代码
+
+function getEncryptedPassword(t) {
+ var jsEncrypt = new je();
+ jsEncrypt.setPublicKey('MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDq04c6My441Gj0UFKgrqUhAUg+kQZeUeWSPlAU9fr4HBPDldAeqzx1UR92KJHuQh/zs1HOamE2dgX9z/2oXcJaqoRIA/FXysx+z2YlJkSk8XQLcQ8EBOkp//MZrixam7lCYpNOjadQBb2Ot0U/Ky+jF2p+Ie8gSZ7/u+Wnr5grywIDAQAB');
+ var e = (new Date).getTime();
+ var i = e ? e + "|" + t : t;
+ return encodeURIComponent(jsEncrypt.encrypt(i));
+}
+
+// 测试样例
+// console.log(getEncryptedPassword("12345678"));
+```
+
+### Python 登录关键代码
+
+```python
+#!/usr/bin/env python3
+# -*- coding: utf-8 -*-
+
+
+import re
+import json
+import time
+import random
+import base64
+from urllib import parse
+
+import execjs
+import requests
+from PIL import Image
+from Cryptodome.PublicKey import RSA
+from Cryptodome.Cipher import PKCS1_v1_5
+
+login_url = '脱敏处理,完整代码关注 GitHub:https://github.com/kgepachong/crawler'
+verify_image_url = '脱敏处理,完整代码关注 GitHub:https://github.com/kgepachong/crawler'
+check_code_url = '脱敏处理,完整代码关注 GitHub:https://github.com/kgepachong/crawler'
+
+headers = {
+ 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
+}
+session = requests.session()
+
+
+def get_jquery():
+ jsonp = ''
+ for _ in range(21):
+ jsonp += str(random.randint(0, 9))
+ jquery = 'jQuery' + jsonp + '_'
+ return jquery
+
+
+def get_dict_from_jquery(text):
+ result = re.findall(r'\((.*?)\)', text)[0]
+ return json.loads(result)
+
+
+def get_encrypted_password_by_javascript(password):
+ # 两个 JavaScript 脚本,两种方法均可
+ with open('gm99_encrypt.js', 'r', encoding='utf-8') as f:
+ # with open('gm99_encrypt_2.js', 'r', encoding='utf-8') as f:
+ exec_js = f.read()
+ encrypted_password = execjs.compile(exec_js).call('getEncryptedPassword', password)
+ return encrypted_password
+
+
+def get_encrypted_password_by_python(password):
+ timestamp = str(int(time.time() * 1000))
+ encrypted_object = timestamp + "|" + password
+ public_key = "脱敏处理,完整代码关注 GitHub:https://github.com/kgepachong/crawler"
+ rsa_key = RSA.import_key(base64.b64decode(public_key)) # 导入读取到的公钥
+ cipher = PKCS1_v1_5.new(rsa_key) # 生成对象
+ encrypted_password = base64.b64encode(cipher.encrypt(encrypted_object.encode(encoding="utf-8")))
+ encrypted_password = parse.quote(encrypted_password)
+ return encrypted_password
+
+
+def get_verify_code():
+ response = session.get(url=verify_image_url, headers=headers)
+ with open('code.png', 'wb') as f:
+ f.write(response.content)
+ image = Image.open('code.png')
+ image.show()
+ code = input('请输入图片验证码: ')
+ return code
+
+
+def check_code(code):
+ timestamp = str(int(time.time() * 1000))
+ params = {
+ 'callback': get_jquery() + timestamp,
+ 'ckcode': code,
+ '_': timestamp,
+ }
+ response = session.get(url=check_code_url, params=params, headers=headers)
+ result = get_dict_from_jquery(response.text)
+ if result['result'] == 1:
+ pass
+ else:
+ raise Exception('验证码输入错误!')
+
+
+def login(username, encrypted_password, code):
+ timestamp = str(int(time.time() * 1000))
+ params = {
+ 'callback': get_jquery() + timestamp,
+ 'encrypt': 1,
+ 'uname': username,
+ 'password': encrypted_password,
+ 'remember': 'checked',
+ 'ckcode': code,
+ '_': timestamp
+ }
+ response = session.get(url=login_url, params=params, headers=headers)
+ result = get_dict_from_jquery(response.text)
+ print(result)
+
+
+def main():
+ # 测试账号:15434947408,密码:iXqC@aJt8fi@VwV
+ username = input('请输入登录账号: ')
+ password = input('请输入登录密码: ')
+
+ # 获取加密后的密码,使用 Python 或者 JavaScript 实现均可
+ encrypted_password = get_encrypted_password_by_javascript(password)
+ # encrypted_password = get_encrypted_password_by_python(password)
+
+ # 获取验证码
+ code = get_verify_code()
+
+ # 校验验证码
+ check_code(code)
+
+ # 登录
+ login(username, encrypted_password, code)
+
+
+if __name__ == '__main__':
+ main()
+```
diff --git a/JSReverse/www_gm99_com/code.png b/JSReverse/www_gm99_com/code.png
new file mode 100644
index 0000000000000000000000000000000000000000..6c68e120a81569e8652356a6b4ee8c55aa4e77d7
Binary files /dev/null and b/JSReverse/www_gm99_com/code.png differ
diff --git a/JSReverse/www_gm99_com/gm99_encrypt.js b/JSReverse/www_gm99_com/gm99_encrypt.js
new file mode 100644
index 0000000000000000000000000000000000000000..999d16f7d99ffc10dd5c838b384c48e7aa87fd91
--- /dev/null
+++ b/JSReverse/www_gm99_com/gm99_encrypt.js
@@ -0,0 +1,2969 @@
+var navigator = {};
+var window = global;
+var eFunc;
+
+!function (t) {
+ function e(s) {
+ if (i[s])
+ return i[s].exports;
+ var n = i[s] = {
+ exports: {},
+ id: s,
+ loaded: !1
+ };
+ return t[s].call(n.exports, n, n.exports, e),
+ n.loaded = !0,
+ n.exports
+ }
+
+ var i = {};
+ eFunc = e;
+}(
+ {
+ 4: function (t, e, i) {
+ var s, n, r, s;
+ s = function (t, e, i) {
+ /*! JSEncrypt v2.3.1 | //npmcdn.com/jsencrypt@2.3.1/LICENSE.txt */
+ !function (t, o) {
+ r = [e],
+ n = o,
+ s = "function" == typeof n ? n.apply(e, r) : n,
+ !(void 0 !== s && (i.exports = s))
+ }(this, function (t) {
+ function e(t, e, i) {
+ null != t && ("number" == typeof t ? this.fromNumber(t, e, i) : null == e && "string" != typeof t ? this.fromString(t, 256) : this.fromString(t, e))
+ }
+
+ function i() {
+ return new e(null)
+ }
+
+ function s(t, e, i, s, n, r) {
+ for (; --r >= 0;) {
+ var o = e * this[t++] + i[s] + n;
+ n = Math.floor(o / 67108864),
+ i[s++] = 67108863 & o
+ }
+ return n
+ }
+
+ function n(t, e, i, s, n, r) {
+ for (var o = 32767 & e, a = e >> 15; --r >= 0;) {
+ var c = 32767 & this[t]
+ , l = this[t++] >> 15
+ , u = a * c + l * o;
+ c = o * c + ((32767 & u) << 15) + i[s] + (1073741823 & n),
+ n = (c >>> 30) + (u >>> 15) + a * l + (n >>> 30),
+ i[s++] = 1073741823 & c
+ }
+ return n
+ }
+
+ function r(t, e, i, s, n, r) {
+ for (var o = 16383 & e, a = e >> 14; --r >= 0;) {
+ var c = 16383 & this[t]
+ , l = this[t++] >> 14
+ , u = a * c + l * o;
+ c = o * c + ((16383 & u) << 14) + i[s] + n,
+ n = (c >> 28) + (u >> 14) + a * l,
+ i[s++] = 268435455 & c
+ }
+ return n
+ }
+
+ function o(t) {
+ return Te.charAt(t)
+ }
+
+ function a(t, e) {
+ var i = Ie[t.charCodeAt(e)];
+ return null == i ? -1 : i
+ }
+
+ function c(t) {
+ for (var e = this.t - 1; e >= 0; --e)
+ t[e] = this[e];
+ t.t = this.t,
+ t.s = this.s
+ }
+
+ function l(t) {
+ this.t = 1,
+ this.s = 0 > t ? -1 : 0,
+ t > 0 ? this[0] = t : -1 > t ? this[0] = t + this.DV : this.t = 0
+ }
+
+ function u(t) {
+ var e = i();
+ return e.fromInt(t),
+ e
+ }
+
+ function p(t, i) {
+ var s;
+ if (16 == i)
+ s = 4;
+ else if (8 == i)
+ s = 3;
+ else if (256 == i)
+ s = 8;
+ else if (2 == i)
+ s = 1;
+ else if (32 == i)
+ s = 5;
+ else {
+ if (4 != i)
+ return void this.fromRadix(t, i);
+ s = 2
+ }
+ this.t = 0,
+ this.s = 0;
+ for (var n = t.length, r = !1, o = 0; --n >= 0;) {
+ var c = 8 == s ? 255 & t[n] : a(t, n);
+ 0 > c ? "-" == t.charAt(n) && (r = !0) : (r = !1,
+ 0 == o ? this[this.t++] = c : o + s > this.DB ? (this[this.t - 1] |= (c & (1 << this.DB - o) - 1) << o,
+ this[this.t++] = c >> this.DB - o) : this[this.t - 1] |= c << o,
+ o += s,
+ o >= this.DB && (o -= this.DB))
+ }
+ 8 == s && 0 != (128 & t[0]) && (this.s = -1,
+ o > 0 && (this[this.t - 1] |= (1 << this.DB - o) - 1 << o)),
+ this.clamp(),
+ r && e.ZERO.subTo(this, this)
+ }
+
+ function d() {
+ for (var t = this.s & this.DM; this.t > 0 && this[this.t - 1] == t;)
+ --this.t
+ }
+
+ function h(t) {
+ if (this.s < 0)
+ return "-" + this.negate().toString(t);
+ var e;
+ if (16 == t)
+ e = 4;
+ else if (8 == t)
+ e = 3;
+ else if (2 == t)
+ e = 1;
+ else if (32 == t)
+ e = 5;
+ else {
+ if (4 != t)
+ return this.toRadix(t);
+ e = 2
+ }
+ var i, s = (1 << e) - 1, n = !1, r = "", a = this.t, c = this.DB - a * this.DB % e;
+ if (a-- > 0)
+ for (c < this.DB && (i = this[a] >> c) > 0 && (n = !0,
+ r = o(i)); a >= 0;)
+ e > c ? (i = (this[a] & (1 << c) - 1) << e - c,
+ i |= this[--a] >> (c += this.DB - e)) : (i = this[a] >> (c -= e) & s,
+ 0 >= c && (c += this.DB,
+ --a)),
+ i > 0 && (n = !0),
+ n && (r += o(i));
+ return n ? r : "0"
+ }
+
+ function f() {
+ var t = i();
+ return e.ZERO.subTo(this, t),
+ t
+ }
+
+ function g() {
+ return this.s < 0 ? this.negate() : this
+ }
+
+ function m(t) {
+ var e = this.s - t.s;
+ if (0 != e)
+ return e;
+ var i = this.t;
+ if (e = i - t.t,
+ 0 != e)
+ return this.s < 0 ? -e : e;
+ for (; --i >= 0;)
+ if (0 != (e = this[i] - t[i]))
+ return e;
+ return 0
+ }
+
+ function _(t) {
+ var e, i = 1;
+ return 0 != (e = t >>> 16) && (t = e,
+ i += 16),
+ 0 != (e = t >> 8) && (t = e,
+ i += 8),
+ 0 != (e = t >> 4) && (t = e,
+ i += 4),
+ 0 != (e = t >> 2) && (t = e,
+ i += 2),
+ 0 != (e = t >> 1) && (t = e,
+ i += 1),
+ i
+ }
+
+ function b() {
+ return this.t <= 0 ? 0 : this.DB * (this.t - 1) + _(this[this.t - 1] ^ this.s & this.DM)
+ }
+
+ function y(t, e) {
+ var i;
+ for (i = this.t - 1; i >= 0; --i)
+ e[i + t] = this[i];
+ for (i = t - 1; i >= 0; --i)
+ e[i] = 0;
+ e.t = this.t + t,
+ e.s = this.s
+ }
+
+ function w(t, e) {
+ for (var i = t; i < this.t; ++i)
+ e[i - t] = this[i];
+ e.t = Math.max(this.t - t, 0),
+ e.s = this.s
+ }
+
+ function k(t, e) {
+ var i, s = t % this.DB, n = this.DB - s, r = (1 << n) - 1, o = Math.floor(t / this.DB),
+ a = this.s << s & this.DM;
+ for (i = this.t - 1; i >= 0; --i)
+ e[i + o + 1] = this[i] >> n | a,
+ a = (this[i] & r) << s;
+ for (i = o - 1; i >= 0; --i)
+ e[i] = 0;
+ e[o] = a,
+ e.t = this.t + o + 1,
+ e.s = this.s,
+ e.clamp()
+ }
+
+ function x(t, e) {
+ e.s = this.s;
+ var i = Math.floor(t / this.DB);
+ if (i >= this.t)
+ return void (e.t = 0);
+ var s = t % this.DB
+ , n = this.DB - s
+ , r = (1 << s) - 1;
+ e[0] = this[i] >> s;
+ for (var o = i + 1; o < this.t; ++o)
+ e[o - i - 1] |= (this[o] & r) << n,
+ e[o - i] = this[o] >> s;
+ s > 0 && (e[this.t - i - 1] |= (this.s & r) << n),
+ e.t = this.t - i,
+ e.clamp()
+ }
+
+ function D(t, e) {
+ for (var i = 0, s = 0, n = Math.min(t.t, this.t); n > i;)
+ s += this[i] - t[i],
+ e[i++] = s & this.DM,
+ s >>= this.DB;
+ if (t.t < this.t) {
+ for (s -= t.s; i < this.t;)
+ s += this[i],
+ e[i++] = s & this.DM,
+ s >>= this.DB;
+ s += this.s
+ } else {
+ for (s += this.s; i < t.t;)
+ s -= t[i],
+ e[i++] = s & this.DM,
+ s >>= this.DB;
+ s -= t.s
+ }
+ e.s = 0 > s ? -1 : 0,
+ -1 > s ? e[i++] = this.DV + s : s > 0 && (e[i++] = s),
+ e.t = i,
+ e.clamp()
+ }
+
+ function S(t, i) {
+ var s = this.abs()
+ , n = t.abs()
+ , r = s.t;
+ for (i.t = r + n.t; --r >= 0;)
+ i[r] = 0;
+ for (r = 0; r < n.t; ++r)
+ i[r + s.t] = s.am(0, n[r], i, r, 0, s.t);
+ i.s = 0,
+ i.clamp(),
+ this.s != t.s && e.ZERO.subTo(i, i)
+ }
+
+ function C(t) {
+ for (var e = this.abs(), i = t.t = 2 * e.t; --i >= 0;)
+ t[i] = 0;
+ for (i = 0; i < e.t - 1; ++i) {
+ var s = e.am(i, e[i], t, 2 * i, 0, 1);
+ (t[i + e.t] += e.am(i + 1, 2 * e[i], t, 2 * i + 1, s, e.t - i - 1)) >= e.DV && (t[i + e.t] -= e.DV,
+ t[i + e.t + 1] = 1)
+ }
+ t.t > 0 && (t[t.t - 1] += e.am(i, e[i], t, 2 * i, 0, 1)),
+ t.s = 0,
+ t.clamp()
+ }
+
+ function T(t, s, n) {
+ var r = t.abs();
+ if (!(r.t <= 0)) {
+ var o = this.abs();
+ if (o.t < r.t)
+ return null != s && s.fromInt(0),
+ void (null != n && this.copyTo(n));
+ null == n && (n = i());
+ var a = i()
+ , c = this.s
+ , l = t.s
+ , u = this.DB - _(r[r.t - 1]);
+ u > 0 ? (r.lShiftTo(u, a),
+ o.lShiftTo(u, n)) : (r.copyTo(a),
+ o.copyTo(n));
+ var p = a.t
+ , d = a[p - 1];
+ if (0 != d) {
+ var h = d * (1 << this.F1) + (p > 1 ? a[p - 2] >> this.F2 : 0)
+ , f = this.FV / h
+ , g = (1 << this.F1) / h
+ , m = 1 << this.F2
+ , v = n.t
+ , b = v - p
+ , y = null == s ? i() : s;
+ for (a.dlShiftTo(b, y),
+ n.compareTo(y) >= 0 && (n[n.t++] = 1,
+ n.subTo(y, n)),
+ e.ONE.dlShiftTo(p, y),
+ y.subTo(a, a); a.t < p;)
+ a[a.t++] = 0;
+ for (; --b >= 0;) {
+ var w = n[--v] == d ? this.DM : Math.floor(n[v] * f + (n[v - 1] + m) * g);
+ if ((n[v] += a.am(0, w, n, b, 0, p)) < w)
+ for (a.dlShiftTo(b, y),
+ n.subTo(y, n); n[v] < --w;)
+ n.subTo(y, n)
+ }
+ null != s && (n.drShiftTo(p, s),
+ c != l && e.ZERO.subTo(s, s)),
+ n.t = p,
+ n.clamp(),
+ u > 0 && n.rShiftTo(u, n),
+ 0 > c && e.ZERO.subTo(n, n)
+ }
+ }
+ }
+
+ function I(t) {
+ var s = i();
+ return this.abs().divRemTo(t, null, s),
+ this.s < 0 && s.compareTo(e.ZERO) > 0 && t.subTo(s, s),
+ s
+ }
+
+ function $(t) {
+ this.m = t
+ }
+
+ function P(t) {
+ return t.s < 0 || t.compareTo(this.m) >= 0 ? t.mod(this.m) : t
+ }
+
+ function R(t) {
+ return t
+ }
+
+ function A(t) {
+ t.divRemTo(this.m, null, t)
+ }
+
+ function E(t, e, i) {
+ t.multiplyTo(e, i),
+ this.reduce(i)
+ }
+
+ function M(t, e) {
+ t.squareTo(e),
+ this.reduce(e)
+ }
+
+ function N() {
+ if (this.t < 1)
+ return 0;
+ var t = this[0];
+ if (0 == (1 & t))
+ return 0;
+ var e = 3 & t;
+ return e = e * (2 - (15 & t) * e) & 15,
+ e = e * (2 - (255 & t) * e) & 255,
+ e = e * (2 - ((65535 & t) * e & 65535)) & 65535,
+ e = e * (2 - t * e % this.DV) % this.DV,
+ e > 0 ? this.DV - e : -e
+ }
+
+ function O(t) {
+ this.m = t,
+ this.mp = t.invDigit(),
+ this.mpl = 32767 & this.mp,
+ this.mph = this.mp >> 15,
+ this.um = (1 << t.DB - 15) - 1,
+ this.mt2 = 2 * t.t
+ }
+
+ function B(t) {
+ var s = i();
+ return t.abs().dlShiftTo(this.m.t, s),
+ s.divRemTo(this.m, null, s),
+ t.s < 0 && s.compareTo(e.ZERO) > 0 && this.m.subTo(s, s),
+ s
+ }
+
+ function j(t) {
+ var e = i();
+ return t.copyTo(e),
+ this.reduce(e),
+ e
+ }
+
+ function L(t) {
+ for (; t.t <= this.mt2;)
+ t[t.t++] = 0;
+ for (var e = 0; e < this.m.t; ++e) {
+ var i = 32767 & t[e]
+ , s = i * this.mpl + ((i * this.mph + (t[e] >> 15) * this.mpl & this.um) << 15) & t.DM;
+ for (i = e + this.m.t,
+ t[i] += this.m.am(0, s, t, e, 0, this.m.t); t[i] >= t.DV;)
+ t[i] -= t.DV,
+ t[++i]++
+ }
+ t.clamp(),
+ t.drShiftTo(this.m.t, t),
+ t.compareTo(this.m) >= 0 && t.subTo(this.m, t)
+ }
+
+ function F(t, e) {
+ t.squareTo(e),
+ this.reduce(e)
+ }
+
+ function K(t, e, i) {
+ t.multiplyTo(e, i),
+ this.reduce(i)
+ }
+
+ function U() {
+ return 0 == (this.t > 0 ? 1 & this[0] : this.s)
+ }
+
+ function V(t, s) {
+ if (t > 4294967295 || 1 > t)
+ return e.ONE;
+ var n = i()
+ , r = i()
+ , o = s.convert(this)
+ , a = _(t) - 1;
+ for (o.copyTo(n); --a >= 0;)
+ if (s.sqrTo(n, r),
+ (t & 1 << a) > 0)
+ s.mulTo(r, o, n);
+ else {
+ var c = n;
+ n = r,
+ r = c
+ }
+ return s.revert(n)
+ }
+
+ function z(t, e) {
+ var i;
+ return i = 256 > t || e.isEven() ? new $(e) : new O(e),
+ this.exp(t, i)
+ }
+
+ function H() {
+ var t = i();
+ return this.copyTo(t),
+ t
+ }
+
+ function q() {
+ if (this.s < 0) {
+ if (1 == this.t)
+ return this[0] - this.DV;
+ if (0 == this.t)
+ return -1
+ } else {
+ if (1 == this.t)
+ return this[0];
+ if (0 == this.t)
+ return 0
+ }
+ return (this[1] & (1 << 32 - this.DB) - 1) << this.DB | this[0]
+ }
+
+ function J() {
+ return 0 == this.t ? this.s : this[0] << 24 >> 24
+ }
+
+ function G() {
+ return 0 == this.t ? this.s : this[0] << 16 >> 16
+ }
+
+ function Y(t) {
+ return Math.floor(Math.LN2 * this.DB / Math.log(t))
+ }
+
+ function W() {
+ return this.s < 0 ? -1 : this.t <= 0 || 1 == this.t && this[0] <= 0 ? 0 : 1
+ }
+
+ function Z(t) {
+ if (null == t && (t = 10),
+ 0 == this.signum() || 2 > t || t > 36)
+ return "0";
+ var e = this.chunkSize(t)
+ , s = Math.pow(t, e)
+ , n = u(s)
+ , r = i()
+ , o = i()
+ , a = "";
+ for (this.divRemTo(n, r, o); r.signum() > 0;)
+ a = (s + o.intValue()).toString(t).substr(1) + a,
+ r.divRemTo(n, r, o);
+ return o.intValue().toString(t) + a
+ }
+
+ function Q(t, i) {
+ this.fromInt(0),
+ null == i && (i = 10);
+ for (var s = this.chunkSize(i), n = Math.pow(i, s), r = !1, o = 0, c = 0, l = 0; l < t.length; ++l) {
+ var u = a(t, l);
+ 0 > u ? "-" == t.charAt(l) && 0 == this.signum() && (r = !0) : (c = i * c + u,
+ ++o >= s && (this.dMultiply(n),
+ this.dAddOffset(c, 0),
+ o = 0,
+ c = 0))
+ }
+ o > 0 && (this.dMultiply(Math.pow(i, o)),
+ this.dAddOffset(c, 0)),
+ r && e.ZERO.subTo(this, this)
+ }
+
+ function X(t, i, s) {
+ if ("number" == typeof i)
+ if (2 > t)
+ this.fromInt(1);
+ else
+ for (this.fromNumber(t, s),
+ this.testBit(t - 1) || this.bitwiseTo(e.ONE.shiftLeft(t - 1), at, this),
+ this.isEven() && this.dAddOffset(1, 0); !this.isProbablePrime(i);)
+ this.dAddOffset(2, 0),
+ this.bitLength() > t && this.subTo(e.ONE.shiftLeft(t - 1), this);
+ else {
+ var n = new Array
+ , r = 7 & t;
+ n.length = (t >> 3) + 1,
+ i.nextBytes(n),
+ r > 0 ? n[0] &= (1 << r) - 1 : n[0] = 0,
+ this.fromString(n, 256)
+ }
+ }
+
+ function tt() {
+ var t = this.t
+ , e = new Array;
+ e[0] = this.s;
+ var i, s = this.DB - t * this.DB % 8, n = 0;
+ if (t-- > 0)
+ for (s < this.DB && (i = this[t] >> s) != (this.s & this.DM) >> s && (e[n++] = i | this.s << this.DB - s); t >= 0;)
+ 8 > s ? (i = (this[t] & (1 << s) - 1) << 8 - s,
+ i |= this[--t] >> (s += this.DB - 8)) : (i = this[t] >> (s -= 8) & 255,
+ 0 >= s && (s += this.DB,
+ --t)),
+ 0 != (128 & i) && (i |= -256),
+ 0 == n && (128 & this.s) != (128 & i) && ++n,
+ (n > 0 || i != this.s) && (e[n++] = i);
+ return e
+ }
+
+ function et(t) {
+ return 0 == this.compareTo(t)
+ }
+
+ function it(t) {
+ return this.compareTo(t) < 0 ? this : t
+ }
+
+ function st(t) {
+ return this.compareTo(t) > 0 ? this : t
+ }
+
+ function nt(t, e, i) {
+ var s, n, r = Math.min(t.t, this.t);
+ for (s = 0; r > s; ++s)
+ i[s] = e(this[s], t[s]);
+ if (t.t < this.t) {
+ for (n = t.s & this.DM,
+ s = r; s < this.t; ++s)
+ i[s] = e(this[s], n);
+ i.t = this.t
+ } else {
+ for (n = this.s & this.DM,
+ s = r; s < t.t; ++s)
+ i[s] = e(n, t[s]);
+ i.t = t.t
+ }
+ i.s = e(this.s, t.s),
+ i.clamp()
+ }
+
+ function rt(t, e) {
+ return t & e
+ }
+
+ function ot(t) {
+ var e = i();
+ return this.bitwiseTo(t, rt, e),
+ e
+ }
+
+ function at(t, e) {
+ return t | e
+ }
+
+ function ct(t) {
+ var e = i();
+ return this.bitwiseTo(t, at, e),
+ e
+ }
+
+ function lt(t, e) {
+ return t ^ e
+ }
+
+ function ut(t) {
+ var e = i();
+ return this.bitwiseTo(t, lt, e),
+ e
+ }
+
+ function pt(t, e) {
+ return t & ~e
+ }
+
+ function dt(t) {
+ var e = i();
+ return this.bitwiseTo(t, pt, e),
+ e
+ }
+
+ function ht() {
+ for (var t = i(), e = 0; e < this.t; ++e)
+ t[e] = this.DM & ~this[e];
+ return t.t = this.t,
+ t.s = ~this.s,
+ t
+ }
+
+ function ft(t) {
+ var e = i();
+ return 0 > t ? this.rShiftTo(-t, e) : this.lShiftTo(t, e),
+ e
+ }
+
+ function gt(t) {
+ var e = i();
+ return 0 > t ? this.lShiftTo(-t, e) : this.rShiftTo(t, e),
+ e
+ }
+
+ function mt(t) {
+ if (0 == t)
+ return -1;
+ var e = 0;
+ return 0 == (65535 & t) && (t >>= 16,
+ e += 16),
+ 0 == (255 & t) && (t >>= 8,
+ e += 8),
+ 0 == (15 & t) && (t >>= 4,
+ e += 4),
+ 0 == (3 & t) && (t >>= 2,
+ e += 2),
+ 0 == (1 & t) && ++e,
+ e
+ }
+
+ function vt() {
+ for (var t = 0; t < this.t; ++t)
+ if (0 != this[t])
+ return t * this.DB + mt(this[t]);
+ return this.s < 0 ? this.t * this.DB : -1
+ }
+
+ function _t(t) {
+ for (var e = 0; 0 != t;)
+ t &= t - 1,
+ ++e;
+ return e
+ }
+
+ function bt() {
+ for (var t = 0, e = this.s & this.DM, i = 0; i < this.t; ++i)
+ t += _t(this[i] ^ e);
+ return t
+ }
+
+ function yt(t) {
+ var e = Math.floor(t / this.DB);
+ return e >= this.t ? 0 != this.s : 0 != (this[e] & 1 << t % this.DB)
+ }
+
+ function wt(t, i) {
+ var s = e.ONE.shiftLeft(t);
+ return this.bitwiseTo(s, i, s),
+ s
+ }
+
+ function kt(t) {
+ return this.changeBit(t, at)
+ }
+
+ function xt(t) {
+ return this.changeBit(t, pt)
+ }
+
+ function Dt(t) {
+ return this.changeBit(t, lt)
+ }
+
+ function St(t, e) {
+ for (var i = 0, s = 0, n = Math.min(t.t, this.t); n > i;)
+ s += this[i] + t[i],
+ e[i++] = s & this.DM,
+ s >>= this.DB;
+ if (t.t < this.t) {
+ for (s += t.s; i < this.t;)
+ s += this[i],
+ e[i++] = s & this.DM,
+ s >>= this.DB;
+ s += this.s
+ } else {
+ for (s += this.s; i < t.t;)
+ s += t[i],
+ e[i++] = s & this.DM,
+ s >>= this.DB;
+ s += t.s
+ }
+ e.s = 0 > s ? -1 : 0,
+ s > 0 ? e[i++] = s : -1 > s && (e[i++] = this.DV + s),
+ e.t = i,
+ e.clamp()
+ }
+
+ function Ct(t) {
+ var e = i();
+ return this.addTo(t, e),
+ e
+ }
+
+ function Tt(t) {
+ var e = i();
+ return this.subTo(t, e),
+ e
+ }
+
+ function It(t) {
+ var e = i();
+ return this.multiplyTo(t, e),
+ e
+ }
+
+ function $t() {
+ var t = i();
+ return this.squareTo(t),
+ t
+ }
+
+ function Pt(t) {
+ var e = i();
+ return this.divRemTo(t, e, null),
+ e
+ }
+
+ function Rt(t) {
+ var e = i();
+ return this.divRemTo(t, null, e),
+ e
+ }
+
+ function At(t) {
+ var e = i()
+ , s = i();
+ return this.divRemTo(t, e, s),
+ new Array(e, s)
+ }
+
+ function Et(t) {
+ this[this.t] = this.am(0, t - 1, this, 0, 0, this.t),
+ ++this.t,
+ this.clamp()
+ }
+
+ function Mt(t, e) {
+ if (0 != t) {
+ for (; this.t <= e;)
+ this[this.t++] = 0;
+ for (this[e] += t; this[e] >= this.DV;)
+ this[e] -= this.DV,
+ ++e >= this.t && (this[this.t++] = 0),
+ ++this[e]
+ }
+ }
+
+ function Nt() {
+ }
+
+ function Ot(t) {
+ return t
+ }
+
+ function Bt(t, e, i) {
+ t.multiplyTo(e, i)
+ }
+
+ function jt(t, e) {
+ t.squareTo(e)
+ }
+
+ function Lt(t) {
+ return this.exp(t, new Nt)
+ }
+
+ function Ft(t, e, i) {
+ var s = Math.min(this.t + t.t, e);
+ for (i.s = 0,
+ i.t = s; s > 0;)
+ i[--s] = 0;
+ var n;
+ for (n = i.t - this.t; n > s; ++s)
+ i[s + this.t] = this.am(0, t[s], i, s, 0, this.t);
+ for (n = Math.min(t.t, e); n > s; ++s)
+ this.am(0, t[s], i, s, 0, e - s);
+ i.clamp()
+ }
+
+ function Kt(t, e, i) {
+ --e;
+ var s = i.t = this.t + t.t - e;
+ for (i.s = 0; --s >= 0;)
+ i[s] = 0;
+ for (s = Math.max(e - this.t, 0); s < t.t; ++s)
+ i[this.t + s - e] = this.am(e - s, t[s], i, 0, 0, this.t + s - e);
+ i.clamp(),
+ i.drShiftTo(1, i)
+ }
+
+ function Ut(t) {
+ this.r2 = i(),
+ this.q3 = i(),
+ e.ONE.dlShiftTo(2 * t.t, this.r2),
+ this.mu = this.r2.divide(t),
+ this.m = t
+ }
+
+ function Vt(t) {
+ if (t.s < 0 || t.t > 2 * this.m.t)
+ return t.mod(this.m);
+ if (t.compareTo(this.m) < 0)
+ return t;
+ var e = i();
+ return t.copyTo(e),
+ this.reduce(e),
+ e
+ }
+
+ function zt(t) {
+ return t
+ }
+
+ function Ht(t) {
+ for (t.drShiftTo(this.m.t - 1, this.r2),
+ t.t > this.m.t + 1 && (t.t = this.m.t + 1,
+ t.clamp()),
+ this.mu.multiplyUpperTo(this.r2, this.m.t + 1, this.q3),
+ this.m.multiplyLowerTo(this.q3, this.m.t + 1, this.r2); t.compareTo(this.r2) < 0;)
+ t.dAddOffset(1, this.m.t + 1);
+ for (t.subTo(this.r2, t); t.compareTo(this.m) >= 0;)
+ t.subTo(this.m, t)
+ }
+
+ function qt(t, e) {
+ t.squareTo(e),
+ this.reduce(e)
+ }
+
+ function Jt(t, e, i) {
+ t.multiplyTo(e, i),
+ this.reduce(i)
+ }
+
+ function Gt(t, e) {
+ var s, n, r = t.bitLength(), o = u(1);
+ if (0 >= r)
+ return o;
+ s = 18 > r ? 1 : 48 > r ? 3 : 144 > r ? 4 : 768 > r ? 5 : 6,
+ n = 8 > r ? new $(e) : e.isEven() ? new Ut(e) : new O(e);
+ var a = new Array
+ , c = 3
+ , l = s - 1
+ , p = (1 << s) - 1;
+ if (a[1] = n.convert(this),
+ s > 1) {
+ var d = i();
+ for (n.sqrTo(a[1], d); p >= c;)
+ a[c] = i(),
+ n.mulTo(d, a[c - 2], a[c]),
+ c += 2
+ }
+ var h, f, g = t.t - 1, m = !0, v = i();
+ for (r = _(t[g]) - 1; g >= 0;) {
+ for (r >= l ? h = t[g] >> r - l & p : (h = (t[g] & (1 << r + 1) - 1) << l - r,
+ g > 0 && (h |= t[g - 1] >> this.DB + r - l)),
+ c = s; 0 == (1 & h);)
+ h >>= 1,
+ --c;
+ if ((r -= c) < 0 && (r += this.DB,
+ --g),
+ m)
+ a[h].copyTo(o),
+ m = !1;
+ else {
+ for (; c > 1;)
+ n.sqrTo(o, v),
+ n.sqrTo(v, o),
+ c -= 2;
+ c > 0 ? n.sqrTo(o, v) : (f = o,
+ o = v,
+ v = f),
+ n.mulTo(v, a[h], o)
+ }
+ for (; g >= 0 && 0 == (t[g] & 1 << r);)
+ n.sqrTo(o, v),
+ f = o,
+ o = v,
+ v = f,
+ --r < 0 && (r = this.DB - 1,
+ --g)
+ }
+ return n.revert(o)
+ }
+
+ function Yt(t) {
+ var e = this.s < 0 ? this.negate() : this.clone()
+ , i = t.s < 0 ? t.negate() : t.clone();
+ if (e.compareTo(i) < 0) {
+ var s = e;
+ e = i,
+ i = s
+ }
+ var n = e.getLowestSetBit()
+ , r = i.getLowestSetBit();
+ if (0 > r)
+ return e;
+ for (r > n && (r = n),
+ r > 0 && (e.rShiftTo(r, e),
+ i.rShiftTo(r, i)); e.signum() > 0;)
+ (n = e.getLowestSetBit()) > 0 && e.rShiftTo(n, e),
+ (n = i.getLowestSetBit()) > 0 && i.rShiftTo(n, i),
+ e.compareTo(i) >= 0 ? (e.subTo(i, e),
+ e.rShiftTo(1, e)) : (i.subTo(e, i),
+ i.rShiftTo(1, i));
+ return r > 0 && i.lShiftTo(r, i),
+ i
+ }
+
+ function Wt(t) {
+ if (0 >= t)
+ return 0;
+ var e = this.DV % t
+ , i = this.s < 0 ? t - 1 : 0;
+ if (this.t > 0)
+ if (0 == e)
+ i = this[0] % t;
+ else
+ for (var s = this.t - 1; s >= 0; --s)
+ i = (e * i + this[s]) % t;
+ return i
+ }
+
+ function Zt(t) {
+ var i = t.isEven();
+ if (this.isEven() && i || 0 == t.signum())
+ return e.ZERO;
+ for (var s = t.clone(), n = this.clone(), r = u(1), o = u(0), a = u(0), c = u(1); 0 != s.signum();) {
+ for (; s.isEven();)
+ s.rShiftTo(1, s),
+ i ? (r.isEven() && o.isEven() || (r.addTo(this, r),
+ o.subTo(t, o)),
+ r.rShiftTo(1, r)) : o.isEven() || o.subTo(t, o),
+ o.rShiftTo(1, o);
+ for (; n.isEven();)
+ n.rShiftTo(1, n),
+ i ? (a.isEven() && c.isEven() || (a.addTo(this, a),
+ c.subTo(t, c)),
+ a.rShiftTo(1, a)) : c.isEven() || c.subTo(t, c),
+ c.rShiftTo(1, c);
+ s.compareTo(n) >= 0 ? (s.subTo(n, s),
+ i && r.subTo(a, r),
+ o.subTo(c, o)) : (n.subTo(s, n),
+ i && a.subTo(r, a),
+ c.subTo(o, c))
+ }
+ return 0 != n.compareTo(e.ONE) ? e.ZERO : c.compareTo(t) >= 0 ? c.subtract(t) : c.signum() < 0 ? (c.addTo(t, c),
+ c.signum() < 0 ? c.add(t) : c) : c
+ }
+
+ function Qt(t) {
+ var e, i = this.abs();
+ if (1 == i.t && i[0] <= $e[$e.length - 1]) {
+ for (e = 0; e < $e.length; ++e)
+ if (i[0] == $e[e])
+ return !0;
+ return !1
+ }
+ if (i.isEven())
+ return !1;
+ for (e = 1; e < $e.length;) {
+ for (var s = $e[e], n = e + 1; n < $e.length && Pe > s;)
+ s *= $e[n++];
+ for (s = i.modInt(s); n > e;)
+ if (s % $e[e++] == 0)
+ return !1
+ }
+ return i.millerRabin(t)
+ }
+
+ function Xt(t) {
+ var s = this.subtract(e.ONE)
+ , n = s.getLowestSetBit();
+ if (0 >= n)
+ return !1;
+ var r = s.shiftRight(n);
+ t = t + 1 >> 1,
+ t > $e.length && (t = $e.length);
+ for (var o = i(), a = 0; t > a; ++a) {
+ o.fromInt($e[Math.floor(Math.random() * $e.length)]);
+ var c = o.modPow(r, this);
+ if (0 != c.compareTo(e.ONE) && 0 != c.compareTo(s)) {
+ for (var l = 1; l++ < n && 0 != c.compareTo(s);)
+ if (c = c.modPowInt(2, this),
+ 0 == c.compareTo(e.ONE))
+ return !1;
+ if (0 != c.compareTo(s))
+ return !1
+ }
+ }
+ return !0
+ }
+
+ function te() {
+ this.i = 0,
+ this.j = 0,
+ this.S = new Array
+ }
+
+ function ee(t) {
+ var e, i, s;
+ for (e = 0; 256 > e; ++e)
+ this.S[e] = e;
+ for (i = 0,
+ e = 0; 256 > e; ++e)
+ i = i + this.S[e] + t[e % t.length] & 255,
+ s = this.S[e],
+ this.S[e] = this.S[i],
+ this.S[i] = s;
+ this.i = 0,
+ this.j = 0
+ }
+
+ function ie() {
+ var t;
+ return this.i = this.i + 1 & 255,
+ this.j = this.j + this.S[this.i] & 255,
+ t = this.S[this.i],
+ this.S[this.i] = this.S[this.j],
+ this.S[this.j] = t,
+ this.S[t + this.S[this.i] & 255]
+ }
+
+ function se() {
+ return new te
+ }
+
+ function ne() {
+ if (null == Re) {
+ for (Re = se(); Me > Ee;) {
+ var t = Math.floor(65536 * Math.random());
+ Ae[Ee++] = 255 & t
+ }
+ for (Re.init(Ae),
+ Ee = 0; Ee < Ae.length; ++Ee)
+ Ae[Ee] = 0;
+ Ee = 0
+ }
+ return Re.next()
+ }
+
+ function re(t) {
+ var e;
+ for (e = 0; e < t.length; ++e)
+ t[e] = ne()
+ }
+
+ function oe() {
+ }
+
+ function ae(t, i) {
+ return new e(t, i)
+ }
+
+ function ce(t, i) {
+ if (i < t.length + 11)
+ return console.error("Message too long for RSA"),
+ null;
+ for (var s = new Array, n = t.length - 1; n >= 0 && i > 0;) {
+ var r = t.charCodeAt(n--);
+ 128 > r ? s[--i] = r : r > 127 && 2048 > r ? (s[--i] = 63 & r | 128,
+ s[--i] = r >> 6 | 192) : (s[--i] = 63 & r | 128,
+ s[--i] = r >> 6 & 63 | 128,
+ s[--i] = r >> 12 | 224)
+ }
+ s[--i] = 0;
+ for (var o = new oe, a = new Array; i > 2;) {
+ for (a[0] = 0; 0 == a[0];)
+ o.nextBytes(a);
+ s[--i] = a[0]
+ }
+ return s[--i] = 2,
+ s[--i] = 0,
+ new e(s)
+ }
+
+ function le() {
+ this.n = null,
+ this.e = 0,
+ this.d = null,
+ this.p = null,
+ this.q = null,
+ this.dmp1 = null,
+ this.dmq1 = null,
+ this.coeff = null
+ }
+
+ function ue(t, e) {
+ null != t && null != e && t.length > 0 && e.length > 0 ? (this.n = ae(t, 16),
+ this.e = parseInt(e, 16)) : console.error("Invalid RSA public key")
+ }
+
+ function pe(t) {
+ return t.modPowInt(this.e, this.n)
+ }
+
+ function de(t) {
+ var e = ce(t, this.n.bitLength() + 7 >> 3);
+ if (null == e)
+ return null;
+ var i = this.doPublic(e);
+ if (null == i)
+ return null;
+ var s = i.toString(16);
+ return 0 == (1 & s.length) ? s : "0" + s
+ }
+
+ function he(t, e) {
+ for (var i = t.toByteArray(), s = 0; s < i.length && 0 == i[s];)
+ ++s;
+ if (i.length - s != e - 1 || 2 != i[s])
+ return null;
+ for (++s; 0 != i[s];)
+ if (++s >= i.length)
+ return null;
+ for (var n = ""; ++s < i.length;) {
+ var r = 255 & i[s];
+ 128 > r ? n += String.fromCharCode(r) : r > 191 && 224 > r ? (n += String.fromCharCode((31 & r) << 6 | 63 & i[s + 1]),
+ ++s) : (n += String.fromCharCode((15 & r) << 12 | (63 & i[s + 1]) << 6 | 63 & i[s + 2]),
+ s += 2)
+ }
+ return n
+ }
+
+ function fe(t, e, i) {
+ null != t && null != e && t.length > 0 && e.length > 0 ? (this.n = ae(t, 16),
+ this.e = parseInt(e, 16),
+ this.d = ae(i, 16)) : console.error("Invalid RSA private key")
+ }
+
+ function ge(t, e, i, s, n, r, o, a) {
+ null != t && null != e && t.length > 0 && e.length > 0 ? (this.n = ae(t, 16),
+ this.e = parseInt(e, 16),
+ this.d = ae(i, 16),
+ this.p = ae(s, 16),
+ this.q = ae(n, 16),
+ this.dmp1 = ae(r, 16),
+ this.dmq1 = ae(o, 16),
+ this.coeff = ae(a, 16)) : console.error("Invalid RSA private key")
+ }
+
+ function me(t, i) {
+ var s = new oe
+ , n = t >> 1;
+ this.e = parseInt(i, 16);
+ for (var r = new e(i, 16); ;) {
+ for (; this.p = new e(t - n, 1, s),
+ 0 != this.p.subtract(e.ONE).gcd(r).compareTo(e.ONE) || !this.p.isProbablePrime(10);)
+ ;
+ for (; this.q = new e(n, 1, s),
+ 0 != this.q.subtract(e.ONE).gcd(r).compareTo(e.ONE) || !this.q.isProbablePrime(10);)
+ ;
+ if (this.p.compareTo(this.q) <= 0) {
+ var o = this.p;
+ this.p = this.q,
+ this.q = o
+ }
+ var a = this.p.subtract(e.ONE)
+ , c = this.q.subtract(e.ONE)
+ , l = a.multiply(c);
+ if (0 == l.gcd(r).compareTo(e.ONE)) {
+ this.n = this.p.multiply(this.q),
+ this.d = r.modInverse(l),
+ this.dmp1 = this.d.mod(a),
+ this.dmq1 = this.d.mod(c),
+ this.coeff = this.q.modInverse(this.p);
+ break
+ }
+ }
+ }
+
+ function ve(t) {
+ if (null == this.p || null == this.q)
+ return t.modPow(this.d, this.n);
+ for (var e = t.mod(this.p).modPow(this.dmp1, this.p), i = t.mod(this.q).modPow(this.dmq1, this.q); e.compareTo(i) < 0;)
+ e = e.add(this.p);
+ return e.subtract(i).multiply(this.coeff).mod(this.p).multiply(this.q).add(i)
+ }
+
+ function _e(t) {
+ var e = ae(t, 16)
+ , i = this.doPrivate(e);
+ return null == i ? null : he(i, this.n.bitLength() + 7 >> 3)
+ }
+
+ function be(t) {
+ var e, i, s = "";
+ for (e = 0; e + 3 <= t.length; e += 3)
+ i = parseInt(t.substring(e, e + 3), 16),
+ s += je.charAt(i >> 6) + je.charAt(63 & i);
+ for (e + 1 == t.length ? (i = parseInt(t.substring(e, e + 1), 16),
+ s += je.charAt(i << 2)) : e + 2 == t.length && (i = parseInt(t.substring(e, e + 2), 16),
+ s += je.charAt(i >> 2) + je.charAt((3 & i) << 4)); (3 & s.length) > 0;)
+ s += Le;
+ return s
+ }
+
+ function ye(t) {
+ var e, i, s = "", n = 0;
+ for (e = 0; e < t.length && t.charAt(e) != Le; ++e)
+ v = je.indexOf(t.charAt(e)),
+ v < 0 || (0 == n ? (s += o(v >> 2),
+ i = 3 & v,
+ n = 1) : 1 == n ? (s += o(i << 2 | v >> 4),
+ i = 15 & v,
+ n = 2) : 2 == n ? (s += o(i),
+ s += o(v >> 2),
+ i = 3 & v,
+ n = 3) : (s += o(i << 2 | v >> 4),
+ s += o(15 & v),
+ n = 0));
+ return 1 == n && (s += o(i << 2)),
+ s
+ }
+
+ var we, ke = 0xdeadbeefcafe, xe = 15715070 == (16777215 & ke);
+ xe && "Microsoft Internet Explorer" == navigator.appName ? (e.prototype.am = n,
+ we = 30) : xe && "Netscape" != navigator.appName ? (e.prototype.am = s,
+ we = 26) : (e.prototype.am = r,
+ we = 28),
+ e.prototype.DB = we,
+ e.prototype.DM = (1 << we) - 1,
+ e.prototype.DV = 1 << we;
+ var De = 52;
+ e.prototype.FV = Math.pow(2, De),
+ e.prototype.F1 = De - we,
+ e.prototype.F2 = 2 * we - De;
+ var Se, Ce, Te = "0123456789abcdefghijklmnopqrstuvwxyz", Ie = new Array;
+ for (Se = "0".charCodeAt(0),
+ Ce = 0; 9 >= Ce; ++Ce)
+ Ie[Se++] = Ce;
+ for (Se = "a".charCodeAt(0),
+ Ce = 10; 36 > Ce; ++Ce)
+ Ie[Se++] = Ce;
+ for (Se = "A".charCodeAt(0),
+ Ce = 10; 36 > Ce; ++Ce)
+ Ie[Se++] = Ce;
+ $.prototype.convert = P,
+ $.prototype.revert = R,
+ $.prototype.reduce = A,
+ $.prototype.mulTo = E,
+ $.prototype.sqrTo = M,
+ O.prototype.convert = B,
+ O.prototype.revert = j,
+ O.prototype.reduce = L,
+ O.prototype.mulTo = K,
+ O.prototype.sqrTo = F,
+ e.prototype.copyTo = c,
+ e.prototype.fromInt = l,
+ e.prototype.fromString = p,
+ e.prototype.clamp = d,
+ e.prototype.dlShiftTo = y,
+ e.prototype.drShiftTo = w,
+ e.prototype.lShiftTo = k,
+ e.prototype.rShiftTo = x,
+ e.prototype.subTo = D,
+ e.prototype.multiplyTo = S,
+ e.prototype.squareTo = C,
+ e.prototype.divRemTo = T,
+ e.prototype.invDigit = N,
+ e.prototype.isEven = U,
+ e.prototype.exp = V,
+ e.prototype.toString = h,
+ e.prototype.negate = f,
+ e.prototype.abs = g,
+ e.prototype.compareTo = m,
+ e.prototype.bitLength = b,
+ e.prototype.mod = I,
+ e.prototype.modPowInt = z,
+ e.ZERO = u(0),
+ e.ONE = u(1),
+ Nt.prototype.convert = Ot,
+ Nt.prototype.revert = Ot,
+ Nt.prototype.mulTo = Bt,
+ Nt.prototype.sqrTo = jt,
+ Ut.prototype.convert = Vt,
+ Ut.prototype.revert = zt,
+ Ut.prototype.reduce = Ht,
+ Ut.prototype.mulTo = Jt,
+ Ut.prototype.sqrTo = qt;
+ var $e = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541, 547, 557, 563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619, 631, 641, 643, 647, 653, 659, 661, 673, 677, 683, 691, 701, 709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787, 797, 809, 811, 821, 823, 827, 829, 839, 853, 857, 859, 863, 877, 881, 883, 887, 907, 911, 919, 929, 937, 941, 947, 953, 967, 971, 977, 983, 991, 997]
+ , Pe = (1 << 26) / $e[$e.length - 1];
+ e.prototype.chunkSize = Y,
+ e.prototype.toRadix = Z,
+ e.prototype.fromRadix = Q,
+ e.prototype.fromNumber = X,
+ e.prototype.bitwiseTo = nt,
+ e.prototype.changeBit = wt,
+ e.prototype.addTo = St,
+ e.prototype.dMultiply = Et,
+ e.prototype.dAddOffset = Mt,
+ e.prototype.multiplyLowerTo = Ft,
+ e.prototype.multiplyUpperTo = Kt,
+ e.prototype.modInt = Wt,
+ e.prototype.millerRabin = Xt,
+ e.prototype.clone = H,
+ e.prototype.intValue = q,
+ e.prototype.byteValue = J,
+ e.prototype.shortValue = G,
+ e.prototype.signum = W,
+ e.prototype.toByteArray = tt,
+ e.prototype.equals = et,
+ e.prototype.min = it,
+ e.prototype.max = st,
+ e.prototype.and = ot,
+ e.prototype.or = ct,
+ e.prototype.xor = ut,
+ e.prototype.andNot = dt,
+ e.prototype.not = ht,
+ e.prototype.shiftLeft = ft,
+ e.prototype.shiftRight = gt,
+ e.prototype.getLowestSetBit = vt,
+ e.prototype.bitCount = bt,
+ e.prototype.testBit = yt,
+ e.prototype.setBit = kt,
+ e.prototype.clearBit = xt,
+ e.prototype.flipBit = Dt,
+ e.prototype.add = Ct,
+ e.prototype.subtract = Tt,
+ e.prototype.multiply = It,
+ e.prototype.divide = Pt,
+ e.prototype.remainder = Rt,
+ e.prototype.divideAndRemainder = At,
+ e.prototype.modPow = Gt,
+ e.prototype.modInverse = Zt,
+ e.prototype.pow = Lt,
+ e.prototype.gcd = Yt,
+ e.prototype.isProbablePrime = Qt,
+ e.prototype.square = $t,
+ te.prototype.init = ee,
+ te.prototype.next = ie;
+ var Re, Ae, Ee, Me = 256;
+ if (null == Ae) {
+ Ae = new Array,
+ Ee = 0;
+ var Ne;
+ if (window.crypto && window.crypto.getRandomValues) {
+ var Oe = new Uint32Array(256);
+ for (window.crypto.getRandomValues(Oe),
+ Ne = 0; Ne < Oe.length; ++Ne)
+ Ae[Ee++] = 255 & Oe[Ne]
+ }
+ var Be = function (t) {
+ if (this.count = this.count || 0,
+ this.count >= 256 || Ee >= Me)
+ return void (window.removeEventListener ? window.removeEventListener("mousemove", Be, !1) : window.detachEvent && window.detachEvent("onmousemove", Be));
+ try {
+ var e = t.x + t.y;
+ Ae[Ee++] = 255 & e,
+ this.count += 1
+ } catch (t) {
+ }
+ };
+ window.addEventListener ? window.addEventListener("mousemove", Be, !1) : window.attachEvent && window.attachEvent("onmousemove", Be)
+ }
+ oe.prototype.nextBytes = re,
+ le.prototype.doPublic = pe,
+ le.prototype.setPublic = ue,
+ le.prototype.encrypt = de,
+ le.prototype.doPrivate = ve,
+ le.prototype.setPrivate = fe,
+ le.prototype.setPrivateEx = ge,
+ le.prototype.generate = me,
+ le.prototype.decrypt = _e,
+ function () {
+ var t = function (t, s, n) {
+ var r = new oe
+ , o = t >> 1;
+ this.e = parseInt(s, 16);
+ var a = new e(s, 16)
+ , c = this
+ , l = function () {
+ var s = function () {
+ if (c.p.compareTo(c.q) <= 0) {
+ var t = c.p;
+ c.p = c.q,
+ c.q = t
+ }
+ var i = c.p.subtract(e.ONE)
+ , s = c.q.subtract(e.ONE)
+ , r = i.multiply(s);
+ 0 == r.gcd(a).compareTo(e.ONE) ? (c.n = c.p.multiply(c.q),
+ c.d = a.modInverse(r),
+ c.dmp1 = c.d.mod(i),
+ c.dmq1 = c.d.mod(s),
+ c.coeff = c.q.modInverse(c.p),
+ setTimeout(function () {
+ n()
+ }, 0)) : setTimeout(l, 0)
+ }
+ , u = function () {
+ c.q = i(),
+ c.q.fromNumberAsync(o, 1, r, function () {
+ c.q.subtract(e.ONE).gcda(a, function (t) {
+ 0 == t.compareTo(e.ONE) && c.q.isProbablePrime(10) ? setTimeout(s, 0) : setTimeout(u, 0)
+ })
+ })
+ }
+ , p = function () {
+ c.p = i(),
+ c.p.fromNumberAsync(t - o, 1, r, function () {
+ c.p.subtract(e.ONE).gcda(a, function (t) {
+ 0 == t.compareTo(e.ONE) && c.p.isProbablePrime(10) ? setTimeout(u, 0) : setTimeout(p, 0)
+ })
+ })
+ };
+ setTimeout(p, 0)
+ };
+ setTimeout(l, 0)
+ };
+ le.prototype.generateAsync = t;
+ var s = function (t, e) {
+ var i = this.s < 0 ? this.negate() : this.clone()
+ , s = t.s < 0 ? t.negate() : t.clone();
+ if (i.compareTo(s) < 0) {
+ var n = i;
+ i = s,
+ s = n
+ }
+ var r = i.getLowestSetBit()
+ , o = s.getLowestSetBit();
+ if (0 > o)
+ return void e(i);
+ o > r && (o = r),
+ o > 0 && (i.rShiftTo(o, i),
+ s.rShiftTo(o, s));
+ var a = function () {
+ (r = i.getLowestSetBit()) > 0 && i.rShiftTo(r, i),
+ (r = s.getLowestSetBit()) > 0 && s.rShiftTo(r, s),
+ i.compareTo(s) >= 0 ? (i.subTo(s, i),
+ i.rShiftTo(1, i)) : (s.subTo(i, s),
+ s.rShiftTo(1, s)),
+ i.signum() > 0 ? setTimeout(a, 0) : (o > 0 && s.lShiftTo(o, s),
+ setTimeout(function () {
+ e(s)
+ }, 0))
+ };
+ setTimeout(a, 10)
+ };
+ e.prototype.gcda = s;
+ var n = function (t, i, s, n) {
+ if ("number" == typeof i)
+ if (2 > t)
+ this.fromInt(1);
+ else {
+ this.fromNumber(t, s),
+ this.testBit(t - 1) || this.bitwiseTo(e.ONE.shiftLeft(t - 1), at, this),
+ this.isEven() && this.dAddOffset(1, 0);
+ var r = this
+ , o = function () {
+ r.dAddOffset(2, 0),
+ r.bitLength() > t && r.subTo(e.ONE.shiftLeft(t - 1), r),
+ r.isProbablePrime(i) ? setTimeout(function () {
+ n()
+ }, 0) : setTimeout(o, 0)
+ };
+ setTimeout(o, 0)
+ }
+ else {
+ var a = new Array
+ , c = 7 & t;
+ a.length = (t >> 3) + 1,
+ i.nextBytes(a),
+ c > 0 ? a[0] &= (1 << c) - 1 : a[0] = 0,
+ this.fromString(a, 256)
+ }
+ };
+ e.prototype.fromNumberAsync = n
+ }();
+ var je = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"
+ , Le = "="
+ , Fe = Fe || {};
+ Fe.env = Fe.env || {};
+ var Ke = Fe
+ , Ue = Object.prototype
+ , Ve = "[object Function]"
+ , ze = ["toString", "valueOf"];
+ Fe.env.parseUA = function (t) {
+ var e, i = function (t) {
+ var e = 0;
+ return parseFloat(t.replace(/\./g, function () {
+ return 1 == e++ ? "" : "."
+ }))
+ }, s = navigator, n = {
+ ie: 0,
+ opera: 0,
+ gecko: 0,
+ webkit: 0,
+ chrome: 0,
+ mobile: null,
+ air: 0,
+ ipad: 0,
+ iphone: 0,
+ ipod: 0,
+ ios: null,
+ android: 0,
+ webos: 0,
+ caja: s && s.cajaVersion,
+ secure: !1,
+ os: null
+ }, r = t || navigator && navigator.userAgent, o = window && window.location, a = o && o.href;
+ return n.secure = a && 0 === a.toLowerCase().indexOf("https"),
+ r && (/windows|win32/i.test(r) ? n.os = "windows" : /macintosh/i.test(r) ? n.os = "macintosh" : /rhino/i.test(r) && (n.os = "rhino"),
+ /KHTML/.test(r) && (n.webkit = 1),
+ e = r.match(/AppleWebKit\/([^\s]*)/),
+ e && e[1] && (n.webkit = i(e[1]),
+ / Mobile\//.test(r) ? (n.mobile = "Apple",
+ e = r.match(/OS ([^\s]*)/),
+ e && e[1] && (e = i(e[1].replace("_", "."))),
+ n.ios = e,
+ n.ipad = n.ipod = n.iphone = 0,
+ e = r.match(/iPad|iPod|iPhone/),
+ e && e[0] && (n[e[0].toLowerCase()] = n.ios)) : (e = r.match(/NokiaN[^\/]*|Android \d\.\d|webOS\/\d\.\d/),
+ e && (n.mobile = e[0]),
+ /webOS/.test(r) && (n.mobile = "WebOS",
+ e = r.match(/webOS\/([^\s]*);/),
+ e && e[1] && (n.webos = i(e[1]))),
+ / Android/.test(r) && (n.mobile = "Android",
+ e = r.match(/Android ([^\s]*);/),
+ e && e[1] && (n.android = i(e[1])))),
+ e = r.match(/Chrome\/([^\s]*)/),
+ e && e[1] ? n.chrome = i(e[1]) : (e = r.match(/AdobeAIR\/([^\s]*)/),
+ e && (n.air = e[0]))),
+ n.webkit || (e = r.match(/Opera[\s\/]([^\s]*)/),
+ e && e[1] ? (n.opera = i(e[1]),
+ e = r.match(/Version\/([^\s]*)/),
+ e && e[1] && (n.opera = i(e[1])),
+ e = r.match(/Opera Mini[^;]*/),
+ e && (n.mobile = e[0])) : (e = r.match(/MSIE\s([^;]*)/),
+ e && e[1] ? n.ie = i(e[1]) : (e = r.match(/Gecko\/([^\s]*)/),
+ e && (n.gecko = 1,
+ e = r.match(/rv:([^\s\)]*)/),
+ e && e[1] && (n.gecko = i(e[1]))))))),
+ n
+ }
+ ,
+ Fe.env.ua = Fe.env.parseUA(),
+ Fe.isFunction = function (t) {
+ return "function" == typeof t || Ue.toString.apply(t) === Ve
+ }
+ ,
+ Fe._IEEnumFix = Fe.env.ua.ie ? function (t, e) {
+ var i, s, n;
+ for (i = 0; i < ze.length; i += 1)
+ s = ze[i],
+ n = e[s],
+ Ke.isFunction(n) && n != Ue[s] && (t[s] = n)
+ }
+ : function () {
+ }
+ ,
+ Fe.extend = function (t, e, i) {
+ if (!e || !t)
+ throw new Error("extend failed, please check that all dependencies are included.");
+ var s, n = function () {
+ };
+ if (n.prototype = e.prototype,
+ t.prototype = new n,
+ t.prototype.constructor = t,
+ t.superclass = e.prototype,
+ e.prototype.constructor == Ue.constructor && (e.prototype.constructor = e),
+ i) {
+ for (s in i)
+ Ke.hasOwnProperty(i, s) && (t.prototype[s] = i[s]);
+ Ke._IEEnumFix(t.prototype, i)
+ }
+ }
+ ,
+ /**
+ * @fileOverview
+ * @name asn1-1.0.js
+ * @author Kenji Urushima kenji.urushima@gmail.com
+ * @version 1.0.2 (2013-May-30)
+ * @since 2.1
+ * @license MIT License
+ */
+ "undefined" != typeof KJUR && KJUR || (KJUR = {}),
+ "undefined" != typeof KJUR.asn1 && KJUR.asn1 || (KJUR.asn1 = {}),
+ KJUR.asn1.ASN1Util = new function () {
+ this.integerToByteHex = function (t) {
+ var e = t.toString(16);
+ return e.length % 2 == 1 && (e = "0" + e),
+ e
+ }
+ ,
+ this.bigIntToMinTwosComplementsHex = function (t) {
+ var i = t.toString(16);
+ if ("-" != i.substr(0, 1))
+ i.length % 2 == 1 ? i = "0" + i : i.match(/^[0-7]/) || (i = "00" + i);
+ else {
+ var s = i.substr(1)
+ , n = s.length;
+ n % 2 == 1 ? n += 1 : i.match(/^[0-7]/) || (n += 2);
+ for (var r = "", o = 0; n > o; o++)
+ r += "f";
+ var a = new e(r, 16)
+ , c = a.xor(t).add(e.ONE);
+ i = c.toString(16).replace(/^-/, "")
+ }
+ return i
+ }
+ ,
+ this.getPEMStringFromHex = function (t, e) {
+ var i = CryptoJS.enc.Hex.parse(t)
+ , s = CryptoJS.enc.Base64.stringify(i)
+ , n = s.replace(/(.{64})/g, "$1\r\n");
+ return n = n.replace(/\r\n$/, ""),
+ "-----BEGIN " + e + "-----\r\n" + n + "\r\n-----END " + e + "-----\r\n"
+ }
+ }
+ ,
+ KJUR.asn1.ASN1Object = function () {
+ var t = "";
+ this.getLengthHexFromValue = function () {
+ if ("undefined" == typeof this.hV || null == this.hV)
+ throw "this.hV is null or undefined.";
+ if (this.hV.length % 2 == 1)
+ throw "value hex must be even length: n=" + t.length + ",v=" + this.hV;
+ var e = this.hV.length / 2
+ , i = e.toString(16);
+ if (i.length % 2 == 1 && (i = "0" + i),
+ 128 > e)
+ return i;
+ var s = i.length / 2;
+ if (s > 15)
+ throw "ASN.1 length too long to represent by 8x: n = " + e.toString(16);
+ var n = 128 + s;
+ return n.toString(16) + i
+ }
+ ,
+ this.getEncodedHex = function () {
+ return (null == this.hTLV || this.isModified) && (this.hV = this.getFreshValueHex(),
+ this.hL = this.getLengthHexFromValue(),
+ this.hTLV = this.hT + this.hL + this.hV,
+ this.isModified = !1),
+ this.hTLV
+ }
+ ,
+ this.getValueHex = function () {
+ return this.getEncodedHex(),
+ this.hV
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return ""
+ }
+ }
+ ,
+ KJUR.asn1.DERAbstractString = function (t) {
+ KJUR.asn1.DERAbstractString.superclass.constructor.call(this),
+ this.getString = function () {
+ return this.s
+ }
+ ,
+ this.setString = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.s = t,
+ this.hV = stohex(this.s)
+ }
+ ,
+ this.setStringHex = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.s = null,
+ this.hV = t
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return this.hV
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.str ? this.setString(t.str) : "undefined" != typeof t.hex && this.setStringHex(t.hex))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERAbstractString, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERAbstractTime = function (t) {
+ KJUR.asn1.DERAbstractTime.superclass.constructor.call(this),
+ this.localDateToUTC = function (t) {
+ utc = t.getTime() + 6e4 * t.getTimezoneOffset();
+ var e = new Date(utc);
+ return e
+ }
+ ,
+ this.formatDate = function (t, e) {
+ var i = this.zeroPadding
+ , s = this.localDateToUTC(t)
+ , n = String(s.getFullYear());
+ "utc" == e && (n = n.substr(2, 2));
+ var r = i(String(s.getMonth() + 1), 2)
+ , o = i(String(s.getDate()), 2)
+ , a = i(String(s.getHours()), 2)
+ , c = i(String(s.getMinutes()), 2)
+ , l = i(String(s.getSeconds()), 2);
+ return n + r + o + a + c + l + "Z"
+ }
+ ,
+ this.zeroPadding = function (t, e) {
+ return t.length >= e ? t : new Array(e - t.length + 1).join("0") + t
+ }
+ ,
+ this.getString = function () {
+ return this.s
+ }
+ ,
+ this.setString = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.s = t,
+ this.hV = stohex(this.s)
+ }
+ ,
+ this.setByDateValue = function (t, e, i, s, n, r) {
+ var o = new Date(Date.UTC(t, e - 1, i, s, n, r, 0));
+ this.setByDate(o)
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return this.hV
+ }
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERAbstractTime, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERAbstractStructured = function (t) {
+ KJUR.asn1.DERAbstractString.superclass.constructor.call(this),
+ this.setByASN1ObjectArray = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.asn1Array = t
+ }
+ ,
+ this.appendASN1Object = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.asn1Array.push(t)
+ }
+ ,
+ this.asn1Array = new Array,
+ "undefined" != typeof t && "undefined" != typeof t.array && (this.asn1Array = t.array)
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERAbstractStructured, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERBoolean = function () {
+ KJUR.asn1.DERBoolean.superclass.constructor.call(this),
+ this.hT = "01",
+ this.hTLV = "0101ff"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERBoolean, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERInteger = function (t) {
+ KJUR.asn1.DERInteger.superclass.constructor.call(this),
+ this.hT = "02",
+ this.setByBigInteger = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.hV = KJUR.asn1.ASN1Util.bigIntToMinTwosComplementsHex(t)
+ }
+ ,
+ this.setByInteger = function (t) {
+ var i = new e(String(t), 10);
+ this.setByBigInteger(i)
+ }
+ ,
+ this.setValueHex = function (t) {
+ this.hV = t
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return this.hV
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.bigint ? this.setByBigInteger(t.bigint) : "undefined" != typeof t.int ? this.setByInteger(t.int) : "undefined" != typeof t.hex && this.setValueHex(t.hex))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERInteger, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERBitString = function (t) {
+ KJUR.asn1.DERBitString.superclass.constructor.call(this),
+ this.hT = "03",
+ this.setHexValueIncludingUnusedBits = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.hV = t
+ }
+ ,
+ this.setUnusedBitsAndHexValue = function (t, e) {
+ if (0 > t || t > 7)
+ throw "unused bits shall be from 0 to 7: u = " + t;
+ var i = "0" + t;
+ this.hTLV = null,
+ this.isModified = !0,
+ this.hV = i + e
+ }
+ ,
+ this.setByBinaryString = function (t) {
+ t = t.replace(/0+$/, "");
+ var e = 8 - t.length % 8;
+ 8 == e && (e = 0);
+ for (var i = 0; e >= i; i++)
+ t += "0";
+ for (var s = "", i = 0; i < t.length - 1; i += 8) {
+ var n = t.substr(i, 8)
+ , r = parseInt(n, 2).toString(16);
+ 1 == r.length && (r = "0" + r),
+ s += r
+ }
+ this.hTLV = null,
+ this.isModified = !0,
+ this.hV = "0" + e + s
+ }
+ ,
+ this.setByBooleanArray = function (t) {
+ for (var e = "", i = 0; i < t.length; i++)
+ e += 1 == t[i] ? "1" : "0";
+ this.setByBinaryString(e)
+ }
+ ,
+ this.newFalseArray = function (t) {
+ for (var e = new Array(t), i = 0; t > i; i++)
+ e[i] = !1;
+ return e
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return this.hV
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.hex ? this.setHexValueIncludingUnusedBits(t.hex) : "undefined" != typeof t.bin ? this.setByBinaryString(t.bin) : "undefined" != typeof t.array && this.setByBooleanArray(t.array))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERBitString, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DEROctetString = function (t) {
+ KJUR.asn1.DEROctetString.superclass.constructor.call(this, t),
+ this.hT = "04"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DEROctetString, KJUR.asn1.DERAbstractString),
+ KJUR.asn1.DERNull = function () {
+ KJUR.asn1.DERNull.superclass.constructor.call(this),
+ this.hT = "05",
+ this.hTLV = "0500"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERNull, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERObjectIdentifier = function (t) {
+ var i = function (t) {
+ var e = t.toString(16);
+ return 1 == e.length && (e = "0" + e),
+ e
+ }
+ , s = function (t) {
+ var s = ""
+ , n = new e(t, 10)
+ , r = n.toString(2)
+ , o = 7 - r.length % 7;
+ 7 == o && (o = 0);
+ for (var a = "", c = 0; o > c; c++)
+ a += "0";
+ r = a + r;
+ for (var c = 0; c < r.length - 1; c += 7) {
+ var l = r.substr(c, 7);
+ c != r.length - 7 && (l = "1" + l),
+ s += i(parseInt(l, 2))
+ }
+ return s
+ };
+ KJUR.asn1.DERObjectIdentifier.superclass.constructor.call(this),
+ this.hT = "06",
+ this.setValueHex = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.s = null,
+ this.hV = t
+ }
+ ,
+ this.setValueOidString = function (t) {
+ if (!t.match(/^[0-9.]+$/))
+ throw "malformed oid string: " + t;
+ var e = ""
+ , n = t.split(".")
+ , r = 40 * parseInt(n[0]) + parseInt(n[1]);
+ e += i(r),
+ n.splice(0, 2);
+ for (var o = 0; o < n.length; o++)
+ e += s(n[o]);
+ this.hTLV = null,
+ this.isModified = !0,
+ this.s = null,
+ this.hV = e
+ }
+ ,
+ this.setValueName = function (t) {
+ if ("undefined" == typeof KJUR.asn1.x509.OID.name2oidList[t])
+ throw "DERObjectIdentifier oidName undefined: " + t;
+ var e = KJUR.asn1.x509.OID.name2oidList[t];
+ this.setValueOidString(e)
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return this.hV
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.oid ? this.setValueOidString(t.oid) : "undefined" != typeof t.hex ? this.setValueHex(t.hex) : "undefined" != typeof t.name && this.setValueName(t.name))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERObjectIdentifier, KJUR.asn1.ASN1Object),
+ KJUR.asn1.DERUTF8String = function (t) {
+ KJUR.asn1.DERUTF8String.superclass.constructor.call(this, t),
+ this.hT = "0c"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERUTF8String, KJUR.asn1.DERAbstractString),
+ KJUR.asn1.DERNumericString = function (t) {
+ KJUR.asn1.DERNumericString.superclass.constructor.call(this, t),
+ this.hT = "12"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERNumericString, KJUR.asn1.DERAbstractString),
+ KJUR.asn1.DERPrintableString = function (t) {
+ KJUR.asn1.DERPrintableString.superclass.constructor.call(this, t),
+ this.hT = "13"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERPrintableString, KJUR.asn1.DERAbstractString),
+ KJUR.asn1.DERTeletexString = function (t) {
+ KJUR.asn1.DERTeletexString.superclass.constructor.call(this, t),
+ this.hT = "14"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERTeletexString, KJUR.asn1.DERAbstractString),
+ KJUR.asn1.DERIA5String = function (t) {
+ KJUR.asn1.DERIA5String.superclass.constructor.call(this, t),
+ this.hT = "16"
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERIA5String, KJUR.asn1.DERAbstractString),
+ KJUR.asn1.DERUTCTime = function (t) {
+ KJUR.asn1.DERUTCTime.superclass.constructor.call(this, t),
+ this.hT = "17",
+ this.setByDate = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.date = t,
+ this.s = this.formatDate(this.date, "utc"),
+ this.hV = stohex(this.s)
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.str ? this.setString(t.str) : "undefined" != typeof t.hex ? this.setStringHex(t.hex) : "undefined" != typeof t.date && this.setByDate(t.date))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERUTCTime, KJUR.asn1.DERAbstractTime),
+ KJUR.asn1.DERGeneralizedTime = function (t) {
+ KJUR.asn1.DERGeneralizedTime.superclass.constructor.call(this, t),
+ this.hT = "18",
+ this.setByDate = function (t) {
+ this.hTLV = null,
+ this.isModified = !0,
+ this.date = t,
+ this.s = this.formatDate(this.date, "gen"),
+ this.hV = stohex(this.s)
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.str ? this.setString(t.str) : "undefined" != typeof t.hex ? this.setStringHex(t.hex) : "undefined" != typeof t.date && this.setByDate(t.date))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERGeneralizedTime, KJUR.asn1.DERAbstractTime),
+ KJUR.asn1.DERSequence = function (t) {
+ KJUR.asn1.DERSequence.superclass.constructor.call(this, t),
+ this.hT = "30",
+ this.getFreshValueHex = function () {
+ for (var t = "", e = 0; e < this.asn1Array.length; e++) {
+ var i = this.asn1Array[e];
+ t += i.getEncodedHex()
+ }
+ return this.hV = t,
+ this.hV
+ }
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERSequence, KJUR.asn1.DERAbstractStructured),
+ KJUR.asn1.DERSet = function (t) {
+ KJUR.asn1.DERSet.superclass.constructor.call(this, t),
+ this.hT = "31",
+ this.getFreshValueHex = function () {
+ for (var t = new Array, e = 0; e < this.asn1Array.length; e++) {
+ var i = this.asn1Array[e];
+ t.push(i.getEncodedHex())
+ }
+ return t.sort(),
+ this.hV = t.join(""),
+ this.hV
+ }
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERSet, KJUR.asn1.DERAbstractStructured),
+ KJUR.asn1.DERTaggedObject = function (t) {
+ KJUR.asn1.DERTaggedObject.superclass.constructor.call(this),
+ this.hT = "a0",
+ this.hV = "",
+ this.isExplicit = !0,
+ this.asn1Object = null,
+ this.setASN1Object = function (t, e, i) {
+ this.hT = e,
+ this.isExplicit = t,
+ this.asn1Object = i,
+ this.isExplicit ? (this.hV = this.asn1Object.getEncodedHex(),
+ this.hTLV = null,
+ this.isModified = !0) : (this.hV = null,
+ this.hTLV = i.getEncodedHex(),
+ this.hTLV = this.hTLV.replace(/^../, e),
+ this.isModified = !1)
+ }
+ ,
+ this.getFreshValueHex = function () {
+ return this.hV
+ }
+ ,
+ "undefined" != typeof t && ("undefined" != typeof t.tag && (this.hT = t.tag),
+ "undefined" != typeof t.explicit && (this.isExplicit = t.explicit),
+ "undefined" != typeof t.obj && (this.asn1Object = t.obj,
+ this.setASN1Object(this.isExplicit, this.hT, this.asn1Object)))
+ }
+ ,
+ Fe.extend(KJUR.asn1.DERTaggedObject, KJUR.asn1.ASN1Object),
+ function (t) {
+ "use strict";
+ var e, i = {};
+ i.decode = function (i) {
+ var s;
+ if (e === t) {
+ var n = "0123456789ABCDEF"
+ , r = " \f\n\r \u2028\u2029";
+ for (e = [],
+ s = 0; 16 > s; ++s)
+ e[n.charAt(s)] = s;
+ for (n = n.toLowerCase(),
+ s = 10; 16 > s; ++s)
+ e[n.charAt(s)] = s;
+ for (s = 0; s < r.length; ++s)
+ e[r.charAt(s)] = -1
+ }
+ var o = []
+ , a = 0
+ , c = 0;
+ for (s = 0; s < i.length; ++s) {
+ var l = i.charAt(s);
+ if ("=" == l)
+ break;
+ if (l = e[l],
+ -1 != l) {
+ if (l === t)
+ throw "Illegal character at offset " + s;
+ a |= l,
+ ++c >= 2 ? (o[o.length] = a,
+ a = 0,
+ c = 0) : a <<= 4
+ }
+ }
+ if (c)
+ throw "Hex encoding incomplete: 4 bits missing";
+ return o
+ }
+ ,
+ window.Hex = i
+ }(),
+ function (t) {
+ "use strict";
+ var e, i = {};
+ i.decode = function (i) {
+ var s;
+ if (e === t) {
+ var n = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"
+ , r = "= \f\n\r \u2028\u2029";
+ for (e = [],
+ s = 0; 64 > s; ++s)
+ e[n.charAt(s)] = s;
+ for (s = 0; s < r.length; ++s)
+ e[r.charAt(s)] = -1
+ }
+ var o = []
+ , a = 0
+ , c = 0;
+ for (s = 0; s < i.length; ++s) {
+ var l = i.charAt(s);
+ if ("=" == l)
+ break;
+ if (l = e[l],
+ -1 != l) {
+ if (l === t)
+ throw "Illegal character at offset " + s;
+ a |= l,
+ ++c >= 4 ? (o[o.length] = a >> 16,
+ o[o.length] = a >> 8 & 255,
+ o[o.length] = 255 & a,
+ a = 0,
+ c = 0) : a <<= 6
+ }
+ }
+ switch (c) {
+ case 1:
+ throw "Base64 encoding incomplete: at least 2 bits missing";
+ case 2:
+ o[o.length] = a >> 10;
+ break;
+ case 3:
+ o[o.length] = a >> 16,
+ o[o.length] = a >> 8 & 255
+ }
+ return o
+ }
+ ,
+ i.re = /-----BEGIN [^-]+-----([A-Za-z0-9+\/=\s]+)-----END [^-]+-----|begin-base64[^\n]+\n([A-Za-z0-9+\/=\s]+)====/,
+ i.unarmor = function (t) {
+ var e = i.re.exec(t);
+ if (e)
+ if (e[1])
+ t = e[1];
+ else {
+ if (!e[2])
+ throw "RegExp out of sync";
+ t = e[2]
+ }
+ return i.decode(t)
+ }
+ ,
+ window.Base64 = i
+ }(),
+ function (t) {
+ "use strict";
+
+ function e(t, i) {
+ t instanceof e ? (this.enc = t.enc,
+ this.pos = t.pos) : (this.enc = t,
+ this.pos = i)
+ }
+
+ function i(t, e, i, s, n) {
+ this.stream = t,
+ this.header = e,
+ this.length = i,
+ this.tag = s,
+ this.sub = n
+ }
+
+ var s = 100
+ , n = "…"
+ , r = {
+ tag: function (t, e) {
+ var i = document.createElement(t);
+ return i.className = e,
+ i
+ },
+ text: function (t) {
+ return document.createTextNode(t)
+ }
+ };
+ e.prototype.get = function (e) {
+ if (e === t && (e = this.pos++),
+ e >= this.enc.length)
+ throw "Requesting byte offset " + e + " on a stream of length " + this.enc.length;
+ return this.enc[e]
+ }
+ ,
+ e.prototype.hexDigits = "0123456789ABCDEF",
+ e.prototype.hexByte = function (t) {
+ return this.hexDigits.charAt(t >> 4 & 15) + this.hexDigits.charAt(15 & t)
+ }
+ ,
+ e.prototype.hexDump = function (t, e, i) {
+ for (var s = "", n = t; e > n; ++n)
+ if (s += this.hexByte(this.get(n)),
+ i !== !0)
+ switch (15 & n) {
+ case 7:
+ s += " ";
+ break;
+ case 15:
+ s += "\n";
+ break;
+ default:
+ s += " "
+ }
+ return s
+ }
+ ,
+ e.prototype.parseStringISO = function (t, e) {
+ for (var i = "", s = t; e > s; ++s)
+ i += String.fromCharCode(this.get(s));
+ return i
+ }
+ ,
+ e.prototype.parseStringUTF = function (t, e) {
+ for (var i = "", s = t; e > s;) {
+ var n = this.get(s++);
+ i += 128 > n ? String.fromCharCode(n) : n > 191 && 224 > n ? String.fromCharCode((31 & n) << 6 | 63 & this.get(s++)) : String.fromCharCode((15 & n) << 12 | (63 & this.get(s++)) << 6 | 63 & this.get(s++))
+ }
+ return i
+ }
+ ,
+ e.prototype.parseStringBMP = function (t, e) {
+ for (var i = "", s = t; e > s; s += 2) {
+ var n = this.get(s)
+ , r = this.get(s + 1);
+ i += String.fromCharCode((n << 8) + r)
+ }
+ return i
+ }
+ ,
+ e.prototype.reTime = /^((?:1[89]|2\d)?\d\d)(0[1-9]|1[0-2])(0[1-9]|[12]\d|3[01])([01]\d|2[0-3])(?:([0-5]\d)(?:([0-5]\d)(?:[.,](\d{1,3}))?)?)?(Z|[-+](?:[0]\d|1[0-2])([0-5]\d)?)?$/,
+ e.prototype.parseTime = function (t, e) {
+ var i = this.parseStringISO(t, e)
+ , s = this.reTime.exec(i);
+ return s ? (i = s[1] + "-" + s[2] + "-" + s[3] + " " + s[4],
+ s[5] && (i += ":" + s[5],
+ s[6] && (i += ":" + s[6],
+ s[7] && (i += "." + s[7]))),
+ s[8] && (i += " UTC",
+ "Z" != s[8] && (i += s[8],
+ s[9] && (i += ":" + s[9]))),
+ i) : "Unrecognized time: " + i
+ }
+ ,
+ e.prototype.parseInteger = function (t, e) {
+ var i = e - t;
+ if (i > 4) {
+ i <<= 3;
+ var s = this.get(t);
+ if (0 === s)
+ i -= 8;
+ else
+ for (; 128 > s;)
+ s <<= 1,
+ --i;
+ return "(" + i + " bit)"
+ }
+ for (var n = 0, r = t; e > r; ++r)
+ n = n << 8 | this.get(r);
+ return n
+ }
+ ,
+ e.prototype.parseBitString = function (t, e) {
+ var i = this.get(t)
+ , s = (e - t - 1 << 3) - i
+ , n = "(" + s + " bit)";
+ if (20 >= s) {
+ var r = i;
+ n += " ";
+ for (var o = e - 1; o > t; --o) {
+ for (var a = this.get(o), c = r; 8 > c; ++c)
+ n += a >> c & 1 ? "1" : "0";
+ r = 0
+ }
+ }
+ return n
+ }
+ ,
+ e.prototype.parseOctetString = function (t, e) {
+ var i = e - t
+ , r = "(" + i + " byte) ";
+ i > s && (e = t + s);
+ for (var o = t; e > o; ++o)
+ r += this.hexByte(this.get(o));
+ return i > s && (r += n),
+ r
+ }
+ ,
+ e.prototype.parseOID = function (t, e) {
+ for (var i = "", s = 0, n = 0, r = t; e > r; ++r) {
+ var o = this.get(r);
+ if (s = s << 7 | 127 & o,
+ n += 7,
+ !(128 & o)) {
+ if ("" === i) {
+ var a = 80 > s ? 40 > s ? 0 : 1 : 2;
+ i = a + "." + (s - 40 * a)
+ } else
+ i += "." + (n >= 31 ? "bigint" : s);
+ s = n = 0
+ }
+ }
+ return i
+ }
+ ,
+ i.prototype.typeName = function () {
+ if (this.tag === t)
+ return "unknown";
+ var e = this.tag >> 6
+ , i = (this.tag >> 5 & 1,
+ 31 & this.tag);
+ switch (e) {
+ case 0:
+ switch (i) {
+ case 0:
+ return "EOC";
+ case 1:
+ return "BOOLEAN";
+ case 2:
+ return "INTEGER";
+ case 3:
+ return "BIT_STRING";
+ case 4:
+ return "OCTET_STRING";
+ case 5:
+ return "NULL";
+ case 6:
+ return "OBJECT_IDENTIFIER";
+ case 7:
+ return "ObjectDescriptor";
+ case 8:
+ return "EXTERNAL";
+ case 9:
+ return "REAL";
+ case 10:
+ return "ENUMERATED";
+ case 11:
+ return "EMBEDDED_PDV";
+ case 12:
+ return "UTF8String";
+ case 16:
+ return "SEQUENCE";
+ case 17:
+ return "SET";
+ case 18:
+ return "NumericString";
+ case 19:
+ return "PrintableString";
+ case 20:
+ return "TeletexString";
+ case 21:
+ return "VideotexString";
+ case 22:
+ return "IA5String";
+ case 23:
+ return "UTCTime";
+ case 24:
+ return "GeneralizedTime";
+ case 25:
+ return "GraphicString";
+ case 26:
+ return "VisibleString";
+ case 27:
+ return "GeneralString";
+ case 28:
+ return "UniversalString";
+ case 30:
+ return "BMPString";
+ default:
+ return "Universal_" + i.toString(16)
+ }
+ case 1:
+ return "Application_" + i.toString(16);
+ case 2:
+ return "[" + i + "]";
+ case 3:
+ return "Private_" + i.toString(16)
+ }
+ }
+ ,
+ i.prototype.reSeemsASCII = /^[ -~]+$/,
+ i.prototype.content = function () {
+ if (this.tag === t)
+ return null;
+ var e = this.tag >> 6
+ , i = 31 & this.tag
+ , r = this.posContent()
+ , o = Math.abs(this.length);
+ if (0 !== e) {
+ if (null !== this.sub)
+ return "(" + this.sub.length + " elem)";
+ var a = this.stream.parseStringISO(r, r + Math.min(o, s));
+ return this.reSeemsASCII.test(a) ? a.substring(0, 2 * s) + (a.length > 2 * s ? n : "") : this.stream.parseOctetString(r, r + o)
+ }
+ switch (i) {
+ case 1:
+ return 0 === this.stream.get(r) ? "false" : "true";
+ case 2:
+ return this.stream.parseInteger(r, r + o);
+ case 3:
+ return this.sub ? "(" + this.sub.length + " elem)" : this.stream.parseBitString(r, r + o);
+ case 4:
+ return this.sub ? "(" + this.sub.length + " elem)" : this.stream.parseOctetString(r, r + o);
+ case 6:
+ return this.stream.parseOID(r, r + o);
+ case 16:
+ case 17:
+ return "(" + this.sub.length + " elem)";
+ case 12:
+ return this.stream.parseStringUTF(r, r + o);
+ case 18:
+ case 19:
+ case 20:
+ case 21:
+ case 22:
+ case 26:
+ return this.stream.parseStringISO(r, r + o);
+ case 30:
+ return this.stream.parseStringBMP(r, r + o);
+ case 23:
+ case 24:
+ return this.stream.parseTime(r, r + o)
+ }
+ return null
+ }
+ ,
+ i.prototype.toString = function () {
+ return this.typeName() + "@" + this.stream.pos + "[header:" + this.header + ",length:" + this.length + ",sub:" + (null === this.sub ? "null" : this.sub.length) + "]"
+ }
+ ,
+ i.prototype.print = function (e) {
+ if (e === t && (e = ""),
+ document.writeln(e + this),
+ null !== this.sub) {
+ e += " ";
+ for (var i = 0, s = this.sub.length; s > i; ++i)
+ this.sub[i].print(e)
+ }
+ }
+ ,
+ i.prototype.toPrettyString = function (e) {
+ e === t && (e = "");
+ var i = e + this.typeName() + " @" + this.stream.pos;
+ if (this.length >= 0 && (i += "+"),
+ i += this.length,
+ 32 & this.tag ? i += " (constructed)" : 3 != this.tag && 4 != this.tag || null === this.sub || (i += " (encapsulates)"),
+ i += "\n",
+ null !== this.sub) {
+ e += " ";
+ for (var s = 0, n = this.sub.length; n > s; ++s)
+ i += this.sub[s].toPrettyString(e)
+ }
+ return i
+ }
+ ,
+ i.prototype.toDOM = function () {
+ var t = r.tag("div", "node");
+ t.asn1 = this;
+ var e = r.tag("div", "head")
+ , i = this.typeName().replace(/_/g, " ");
+ e.innerHTML = i;
+ var s = this.content();
+ if (null !== s) {
+ s = String(s).replace(/",
+ i += "Length: " + this.header + "+",
+ i += this.length >= 0 ? this.length : -this.length + " (undefined)",
+ 32 & this.tag ? i += "
+ * This name space provides following name spaces:
+ *
(constructed)" : 3 != this.tag && 4 != this.tag || null === this.sub || (i += "
(encapsulates)"),
+ null !== s && (i += "
Value:
" + s + "",
+ "object" == typeof oids && 6 == this.tag)) {
+ var a = oids[s];
+ a && (a.d && (i += "
" + a.d),
+ a.c && (i += "
" + a.c),
+ a.w && (i += "
(warning!)"))
+ }
+ o.innerHTML = i,
+ t.appendChild(o);
+ var c = r.tag("div", "sub");
+ if (null !== this.sub)
+ for (var l = 0, u = this.sub.length; u > l; ++l)
+ c.appendChild(this.sub[l].toDOM());
+ return t.appendChild(c),
+ e.onclick = function () {
+ t.className = "node collapsed" == t.className ? "node" : "node collapsed"
+ }
+ ,
+ t
+ }
+ ,
+ i.prototype.posStart = function () {
+ return this.stream.pos
+ }
+ ,
+ i.prototype.posContent = function () {
+ return this.stream.pos + this.header
+ }
+ ,
+ i.prototype.posEnd = function () {
+ return this.stream.pos + this.header + Math.abs(this.length)
+ }
+ ,
+ i.prototype.fakeHover = function (t) {
+ this.node.className += " hover",
+ t && (this.head.className += " hover")
+ }
+ ,
+ i.prototype.fakeOut = function (t) {
+ var e = / ?hover/;
+ this.node.className = this.node.className.replace(e, ""),
+ t && (this.head.className = this.head.className.replace(e, ""))
+ }
+ ,
+ i.prototype.toHexDOM_sub = function (t, e, i, s, n) {
+ if (!(s >= n)) {
+ var o = r.tag("span", e);
+ o.appendChild(r.text(i.hexDump(s, n))),
+ t.appendChild(o)
+ }
+ }
+ ,
+ i.prototype.toHexDOM = function (e) {
+ var i = r.tag("span", "hex");
+ if (e === t && (e = i),
+ this.head.hexNode = i,
+ this.head.onmouseover = function () {
+ this.hexNode.className = "hexCurrent"
+ }
+ ,
+ this.head.onmouseout = function () {
+ this.hexNode.className = "hex"
+ }
+ ,
+ i.asn1 = this,
+ i.onmouseover = function () {
+ var t = !e.selected;
+ t && (e.selected = this.asn1,
+ this.className = "hexCurrent"),
+ this.asn1.fakeHover(t)
+ }
+ ,
+ i.onmouseout = function () {
+ var t = e.selected == this.asn1;
+ this.asn1.fakeOut(t),
+ t && (e.selected = null,
+ this.className = "hex")
+ }
+ ,
+ this.toHexDOM_sub(i, "tag", this.stream, this.posStart(), this.posStart() + 1),
+ this.toHexDOM_sub(i, this.length >= 0 ? "dlen" : "ulen", this.stream, this.posStart() + 1, this.posContent()),
+ null === this.sub)
+ i.appendChild(r.text(this.stream.hexDump(this.posContent(), this.posEnd())));
+ else if (this.sub.length > 0) {
+ var s = this.sub[0]
+ , n = this.sub[this.sub.length - 1];
+ this.toHexDOM_sub(i, "intro", this.stream, this.posContent(), s.posStart());
+ for (var o = 0, a = this.sub.length; a > o; ++o)
+ i.appendChild(this.sub[o].toHexDOM(e));
+ this.toHexDOM_sub(i, "outro", this.stream, n.posEnd(), this.posEnd())
+ }
+ return i
+ }
+ ,
+ i.prototype.toHexString = function (t) {
+ return this.stream.hexDump(this.posStart(), this.posEnd(), !0)
+ }
+ ,
+ i.decodeLength = function (t) {
+ var e = t.get()
+ , i = 127 & e;
+ if (i == e)
+ return i;
+ if (i > 3)
+ throw "Length over 24 bits not supported at position " + (t.pos - 1);
+ if (0 === i)
+ return -1;
+ e = 0;
+ for (var s = 0; i > s; ++s)
+ e = e << 8 | t.get();
+ return e
+ }
+ ,
+ i.hasContent = function (t, s, n) {
+ if (32 & t)
+ return !0;
+ if (3 > t || t > 4)
+ return !1;
+ var r = new e(n);
+ 3 == t && r.get();
+ var o = r.get();
+ if (o >> 6 & 1)
+ return !1;
+ try {
+ var a = i.decodeLength(r);
+ return r.pos - n.pos + a == s
+ } catch (t) {
+ return !1
+ }
+ }
+ ,
+ i.decode = function (t) {
+ t instanceof e || (t = new e(t, 0));
+ var s = new e(t)
+ , n = t.get()
+ , r = i.decodeLength(t)
+ , o = t.pos - s.pos
+ , a = null;
+ if (i.hasContent(n, r, t)) {
+ var c = t.pos;
+ if (3 == n && t.get(),
+ a = [],
+ r >= 0) {
+ for (var l = c + r; t.pos < l;)
+ a[a.length] = i.decode(t);
+ if (t.pos != l)
+ throw "Content size is not correct for container starting at offset " + c
+ } else
+ try {
+ for (; ;) {
+ var u = i.decode(t);
+ if (0 === u.tag)
+ break;
+ a[a.length] = u
+ }
+ r = c - t.pos
+ } catch (t) {
+ throw "Exception while decoding undefined length content: " + t
+ }
+ } else
+ t.pos += r;
+ return new i(s, o, r, n, a)
+ }
+ ,
+ i.test = function () {
+ for (var t = [{
+ value: [39],
+ expected: 39
+ }, {
+ value: [129, 201],
+ expected: 201
+ }, {
+ value: [131, 254, 220, 186],
+ expected: 16702650
+ }], s = 0, n = t.length; n > s; ++s) {
+ var r = new e(t[s].value, 0)
+ , o = i.decodeLength(r);
+ o != t[s].expected && document.write("In test[" + s + "] expected " + t[s].expected + " got " + o + "\n")
+ }
+ }
+ ,
+ window.ASN1 = i
+ }(),
+ ASN1.prototype.getHexStringValue = function () {
+ var t = this.toHexString()
+ , e = 2 * this.header
+ , i = 2 * this.length;
+ return t.substr(e, i)
+ }
+ ,
+ le.prototype.parseKey = function (t) {
+ try {
+ var e = 0
+ , i = 0
+ , s = /^\s*(?:[0-9A-Fa-f][0-9A-Fa-f]\s*)+$/
+ , n = s.test(t) ? Hex.decode(t) : Base64.unarmor(t)
+ , r = ASN1.decode(n);
+ if (3 === r.sub.length && (r = r.sub[2].sub[0]),
+ 9 === r.sub.length) {
+ e = r.sub[1].getHexStringValue(),
+ this.n = ae(e, 16),
+ i = r.sub[2].getHexStringValue(),
+ this.e = parseInt(i, 16);
+ var o = r.sub[3].getHexStringValue();
+ this.d = ae(o, 16);
+ var a = r.sub[4].getHexStringValue();
+ this.p = ae(a, 16);
+ var c = r.sub[5].getHexStringValue();
+ this.q = ae(c, 16);
+ var l = r.sub[6].getHexStringValue();
+ this.dmp1 = ae(l, 16);
+ var u = r.sub[7].getHexStringValue();
+ this.dmq1 = ae(u, 16);
+ var p = r.sub[8].getHexStringValue();
+ this.coeff = ae(p, 16)
+ } else {
+ if (2 !== r.sub.length)
+ return !1;
+ var d = r.sub[1]
+ , h = d.sub[0];
+ e = h.sub[0].getHexStringValue(),
+ this.n = ae(e, 16),
+ i = h.sub[1].getHexStringValue(),
+ this.e = parseInt(i, 16)
+ }
+ return !0
+ } catch (t) {
+ return !1
+ }
+ }
+ ,
+ le.prototype.getPrivateBaseKey = function () {
+ var t = {
+ array: [new KJUR.asn1.DERInteger({
+ int: 0
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.n
+ }), new KJUR.asn1.DERInteger({
+ int: this.e
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.d
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.p
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.q
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.dmp1
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.dmq1
+ }), new KJUR.asn1.DERInteger({
+ bigint: this.coeff
+ })]
+ }
+ , e = new KJUR.asn1.DERSequence(t);
+ return e.getEncodedHex()
+ }
+ ,
+ le.prototype.getPrivateBaseKeyB64 = function () {
+ return be(this.getPrivateBaseKey())
+ }
+ ,
+ le.prototype.getPublicBaseKey = function () {
+ var t = {
+ array: [new KJUR.asn1.DERObjectIdentifier({
+ oid: "1.2.840.113549.1.1.1"
+ }), new KJUR.asn1.DERNull]
+ }
+ , e = new KJUR.asn1.DERSequence(t);
+ t = {
+ array: [new KJUR.asn1.DERInteger({
+ bigint: this.n
+ }), new KJUR.asn1.DERInteger({
+ int: this.e
+ })]
+ };
+ var i = new KJUR.asn1.DERSequence(t);
+ t = {
+ hex: "00" + i.getEncodedHex()
+ };
+ var s = new KJUR.asn1.DERBitString(t);
+ t = {
+ array: [e, s]
+ };
+ var n = new KJUR.asn1.DERSequence(t);
+ return n.getEncodedHex()
+ }
+ ,
+ le.prototype.getPublicBaseKeyB64 = function () {
+ return be(this.getPublicBaseKey())
+ }
+ ,
+ le.prototype.wordwrap = function (t, e) {
+ if (e = e || 64,
+ !t)
+ return t;
+ var i = "(.{1," + e + "})( +|$\n?)|(.{1," + e + "})";
+ return t.match(RegExp(i, "g")).join("\n")
+ }
+ ,
+ le.prototype.getPrivateKey = function () {
+ var t = "-----BEGIN RSA PRIVATE KEY-----\n";
+ return t += this.wordwrap(this.getPrivateBaseKeyB64()) + "\n",
+ t += "-----END RSA PRIVATE KEY-----"
+ }
+ ,
+ le.prototype.getPublicKey = function () {
+ var t = "-----BEGIN PUBLIC KEY-----\n";
+ return t += this.wordwrap(this.getPublicBaseKeyB64()) + "\n",
+ t += "-----END PUBLIC KEY-----"
+ }
+ ,
+ le.prototype.hasPublicKeyProperty = function (t) {
+ return t = t || {},
+ t.hasOwnProperty("n") && t.hasOwnProperty("e")
+ }
+ ,
+ le.prototype.hasPrivateKeyProperty = function (t) {
+ return t = t || {},
+ t.hasOwnProperty("n") && t.hasOwnProperty("e") && t.hasOwnProperty("d") && t.hasOwnProperty("p") && t.hasOwnProperty("q") && t.hasOwnProperty("dmp1") && t.hasOwnProperty("dmq1") && t.hasOwnProperty("coeff")
+ }
+ ,
+ le.prototype.parsePropertiesFrom = function (t) {
+ this.n = t.n,
+ this.e = t.e,
+ t.hasOwnProperty("d") && (this.d = t.d,
+ this.p = t.p,
+ this.q = t.q,
+ this.dmp1 = t.dmp1,
+ this.dmq1 = t.dmq1,
+ this.coeff = t.coeff)
+ }
+ ;
+ var He = function (t) {
+ le.call(this),
+ t && ("string" == typeof t ? this.parseKey(t) : (this.hasPrivateKeyProperty(t) || this.hasPublicKeyProperty(t)) && this.parsePropertiesFrom(t))
+ };
+ He.prototype = new le,
+ He.prototype.constructor = He;
+ var qe = function (t) {
+ t = t || {},
+ this.default_key_size = parseInt(t.default_key_size) || 1024,
+ this.default_public_exponent = t.default_public_exponent || "010001",
+ this.log = t.log || !1,
+ this.key = null
+ };
+ qe.prototype.setKey = function (t) {
+ this.log && this.key && console.warn("A key was already set, overriding existing."),
+ this.key = new He(t)
+ }
+ ,
+ qe.prototype.setPrivateKey = function (t) {
+ this.setKey(t)
+ }
+ ,
+ qe.prototype.setPublicKey = function (t) {
+ this.setKey(t)
+ }
+ ,
+ qe.prototype.decrypt = function (t) {
+ try {
+ return this.getKey().decrypt(ye(t))
+ } catch (t) {
+ return !1
+ }
+ }
+ ,
+ qe.prototype.encrypt = function (t) {
+ try {
+ return be(this.getKey().encrypt(t))
+ } catch (t) {
+ return !1
+ }
+ }
+ ,
+ qe.prototype.getKey = function (t) {
+ if (!this.key) {
+ if (this.key = new He,
+ t && "[object Function]" === {}.toString.call(t))
+ return void this.key.generateAsync(this.default_key_size, this.default_public_exponent, t);
+ this.key.generate(this.default_key_size, this.default_public_exponent)
+ }
+ return this.key
+ }
+ ,
+ qe.prototype.getPrivateKey = function () {
+ return this.getKey().getPrivateKey()
+ }
+ ,
+ qe.prototype.getPrivateKeyB64 = function () {
+ return this.getKey().getPrivateBaseKeyB64()
+ }
+ ,
+ qe.prototype.getPublicKey = function () {
+ return this.getKey().getPublicKey()
+ }
+ ,
+ qe.prototype.getPublicKeyB64 = function () {
+ return this.getKey().getPublicBaseKeyB64()
+ }
+ ,
+ qe.version = "2.3.1",
+ t.JSEncrypt = qe
+ })
+ }
+ .call(e, i, e, t),
+ !(void 0 !== s && (t.exports = s))
+ },
+ 3: function (t, e, i) {
+ var s;
+ s = function (t, e, s) {
+ function n() {
+ "undefined" != typeof r && (this.jsencrypt = new r.JSEncrypt,
+ this.jsencrypt.setPublicKey("-----BEGIN PUBLIC KEY-----MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDq04c6My441Gj0UFKgrqUhAUg+kQZeUeWSPlAU9fr4HBPDldAeqzx1UR92KJHuQh/zs1HOamE2dgX9z/2oXcJaqoRIA/FXysx+z2YlJkSk8XQLcQ8EBOkp//MZrixam7lCYpNOjadQBb2Ot0U/Ky+jF2p+Ie8gSZ7/u+Wnr5grywIDAQAB-----END PUBLIC KEY-----"))
+ }
+
+ var r = i("4");
+ n.prototype.encode = function (t, e) {
+ var i = e ? e + "|" + t : t;
+ return encodeURIComponent(this.jsencrypt.encrypt(i))
+ }
+ ,
+ s.exports = n
+ }
+ .call(e, i, e, t),
+ !(void 0 !== s && (t.exports = s))
+ }
+ }
+)
+
+
+function getEncryptedPassword(password) {
+ var timestamp = (new Date).getTime();
+ var encryptFunc = eFunc("3");
+ var encrypt = new encryptFunc;
+ return encrypt.encode(password, timestamp)
+}
+
+// 测试样例
+// console.log(getEncryptedPassword("12345678"))
diff --git a/JSReverse/www_gm99_com/gm99_encrypt_2.js b/JSReverse/www_gm99_com/gm99_encrypt_2.js
new file mode 100644
index 0000000000000000000000000000000000000000..b4f65aa5874796d65c59805e62acda4fededf635
--- /dev/null
+++ b/JSReverse/www_gm99_com/gm99_encrypt_2.js
@@ -0,0 +1,4635 @@
+/*
+引用 jsencrypt 加密模块,此脚适合在 nodejs 环境下运行。
+1、使用 require 语句引用,前提是使用 npm 安装过;
+2、将 jsencrypt.js 直接粘贴到此脚本中使用,同时要将结尾 exports.JSEncrypt = JSEncrypt; 改为 je = JSEncrypt 导出方法。
+PS:需要定义 var navigator = {}; var window = global;,否则提示未定义。
+*/
+
+// ========================= 1、require 方式引用 =========================
+// var je = require("jsencrypt")
+
+// ==================== 2、直接将 jsencrypt.js 复制过来 ====================
+/*! JSEncrypt v2.3.1 | https://npmcdn.com/jsencrypt@2.3.1/LICENSE.txt */
+var navigator = {};
+var window = global;
+
+(function (root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD
+ define(['exports'], factory);
+ } else if (typeof exports === 'object' && typeof exports.nodeName !== 'string') {
+ // Node, CommonJS-like
+ factory(module.exports);
+ } else {
+ factory(root);
+ }
+})(this, function (exports) {
+ // Copyright (c) 2005 Tom Wu
+// All Rights Reserved.
+// See "LICENSE" for details.
+
+// Basic JavaScript BN library - subset useful for RSA encryption.
+
+// Bits per digit
+ var dbits;
+
+// JavaScript engine analysis
+ var canary = 0xdeadbeefcafe;
+ var j_lm = ((canary & 0xffffff) == 0xefcafe);
+
+// (public) Constructor
+ function BigInteger(a, b, c) {
+ if (a != null)
+ if ("number" == typeof a) this.fromNumber(a, b, c);
+ else if (b == null && "string" != typeof a) this.fromString(a, 256);
+ else this.fromString(a, b);
+ }
+
+// return new, unset BigInteger
+ function nbi() {
+ return new BigInteger(null);
+ }
+
+// am: Compute w_j += (x*this_i), propagate carries,
+// c is initial carry, returns final carry.
+// c < 3*dvalue, x < 2*dvalue, this_i < dvalue
+// We need to select the fastest one that works in this environment.
+
+// am1: use a single mult and divide to get the high bits,
+// max digit bits should be 26 because
+// max internal value = 2*dvalue^2-2*dvalue (< 2^53)
+ function am1(i, x, w, j, c, n) {
+ while (--n >= 0) {
+ var v = x * this[i++] + w[j] + c;
+ c = Math.floor(v / 0x4000000);
+ w[j++] = v & 0x3ffffff;
+ }
+ return c;
+ }
+
+// am2 avoids a big mult-and-extract completely.
+// Max digit bits should be <= 30 because we do bitwise ops
+// on values up to 2*hdvalue^2-hdvalue-1 (< 2^31)
+ function am2(i, x, w, j, c, n) {
+ var xl = x & 0x7fff, xh = x >> 15;
+ while (--n >= 0) {
+ var l = this[i] & 0x7fff;
+ var h = this[i++] >> 15;
+ var m = xh * l + h * xl;
+ l = xl * l + ((m & 0x7fff) << 15) + w[j] + (c & 0x3fffffff);
+ c = (l >>> 30) + (m >>> 15) + xh * h + (c >>> 30);
+ w[j++] = l & 0x3fffffff;
+ }
+ return c;
+ }
+
+// Alternately, set max digit bits to 28 since some
+// browsers slow down when dealing with 32-bit numbers.
+ function am3(i, x, w, j, c, n) {
+ var xl = x & 0x3fff, xh = x >> 14;
+ while (--n >= 0) {
+ var l = this[i] & 0x3fff;
+ var h = this[i++] >> 14;
+ var m = xh * l + h * xl;
+ l = xl * l + ((m & 0x3fff) << 14) + w[j] + c;
+ c = (l >> 28) + (m >> 14) + xh * h;
+ w[j++] = l & 0xfffffff;
+ }
+ return c;
+ }
+
+ if (j_lm && (navigator.appName == "Microsoft Internet Explorer")) {
+ BigInteger.prototype.am = am2;
+ dbits = 30;
+ } else if (j_lm && (navigator.appName != "Netscape")) {
+ BigInteger.prototype.am = am1;
+ dbits = 26;
+ } else { // Mozilla/Netscape seems to prefer am3
+ BigInteger.prototype.am = am3;
+ dbits = 28;
+ }
+
+ BigInteger.prototype.DB = dbits;
+ BigInteger.prototype.DM = ((1 << dbits) - 1);
+ BigInteger.prototype.DV = (1 << dbits);
+
+ var BI_FP = 52;
+ BigInteger.prototype.FV = Math.pow(2, BI_FP);
+ BigInteger.prototype.F1 = BI_FP - dbits;
+ BigInteger.prototype.F2 = 2 * dbits - BI_FP;
+
+// Digit conversions
+ var BI_RM = "0123456789abcdefghijklmnopqrstuvwxyz";
+ var BI_RC = new Array();
+ var rr, vv;
+ rr = "0".charCodeAt(0);
+ for (vv = 0; vv <= 9; ++vv) BI_RC[rr++] = vv;
+ rr = "a".charCodeAt(0);
+ for (vv = 10; vv < 36; ++vv) BI_RC[rr++] = vv;
+ rr = "A".charCodeAt(0);
+ for (vv = 10; vv < 36; ++vv) BI_RC[rr++] = vv;
+
+ function int2char(n) {
+ return BI_RM.charAt(n);
+ }
+
+ function intAt(s, i) {
+ var c = BI_RC[s.charCodeAt(i)];
+ return (c == null) ? -1 : c;
+ }
+
+// (protected) copy this to r
+ function bnpCopyTo(r) {
+ for (var i = this.t - 1; i >= 0; --i) r[i] = this[i];
+ r.t = this.t;
+ r.s = this.s;
+ }
+
+// (protected) set from integer value x, -DV <= x < DV
+ function bnpFromInt(x) {
+ this.t = 1;
+ this.s = (x < 0) ? -1 : 0;
+ if (x > 0) this[0] = x;
+ else if (x < -1) this[0] = x + this.DV;
+ else this.t = 0;
+ }
+
+// return bigint initialized to value
+ function nbv(i) {
+ var r = nbi();
+ r.fromInt(i);
+ return r;
+ }
+
+// (protected) set from string and radix
+ function bnpFromString(s, b) {
+ var k;
+ if (b == 16) k = 4;
+ else if (b == 8) k = 3;
+ else if (b == 256) k = 8; // byte array
+ else if (b == 2) k = 1;
+ else if (b == 32) k = 5;
+ else if (b == 4) k = 2;
+ else {
+ this.fromRadix(s, b);
+ return;
+ }
+ this.t = 0;
+ this.s = 0;
+ var i = s.length, mi = false, sh = 0;
+ while (--i >= 0) {
+ var x = (k == 8) ? s[i] & 0xff : intAt(s, i);
+ if (x < 0) {
+ if (s.charAt(i) == "-") mi = true;
+ continue;
+ }
+ mi = false;
+ if (sh == 0)
+ this[this.t++] = x;
+ else if (sh + k > this.DB) {
+ this[this.t - 1] |= (x & ((1 << (this.DB - sh)) - 1)) << sh;
+ this[this.t++] = (x >> (this.DB - sh));
+ } else
+ this[this.t - 1] |= x << sh;
+ sh += k;
+ if (sh >= this.DB) sh -= this.DB;
+ }
+ if (k == 8 && (s[0] & 0x80) != 0) {
+ this.s = -1;
+ if (sh > 0) this[this.t - 1] |= ((1 << (this.DB - sh)) - 1) << sh;
+ }
+ this.clamp();
+ if (mi) BigInteger.ZERO.subTo(this, this);
+ }
+
+// (protected) clamp off excess high words
+ function bnpClamp() {
+ var c = this.s & this.DM;
+ while (this.t > 0 && this[this.t - 1] == c) --this.t;
+ }
+
+// (public) return string representation in given radix
+ function bnToString(b) {
+ if (this.s < 0) return "-" + this.negate().toString(b);
+ var k;
+ if (b == 16) k = 4;
+ else if (b == 8) k = 3;
+ else if (b == 2) k = 1;
+ else if (b == 32) k = 5;
+ else if (b == 4) k = 2;
+ else return this.toRadix(b);
+ var km = (1 << k) - 1, d, m = false, r = "", i = this.t;
+ var p = this.DB - (i * this.DB) % k;
+ if (i-- > 0) {
+ if (p < this.DB && (d = this[i] >> p) > 0) {
+ m = true;
+ r = int2char(d);
+ }
+ while (i >= 0) {
+ if (p < k) {
+ d = (this[i] & ((1 << p) - 1)) << (k - p);
+ d |= this[--i] >> (p += this.DB - k);
+ } else {
+ d = (this[i] >> (p -= k)) & km;
+ if (p <= 0) {
+ p += this.DB;
+ --i;
+ }
+ }
+ if (d > 0) m = true;
+ if (m) r += int2char(d);
+ }
+ }
+ return m ? r : "0";
+ }
+
+// (public) -this
+ function bnNegate() {
+ var r = nbi();
+ BigInteger.ZERO.subTo(this, r);
+ return r;
+ }
+
+// (public) |this|
+ function bnAbs() {
+ return (this.s < 0) ? this.negate() : this;
+ }
+
+// (public) return + if this > a, - if this < a, 0 if equal
+ function bnCompareTo(a) {
+ var r = this.s - a.s;
+ if (r != 0) return r;
+ var i = this.t;
+ r = i - a.t;
+ if (r != 0) return (this.s < 0) ? -r : r;
+ while (--i >= 0) if ((r = this[i] - a[i]) != 0) return r;
+ return 0;
+ }
+
+// returns bit length of the integer x
+ function nbits(x) {
+ var r = 1, t;
+ if ((t = x >>> 16) != 0) {
+ x = t;
+ r += 16;
+ }
+ if ((t = x >> 8) != 0) {
+ x = t;
+ r += 8;
+ }
+ if ((t = x >> 4) != 0) {
+ x = t;
+ r += 4;
+ }
+ if ((t = x >> 2) != 0) {
+ x = t;
+ r += 2;
+ }
+ if ((t = x >> 1) != 0) {
+ x = t;
+ r += 1;
+ }
+ return r;
+ }
+
+// (public) return the number of bits in "this"
+ function bnBitLength() {
+ if (this.t <= 0) return 0;
+ return this.DB * (this.t - 1) + nbits(this[this.t - 1] ^ (this.s & this.DM));
+ }
+
+// (protected) r = this << n*DB
+ function bnpDLShiftTo(n, r) {
+ var i;
+ for (i = this.t - 1; i >= 0; --i) r[i + n] = this[i];
+ for (i = n - 1; i >= 0; --i) r[i] = 0;
+ r.t = this.t + n;
+ r.s = this.s;
+ }
+
+// (protected) r = this >> n*DB
+ function bnpDRShiftTo(n, r) {
+ for (var i = n; i < this.t; ++i) r[i - n] = this[i];
+ r.t = Math.max(this.t - n, 0);
+ r.s = this.s;
+ }
+
+// (protected) r = this << n
+ function bnpLShiftTo(n, r) {
+ var bs = n % this.DB;
+ var cbs = this.DB - bs;
+ var bm = (1 << cbs) - 1;
+ var ds = Math.floor(n / this.DB), c = (this.s << bs) & this.DM, i;
+ for (i = this.t - 1; i >= 0; --i) {
+ r[i + ds + 1] = (this[i] >> cbs) | c;
+ c = (this[i] & bm) << bs;
+ }
+ for (i = ds - 1; i >= 0; --i) r[i] = 0;
+ r[ds] = c;
+ r.t = this.t + ds + 1;
+ r.s = this.s;
+ r.clamp();
+ }
+
+// (protected) r = this >> n
+ function bnpRShiftTo(n, r) {
+ r.s = this.s;
+ var ds = Math.floor(n / this.DB);
+ if (ds >= this.t) {
+ r.t = 0;
+ return;
+ }
+ var bs = n % this.DB;
+ var cbs = this.DB - bs;
+ var bm = (1 << bs) - 1;
+ r[0] = this[ds] >> bs;
+ for (var i = ds + 1; i < this.t; ++i) {
+ r[i - ds - 1] |= (this[i] & bm) << cbs;
+ r[i - ds] = this[i] >> bs;
+ }
+ if (bs > 0) r[this.t - ds - 1] |= (this.s & bm) << cbs;
+ r.t = this.t - ds;
+ r.clamp();
+ }
+
+// (protected) r = this - a
+ function bnpSubTo(a, r) {
+ var i = 0, c = 0, m = Math.min(a.t, this.t);
+ while (i < m) {
+ c += this[i] - a[i];
+ r[i++] = c & this.DM;
+ c >>= this.DB;
+ }
+ if (a.t < this.t) {
+ c -= a.s;
+ while (i < this.t) {
+ c += this[i];
+ r[i++] = c & this.DM;
+ c >>= this.DB;
+ }
+ c += this.s;
+ } else {
+ c += this.s;
+ while (i < a.t) {
+ c -= a[i];
+ r[i++] = c & this.DM;
+ c >>= this.DB;
+ }
+ c -= a.s;
+ }
+ r.s = (c < 0) ? -1 : 0;
+ if (c < -1) r[i++] = this.DV + c;
+ else if (c > 0) r[i++] = c;
+ r.t = i;
+ r.clamp();
+ }
+
+// (protected) r = this * a, r != this,a (HAC 14.12)
+// "this" should be the larger one if appropriate.
+ function bnpMultiplyTo(a, r) {
+ var x = this.abs(), y = a.abs();
+ var i = x.t;
+ r.t = i + y.t;
+ while (--i >= 0) r[i] = 0;
+ for (i = 0; i < y.t; ++i) r[i + x.t] = x.am(0, y[i], r, i, 0, x.t);
+ r.s = 0;
+ r.clamp();
+ if (this.s != a.s) BigInteger.ZERO.subTo(r, r);
+ }
+
+// (protected) r = this^2, r != this (HAC 14.16)
+ function bnpSquareTo(r) {
+ var x = this.abs();
+ var i = r.t = 2 * x.t;
+ while (--i >= 0) r[i] = 0;
+ for (i = 0; i < x.t - 1; ++i) {
+ var c = x.am(i, x[i], r, 2 * i, 0, 1);
+ if ((r[i + x.t] += x.am(i + 1, 2 * x[i], r, 2 * i + 1, c, x.t - i - 1)) >= x.DV) {
+ r[i + x.t] -= x.DV;
+ r[i + x.t + 1] = 1;
+ }
+ }
+ if (r.t > 0) r[r.t - 1] += x.am(i, x[i], r, 2 * i, 0, 1);
+ r.s = 0;
+ r.clamp();
+ }
+
+// (protected) divide this by m, quotient and remainder to q, r (HAC 14.20)
+// r != q, this != m. q or r may be null.
+ function bnpDivRemTo(m, q, r) {
+ var pm = m.abs();
+ if (pm.t <= 0) return;
+ var pt = this.abs();
+ if (pt.t < pm.t) {
+ if (q != null) q.fromInt(0);
+ if (r != null) this.copyTo(r);
+ return;
+ }
+ if (r == null) r = nbi();
+ var y = nbi(), ts = this.s, ms = m.s;
+ var nsh = this.DB - nbits(pm[pm.t - 1]); // normalize modulus
+ if (nsh > 0) {
+ pm.lShiftTo(nsh, y);
+ pt.lShiftTo(nsh, r);
+ } else {
+ pm.copyTo(y);
+ pt.copyTo(r);
+ }
+ var ys = y.t;
+ var y0 = y[ys - 1];
+ if (y0 == 0) return;
+ var yt = y0 * (1 << this.F1) + ((ys > 1) ? y[ys - 2] >> this.F2 : 0);
+ var d1 = this.FV / yt, d2 = (1 << this.F1) / yt, e = 1 << this.F2;
+ var i = r.t, j = i - ys, t = (q == null) ? nbi() : q;
+ y.dlShiftTo(j, t);
+ if (r.compareTo(t) >= 0) {
+ r[r.t++] = 1;
+ r.subTo(t, r);
+ }
+ BigInteger.ONE.dlShiftTo(ys, t);
+ t.subTo(y, y); // "negative" y so we can replace sub with am later
+ while (y.t < ys) y[y.t++] = 0;
+ while (--j >= 0) {
+ // Estimate quotient digit
+ var qd = (r[--i] == y0) ? this.DM : Math.floor(r[i] * d1 + (r[i - 1] + e) * d2);
+ if ((r[i] += y.am(0, qd, r, j, 0, ys)) < qd) { // Try it out
+ y.dlShiftTo(j, t);
+ r.subTo(t, r);
+ while (r[i] < --qd) r.subTo(t, r);
+ }
+ }
+ if (q != null) {
+ r.drShiftTo(ys, q);
+ if (ts != ms) BigInteger.ZERO.subTo(q, q);
+ }
+ r.t = ys;
+ r.clamp();
+ if (nsh > 0) r.rShiftTo(nsh, r); // Denormalize remainder
+ if (ts < 0) BigInteger.ZERO.subTo(r, r);
+ }
+
+// (public) this mod a
+ function bnMod(a) {
+ var r = nbi();
+ this.abs().divRemTo(a, null, r);
+ if (this.s < 0 && r.compareTo(BigInteger.ZERO) > 0) a.subTo(r, r);
+ return r;
+ }
+
+// Modular reduction using "classic" algorithm
+ function Classic(m) {
+ this.m = m;
+ }
+
+ function cConvert(x) {
+ if (x.s < 0 || x.compareTo(this.m) >= 0) return x.mod(this.m);
+ else return x;
+ }
+
+ function cRevert(x) {
+ return x;
+ }
+
+ function cReduce(x) {
+ x.divRemTo(this.m, null, x);
+ }
+
+ function cMulTo(x, y, r) {
+ x.multiplyTo(y, r);
+ this.reduce(r);
+ }
+
+ function cSqrTo(x, r) {
+ x.squareTo(r);
+ this.reduce(r);
+ }
+
+ Classic.prototype.convert = cConvert;
+ Classic.prototype.revert = cRevert;
+ Classic.prototype.reduce = cReduce;
+ Classic.prototype.mulTo = cMulTo;
+ Classic.prototype.sqrTo = cSqrTo;
+
+// (protected) return "-1/this % 2^DB"; useful for Mont. reduction
+// justification:
+// xy == 1 (mod m)
+// xy = 1+km
+// xy(2-xy) = (1+km)(1-km)
+// x[y(2-xy)] = 1-k^2m^2
+// x[y(2-xy)] == 1 (mod m^2)
+// if y is 1/x mod m, then y(2-xy) is 1/x mod m^2
+// should reduce x and y(2-xy) by m^2 at each step to keep size bounded.
+// JS multiply "overflows" differently from C/C++, so care is needed here.
+ function bnpInvDigit() {
+ if (this.t < 1) return 0;
+ var x = this[0];
+ if ((x & 1) == 0) return 0;
+ var y = x & 3; // y == 1/x mod 2^2
+ y = (y * (2 - (x & 0xf) * y)) & 0xf; // y == 1/x mod 2^4
+ y = (y * (2 - (x & 0xff) * y)) & 0xff; // y == 1/x mod 2^8
+ y = (y * (2 - (((x & 0xffff) * y) & 0xffff))) & 0xffff; // y == 1/x mod 2^16
+ // last step - calculate inverse mod DV directly;
+ // assumes 16 < DB <= 32 and assumes ability to handle 48-bit ints
+ y = (y * (2 - x * y % this.DV)) % this.DV; // y == 1/x mod 2^dbits
+ // we really want the negative inverse, and -DV < y < DV
+ return (y > 0) ? this.DV - y : -y;
+ }
+
+// Montgomery reduction
+ function Montgomery(m) {
+ this.m = m;
+ this.mp = m.invDigit();
+ this.mpl = this.mp & 0x7fff;
+ this.mph = this.mp >> 15;
+ this.um = (1 << (m.DB - 15)) - 1;
+ this.mt2 = 2 * m.t;
+ }
+
+// xR mod m
+ function montConvert(x) {
+ var r = nbi();
+ x.abs().dlShiftTo(this.m.t, r);
+ r.divRemTo(this.m, null, r);
+ if (x.s < 0 && r.compareTo(BigInteger.ZERO) > 0) this.m.subTo(r, r);
+ return r;
+ }
+
+// x/R mod m
+ function montRevert(x) {
+ var r = nbi();
+ x.copyTo(r);
+ this.reduce(r);
+ return r;
+ }
+
+// x = x/R mod m (HAC 14.32)
+ function montReduce(x) {
+ while (x.t <= this.mt2) // pad x so am has enough room later
+ x[x.t++] = 0;
+ for (var i = 0; i < this.m.t; ++i) {
+ // faster way of calculating u0 = x[i]*mp mod DV
+ var j = x[i] & 0x7fff;
+ var u0 = (j * this.mpl + (((j * this.mph + (x[i] >> 15) * this.mpl) & this.um) << 15)) & x.DM;
+ // use am to combine the multiply-shift-add into one call
+ j = i + this.m.t;
+ x[j] += this.m.am(0, u0, x, i, 0, this.m.t);
+ // propagate carry
+ while (x[j] >= x.DV) {
+ x[j] -= x.DV;
+ x[++j]++;
+ }
+ }
+ x.clamp();
+ x.drShiftTo(this.m.t, x);
+ if (x.compareTo(this.m) >= 0) x.subTo(this.m, x);
+ }
+
+// r = "x^2/R mod m"; x != r
+ function montSqrTo(x, r) {
+ x.squareTo(r);
+ this.reduce(r);
+ }
+
+// r = "xy/R mod m"; x,y != r
+ function montMulTo(x, y, r) {
+ x.multiplyTo(y, r);
+ this.reduce(r);
+ }
+
+ Montgomery.prototype.convert = montConvert;
+ Montgomery.prototype.revert = montRevert;
+ Montgomery.prototype.reduce = montReduce;
+ Montgomery.prototype.mulTo = montMulTo;
+ Montgomery.prototype.sqrTo = montSqrTo;
+
+// (protected) true iff this is even
+ function bnpIsEven() {
+ return ((this.t > 0) ? (this[0] & 1) : this.s) == 0;
+ }
+
+// (protected) this^e, e < 2^32, doing sqr and mul with "r" (HAC 14.79)
+ function bnpExp(e, z) {
+ if (e > 0xffffffff || e < 1) return BigInteger.ONE;
+ var r = nbi(), r2 = nbi(), g = z.convert(this), i = nbits(e) - 1;
+ g.copyTo(r);
+ while (--i >= 0) {
+ z.sqrTo(r, r2);
+ if ((e & (1 << i)) > 0) z.mulTo(r2, g, r);
+ else {
+ var t = r;
+ r = r2;
+ r2 = t;
+ }
+ }
+ return z.revert(r);
+ }
+
+// (public) this^e % m, 0 <= e < 2^32
+ function bnModPowInt(e, m) {
+ var z;
+ if (e < 256 || m.isEven()) z = new Classic(m); else z = new Montgomery(m);
+ return this.exp(e, z);
+ }
+
+// protected
+ BigInteger.prototype.copyTo = bnpCopyTo;
+ BigInteger.prototype.fromInt = bnpFromInt;
+ BigInteger.prototype.fromString = bnpFromString;
+ BigInteger.prototype.clamp = bnpClamp;
+ BigInteger.prototype.dlShiftTo = bnpDLShiftTo;
+ BigInteger.prototype.drShiftTo = bnpDRShiftTo;
+ BigInteger.prototype.lShiftTo = bnpLShiftTo;
+ BigInteger.prototype.rShiftTo = bnpRShiftTo;
+ BigInteger.prototype.subTo = bnpSubTo;
+ BigInteger.prototype.multiplyTo = bnpMultiplyTo;
+ BigInteger.prototype.squareTo = bnpSquareTo;
+ BigInteger.prototype.divRemTo = bnpDivRemTo;
+ BigInteger.prototype.invDigit = bnpInvDigit;
+ BigInteger.prototype.isEven = bnpIsEven;
+ BigInteger.prototype.exp = bnpExp;
+
+// public
+ BigInteger.prototype.toString = bnToString;
+ BigInteger.prototype.negate = bnNegate;
+ BigInteger.prototype.abs = bnAbs;
+ BigInteger.prototype.compareTo = bnCompareTo;
+ BigInteger.prototype.bitLength = bnBitLength;
+ BigInteger.prototype.mod = bnMod;
+ BigInteger.prototype.modPowInt = bnModPowInt;
+
+// "constants"
+ BigInteger.ZERO = nbv(0);
+ BigInteger.ONE = nbv(1);
+
+// Copyright (c) 2005-2009 Tom Wu
+// All Rights Reserved.
+// See "LICENSE" for details.
+
+// Extended JavaScript BN functions, required for RSA private ops.
+
+// Version 1.1: new BigInteger("0", 10) returns "proper" zero
+// Version 1.2: square() API, isProbablePrime fix
+
+// (public)
+ function bnClone() {
+ var r = nbi();
+ this.copyTo(r);
+ return r;
+ }
+
+// (public) return value as integer
+ function bnIntValue() {
+ if (this.s < 0) {
+ if (this.t == 1) return this[0] - this.DV;
+ else if (this.t == 0) return -1;
+ } else if (this.t == 1) return this[0];
+ else if (this.t == 0) return 0;
+ // assumes 16 < DB < 32
+ return ((this[1] & ((1 << (32 - this.DB)) - 1)) << this.DB) | this[0];
+ }
+
+// (public) return value as byte
+ function bnByteValue() {
+ return (this.t == 0) ? this.s : (this[0] << 24) >> 24;
+ }
+
+// (public) return value as short (assumes DB>=16)
+ function bnShortValue() {
+ return (this.t == 0) ? this.s : (this[0] << 16) >> 16;
+ }
+
+// (protected) return x s.t. r^x < DV
+ function bnpChunkSize(r) {
+ return Math.floor(Math.LN2 * this.DB / Math.log(r));
+ }
+
+// (public) 0 if this == 0, 1 if this > 0
+ function bnSigNum() {
+ if (this.s < 0) return -1;
+ else if (this.t <= 0 || (this.t == 1 && this[0] <= 0)) return 0;
+ else return 1;
+ }
+
+// (protected) convert to radix string
+ function bnpToRadix(b) {
+ if (b == null) b = 10;
+ if (this.signum() == 0 || b < 2 || b > 36) return "0";
+ var cs = this.chunkSize(b);
+ var a = Math.pow(b, cs);
+ var d = nbv(a), y = nbi(), z = nbi(), r = "";
+ this.divRemTo(d, y, z);
+ while (y.signum() > 0) {
+ r = (a + z.intValue()).toString(b).substr(1) + r;
+ y.divRemTo(d, y, z);
+ }
+ return z.intValue().toString(b) + r;
+ }
+
+// (protected) convert from radix string
+ function bnpFromRadix(s, b) {
+ this.fromInt(0);
+ if (b == null) b = 10;
+ var cs = this.chunkSize(b);
+ var d = Math.pow(b, cs), mi = false, j = 0, w = 0;
+ for (var i = 0; i < s.length; ++i) {
+ var x = intAt(s, i);
+ if (x < 0) {
+ if (s.charAt(i) == "-" && this.signum() == 0) mi = true;
+ continue;
+ }
+ w = b * w + x;
+ if (++j >= cs) {
+ this.dMultiply(d);
+ this.dAddOffset(w, 0);
+ j = 0;
+ w = 0;
+ }
+ }
+ if (j > 0) {
+ this.dMultiply(Math.pow(b, j));
+ this.dAddOffset(w, 0);
+ }
+ if (mi) BigInteger.ZERO.subTo(this, this);
+ }
+
+// (protected) alternate constructor
+ function bnpFromNumber(a, b, c) {
+ if ("number" == typeof b) {
+ // new BigInteger(int,int,RNG)
+ if (a < 2) this.fromInt(1);
+ else {
+ this.fromNumber(a, c);
+ if (!this.testBit(a - 1)) // force MSB set
+ this.bitwiseTo(BigInteger.ONE.shiftLeft(a - 1), op_or, this);
+ if (this.isEven()) this.dAddOffset(1, 0); // force odd
+ while (!this.isProbablePrime(b)) {
+ this.dAddOffset(2, 0);
+ if (this.bitLength() > a) this.subTo(BigInteger.ONE.shiftLeft(a - 1), this);
+ }
+ }
+ } else {
+ // new BigInteger(int,RNG)
+ var x = new Array(), t = a & 7;
+ x.length = (a >> 3) + 1;
+ b.nextBytes(x);
+ if (t > 0) x[0] &= ((1 << t) - 1); else x[0] = 0;
+ this.fromString(x, 256);
+ }
+ }
+
+// (public) convert to bigendian byte array
+ function bnToByteArray() {
+ var i = this.t, r = new Array();
+ r[0] = this.s;
+ var p = this.DB - (i * this.DB) % 8, d, k = 0;
+ if (i-- > 0) {
+ if (p < this.DB && (d = this[i] >> p) != (this.s & this.DM) >> p)
+ r[k++] = d | (this.s << (this.DB - p));
+ while (i >= 0) {
+ if (p < 8) {
+ d = (this[i] & ((1 << p) - 1)) << (8 - p);
+ d |= this[--i] >> (p += this.DB - 8);
+ } else {
+ d = (this[i] >> (p -= 8)) & 0xff;
+ if (p <= 0) {
+ p += this.DB;
+ --i;
+ }
+ }
+ if ((d & 0x80) != 0) d |= -256;
+ if (k == 0 && (this.s & 0x80) != (d & 0x80)) ++k;
+ if (k > 0 || d != this.s) r[k++] = d;
+ }
+ }
+ return r;
+ }
+
+ function bnEquals(a) {
+ return (this.compareTo(a) == 0);
+ }
+
+ function bnMin(a) {
+ return (this.compareTo(a) < 0) ? this : a;
+ }
+
+ function bnMax(a) {
+ return (this.compareTo(a) > 0) ? this : a;
+ }
+
+// (protected) r = this op a (bitwise)
+ function bnpBitwiseTo(a, op, r) {
+ var i, f, m = Math.min(a.t, this.t);
+ for (i = 0; i < m; ++i) r[i] = op(this[i], a[i]);
+ if (a.t < this.t) {
+ f = a.s & this.DM;
+ for (i = m; i < this.t; ++i) r[i] = op(this[i], f);
+ r.t = this.t;
+ } else {
+ f = this.s & this.DM;
+ for (i = m; i < a.t; ++i) r[i] = op(f, a[i]);
+ r.t = a.t;
+ }
+ r.s = op(this.s, a.s);
+ r.clamp();
+ }
+
+// (public) this & a
+ function op_and(x, y) {
+ return x & y;
+ }
+
+ function bnAnd(a) {
+ var r = nbi();
+ this.bitwiseTo(a, op_and, r);
+ return r;
+ }
+
+// (public) this | a
+ function op_or(x, y) {
+ return x | y;
+ }
+
+ function bnOr(a) {
+ var r = nbi();
+ this.bitwiseTo(a, op_or, r);
+ return r;
+ }
+
+// (public) this ^ a
+ function op_xor(x, y) {
+ return x ^ y;
+ }
+
+ function bnXor(a) {
+ var r = nbi();
+ this.bitwiseTo(a, op_xor, r);
+ return r;
+ }
+
+// (public) this & ~a
+ function op_andnot(x, y) {
+ return x & ~y;
+ }
+
+ function bnAndNot(a) {
+ var r = nbi();
+ this.bitwiseTo(a, op_andnot, r);
+ return r;
+ }
+
+// (public) ~this
+ function bnNot() {
+ var r = nbi();
+ for (var i = 0; i < this.t; ++i) r[i] = this.DM & ~this[i];
+ r.t = this.t;
+ r.s = ~this.s;
+ return r;
+ }
+
+// (public) this << n
+ function bnShiftLeft(n) {
+ var r = nbi();
+ if (n < 0) this.rShiftTo(-n, r); else this.lShiftTo(n, r);
+ return r;
+ }
+
+// (public) this >> n
+ function bnShiftRight(n) {
+ var r = nbi();
+ if (n < 0) this.lShiftTo(-n, r); else this.rShiftTo(n, r);
+ return r;
+ }
+
+// return index of lowest 1-bit in x, x < 2^31
+ function lbit(x) {
+ if (x == 0) return -1;
+ var r = 0;
+ if ((x & 0xffff) == 0) {
+ x >>= 16;
+ r += 16;
+ }
+ if ((x & 0xff) == 0) {
+ x >>= 8;
+ r += 8;
+ }
+ if ((x & 0xf) == 0) {
+ x >>= 4;
+ r += 4;
+ }
+ if ((x & 3) == 0) {
+ x >>= 2;
+ r += 2;
+ }
+ if ((x & 1) == 0) ++r;
+ return r;
+ }
+
+// (public) returns index of lowest 1-bit (or -1 if none)
+ function bnGetLowestSetBit() {
+ for (var i = 0; i < this.t; ++i)
+ if (this[i] != 0) return i * this.DB + lbit(this[i]);
+ if (this.s < 0) return this.t * this.DB;
+ return -1;
+ }
+
+// return number of 1 bits in x
+ function cbit(x) {
+ var r = 0;
+ while (x != 0) {
+ x &= x - 1;
+ ++r;
+ }
+ return r;
+ }
+
+// (public) return number of set bits
+ function bnBitCount() {
+ var r = 0, x = this.s & this.DM;
+ for (var i = 0; i < this.t; ++i) r += cbit(this[i] ^ x);
+ return r;
+ }
+
+// (public) true iff nth bit is set
+ function bnTestBit(n) {
+ var j = Math.floor(n / this.DB);
+ if (j >= this.t) return (this.s != 0);
+ return ((this[j] & (1 << (n % this.DB))) != 0);
+ }
+
+// (protected) this op (1<
+ *
+ *
+ * This is ITU-T X.690 ASN.1 DER encoder class library and + * class structure and methods is very similar to + * org.bouncycastle.asn1 package of + * well known BouncyCaslte Cryptography Library. + * + *