# <tabs> Development
The <tabs> component is a common UI component for navigation. It allows quick access to different functions of an app. For details, see [tabs](../reference/arkui-js/js-components-container-tabs.md).
## Creating Tabs
Create a <tabs> component in the .hml file under pages/index.
```
item1
item2
content1
content2
```
```
/* xxx.css */
.container {
flex-direction: column;
justify-content: center;
align-items: center;
background-color: #F1F3F5;
}
.text{
width: 100%;
height: 100%;
justify-content: center;
align-items: center;
}
```
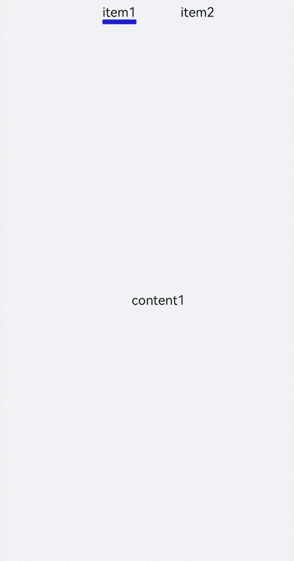
## Setting the Tabs Orientation
By default, the active tab of a <tabs> component is the one with the specified index. To show the <tabs> vertically, set the vertical attribute to true.
```
```
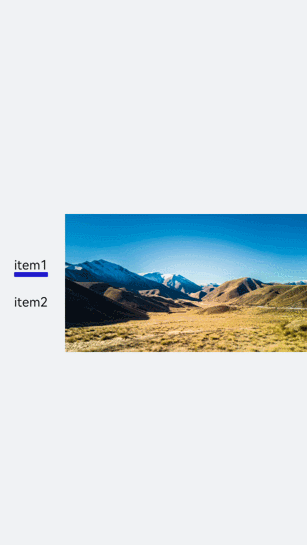
Set the mode attribute to enable the child components of the to be evenly distributed. Set the scrollable attribute to disable scrolling of the .
```
```
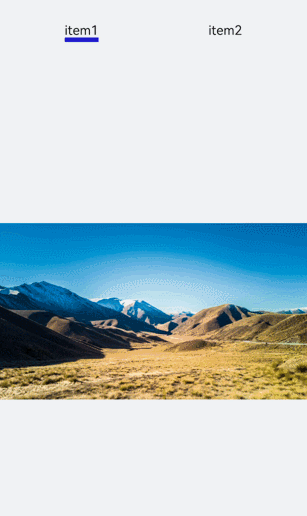
## Setting the Style
Set the background color, border, and tab-content layout of the <tabs> component.
```
item1
item2
content1
content2
```
```
/* xxx.css */
.container {
flex-direction: column;
justify-content: flex-start;
align-items: center;
background-color:#F1F3F5;
}
.tabs{
margin-top: 20px;
border: 1px solid #2262ef;
width: 99%;
padding: 10px;
}
.tabBar{
width: 100%;
border: 1px solid #78abec;
}
.tabContent{
width: 100%;
margin-top: 10px;
height: 300px;
color: blue;
justify-content: center; align-items: center;
}
```

## Displaying the Tab Index
Add the change event for the <tabs> component to display the index of the current tab after tab switching.
```
```
```
/* index.js */
import prompt from '@system.prompt';
export default {
tabChange(e){
prompt.showToast({
message: "Tab index: " + e.index
})
}
}
```
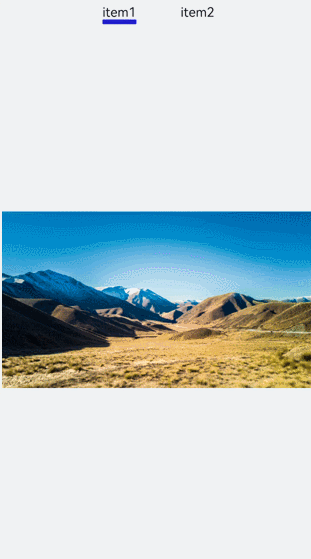
>  **NOTE**:
> - A <tabs> can wrap at most one [](../reference/arkui-js/js-components-container-tab-bar.md) and at most one [](../reference/arkui-js/js-components-container-tab-content.md).
## Example Scenario
In this example, you can switch between tabs and the active tab has the title text in red with an underline below.
Use the <tabs>, , and components to implement tab switching. Then define the arrays and attributes. Add the change event to change the attribute values in the arrays so that the active tab has a different font color and an underline.
```
```
```
/* xxx.css */
.container{
background-color:#F1F3F5;
}
.tab_bar {
width: 100%;
}
.tab_item {
flex-direction: column;
align-items: center;
}
.tab_item text {
font-size: 32px;
}
.item-container {
justify-content: center;
flex-direction: column;
}
.underline-show {
height: 2px;
width: 160px;
background-color: #FF4500;
margin-top: 7.5px;
}
.underline-hide {
height: 2px;
margin-top: 7.5px;
width: 160px;
}
```
```
/* index.js */
import prompt from '@system.prompt';
export default {
data() {
return {
datas: {
color_normal: '#878787',
color_active: '#ff4500',
show: true,
list: [{
i: 0,
color: '#ff4500',
show: true,
title: 'List1'
}, {
i: 1,
color: '#878787',
show: false,
title: 'List2'
}, {
i: 2,
color: '#878787',
show: false,
title: 'List3'
}]
}
}
},
changeTabactive (e) {
for (let i = 0; i < this.datas.list.length; i++) {
let element = this.datas.list[i];
element.show = false;
element.color = this.datas.color_normal;
if (i === e.index) {
element.show = true;
element.color = this.datas.color_active;
}
}
}
}
```
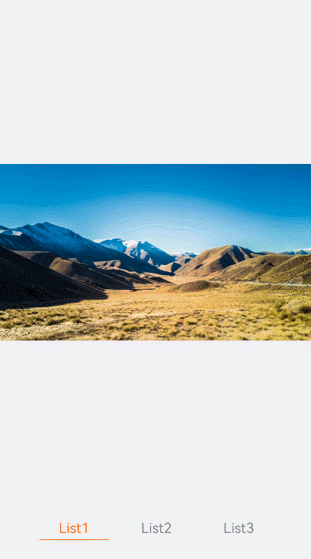