Merge branch '34102-online-view-of-artifacts-fe' into 'master'
Add external link for online artifacts Closes #34102 See merge request gitlab-org/gitlab-ce!14399
Showing
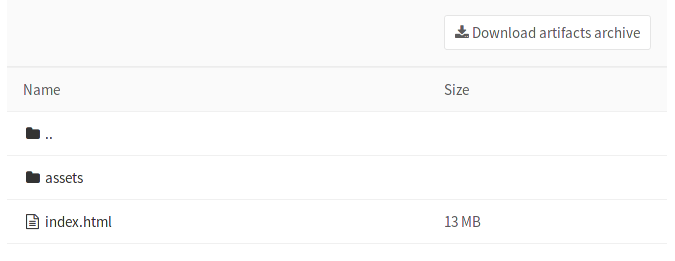
| W: | H:
| W: | H:
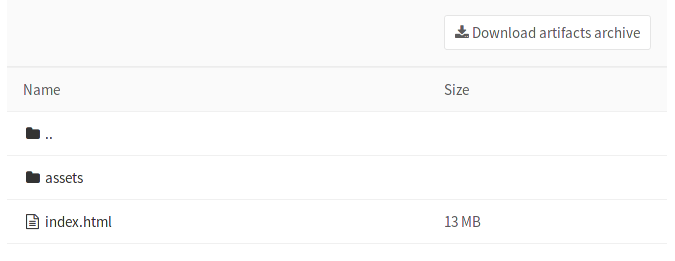
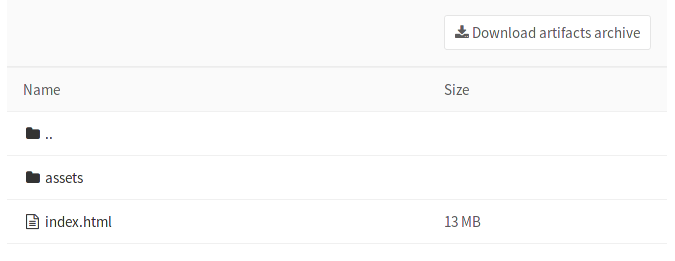
Add external link for online artifacts Closes #34102 See merge request gitlab-org/gitlab-ce!14399
3.7 KB | W: | H:
3.9 KB | W: | H: