# 单词搜索
给定一个 m x n
二维字符网格 board
和一个字符串单词 word
。如果 word
存在于网格中,返回 true
;否则,返回 false
。
单词必须按照字母顺序,通过相邻的单元格内的字母构成,其中“相邻”单元格是那些水平相邻或垂直相邻的单元格。同一个单元格内的字母不允许被重复使用。
示例 1:
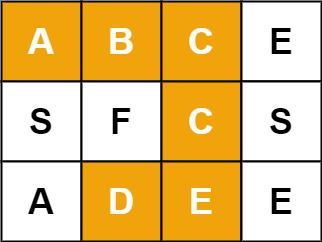
输入:board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED"
输出:true
示例 2:
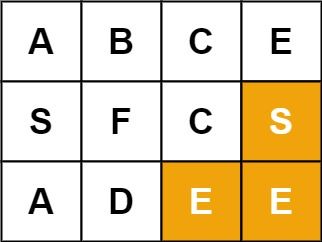
输入:board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "SEE"
输出:true
示例 3:
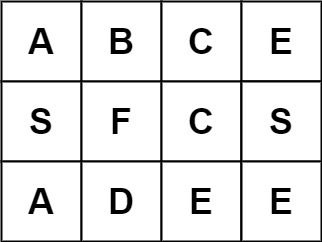
输入:board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCB"
输出:false
提示:
m == board.length
n = board[i].length
1 <= m, n <= 6
1 <= word.length <= 15
board
和 word
仅由大小写英文字母组成
进阶:你可以使用搜索剪枝的技术来优化解决方案,使其在 board
更大的情况下可以更快解决问题?
## template
```python
class Solution(object):
def exist(self, board, word):
"""
:type board: List[List[str]]
:type word: str
:rtype: bool
"""
check_board = [[True] * len(board[0]) for _ in range(len(board))]
for i in range(len(board)):
for j in range(len(board[0])):
if board[i][j] == word[0] and check_board:
check_board[i][j] = False
res = self.check_exist(check_board, board, word, 1, len(word), i, j)
if res:
return True
check_board[i][j] = True
return False
def check_exist(self, check_board, board, word, index, ls, row, col):
if index == ls:
return True
for temp in [(0, 1),(0, -1),(1, 0),(-1, 0)]:
curr_row = row + temp[0]
curr_col = col + temp[1]
if curr_row >= 0 and curr_row < len(board) and curr_col >= 0 and curr_col < len(board[0]):
if check_board[curr_row][curr_col] and board[curr_row][curr_col] == word[index]:
check_board[curr_row][curr_col] = False
res = self.check_exist(check_board, board, word, index + 1, len(word), curr_row, curr_col)
if res:
return res
check_board[curr_row][curr_col] = True
return False
if __name__ == "__main__":
s = Solution()
print (s.exist(board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED"))
```
## 答案
```python
```
## 选项
### A
```python
```
### B
```python
```
### C
```python
```