# Transformation
Transformation attributes allow you to rotate, translate, scale, or transform a component.
> **NOTE**
>
> The APIs of this module are supported since API version 7. Updates will be marked with a superscript to indicate their earliest API version.
## Attributes
| Name | Type | Description |
| --------- | ------------------------------------------------------------ | ------------------------------------------------------------ |
| rotate | {
x?: number,
y?: number,
z?: number,
angle?: number \| string,
centerX?: number \| string,
centerY?: number \| string
} | Rotation axis. A positive angle indicates a clockwise rotation, and a negative angle indicates a counterclockwise rotation. The default value is **0**. **centerX** and **centerY** are used to set the rotation center point.
Default value:
{
x: 0,
y: 0,
z: 0,
angle: 0,
centerX: '50%',
centerY: '50%'
} |
| translate | {
x?: number \| string,
y?: number \| string,
z? : number \| string
} | Translation distance along the x-, y-, and z-axis. The translation direction is determined by the positive and negative values. The value cannot be a percentage.
Default value:
{
x: 0,
y: 0,
z: 0
} |
| scale | {
x?: number,
y?: number,
z?: number,
centerX?: number \| string,
centerY?: number \| string
} | Scale ratio along the x-, y-, and z-axis. The default value is **1**. **centerX** and **centerY** are used to set the scale center point.
Default value:
{
x: 1,
y: 1,
z: 1,
centerX:'50%',
centerY:'50%'
} |
| transform | Matrix4Transit | Transformation matrix of the component. |
## Example
```ts
// xxx.ets
import matrix4 from '@ohos.matrix4'
@Entry
@Component
struct TransformExample {
build() {
Column() {
Text('rotate').width('90%').fontColor(0xCCCCCC).padding(15).fontSize(14)
Row()
.rotate({
x: 1,
y: 1,
z: 1,
centerX: '50%',
centerY: '50%',
angle: 300
}) // The component rotates around the center point of the rotation axis (1,1,1) clockwise by 300 degrees.
.width(100).height(100).backgroundColor(0xAFEEEE)
Text('translate').width('90%').fontColor(0xCCCCCC).padding(10).fontSize(14)
Row()
.translate({ x: 100, y: 10 }) // The component translates by 100 along the x-axis and by 10 along the y-axis.
.width(100).height(100).backgroundColor(0xAFEEEE).margin({ bottom: 10 })
Text('scale').width('90%').fontColor(0xCCCCCC).padding(15).fontSize(14)
Row()
.scale({ x: 2, y: 0.5}) // The height and width are doubled. The z-axis has no effect in 2D mode.
.width(100).height(100).backgroundColor(0xAFEEEE)
Text('Matrix4').width('90%').fontColor(0xCCCCCC).padding(15).fontSize(14)
Row()
.width(100).height(100).backgroundColor(0xAFEEEE)
.transform(matrix4.identity().translate({ x: 50, y: 50 }).scale({ x: 1.5, y: 1 }).rotate({
x: 0,
y: 0,
z: 1,
angle: 60
}))
}.width('100%').margin({ top: 5 })
}
}
```
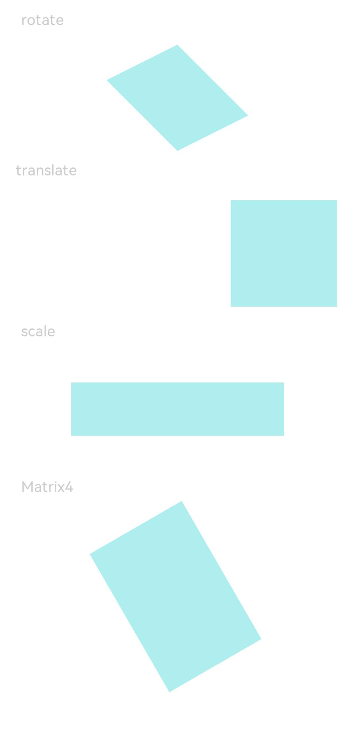