# <rating> Development
The **<rating>** component provides a rating bar used for reviews and ratings. For details, see [rating](../reference/arkui-js/js-components-basic-rating.md).
## Creating a <rating> Component
Create a **<rating>** component in the .hml file under **pages/index**.
```
```
```
/* xxx.css */
.container {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: #F1F3F5;
}
rating {
width: 80%;
height: 150px;
}
```
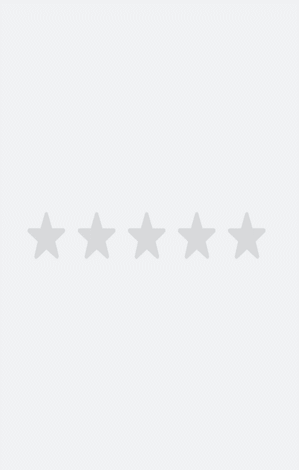
## Setting the Rating Level
Use the **<rating>** component to set the number of stars in a rating bar and the current rating using the **numstars** and **rating** attributes, respectively.
```
```
```
/* xxx.css */
.container {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: #F1F3F5;
}
rating {
width: 80%;
height: 150px;
}
```
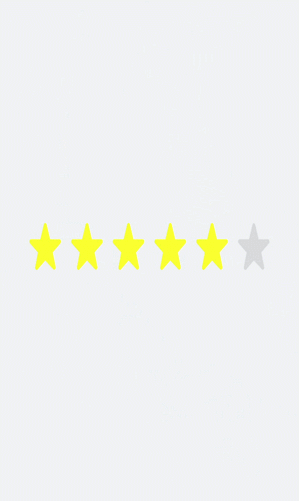
## Setting the Rating Style
Use the **<rating>** component to set the background images when a rating star is unselected, selected, and partially selected using the **star-background**, **star-foreground**, and **star-secondary** attributes, respectively.
```
```
```
/* xxx.css */
.container {
width: 100%;
height: 100%;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #F1F3F5;
}
```
```
/* index.js */
export default {
data: {
backstar: 'common/love.png',
secstar: 'common/love.png',
forestar: 'common/love1.png',
ratewidth: '400px',
rateheight: '150px'
},
onInit(){
}
}
```
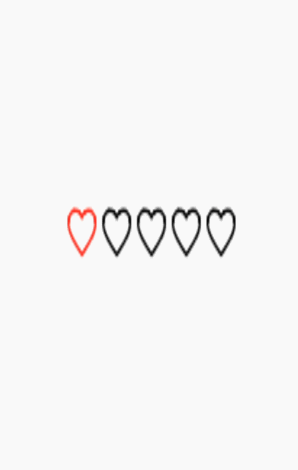
>  **NOTE:**
> - You must set **star-background**, **star-secondary**, and **star-foreground**. Otherwise, the grey rating star applies, indicating that the image source is incorrectly set.
>
> - The **star-background**, **star-secondary**, and **star-foreground** attributes support only PNG and JPG images in the local path.
## Binding Events
Add the **change** event to the <rating> component to print the current rating.
```
```
```
.container {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: #F1F3F5;
}
rating {
width: 80%;
height: 150px;
}
```
```
import prompt from '@system.prompt';
export default {
showrating(e) {
prompt.showToast({
message:'Current Rating' + e.rating
})
}
}
```
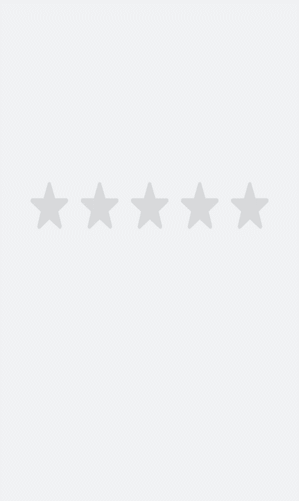
## Example Scenario
Change the switch status to toggle between the star background images and drag the slider to adjust the rating values.
```
Replacing a custom image
numstars {{stars}}
rating {{rate}}
```
```
/* xxx.css */
.myrating:active {
width: 500px;
height: 100px;
}
switch{
font-size: 40px;
}
```
```
/* index.js */
import prompt from '@system.prompt';
export default {
data: {
backstar: '',
secstar: '',
forestar: '',
stars: 5,
ratewidth: '300px',
rateheight: '60px',
step: 0.5,
rate: 0
},
onInit(){
},
setstar(e) {
if (e.checked == true) {
this.backstar = 'common/love.png'
this.secstar = 'common/love.png'
this.forestar = 'common/love1.png'
} else {
this.backstar = ''
this.secstar = ''
this.forestar = ''
}
},
setnumstars(e) {
this.stars = e.progress
this.ratewidth = 60 * parseInt(this.stars) + 'px'
},
setstep(e) {
this.step = e.progress
},
setrating(e){
this.rate = e.progress
},
showrating(e) {
prompt.showToast({
message:'Current Rating' + e.rating
})
}
}
```
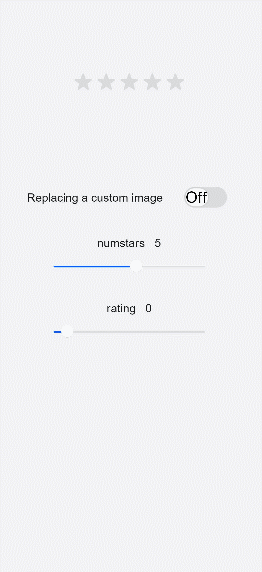