Merge branch 'master' of gitee.com:openharmony/docs into master
Signed-off-by: Nlvqiang214 <lvqiang1@huawei.com>
Showing
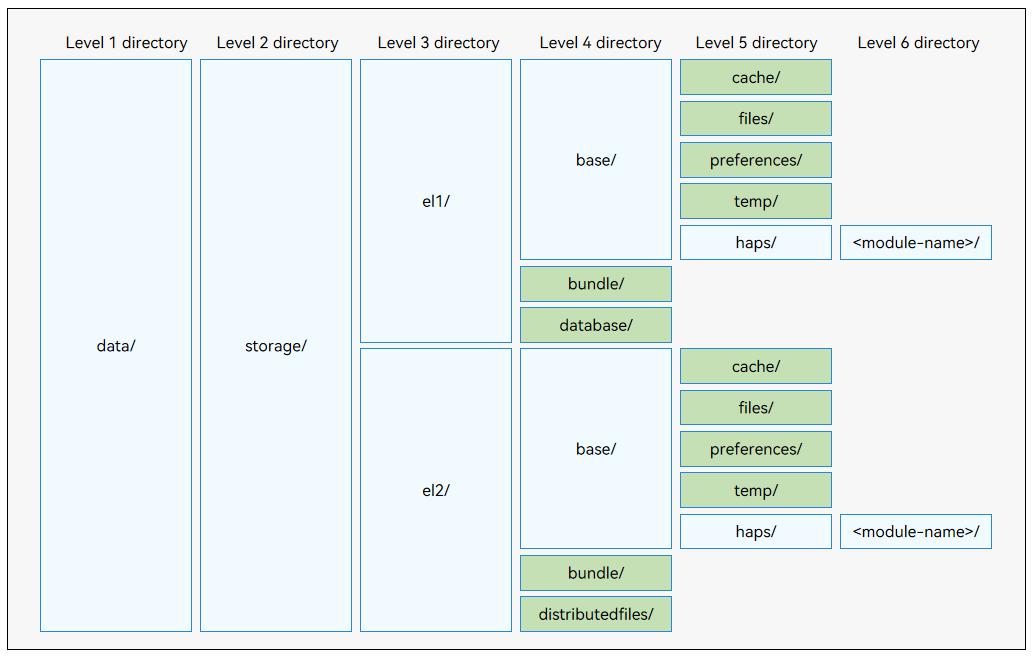
| W: | H:
| W: | H:
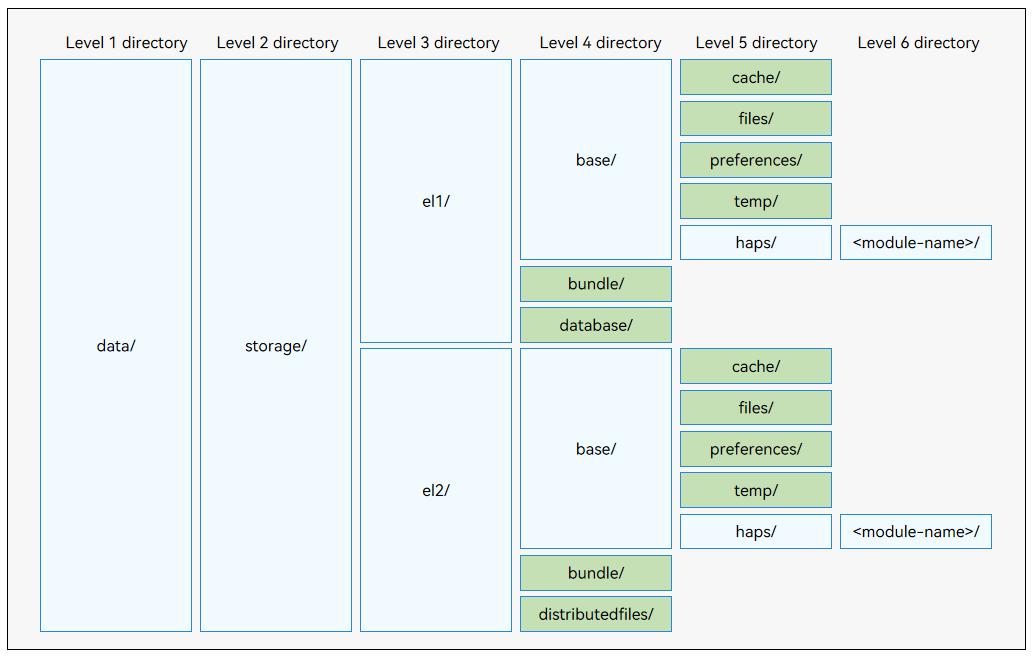
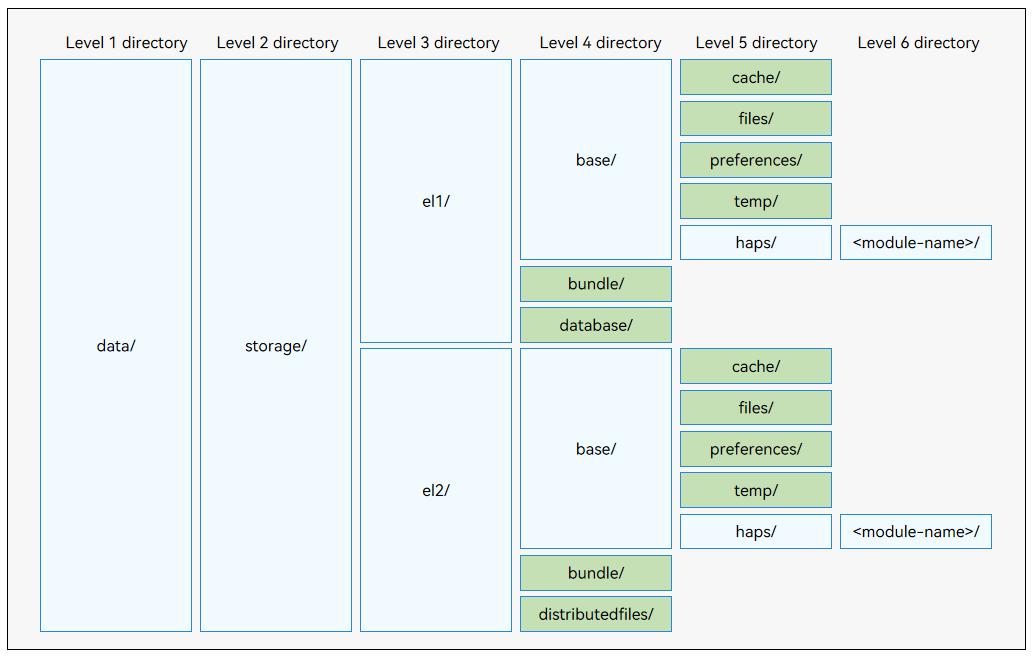
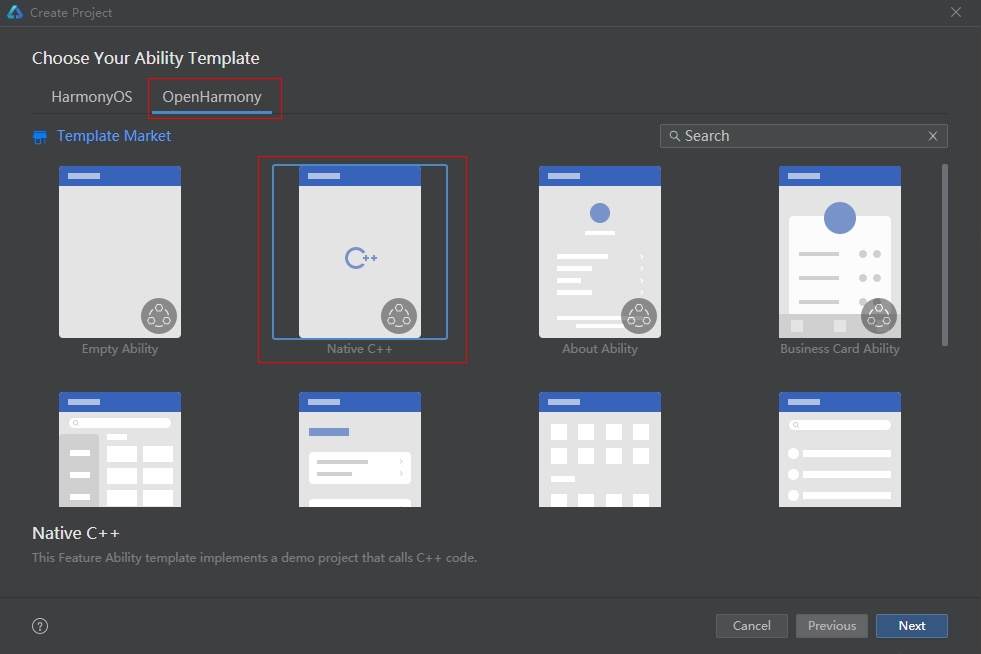
| W: | H:
| W: | H:
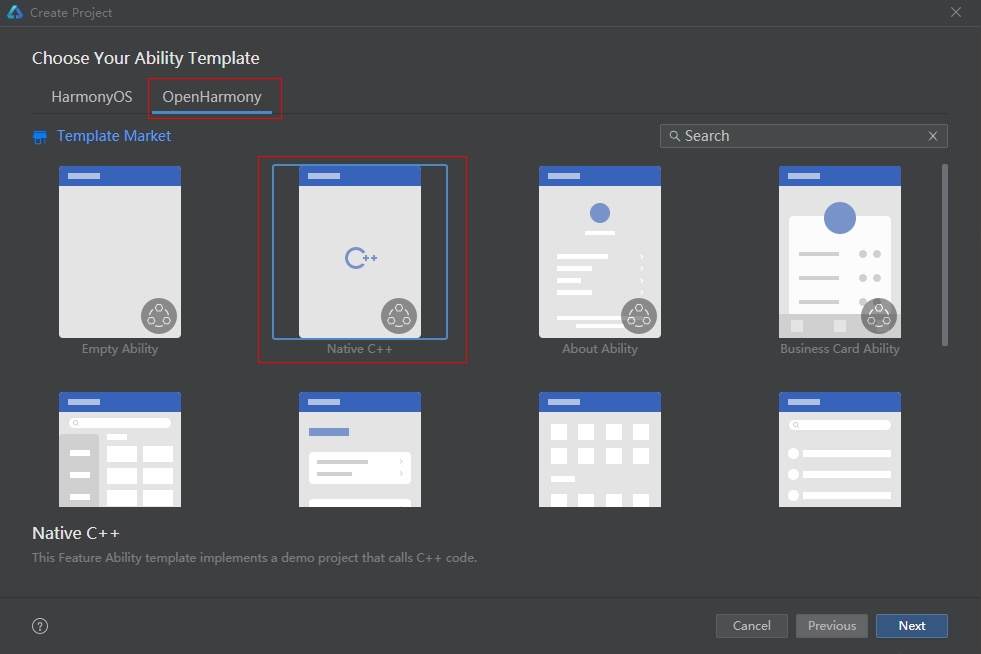
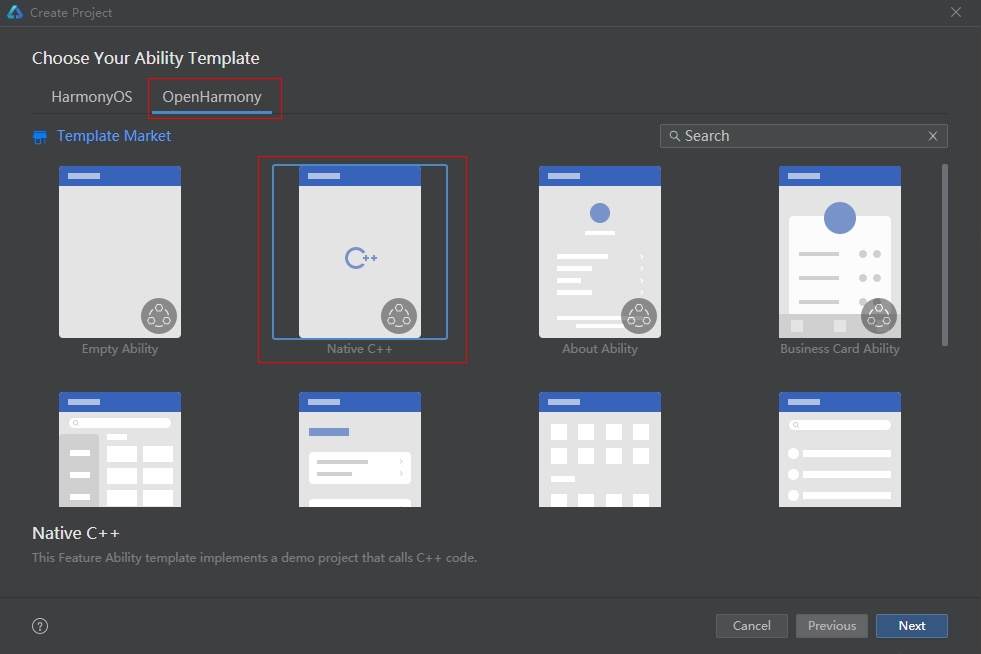
此差异已折叠。
89.0 KB
39.5 KB
43.6 KB
38.9 KB
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。
此差异已折叠。