min;
+ /** initialize your data structure here. */
+ MinStack()
+ {
+ }
+
+ void push(int x)
+ {
+ s.push(x);
+ if (min.empty() || x <= min.top())
+ {
+ min.push(x);
+ }
+ }
+
+ void pop()
+ {
+ if (s.top() == min.top())
+ min.pop();
+ s.pop();
+ }
+
+ int top()
+ {
+ return s.top();
+ }
+ int getMin()
+ {
+ return min.top();
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..8f385b811a74b7879d827a5aa925ff73a609be10
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-2bc75d75d57b4807a2edf0eb18eb5c8c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e4116689d660dd11aa933cb0d75d4aa42fb5c27c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "2450b377ecaa4e0a9dd4f7d682a4af21",
+ "author": "csdn.net",
+ "keywords": "哈希表,链表,双指针",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..818e6a2e20b34f40749e4b8edd5c262a3ec9f1a8
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/114.exercises/solution.md"
@@ -0,0 +1,126 @@
+# 相交链表
+
+给你两个单链表的头节点 headA
和 headB
,请你找出并返回两个单链表相交的起始节点。如果两个链表没有交点,返回 null
。
+
+图示两个链表在节点 c1
开始相交:
+
+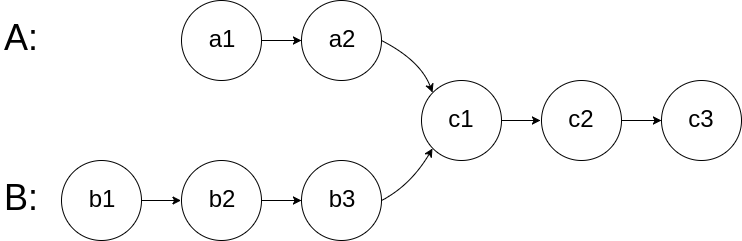
+
+题目数据 保证 整个链式结构中不存在环。
+
+注意,函数返回结果后,链表必须 保持其原始结构 。
+
+
+
+示例 1:
+
+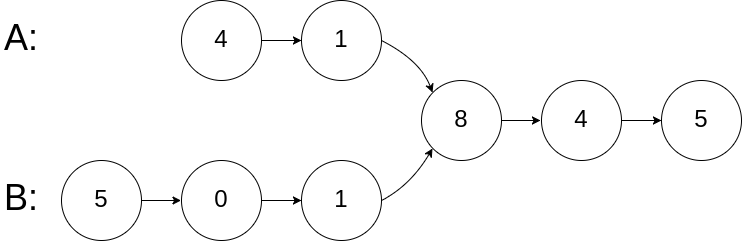
+
+
+输入:intersectVal = 8, listA = [4,1,8,4,5], listB = [5,0,1,8,4,5], skipA = 2, skipB = 3
+输出:Intersected at '8'
+解释:相交节点的值为 8 (注意,如果两个链表相交则不能为 0)。
+从各自的表头开始算起,链表 A 为 [4,1,8,4,5],链表 B 为 [5,0,1,8,4,5]。
+在 A 中,相交节点前有 2 个节点;在 B 中,相交节点前有 3 个节点。
+
+
+示例 2:
+
+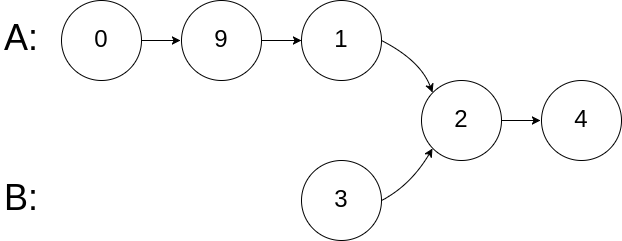
+
+
+输入:intersectVal = 2, listA = [0,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1
+输出:Intersected at '2'
+解释:相交节点的值为 2 (注意,如果两个链表相交则不能为 0)。
+从各自的表头开始算起,链表 A 为 [0,9,1,2,4],链表 B 为 [3,2,4]。
+在 A 中,相交节点前有 3 个节点;在 B 中,相交节点前有 1 个节点。
+
+
+示例 3:
+
+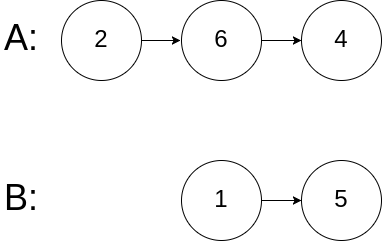
+
+
+输入:intersectVal = 0, listA = [2,6,4], listB = [1,5], skipA = 3, skipB = 2
+输出:null
+解释:从各自的表头开始算起,链表 A 为 [2,6,4],链表 B 为 [1,5]。
+由于这两个链表不相交,所以 intersectVal 必须为 0,而 skipA 和 skipB 可以是任意值。
+这两个链表不相交,因此返回 null 。
+
+
+
+
+提示:
+
+
+ listA
中节点数目为 m
+ listB
中节点数目为 n
+ 0 <= m, n <= 3 * 104
+ 1 <= Node.val <= 105
+ 0 <= skipA <= m
+ 0 <= skipB <= n
+ - 如果
listA
和 listB
没有交点,intersectVal
为 0
+ - 如果
listA
和 listB
有交点,intersectVal == listA[skipA + 1] == listB[skipB + 1]
+
+
+
+
+进阶:你能否设计一个时间复杂度 O(n)
、仅用 O(1)
内存的解决方案?
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ ListNode *getIntersectionNode(ListNode *headA, ListNode *headB)
+ {
+
+ if (!headA || !headB)
+ {
+ return NULL;
+ }
+
+ ListNode *cur1 = headA;
+ ListNode *cur2 = headB;
+ while (cur1 != cur2)
+ {
+ cur1 = cur1 ? cur1->next : headB;
+ cur2 = cur2 ? cur2->next : headA;
+ }
+ return cur1;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..632dc84bad20079eae73cd6940ce2455ef58b942
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-68803d4d5df0420eabfef657c0df9560",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..97e92c9025845439fb9e64c6a1069aab6a354f57
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "e15e9d7a6a034088b2384779c02275af",
+ "author": "csdn.net",
+ "keywords": "数组,双指针,二分查找",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..38ace9cf84394b3d58c7ef77c3fb10b6f30909ca
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/115.exercises/solution.md"
@@ -0,0 +1,103 @@
+# 两数之和 II
+
+给定一个已按照 非递减顺序排列 的整数数组 numbers
,请你从数组中找出两个数满足相加之和等于目标数 target
。
+
+函数应该以长度为 2
的整数数组的形式返回这两个数的下标值。numbers
的下标 从 1 开始计数 ,所以答案数组应当满足 1 <= answer[0] < answer[1] <= numbers.length
。
+
+你可以假设每个输入 只对应唯一的答案 ,而且你 不可以 重复使用相同的元素。
+
+
+示例 1:
+
+
+输入:numbers = [2,7,11,15], target = 9
+输出:[1,2]
+解释:2 与 7 之和等于目标数 9 。因此 index1 = 1, index2 = 2 。
+
+
+示例 2:
+
+
+输入:numbers = [2,3,4], target = 6
+输出:[1,3]
+
+
+示例 3:
+
+
+输入:numbers = [-1,0], target = -1
+输出:[1,2]
+
+
+
+
+提示:
+
+
+ 2 <= numbers.length <= 3 * 104
+ -1000 <= numbers[i] <= 1000
+ numbers
按 非递减顺序 排列
+ -1000 <= target <= 1000
+ - 仅存在一个有效答案
+
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ vector twoSum(vector &numbers, int target)
+ {
+ int low = 0, high = numbers.size() - 1;
+ while (low < high)
+ {
+ int sum = numbers[low] + numbers[high];
+ if (sum == target)
+ {
+ return {low + 1, high + 1};
+ }
+ if (sum < target)
+ {
+ ++low;
+ }
+ else
+ {
+ --high;
+ }
+ }
+ return {-1, -1};
+ }
+}
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..94e61fcabd31714a033158b8937208ca5e29652e
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-8337bc578dd54305bcbcf6445d06af30",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..746bb1754ee7c729c618497c0e07bd2afe6ebfd3
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "787031c1bf0b4f81b66999b3f4b42146",
+ "author": "csdn.net",
+ "keywords": "数学,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..6e6ad1ee43c310dbcce58ba992ef482f8933f6ec
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/116.exercises/solution.md"
@@ -0,0 +1,112 @@
+# Excel表列名称
+
+给你一个整数 columnNumber
,返回它在 Excel 表中相对应的列名称。
+
+例如:
+
+
+A -> 1
+B -> 2
+C -> 3
+...
+Z -> 26
+AA -> 27
+AB -> 28
+...
+
+
+
+
+示例 1:
+
+
+输入:columnNumber = 1
+输出:"A"
+
+
+示例 2:
+
+
+输入:columnNumber = 28
+输出:"AB"
+
+
+示例 3:
+
+
+输入:columnNumber = 701
+输出:"ZY"
+
+
+示例 4:
+
+
+输入:columnNumber = 2147483647
+输出:"FXSHRXW"
+
+
+
+
+提示:
+
+
+ 1 <= columnNumber <= 231 - 1
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ string convertToTitle(int n)
+ {
+ string res;
+ while (n)
+ {
+ int temp = n % 26;
+ n /= 26;
+ if (temp)
+ res.push_back('A' + temp - 1);
+ else
+ {
+ res.push_back('Z');
+ n--;
+ }
+ }
+ reverse(res.begin(), res.end());
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..cd9d27729469638e75e8af79f111831c8c01cddd
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-962b9124033e41d49aa3481c7dfab494",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..87e54c15dc3a4adc3d529bfa517a481105c73d13
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "3063239bef37448aaddac259ac4b4649",
+ "author": "csdn.net",
+ "keywords": "数组,哈希表,分治,计数,排序",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..fb2b1813d5fbaa74d161ca3da5ad4009bbbadb50
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/117.exercises/solution.md"
@@ -0,0 +1,83 @@
+# 多数元素
+
+给定一个大小为 n 的数组,找到其中的多数元素。多数元素是指在数组中出现次数 大于 ⌊ n/2 ⌋
的元素。
+
+你可以假设数组是非空的,并且给定的数组总是存在多数元素。
+
+
+
+示例 1:
+
+
+输入:[3,2,3]
+输出:3
+
+示例 2:
+
+
+输入:[2,2,1,1,1,2,2]
+输出:2
+
+
+
+
+进阶:
+
+
+ - 尝试设计时间复杂度为 O(n)、空间复杂度为 O(1) 的算法解决此问题。
+
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int majorityElement(vector &nums)
+ {
+ unordered_map counts;
+ int majority = 0, cnt = 0;
+ for (int num : nums)
+ {
+ ++counts[num];
+ if (counts[num] > cnt)
+ {
+ majority = num;
+ cnt = counts[num];
+ }
+ }
+ return majority;
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..d8340c3cd9e0f30cdd74dc041f37a75de7f50396
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-bc50522c68bf48fd97e6f613722f66fa",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..57d7043585809d2aea4a9e5014398bb42b26d1b0
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "ba1063921dbe41418dee9ce903777741",
+ "author": "csdn.net",
+ "keywords": "数学,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..f9757c1eeb79b51b751792e4b8f4e95120bc9fe2
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/118.exercises/solution.md"
@@ -0,0 +1,108 @@
+# Excel表列序号
+
+给你一个字符串 columnTitle
,表示 Excel 表格中的列名称。返回该列名称对应的列序号。
+
+
+
+例如,
+
+
+ A -> 1
+ B -> 2
+ C -> 3
+ ...
+ Z -> 26
+ AA -> 27
+ AB -> 28
+ ...
+
+
+
+
+示例 1:
+
+
+输入: columnTitle = "A"
+输出: 1
+
+
+示例 2:
+
+
+输入: columnTitle = "AB"
+输出: 28
+
+
+示例 3:
+
+
+输入: columnTitle = "ZY"
+输出: 701
+
+示例 4:
+
+
+输入: columnTitle = "FXSHRXW"
+输出: 2147483647
+
+
+
+
+提示:
+
+
+ 1 <= columnTitle.length <= 7
+ columnTitle
仅由大写英文组成
+ columnTitle
在范围 ["A", "FXSHRXW"]
内
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int titleToNumber(string s)
+ {
+
+ long num = 0;
+
+ for (int i = 0; i < s.size(); i++)
+ {
+ num = (num * 26) + (s[i] - 64);
+ }
+ return num;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..c83c3a754cf0a8c1b762ffa33600be1b7e5a1ebb
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-345aecfe47a24f5aa0a5a616aa82d801",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..bf63c204d2610a00403c739f8475060bb266b0e4
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "090510ef86b14cb08933325e85f553dd",
+ "author": "csdn.net",
+ "keywords": "数学",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..3e5d8db58a343703664e0dbcfabe65bf19f290a3
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/119.exercises/solution.md"
@@ -0,0 +1,105 @@
+# 阶乘后的零
+
+给定一个整数 n
,返回 n!
结果中尾随零的数量。
+
+提示 n! = n * (n - 1) * (n - 2) * ... * 3 * 2 * 1
+
+
+
+示例 1:
+
+
+输入:n = 3
+输出:0
+解释:3! = 6 ,不含尾随 0
+
+
+示例 2:
+
+
+输入:n = 5
+输出:1
+解释:5! = 120 ,有一个尾随 0
+
+
+示例 3:
+
+
+输入:n = 0
+输出:0
+
+
+
+
+提示:
+
+
+
+
+
+进阶:你可以设计并实现对数时间复杂度的算法来解决此问题吗?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int trailingZeroes(int n)
+ {
+ int numOfZeros = 0;
+
+ while (n > 0)
+ {
+ numOfZeros += numOf5(n);
+ n--;
+ }
+
+ return numOfZeros;
+ }
+ int numOf5(int num)
+ {
+ int count = 0;
+ while ((num > 1) && (num % 5 == 0))
+ {
+ count++;
+ num /= 5;
+ }
+ return count;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..9d608750f11f421481cf2b84c3e60a05cd5ca9ad
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-524ced2fd5dd476bbfa3314db321e961",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3000ac31eac6ec656eeaeffa6b3aa4462734c53e
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "1bf7c916bc354627b654aff938342c0f",
+ "author": "csdn.net",
+ "keywords": "位运算,分治",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..d62bba09387b08898f9133ed45f08b41097085ff
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/120.exercises/solution.md"
@@ -0,0 +1,93 @@
+# 颠倒二进制位
+
+颠倒给定的 32 位无符号整数的二进制位。
+
+提示:
+
+
+ - 请注意,在某些语言(如 Java)中,没有无符号整数类型。在这种情况下,输入和输出都将被指定为有符号整数类型,并且不应影响您的实现,因为无论整数是有符号的还是无符号的,其内部的二进制表示形式都是相同的。
+ - 在 Java 中,编译器使用二进制补码记法来表示有符号整数。因此,在 示例 2 中,输入表示有符号整数
-3
,输出表示有符号整数 -1073741825
。
+
+
+
+
+示例 1:
+
+
+输入:n = 00000010100101000001111010011100
+输出:964176192 (00111001011110000010100101000000)
+解释:输入的二进制串 00000010100101000001111010011100 表示无符号整数 43261596,
+ 因此返回 964176192,其二进制表示形式为 00111001011110000010100101000000。
+
+示例 2:
+
+
+输入:n = 11111111111111111111111111111101
+输出:3221225471 (10111111111111111111111111111111)
+解释:输入的二进制串 11111111111111111111111111111101 表示无符号整数 4294967293,
+ 因此返回 3221225471 其二进制表示形式为 10111111111111111111111111111111 。
+
+
+
+提示:
+
+
+
+
+
+进阶: 如果多次调用这个函数,你将如何优化你的算法?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ uint32_t reverseBits(uint32_t n)
+ {
+ uint32_t res = 0;
+
+ for (int i = 0; i < 32; i++)
+ {
+ res <<= 1;
+ res |= n & 1;
+ n >>= 1;
+ }
+
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..06c626eba58be2d37c70a8b2deb2e2f6cac004da
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-e7e53eb27fac437d9d1a3dbdc258d5bf",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..1a3dc9a75b3dfd528a8e643718c410b0c0a64a51
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "e9ed6c10cc8046fdb9c2f303c9827372",
+ "author": "csdn.net",
+ "keywords": "位运算",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..e1880f15e5a818224870a10f42009a771e3a9a9e
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/121.exercises/solution.md"
@@ -0,0 +1,110 @@
+# 位1的个数
+
+编写一个函数,输入是一个无符号整数(以二进制串的形式),返回其二进制表达式中数字位数为 '1' 的个数(也被称为汉明重量)。
+
+
+
+提示:
+
+
+ - 请注意,在某些语言(如 Java)中,没有无符号整数类型。在这种情况下,输入和输出都将被指定为有符号整数类型,并且不应影响您的实现,因为无论整数是有符号的还是无符号的,其内部的二进制表示形式都是相同的。
+ - 在 Java 中,编译器使用二进制补码记法来表示有符号整数。因此,在上面的 示例 3 中,输入表示有符号整数
-3
。
+
+
+
+
+示例 1:
+
+
+输入:00000000000000000000000000001011
+输出:3
+解释:输入的二进制串 00000000000000000000000000001011 中,共有三位为 '1'。
+
+
+示例 2:
+
+
+输入:00000000000000000000000010000000
+输出:1
+解释:输入的二进制串 00000000000000000000000010000000 中,共有一位为 '1'。
+
+
+示例 3:
+
+
+输入:11111111111111111111111111111101
+输出:31
+解释:输入的二进制串 11111111111111111111111111111101 中,共有 31 位为 '1'。
+
+
+
+提示:
+
+
+
+
+
+
+
+进阶:
+
+
+ - 如果多次调用这个函数,你将如何优化你的算法?
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int hammingWeight(uint32_t n)
+ {
+ int count = 0;
+ uint32_t res = 1;
+ for (int i = 0; i < 32; i++)
+ {
+ if (res & n)
+ {
+ count++;
+ }
+ n >>= 1;
+ }
+ return count;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f16e18d0cbb93a2b7cb1a7b1c6a72719ee6babef
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-15f39c759f9b4aa99bb75b00bcf0580c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ac58ff5584edcf978227223596c4c88ac3b42737
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "dd2a5b9e9ed849f5b905fbbd20b230ea",
+ "author": "csdn.net",
+ "keywords": "哈希表,数学,双指针",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..10954e2884bbeb2bdb400baac55eafbe859ad34c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/122.exercises/solution.md"
@@ -0,0 +1,102 @@
+# 快乐数
+
+编写一个算法来判断一个数 n
是不是快乐数。
+
+「快乐数」定义为:
+
+
+ - 对于一个正整数,每一次将该数替换为它每个位置上的数字的平方和。
+ - 然后重复这个过程直到这个数变为 1,也可能是 无限循环 但始终变不到 1。
+ - 如果 可以变为 1,那么这个数就是快乐数。
+
+
+如果 n
是快乐数就返回 true
;不是,则返回 false
。
+
+
+
+示例 1:
+
+
+输入:19
+输出:true
+解释:
+12 + 92 = 82
+82 + 22 = 68
+62 + 82 = 100
+12 + 02 + 02 = 1
+
+
+示例 2:
+
+
+输入:n = 2
+输出:false
+
+
+
+
+提示:
+
+
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool isHappy(int n)
+ {
+ set s;
+ while (n != 1)
+ {
+ int t = 0;
+ while (n)
+ {
+ t += (n % 10) * (n % 10);
+ n /= 10;
+ }
+
+ int size = s.size();
+ s.insert(t);
+ if (size == s.size())
+ return false;
+ n = t;
+ }
+ return true;
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e3b65c9a4dffbbb05da3d1b7e2f6b3e2a8603536
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-ae3f500a3fd948b59a5433ed9e3abee5",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..0f461ff3f3178ead82f52fa2d070f8f215d25c26
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "764671b466ae41c7918438e006b78d38",
+ "author": "csdn.net",
+ "keywords": "递归,链表",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..4b34eddad1d3a5b53503fb1a9b3657f7768e5aa9
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/123.exercises/solution.md"
@@ -0,0 +1,104 @@
+# 移除链表元素
+
+给你一个链表的头节点 head
和一个整数 val
,请你删除链表中所有满足 Node.val == val
的节点,并返回 新的头节点 。
+
+
+示例 1:
+
+
+输入:head = [1,2,6,3,4,5,6], val = 6
+输出:[1,2,3,4,5]
+
+
+示例 2:
+
+
+输入:head = [], val = 1
+输出:[]
+
+
+示例 3:
+
+
+输入:head = [7,7,7,7], val = 7
+输出:[]
+
+
+
+
+提示:
+
+
+ - 列表中的节点数目在范围
[0, 104]
内
+ 1 <= Node.val <= 50
+ 0 <= val <= 50
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ ListNode *removeElements(ListNode *head, int val)
+ {
+ ListNode *dumynode = new ListNode(0);
+ dumynode->next = head;
+ ListNode *fast = dumynode->next;
+ ListNode *slow = dumynode;
+ while (fast != NULL)
+ {
+ if (fast->val == val)
+ {
+ slow->next = slow->next->next;
+ }
+ else
+ {
+ slow = slow->next;
+ }
+ fast = fast->next;
+ }
+ ListNode *ret = dumynode->next;
+ delete dumynode;
+ return ret;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..af598965ab98dc8aa5e259444457c230b07d022c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-7259fff1111e46ffa29f196ba542ba00",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ffc08bd15a61fb665a0b79349efb40bf5b0a7f8f
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "27936a1703b94c82bcc9f781c9194893",
+ "author": "csdn.net",
+ "keywords": "数组,数学,枚举,数论",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..5aec7be0c0d725dd8cb6ffc09807c59308a3eb60
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/124.exercises/solution.md"
@@ -0,0 +1,89 @@
+# 计数质数
+
+统计所有小于非负整数 n
的质数的数量。
+
+
+
+示例 1:
+
+输入:n = 10
+输出:4
+解释:小于 10 的质数一共有 4 个, 它们是 2, 3, 5, 7 。
+
+
+示例 2:
+
+输入:n = 0
+输出:0
+
+
+示例 3:
+
+输入:n = 1
+输出:0
+
+
+
+
+提示:
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int countPrimes(int n)
+ {
+ vector primesMap(n, true);
+ int count = 0;
+ for (int i = 2; i < n; i++)
+ {
+ if (primesMap[i])
+ {
+ count++;
+ for (int j = 2 * i; j < n; j += i)
+ {
+ primesMap[j] = false;
+ }
+ }
+ }
+ return count;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..02cda2e05b909c1db3c99a80261c6e2142fc87db
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-0e10486e4e8640adae0e951917a26845",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..9117eeee416a667ea011458d638e5f13cbeccd2c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "a240d4ffd705492787e87d9cf88bcab4",
+ "author": "csdn.net",
+ "keywords": "哈希表,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..b975a7446ef41fee5fb3ee74dc5d5f4a76750842
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/125.exercises/solution.md"
@@ -0,0 +1,98 @@
+# 同构字符串
+
+给定两个字符串 s 和 t,判断它们是否是同构的。
+
+如果 s 中的字符可以按某种映射关系替换得到 t ,那么这两个字符串是同构的。
+
+每个出现的字符都应当映射到另一个字符,同时不改变字符的顺序。不同字符不能映射到同一个字符上,相同字符只能映射到同一个字符上,字符可以映射到自己本身。
+
+
+
+示例 1:
+
+
+输入:s = "egg",
t = "add"
+输出:true
+
+
+示例 2:
+
+
+输入:s = "foo",
t = "bar"
+输出:false
+
+示例 3:
+
+
+输入:s = "paper",
t = "title"
+输出:true
+
+
+
+提示:
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool isIsomorphic(string s, string t)
+ {
+ vector m(128, -1);
+ for (int i = 0; i < s.size(); ++i)
+ {
+ if (m[s[i]] != -1)
+ {
+ if (m[s[i]] != t[i])
+ return false;
+ }
+ else
+ {
+ for (auto v : m)
+ {
+ if (v == t[i])
+ return false;
+ }
+ m[s[i]] = t[i];
+ }
+ }
+ return true;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..c9d067e114fdaadfe2685844ea26f9dd0781083b
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-88db4509fe484d61a6dcf07943003cb1",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..08c173ea1c4adcb2420b6634b04f2f8213435f03
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "d26956dec2844546a98f1ea107b00a65",
+ "author": "csdn.net",
+ "keywords": "递归,链表",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9fd32f3f95c9da6f5543309195259993612160e1
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/126.exercises/solution.md"
@@ -0,0 +1,112 @@
+# 反转链表
+
+给你单链表的头节点 head
,请你反转链表,并返回反转后的链表。
+
+
+
+
+
示例 1:
+
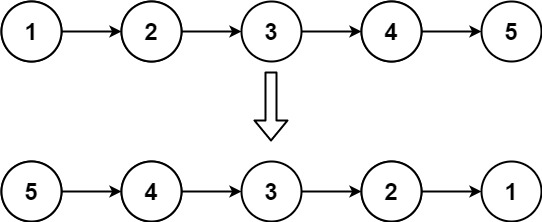
+
+输入:head = [1,2,3,4,5]
+输出:[5,4,3,2,1]
+
+
+
示例 2:
+
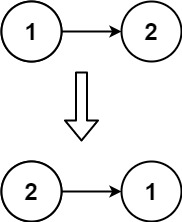
+
+输入:head = [1,2]
+输出:[2,1]
+
+
+
示例 3:
+
+
+输入:head = []
+输出:[]
+
+
+
+
+
提示:
+
+
+ - 链表中节点的数目范围是
[0, 5000]
+ -5000 <= Node.val <= 5000
+
+
+
+
+
进阶:链表可以选用迭代或递归方式完成反转。你能否用两种方法解决这道题?
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ ListNode *reverseList(ListNode *head)
+ {
+ if (head == NULL)
+ return NULL;
+ ListNode *node = NULL;
+ ListNode *temp = head;
+ ListNode *temp1 = head;
+ while (true)
+ {
+ if (temp->next == NULL)
+ {
+ temp1 = temp;
+ temp1->next = node;
+ break;
+ }
+ temp1 = temp;
+ temp = temp->next;
+ temp1->next = node;
+ node = temp1;
+ }
+ return temp1;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..0947c089a7348d38db0143bf3d94e5b7b3d2ce8f
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-510c86fa67d842068f7c3f018e8f89d9",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..965b5c3cf3fd90effc8d915addde2af95821fe13
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "c1b8804fd7a14cef8cd5f20e0f4ff762",
+ "author": "csdn.net",
+ "keywords": "数组,哈希表,排序",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..a56993f859ae443d5cf342385c797d01e32ef7cd
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/127.exercises/solution.md"
@@ -0,0 +1,81 @@
+# 存在重复元素
+
+给定一个整数数组,判断是否存在重复元素。
+
+如果存在一值在数组中出现至少两次,函数返回 true
。如果数组中每个元素都不相同,则返回 false
。
+
+
+
+示例 1:
+
+
+输入: [1,2,3,1]
+输出: true
+
+示例 2:
+
+
+输入: [1,2,3,4]
+输出: false
+
+示例 3:
+
+
+输入: [1,1,1,3,3,4,3,2,4,2]
+输出: true
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool containsDuplicate(vector &nums)
+ {
+ if (nums.empty())
+ {
+ return false;
+ }
+ sort(nums.begin(), nums.begin() + nums.size());
+ for (int i = 0; i < nums.size() - 1; i++)
+ {
+ if (nums[i] == nums[i + 1])
+ {
+ return true;
+ }
+ }
+ return false;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..790b05b8af9e349b400efb7bf0e84ac30c62c673
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-6326da4a969f49dc810d995343223f5e",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b70e9b7f551637a9bc14e6bef02ac01d66791e3a
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "243066531cd64f568c3a8386611c0089",
+ "author": "csdn.net",
+ "keywords": "数组,哈希表,滑动窗口",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..7f4bbe9e9a2d7784d168e9ac6e2a0635b018f94d
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/128.exercises/solution.md"
@@ -0,0 +1,79 @@
+# 存在重复元素 II
+
+给定一个整数数组和一个整数 k,判断数组中是否存在两个不同的索引 i 和 j,使得 nums [i] = nums [j],并且 i 和 j 的差的 绝对值 至多为 k。
+
+
+
+示例 1:
+
+输入: nums = [1,2,3,1], k = 3
+输出: true
+
+示例 2:
+
+输入: nums = [1,0,1,1], k = 1
+输出: true
+
+示例 3:
+
+输入: nums = [1,2,3,1,2,3], k = 2
+输出: false
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool containsNearbyDuplicate(vector &nums, int k)
+ {
+ int n = nums.size(), idx = 0;
+ unordered_map nmap;
+ for (int i = 0; i < n; ++i)
+ {
+ auto iter = nmap.find(nums[i]);
+ if (iter != nmap.end())
+ {
+ if (i - iter->second <= k)
+ return true;
+ else
+ iter->second = i;
+ }
+ else
+ nmap[nums[i]] = i;
+ }
+ return false;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3fb9542ee9f9c8eb8dc54e79194a0007e7d6c71c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-50e1cb8edc1d4dc19b4d95ab248cbe36",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7d17debd77394d96f68bb6d9a023de1b3cab4fce
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "86948c5e7a8a44ce9a4c9acc737a3770",
+ "author": "csdn.net",
+ "keywords": "栈,设计,队列",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..07a8ea7ca0b6a62f8605e636229f3f0e16c5cebb
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/129.exercises/solution.md"
@@ -0,0 +1,130 @@
+# 用队列实现栈
+
+请你仅使用两个队列实现一个后入先出(LIFO)的栈,并支持普通栈的全部四种操作(push
、top
、pop
和 empty
)。
+
+实现 MyStack
类:
+
+
+ void push(int x)
将元素 x 压入栈顶。
+ int pop()
移除并返回栈顶元素。
+ int top()
返回栈顶元素。
+ boolean empty()
如果栈是空的,返回 true
;否则,返回 false
。
+
+
+
+
+注意:
+
+
+ - 你只能使用队列的基本操作 —— 也就是
push to back
、peek/pop from front
、size
和 is empty
这些操作。
+ - 你所使用的语言也许不支持队列。 你可以使用 list (列表)或者 deque(双端队列)来模拟一个队列 , 只要是标准的队列操作即可。
+
+
+
+
+示例:
+
+
+输入:
+["MyStack", "push", "push", "top", "pop", "empty"]
+[[], [1], [2], [], [], []]
+输出:
+[null, null, null, 2, 2, false]
+
+解释:
+MyStack myStack = new MyStack();
+myStack.push(1);
+myStack.push(2);
+myStack.top(); // 返回 2
+myStack.pop(); // 返回 2
+myStack.empty(); // 返回 False
+
+
+
+
+提示:
+
+
+ 1 <= x <= 9
+ - 最多调用
100
次 push
、pop
、top
和 empty
+ - 每次调用
pop
和 top
都保证栈不为空
+
+
+
+
+进阶:你能否实现每种操作的均摊时间复杂度为 O(1)
的栈?换句话说,执行 n
个操作的总时间复杂度 O(n)
,尽管其中某个操作可能需要比其他操作更长的时间。你可以使用两个以上的队列。
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class MyStack
+{
+public:
+ MyStack()
+ {
+ }
+ void push(int x)
+ {
+ std::queue temp_queue;
+ temp_queue.push(x);
+ while (!_data.empty())
+ {
+ temp_queue.push(_data.front());
+ _data.pop();
+ }
+ while (!temp_queue.empty())
+ {
+ _data.push(temp_queue.front());
+ temp_queue.pop();
+ }
+ }
+ int pop()
+ {
+ int x = _data.front();
+ _data.pop();
+ return x;
+ }
+ int top()
+ {
+ return _data.front();
+ }
+ bool empty()
+ {
+ return _data.empty();
+ }
+
+private:
+ std::queue _data;
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..33f85fa0f344e1fa44205005130eccfcc17fe2d9
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-e1a1c4598bdb483a8b73a902222956df",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..520ee5a4b83d7c30bc78067254186c3bf7f6740b
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "68c7ad63c6ac42af89a763d04013e0f2",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..65e7812370ad625806e0f069c5eb9653c701ac37
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/130.exercises/solution.md"
@@ -0,0 +1,85 @@
+# 翻转二叉树
+
+翻转一棵二叉树。
+
+示例:
+
+输入:
+
+ 4
+ / \
+ 2 7
+ / \ / \
+1 3 6 9
+
+输出:
+
+ 4
+ / \
+ 7 2
+ / \ / \
+9 6 3 1
+
+备注:
+这个问题是受到 Max Howell 的 原问题 启发的 :
+
+谷歌:我们90%的工程师使用您编写的软件(Homebrew),但是您却无法在面试时在白板上写出翻转二叉树这道题,这太糟糕了。
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ TreeNode *invertTree(TreeNode *root)
+ {
+ if (!root)
+ {
+ return root;
+ }
+ TreeNode *temp = root->left;
+ root->left = invertTree(root->right);
+ root->right = invertTree(temp);
+ return root;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..d91fc776a5d19fd7b7c5f5d9a50199aee16dc110
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-3ee24b6deba740379e077abdc180342f",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..6ef8b113a26cff7895e526da143dfbd6e26e0696
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "79ea6f8b6f134986bb083e68baeb4266",
+ "author": "csdn.net",
+ "keywords": "数组",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..7dd8f72164da8f250edfe2916eb489f49574bf37
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/131.exercises/solution.md"
@@ -0,0 +1,127 @@
+# 汇总区间
+
+给定一个无重复元素的有序整数数组 nums
。
+
+返回 恰好覆盖数组中所有数字 的 最小有序 区间范围列表。也就是说,nums
的每个元素都恰好被某个区间范围所覆盖,并且不存在属于某个范围但不属于 nums
的数字 x
。
+
+列表中的每个区间范围 [a,b]
应该按如下格式输出:
+
+
+ "a->b"
,如果 a != b
+ "a"
,如果 a == b
+
+
+
+
+示例 1:
+
+
+输入:nums = [0,1,2,4,5,7]
+输出:["0->2","4->5","7"]
+解释:区间范围是:
+[0,2] --> "0->2"
+[4,5] --> "4->5"
+[7,7] --> "7"
+
+
+示例 2:
+
+
+输入:nums = [0,2,3,4,6,8,9]
+输出:["0","2->4","6","8->9"]
+解释:区间范围是:
+[0,0] --> "0"
+[2,4] --> "2->4"
+[6,6] --> "6"
+[8,9] --> "8->9"
+
+
+示例 3:
+
+
+输入:nums = []
+输出:[]
+
+
+示例 4:
+
+
+输入:nums = [-1]
+输出:["-1"]
+
+
+示例 5:
+
+
+输入:nums = [0]
+输出:["0"]
+
+
+
+
+提示:
+
+
+ 0 <= nums.length <= 20
+ -231 <= nums[i] <= 231 - 1
+ nums
中的所有值都 互不相同
+ nums
按升序排列
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ vector summaryRanges(vector &nums)
+ {
+ int n = nums.size();
+ vector ans;
+ int i = 0;
+ while (i < n)
+ {
+ int j = i;
+ while (j + 1 < n && nums[j + 1] == nums[j] + 1)
+ j++;
+ if (i == j)
+ ans.push_back(to_string(nums[i]));
+ else
+ ans.push_back(to_string(nums[i]) + "->" + to_string(nums[j]));
+ i = j + 1;
+ }
+ return ans;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e39d89943313a82fc95676b072010e9e6a7a4699
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-73795c2480d4451cbba108521a80078c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e511e4ce6ac501cd83c3c9f53506a1e0733656eb
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "4ca1d7d6fdc3440390e82ed46d4bed70",
+ "author": "csdn.net",
+ "keywords": "位运算,递归,数学",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..b2d9ce3c926eceb6370fb1baac6c231b72f62158
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/132.exercises/solution.md"
@@ -0,0 +1,105 @@
+# 2 的幂
+
+给你一个整数 n
,请你判断该整数是否是 2 的幂次方。如果是,返回 true
;否则,返回 false
。
+
+如果存在一个整数 x
使得 n == 2x
,则认为 n
是 2 的幂次方。
+
+
+
+示例 1:
+
+
+输入:n = 1
+输出:true
+解释:20 = 1
+
+
+示例 2:
+
+
+输入:n = 16
+输出:true
+解释:24 = 16
+
+
+示例 3:
+
+
+输入:n = 3
+输出:false
+
+
+示例 4:
+
+
+输入:n = 4
+输出:true
+
+
+示例 5:
+
+
+输入:n = 5
+输出:false
+
+
+
+
+提示:
+
+
+
+
+
+进阶:你能够不使用循环/递归解决此问题吗?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool isPowerOfTwo(int n)
+ {
+ int cur = 0;
+ for (int i = 0; i < 31; i++)
+ {
+ cur += (n >> i) & 1;
+ }
+ return n > 0 && cur == 1;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..109d9dd5dbf7560fd24452e9378d051f8af0d42c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-541c93e32cbc42deb82573d4759671e5",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..22f35787eaf19b1bbb1a836ab42acec2f293c57f
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "147ed9774f9941238c0c45a42cb896cc",
+ "author": "csdn.net",
+ "keywords": "栈,设计,队列",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..b3d46a38ff59e482180ca508a202da4a28732393
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/133.exercises/solution.md"
@@ -0,0 +1,144 @@
+# 用栈实现队列
+
+请你仅使用两个栈实现先入先出队列。队列应当支持一般队列支持的所有操作(push
、pop
、peek
、empty
):
+
+实现 MyQueue
类:
+
+
+ void push(int x)
将元素 x 推到队列的末尾
+ int pop()
从队列的开头移除并返回元素
+ int peek()
返回队列开头的元素
+ boolean empty()
如果队列为空,返回 true
;否则,返回 false
+
+
+
+
+说明:
+
+
+ - 你只能使用标准的栈操作 —— 也就是只有
push to top
, peek/pop from top
, size
, 和 is empty
操作是合法的。
+ - 你所使用的语言也许不支持栈。你可以使用 list 或者 deque(双端队列)来模拟一个栈,只要是标准的栈操作即可。
+
+
+
+
+进阶:
+
+
+ - 你能否实现每个操作均摊时间复杂度为
O(1)
的队列?换句话说,执行 n
个操作的总时间复杂度为 O(n)
,即使其中一个操作可能花费较长时间。
+
+
+
+
+示例:
+
+
+输入:
+["MyQueue", "push", "push", "peek", "pop", "empty"]
+[[], [1], [2], [], [], []]
+输出:
+[null, null, null, 1, 1, false]
+
+解释:
+MyQueue myQueue = new MyQueue();
+myQueue.push(1); // queue is: [1]
+myQueue.push(2); // queue is: [1, 2] (leftmost is front of the queue)
+myQueue.peek(); // return 1
+myQueue.pop(); // return 1, queue is [2]
+myQueue.empty(); // return false
+
+
+
+
+
+
+提示:
+
+
+ 1 <= x <= 9
+ - 最多调用
100
次 push
、pop
、peek
和 empty
+ - 假设所有操作都是有效的 (例如,一个空的队列不会调用
pop
或者 peek
操作)
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class MyQueue
+{
+public:
+ /** Initialize your data structure here. */
+ stack a, b;
+
+ MyQueue()
+ {
+ }
+
+ /** Push element x to the back of queue. */
+ void push(int x)
+ {
+ while (!b.empty())
+ {
+ a.push(b.top());
+ b.pop();
+ }
+ b.push(x);
+ while (!a.empty())
+ {
+ b.push(a.top());
+ a.pop();
+ }
+ }
+
+ /** Removes the element from in front of queue and returns that element. */
+ int pop()
+ {
+ int res = b.top();
+ b.pop();
+ return res;
+ }
+
+ /** Get the front element. */
+ int peek()
+ {
+ return b.top();
+ }
+
+ /** Returns whether the queue is empty. */
+ bool empty()
+ {
+ return b.empty();
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..048320e6854ce365b2dde0341a8132af415d1131
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-9416ddb972a34cf1aa8a10edf76ce65f",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..97b5fda929a80fa57d6584e93c38908a41dd9f50
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "3fa672cdbfc84d7893fb5d42da794ab2",
+ "author": "csdn.net",
+ "keywords": "栈,递归,链表,双指针",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..2bd533e93583028741b4afbde7cdc0d6788db3fc
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/134.exercises/solution.md"
@@ -0,0 +1,94 @@
+# 回文链表
+
+给你一个单链表的头节点 head
,请你判断该链表是否为回文链表。如果是,返回 true
;否则,返回 false
。
+
+
+
+示例 1:
+
+
+输入:head = [1,2,2,1]
+输出:true
+
+
+示例 2:
+
+
+输入:head = [1,2]
+输出:false
+
+
+
+
+提示:
+
+
+ - 链表中节点数目在范围
[1, 105]
内
+ 0 <= Node.val <= 9
+
+
+
+
+进阶:你能否用 O(n)
时间复杂度和 O(1)
空间复杂度解决此题?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ bool isPalindrome(ListNode *head)
+ {
+ vector v;
+ while (head != NULL)
+ {
+ v.push_back(head->val);
+ head = head->next;
+ }
+ for (int i = 0; i < v.size(); i++)
+ {
+ if (v[i] != v[v.size() - i - 1])
+ return false;
+ }
+ return true;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e4f46f6e4a94633c80419ca41b5eef91f9198c70
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-27db96ec9b784632b5e93fa1cec6df61",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b5514f6f15af73e36450263696282abad8938a50
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "13e6888b14114d16b05c8513cbdde328",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,二叉搜索树,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..7ddce001c1764530dd92cc5e45a7419416b3bf5f
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/135.exercises/solution.md"
@@ -0,0 +1,92 @@
+# 二叉搜索树的最近公共祖先
+
+给定一个二叉搜索树, 找到该树中两个指定节点的最近公共祖先。
+
+百度百科中最近公共祖先的定义为:“对于有根树 T 的两个结点 p、q,最近公共祖先表示为一个结点 x,满足 x 是 p、q 的祖先且 x 的深度尽可能大(一个节点也可以是它自己的祖先)。”
+
+例如,给定如下二叉搜索树: root = [6,2,8,0,4,7,9,null,null,3,5]
+
+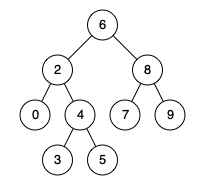
+
+
+
+示例 1:
+
+输入: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 8
+输出: 6
+解释: 节点 2
和节点 8
的最近公共祖先是 6。
+
+
+示例 2:
+
+输入: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 4
+输出: 2
+解释: 节点 2
和节点 4
的最近公共祖先是 2
, 因为根据定义最近公共祖先节点可以为节点本身。
+
+
+
+说明:
+
+
+ - 所有节点的值都是唯一的。
+ - p、q 为不同节点且均存在于给定的二叉搜索树中。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ TreeNode *lowestCommonAncestor(TreeNode *root, TreeNode *p, TreeNode *q)
+ {
+ if (root == NULL)
+ return NULL;
+ if ((root->val > q->val) && (root->val > p->val))
+ return lowestCommonAncestor(root->left, p, q);
+ else if ((root->val < q->val) && (root->val < p->val))
+ return lowestCommonAncestor(root->right, p, q);
+ return root;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b65dab7e226332e9b6feb8da2014d00e76c98b2c
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-b1b34d10555f424f9012194aff09f895",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..912f2271e97ebbc69bce4d9755c7a3d1a9b36efd
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "d79649cb8a834b20905d373e6708efce",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..747c823ac19b96203039c91914092b38fbbc1398
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/98.exercises/solution.md"
@@ -0,0 +1,94 @@
+# 对称二叉树
+
+给定一个二叉树,检查它是否是镜像对称的。
+
+
+
+例如,二叉树 [1,2,2,3,4,4,3]
是对称的。
+
+ 1
+ / \
+ 2 2
+ / \ / \
+3 4 4 3
+
+
+
+
+但是下面这个 [1,2,2,null,3,null,3]
则不是镜像对称的:
+
+ 1
+ / \
+ 2 2
+ \ \
+ 3 3
+
+
+
+
+进阶:
+
+你可以运用递归和迭代两种方法解决这个问题吗?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ bool haha(TreeNode *l, TreeNode *r)
+ {
+ if (!l && !r)
+ return true;
+ if (!l || !r)
+ return false;
+ if (l->val != r->val)
+ return false;
+ return haha(l->right, r->left) & haha(l->left, r->right);
+ }
+ bool isSymmetric(TreeNode *root)
+ {
+ if (!root)
+ return true;
+ return haha(root->left, root->right);
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/config.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..30d193abd86dd9009b11f9c1a0d1b57d5af3f855
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-b34e8ed950f64727b2b4653b8433dc22",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/solution.json" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..2cf31e507b9a8c06750f254644c3ab3ec0acdea9
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "32cd436081f24793a212d2ea766f0ba1",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/solution.md" "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..dd3a1892c265cd372d21063326cf72490d5332e3
--- /dev/null
+++ "b/data/1.dailycode\345\210\235\351\230\266/1.cpp/99.exercises/solution.md"
@@ -0,0 +1,79 @@
+# 二叉树的最大深度
+
+给定一个二叉树,找出其最大深度。
+
+二叉树的深度为根节点到最远叶子节点的最长路径上的节点数。
+
+说明: 叶子节点是指没有子节点的节点。
+
+示例:
+给定二叉树 [3,9,20,null,null,15,7]
,
+
+ 3
+ / \
+ 9 20
+ / \
+ 15 7
+
+返回它的最大深度 3 。
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ int maxDepth(TreeNode *root)
+ {
+ int depth = 0;
+ if (root == NULL)
+ {
+ return 0;
+ }
+ else
+ {
+ depth = max(maxDepth(root->left), maxDepth(root->right));
+ return 1 + depth;
+ }
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..d017db5ce78ded8a866e0a65744ef616b6f17838
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-9c576c7dcc0247e2ae923077462c4baa",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..2453785c835b7f0ff6d0f160dd016e3c10b486df
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "aaa09326cb5644cc8065da1415bc2c12",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..436bf0907707e22ef63e184273ea432db94b2d54
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/100.exercises/solution.md"
@@ -0,0 +1,137 @@
+# 求根节点到叶节点数字之和
+
+给你一个二叉树的根节点 root
,树中每个节点都存放有一个 0
到 9
之间的数字。
+
+
+
每条从根节点到叶节点的路径都代表一个数字:
+
+
+ - 例如,从根节点到叶节点的路径
1 -> 2 -> 3
表示数字 123
。
+
+
+
计算从根节点到叶节点生成的 所有数字之和 。
+
+
叶节点 是指没有子节点的节点。
+
+
+
+
示例 1:
+
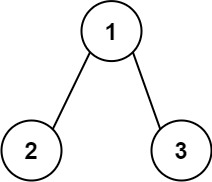
+
+输入:root = [1,2,3]
+输出:25
+解释:
+从根到叶子节点路径 1->2
代表数字 12
+从根到叶子节点路径 1->3
代表数字 13
+因此,数字总和 = 12 + 13 = 25
+
+
示例 2:
+
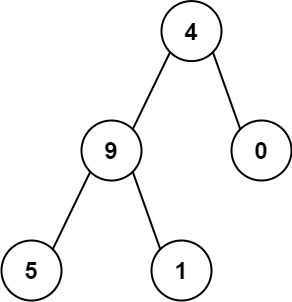
+
+输入:root = [4,9,0,5,1]
+输出:1026
+解释:
+从根到叶子节点路径 4->9->5
代表数字 495
+从根到叶子节点路径 4->9->1
代表数字 491
+从根到叶子节点路径 4->0
代表数字 40
+因此,数字总和 = 495 + 491 + 40 = 1026
+
+
+
+
+
提示:
+
+
+ - 树中节点的数目在范围
[1, 1000]
内
+ 0 <= Node.val <= 9
+ - 树的深度不超过
10
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ int sumNumbers(TreeNode *root)
+ {
+ if (root == nullptr)
+ return 0;
+ int result = 0;
+ vector num;
+ vector temp;
+ digui(root, &num, &temp);
+ for (int i = 0; i < num.size(); i++)
+ {
+ result = result + num[i];
+ }
+ return result;
+ }
+ void digui(TreeNode *root, vector *num, vector *temp)
+ {
+ temp->push_back(root->val);
+ if (root->left == nullptr && root->right == nullptr)
+ {
+ int sum = 0;
+ for (int i = temp->size() - 1; i >= 0; i--)
+ {
+ /*if (i==0 && (*temp)[0]==0) {
+ continue;
+ }*/
+ int howi = (*temp)[i];
+ sum = sum + howi * pow(10, (temp->size() - i - 1));
+ }
+ num->push_back(sum);
+ temp->pop_back();
+ return;
+ }
+ if (root->left)
+ digui(root->left, num, temp);
+ if (root->right)
+ digui(root->right, num, temp);
+ temp->pop_back();
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..74dfb6e02e635daf5de4d7203cfc6fd1f9a90a34
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-0c3ef2e1bd1242338188dd04275436e9",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..107b6055f33311d2eb0318e0a136716c91930d95
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "da6922e6926a4e50899352935ffd9566",
+ "author": "csdn.net",
+ "keywords": "深度优先搜索,广度优先搜索,并查集,数组,矩阵",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..cd76118caaaa542366a9d3b83dc06b084409fe3b
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/101.exercises/solution.md"
@@ -0,0 +1,131 @@
+# 被围绕的区域
+
+给你一个 m x n
的矩阵 board
,由若干字符 'X'
和 'O'
,找到所有被 'X'
围绕的区域,并将这些区域里所有的 'O'
用 'X'
填充。
+
+
+
+
+
示例 1:
+
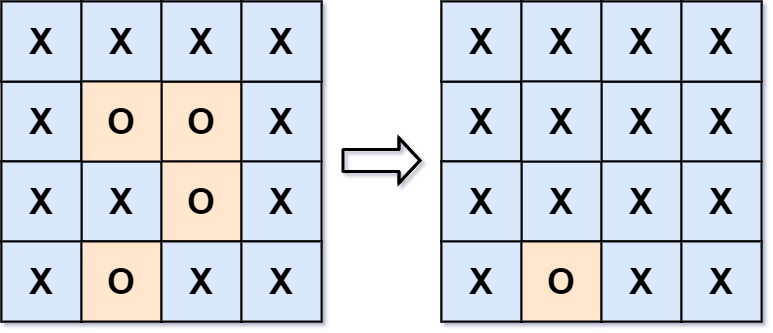
+
+输入:board = [["X","X","X","X"],["X","O","O","X"],["X","X","O","X"],["X","O","X","X"]]
+输出:[["X","X","X","X"],["X","X","X","X"],["X","X","X","X"],["X","O","X","X"]]
+解释:被围绕的区间不会存在于边界上,换句话说,任何边界上的 'O'
都不会被填充为 'X'
。 任何不在边界上,或不与边界上的 'O'
相连的 'O'
最终都会被填充为 'X'
。如果两个元素在水平或垂直方向相邻,则称它们是“相连”的。
+
+
+
示例 2:
+
+
+输入:board = [["X"]]
+输出:[["X"]]
+
+
+
+
+
提示:
+
+
+ m == board.length
+ n == board[i].length
+ 1 <= m, n <= 200
+ board[i][j]
为 'X'
或 'O'
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int m, n;
+ void solve(vector> &board)
+ {
+ m = board.size();
+ if (!m)
+ return;
+ n = board[0].size();
+ if (!n)
+ return;
+ for (int i = 0; i < m; ++i)
+ {
+ if (i == 0 || i == m - 1)
+ {
+ for (int j = 0; j < n; ++j)
+ {
+ if (board[i][j] == 'O')
+ dfs(board, i, j);
+ }
+ }
+ else
+ {
+ if (board[i][0] == 'O')
+ dfs(board, i, 0);
+ if (board[i][n - 1] == 'O')
+ dfs(board, i, n - 1);
+ }
+ }
+ for (int i = 0; i < m; ++i)
+ for (int j = 0; j < n; ++j)
+ {
+ if (board[i][j] == 'A')
+ board[i][j] = 'O';
+ else
+ board[i][j] = 'X';
+ }
+ }
+ void dfs(vector> &board, int i, int j)
+ {
+ board[i][j] = 'A';
+ if (i - 1 < m && i - 1 >= 0 && j < n && j >= 0 && board[i - 1][j] == 'O')
+ {
+ dfs(board, i - 1, j);
+ }
+ if (i + 1 < m && i + 1 >= 0 && j < n && j >= 0 && board[i + 1][j] == 'O')
+ {
+ dfs(board, i + 1, j);
+ }
+ if (i < m && i >= 0 && j - 1 < n && j - 1 >= 0 && board[i][j - 1] == 'O')
+ {
+ dfs(board, i, j - 1);
+ }
+ if (i < m && i >= 0 && j + 1 < n && j + 1 >= 0 && board[i][j + 1] == 'O')
+ {
+ dfs(board, i, j + 1);
+ }
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e6e7189c470c590bb432fa2c85a0e5631b87174e
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-6595ec837a47451bb033b3d82cbcf542",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f54885f6076789613939286bafc9c07abf7b24f1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "e58bd889e1d14d47b472202ff1401855",
+ "author": "csdn.net",
+ "keywords": "字符串,动态规划,回溯",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..b8653cf5324782754e27531ed6c35eedfdbdc764
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/102.exercises/solution.md"
@@ -0,0 +1,103 @@
+# 分割回文串
+
+给你一个字符串 s
,请你将 s
分割成一些子串,使每个子串都是 回文串 。返回 s
所有可能的分割方案。
+
+回文串 是正着读和反着读都一样的字符串。
+
+
+
+示例 1:
+
+
+输入:s = "aab"
+输出:[["a","a","b"],["aa","b"]]
+
+
+示例 2:
+
+
+输入:s = "a"
+输出:[["a"]]
+
+
+
+
+提示:
+
+
+ 1 <= s.length <= 16
+ s
仅由小写英文字母组成
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool isPali(string s)
+ {
+ for (int i = 0; i < s.length() / 2; i++)
+ if (s[i] != s[s.length() - i - 1])
+ return false;
+ return true;
+ }
+
+ void dfs(vector> &ans, vector &tmp, int n, string s)
+ {
+ if (n == s.length())
+ {
+ ans.push_back(tmp);
+ return;
+ }
+ for (int i = n; i < s.length(); i++)
+ {
+ if (isPali(s.substr(n, i - n + 1)))
+ {
+ tmp.push_back(s.substr(n, i - n + 1));
+ dfs(ans, tmp, i + 1, s);
+ tmp.pop_back();
+ }
+ }
+ }
+
+ vector> partition(string s)
+ {
+ vector> ans;
+ vector tmp;
+ dfs(ans, tmp, 0, s);
+ return ans;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e99d0bb9b7895d20fd1c88a7827365b5da8a1bc1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-2e0242542523489684fe462e57e5dc2d",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..064bec575a476db85ff612b72243dfefd217c967
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "bf408a7721784878b90fb71b52238074",
+ "author": "csdn.net",
+ "keywords": "深度优先搜索,广度优先搜索,图,哈希表",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..89b5548bc69699af056d1511803de6abb3e61ac2
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/103.exercises/solution.md"
@@ -0,0 +1,146 @@
+# 克隆图
+
+给你无向 连通 图中一个节点的引用,请你返回该图的 深拷贝(克隆)。
+
+图中的每个节点都包含它的值 val
(int
) 和其邻居的列表(list[Node]
)。
+
+class Node {
+ public int val;
+ public List<Node> neighbors;
+}
+
+
+
+测试用例格式:
+
+简单起见,每个节点的值都和它的索引相同。例如,第一个节点值为 1(val = 1
),第二个节点值为 2(val = 2
),以此类推。该图在测试用例中使用邻接列表表示。
+
+邻接列表 是用于表示有限图的无序列表的集合。每个列表都描述了图中节点的邻居集。
+
+给定节点将始终是图中的第一个节点(值为 1)。你必须将 给定节点的拷贝 作为对克隆图的引用返回。
+
+
+
+示例 1:
+
+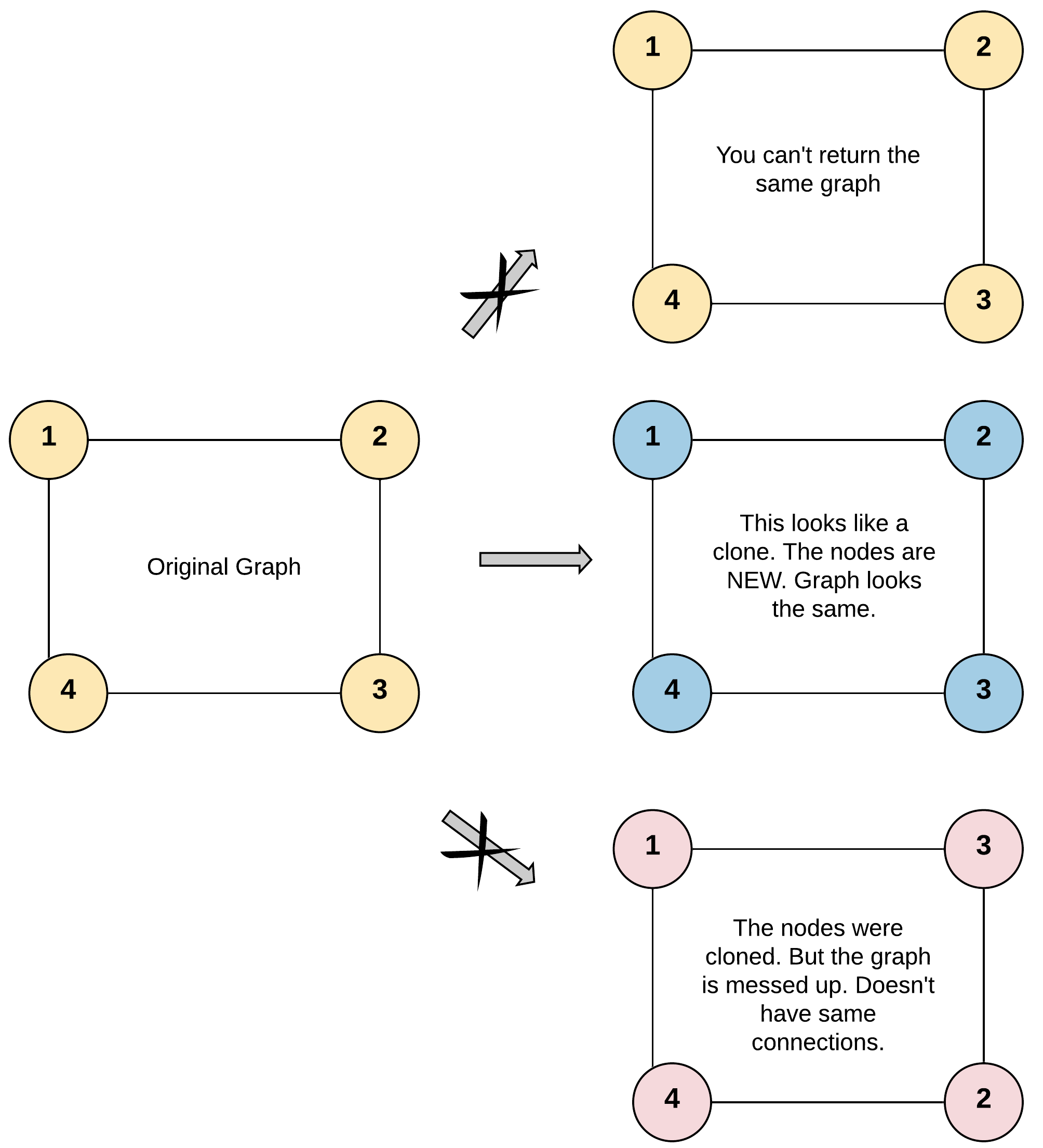
+
+输入:adjList = [[2,4],[1,3],[2,4],[1,3]]
+输出:[[2,4],[1,3],[2,4],[1,3]]
+解释:
+图中有 4 个节点。
+节点 1 的值是 1,它有两个邻居:节点 2 和 4 。
+节点 2 的值是 2,它有两个邻居:节点 1 和 3 。
+节点 3 的值是 3,它有两个邻居:节点 2 和 4 。
+节点 4 的值是 4,它有两个邻居:节点 1 和 3 。
+
+
+示例 2:
+
+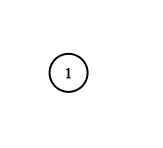
+
+输入:adjList = [[]]
+输出:[[]]
+解释:输入包含一个空列表。该图仅仅只有一个值为 1 的节点,它没有任何邻居。
+
+
+示例 3:
+
+输入:adjList = []
+输出:[]
+解释:这个图是空的,它不含任何节点。
+
+
+示例 4:
+
+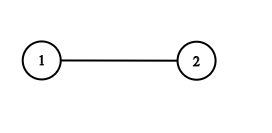
+
+输入:adjList = [[2],[1]]
+输出:[[2],[1]]
+
+
+
+提示:
+
+
+ - 节点数不超过 100 。
+ - 每个节点值
Node.val
都是唯一的,1 <= Node.val <= 100
。
+ - 无向图是一个简单图,这意味着图中没有重复的边,也没有自环。
+ - 由于图是无向的,如果节点 p 是节点 q 的邻居,那么节点 q 也必须是节点 p 的邻居。
+ - 图是连通图,你可以从给定节点访问到所有节点。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Node
+{
+public:
+ int val;
+ vector neighbors;
+
+ Node() {}
+
+ Node(int _val, vector _neighbors)
+ {
+ val = _val;
+ neighbors = _neighbors;
+ }
+};
+
+class Solution
+{
+public:
+ Node *cloneGraph(Node *node)
+ {
+ unordered_map m;
+ return helper(node, m);
+ }
+ Node *helper(Node *node, unordered_map &m)
+ {
+ if (!node)
+ return NULL;
+ if (m.count(node))
+ return m[node];
+ Node *clone = new Node(node->val);
+ m[node] = clone;
+ for (Node *neighbor : node->neighbors)
+ {
+ clone->neighbors.push_back(helper(neighbor, m));
+ }
+ return clone;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b865010482f0fdf613e2d8ec9532c920cb02db5c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-75dace4205f44a3598936beb6e9bab7a",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..9a00f2bd1bf8eaf61b6ec218e75ec38f935bcc4e
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "1f2b3e0e87e44f42a819c33feb21cce4",
+ "author": "csdn.net",
+ "keywords": "贪心,数组",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..4786b640caf11260f7e7f9f1bb887428589394bc
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/104.exercises/solution.md"
@@ -0,0 +1,110 @@
+# 加油站
+
+在一条环路上有 N 个加油站,其中第 i 个加油站有汽油 gas[i]
升。
+
+你有一辆油箱容量无限的的汽车,从第 i 个加油站开往第 i+1 个加油站需要消耗汽油 cost[i]
升。你从其中的一个加油站出发,开始时油箱为空。
+
+如果你可以绕环路行驶一周,则返回出发时加油站的编号,否则返回 -1。
+
+说明:
+
+
+ - 如果题目有解,该答案即为唯一答案。
+ - 输入数组均为非空数组,且长度相同。
+ - 输入数组中的元素均为非负数。
+
+
+示例 1:
+
+输入:
+gas = [1,2,3,4,5]
+cost = [3,4,5,1,2]
+
+输出: 3
+
+解释:
+从 3 号加油站(索引为 3 处)出发,可获得 4 升汽油。此时油箱有 = 0 + 4 = 4 升汽油
+开往 4 号加油站,此时油箱有 4 - 1 + 5 = 8 升汽油
+开往 0 号加油站,此时油箱有 8 - 2 + 1 = 7 升汽油
+开往 1 号加油站,此时油箱有 7 - 3 + 2 = 6 升汽油
+开往 2 号加油站,此时油箱有 6 - 4 + 3 = 5 升汽油
+开往 3 号加油站,你需要消耗 5 升汽油,正好足够你返回到 3 号加油站。
+因此,3 可为起始索引。
+
+示例 2:
+
+输入:
+gas = [2,3,4]
+cost = [3,4,3]
+
+输出: -1
+
+解释:
+你不能从 0 号或 1 号加油站出发,因为没有足够的汽油可以让你行驶到下一个加油站。
+我们从 2 号加油站出发,可以获得 4 升汽油。 此时油箱有 = 0 + 4 = 4 升汽油
+开往 0 号加油站,此时油箱有 4 - 3 + 2 = 3 升汽油
+开往 1 号加油站,此时油箱有 3 - 3 + 3 = 3 升汽油
+你无法返回 2 号加油站,因为返程需要消耗 4 升汽油,但是你的油箱只有 3 升汽油。
+因此,无论怎样,你都不可能绕环路行驶一周。
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int canCompleteCircuit(vector &gas, vector &cost)
+ {
+ int index = 0;
+ int sum = 0;
+ int csum = 0;
+ for (int i = 0; i < gas.size(); i++)
+ {
+ int temp = gas[i] - cost[i];
+ sum += temp;
+ if (csum > 0)
+ {
+ csum += gas[i] - cost[i];
+ }
+ else
+ {
+ csum = gas[i] - cost[i];
+ index = i;
+ }
+ }
+ return sum >= 0 ? index : -1;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..a67408d53dd860395f5095e9f6dc3b5e8f1578b1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-0de614ddd4a841aca80d2037ae64bfe9",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..582f78c609f894ec5399250bd88e34bcbecc859f
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "afa57be04ea148dcbe59f3b4b37f9e99",
+ "author": "csdn.net",
+ "keywords": "位运算,数组",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9c0c0a45c1f7f91520adf83815a676fec8b012bb
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/105.exercises/solution.md"
@@ -0,0 +1,100 @@
+# 只出现一次的数字 II
+
+给你一个整数数组 nums
,除某个元素仅出现 一次 外,其余每个元素都恰出现 三次 。请你找出并返回那个只出现了一次的元素。
+
+
+
+示例 1:
+
+
+输入:nums = [2,2,3,2]
+输出:3
+
+
+示例 2:
+
+
+输入:nums = [0,1,0,1,0,1,99]
+输出:99
+
+
+
+
+提示:
+
+
+ 1 <= nums.length <= 3 * 104
+ -231 <= nums[i] <= 231 - 1
+ nums
中,除某个元素仅出现 一次 外,其余每个元素都恰出现 三次
+
+
+
+
+进阶:你的算法应该具有线性时间复杂度。 你可以不使用额外空间来实现吗?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int singleNumber(vector &nums)
+ {
+ sort(nums.begin(), nums.end());
+ int res = 0;
+ int i = 0;
+ for (int j = 1; j < nums.size(); j++)
+ {
+ if (nums[j] != nums[i])
+ {
+ if (j - i == 1)
+ {
+ res = nums[i];
+ break;
+ }
+ else
+ {
+ i = j;
+ }
+ }
+ }
+ if (i == nums.size() - 1)
+ {
+ res = nums[i];
+ }
+ return res;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3c1ca34b11e3f5bcde7926790388a979c812b40a
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-ee972a8c3ad94ef4937a12be5da23849",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..a92f062db0a7f59ff4661c26b830e16d0432020e
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "504efc590cbb42e49757004b04b3e4e8",
+ "author": "csdn.net",
+ "keywords": "哈希表,链表",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..ff6fecccfc730f9f343ed12eecc8bfc021e7752d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/106.exercises/solution.md"
@@ -0,0 +1,153 @@
+# 复制带随机指针的链表
+
+给你一个长度为 n
的链表,每个节点包含一个额外增加的随机指针 random
,该指针可以指向链表中的任何节点或空节点。
+
+构造这个链表的 深拷贝。 深拷贝应该正好由 n
个 全新 节点组成,其中每个新节点的值都设为其对应的原节点的值。新节点的 next
指针和 random
指针也都应指向复制链表中的新节点,并使原链表和复制链表中的这些指针能够表示相同的链表状态。复制链表中的指针都不应指向原链表中的节点 。
+
+例如,如果原链表中有 X
和 Y
两个节点,其中 X.random --> Y
。那么在复制链表中对应的两个节点 x
和 y
,同样有 x.random --> y
。
+
+返回复制链表的头节点。
+
+用一个由 n
个节点组成的链表来表示输入/输出中的链表。每个节点用一个 [val, random_index]
表示:
+
+
+ val
:一个表示 Node.val
的整数。
+ random_index
:随机指针指向的节点索引(范围从 0
到 n-1
);如果不指向任何节点,则为 null
。
+
+
+你的代码 只 接受原链表的头节点 head
作为传入参数。
+
+
+
+示例 1:
+
+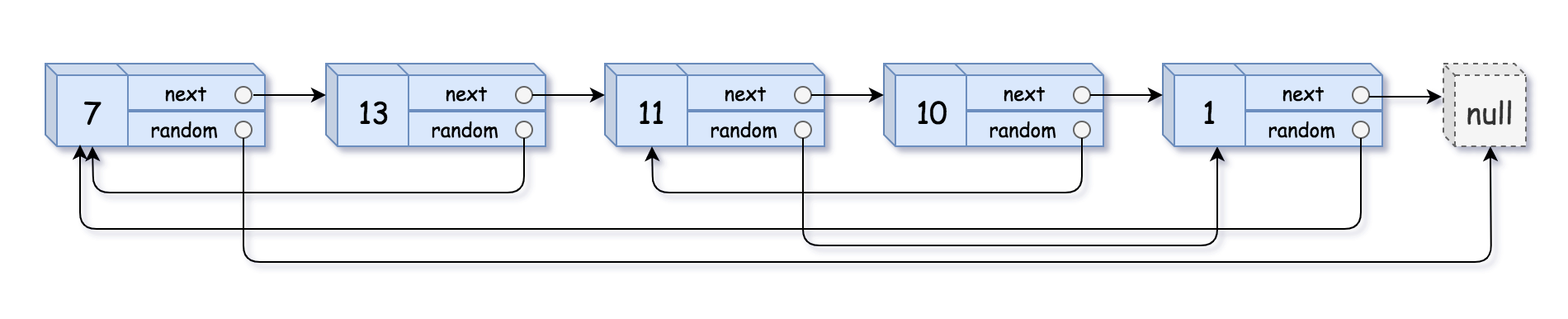
+
+
+输入:head = [[7,null],[13,0],[11,4],[10,2],[1,0]]
+输出:[[7,null],[13,0],[11,4],[10,2],[1,0]]
+
+
+示例 2:
+
+
+
+
+输入:head = [[1,1],[2,1]]
+输出:[[1,1],[2,1]]
+
+
+示例 3:
+
+
+
+
+输入:head = [[3,null],[3,0],[3,null]]
+输出:[[3,null],[3,0],[3,null]]
+
+
+示例 4:
+
+
+输入:head = []
+输出:[]
+解释:给定的链表为空(空指针),因此返回 null。
+
+
+
+
+提示:
+
+
+ 0 <= n <= 1000
+ -10000 <= Node.val <= 10000
+ Node.random
为空(null)或指向链表中的节点。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Node
+{
+public:
+ int val;
+ Node *next;
+ Node *random;
+
+ Node() {}
+
+ Node(int _val, Node *_next, Node *_random)
+ {
+ val = _val;
+ next = _next;
+ random = _random;
+ }
+};
+
+class Solution
+{
+public:
+ Node *copyRandomList(Node *head)
+ {
+ std::map node_map;
+ std::vector node_vec;
+ Node *ptr = head;
+ int i = 0;
+ while (ptr)
+ {
+
+ node_vec.push_back(new Node(ptr->val, NULL, NULL));
+ node_map[ptr] = i;
+ ptr = ptr->next;
+ i++;
+ }
+ node_vec.push_back(0);
+ ptr = head;
+ i = 0;
+ while (ptr)
+ {
+ node_vec[i]->next = node_vec[i + 1];
+ if (ptr->random)
+ {
+ int id = node_map[ptr->random];
+ node_vec[i]->random = node_vec[id];
+ }
+ ptr = ptr->next;
+ i++;
+ }
+ return node_vec[0];
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..22715667ed2861d4c54c44038be147000057c452
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-7ab771c2a0454a8d88061ebc100eb41e",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..d4371f9efd3e294ff834305328ea60cf5b586c04
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "a999e9a2aa0e4d8f945cfe6efca6fab9",
+ "author": "csdn.net",
+ "keywords": "字典树,记忆化搜索,哈希表,字符串,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9d33833a9a1e8b8b3d1323b49412da4da6f7137d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/107.exercises/solution.md"
@@ -0,0 +1,94 @@
+# 单词拆分
+
+给定一个非空字符串 s 和一个包含非空单词的列表 wordDict,判定 s 是否可以被空格拆分为一个或多个在字典中出现的单词。
+
+说明:
+
+
+ - 拆分时可以重复使用字典中的单词。
+ - 你可以假设字典中没有重复的单词。
+
+
+示例 1:
+
+输入: s = "leetcode", wordDict = ["leet", "code"]
+输出: true
+解释: 返回 true 因为 "leetcode" 可以被拆分成 "leet code"。
+
+
+示例 2:
+
+输入: s = "applepenapple", wordDict = ["apple", "pen"]
+输出: true
+解释: 返回 true 因为 "
applepenapple"
可以被拆分成 "
apple pen apple"
。
+ 注意你可以重复使用字典中的单词。
+
+
+示例 3:
+
+输入: s = "catsandog", wordDict = ["cats", "dog", "sand", "and", "cat"]
+输出: false
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool wordBreak(string s, vector &wordDict)
+ {
+ map tmp;
+ for (int i = 0; i < wordDict.size(); i++)
+ {
+ tmp[wordDict[i]]++;
+ }
+ int n = s.length();
+ vector res(n + 1, false);
+ res[0] = true;
+ for (int i = 0; i <= n; i++)
+ {
+ for (int j = 0; j < i; j++)
+ {
+ if (res[j] && tmp[s.substr(j, i - j)])
+ {
+ res[i] = true;
+ break;
+ }
+ }
+ }
+ return res[n];
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..8f8f54626d4517b98f5b1d486a20df9e567c8e54
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-c86e8718d6a14cbcb86586cfeded8a86",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..cb710842da2862ddca0ed998f6573a2c2b6e5cb5
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "c57e84df7922455895cfc253eff07450",
+ "author": "csdn.net",
+ "keywords": "哈希表,链表,双指针",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..d926ada5126c44532acd7b23a7d93420d2f33e2b
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/108.exercises/solution.md"
@@ -0,0 +1,125 @@
+# 环形链表 II
+
+给定一个链表,返回链表开始入环的第一个节点。 如果链表无环,则返回 null
。
+
+为了表示给定链表中的环,我们使用整数 pos
来表示链表尾连接到链表中的位置(索引从 0 开始)。 如果 pos
是 -1
,则在该链表中没有环。注意,pos
仅仅是用于标识环的情况,并不会作为参数传递到函数中。
+
+说明:不允许修改给定的链表。
+
+进阶:
+
+
+
+
+
+示例 1:
+
+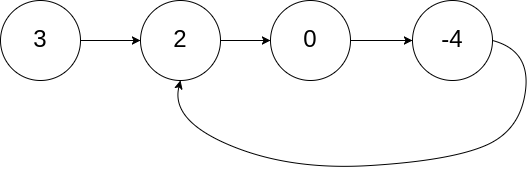
+
+
+输入:head = [3,2,0,-4], pos = 1
+输出:返回索引为 1 的链表节点
+解释:链表中有一个环,其尾部连接到第二个节点。
+
+
+示例 2:
+
+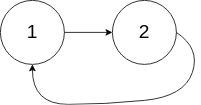
+
+
+输入:head = [1,2], pos = 0
+输出:返回索引为 0 的链表节点
+解释:链表中有一个环,其尾部连接到第一个节点。
+
+
+示例 3:
+
+
+
+
+输入:head = [1], pos = -1
+输出:返回 null
+解释:链表中没有环。
+
+
+
+
+提示:
+
+
+ - 链表中节点的数目范围在范围
[0, 104]
内
+ -105 <= Node.val <= 105
+ pos
的值为 -1
或者链表中的一个有效索引
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ ListNode *detectCycle(ListNode *head)
+ {
+ ListNode *slow, *fast, *p;
+ slow = fast = p = head;
+ while (fast && fast->next)
+ {
+ slow = slow->next;
+ fast = fast->next->next;
+
+ if (slow == fast)
+ {
+ while (p != slow)
+ {
+ p = p->next;
+ slow = slow->next;
+ }
+ return p;
+ }
+ }
+
+ return nullptr;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..a816e91c4defb30546019c35a0c56253168d8b11
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-0f4fa52ce6504716996db85e4c9e493a",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..72a3c8d807c0e291dde34f358d263fa30e8d656a
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "f5a81fc3e5a040ea8e0429a1e3cf941f",
+ "author": "csdn.net",
+ "keywords": "栈,递归,链表,双指针",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..7872033b6dec78880fb797e5e033a2135f516c0f
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/109.exercises/solution.md"
@@ -0,0 +1,121 @@
+# 重排链表
+
+给定一个单链表 L
的头节点 head
,单链表 L
表示为:
+
+ L0 → L1 → … → Ln-1 → Ln
+请将其重新排列后变为:
+
+L0 → Ln → L1 → Ln-1 → L2 → Ln-2 → …
+
+不能只是单纯的改变节点内部的值,而是需要实际的进行节点交换。
+
+
+
+示例 1:
+
+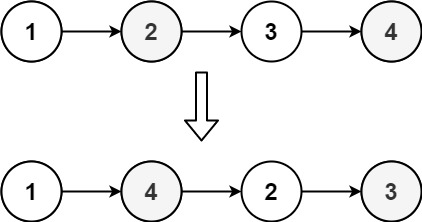
+
+
+输入: head = [1,2,3,4]
+输出: [1,4,2,3]
+
+示例 2:
+
+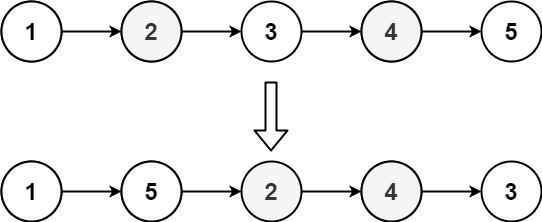
+
+
+输入: head = [1,2,3,4,5]
+输出: [1,5,2,4,3]
+
+
+
+提示:
+
+
+ - 链表的长度范围为
[1, 5 * 104]
+ 1 <= node.val <= 1000
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ void reorderList(ListNode *head)
+ {
+ ListNode *l1 = head, *l2 = head;
+ ListNode *tep = head;
+ int len = 0;
+ while (tep)
+ {
+ len++;
+ tep = tep->next;
+ }
+ if (len <= 2)
+ return;
+ int len1 = len / 2 + len % 2;
+ int len2 = len / 2;
+ for (int i = 0; i < len1 - 1; i++)
+ {
+ head = head->next;
+ }
+ l2 = head->next;
+ head->next = nullptr;
+ head = l1;
+ stack stk;
+ while (l2)
+ {
+ stk.push(l2);
+ l2 = l2->next;
+ }
+ while (!stk.empty())
+ {
+ tep = stk.top();
+ stk.pop();
+ tep->next = l1->next;
+ l1->next = tep;
+ l1 = tep->next;
+ }
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..0e9e000fdccd4f93c849371f9c63b419854f70fc
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-a60d5b2274744417bc065e034719adb5",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3cd7bf7d5ea20c2ba1411d4832b2b0ac47ff697c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "6925248d8d4b4971ace262cdfc82e08b",
+ "author": "csdn.net",
+ "keywords": "设计,哈希表,链表,双向链表",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..b8c24e16f256f896055e481bafefab3cd668c5ef
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/110.exercises/solution.md"
@@ -0,0 +1,150 @@
+# LRU 缓存机制
+
+
+
+
+
+
实现 LRUCache
类:
+
+
+ LRUCache(int capacity)
以正整数作为容量 capacity
初始化 LRU 缓存
+ int get(int key)
如果关键字 key
存在于缓存中,则返回关键字的值,否则返回 -1
。
+ void put(int key, int value)
如果关键字已经存在,则变更其数据值;如果关键字不存在,则插入该组「关键字-值」。当缓存容量达到上限时,它应该在写入新数据之前删除最久未使用的数据值,从而为新的数据值留出空间。
+
+
+
+
+
+
+进阶:你是否可以在 O(1)
时间复杂度内完成这两种操作?
+
+
+
+示例:
+
+
+输入
+["LRUCache", "put", "put", "get", "put", "get", "put", "get", "get", "get"]
+[[2], [1, 1], [2, 2], [1], [3, 3], [2], [4, 4], [1], [3], [4]]
+输出
+[null, null, null, 1, null, -1, null, -1, 3, 4]
+
+解释
+LRUCache lRUCache = new LRUCache(2);
+lRUCache.put(1, 1); // 缓存是 {1=1}
+lRUCache.put(2, 2); // 缓存是 {1=1, 2=2}
+lRUCache.get(1); // 返回 1
+lRUCache.put(3, 3); // 该操作会使得关键字 2 作废,缓存是 {1=1, 3=3}
+lRUCache.get(2); // 返回 -1 (未找到)
+lRUCache.put(4, 4); // 该操作会使得关键字 1 作废,缓存是 {4=4, 3=3}
+lRUCache.get(1); // 返回 -1 (未找到)
+lRUCache.get(3); // 返回 3
+lRUCache.get(4); // 返回 4
+
+
+
+
+提示:
+
+
+ 1 <= capacity <= 3000
+ 0 <= key <= 10000
+ 0 <= value <= 105
+ - 最多调用
2 * 105
次 get
和 put
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class LRUCache
+{
+private:
+ int _cap;
+
+ list> cache;
+
+ unordered_map>::iterator> umap;
+
+public:
+ LRUCache(int capacity)
+ {
+ _cap = capacity;
+ }
+
+ int get(int key)
+ {
+ auto iter = umap.find(key);
+ if (iter == umap.end())
+ return -1;
+
+ pair kv = *umap[key];
+ cache.erase(umap[key]);
+ cache.push_front(kv);
+ umap[key] = cache.begin();
+
+ return kv.second;
+ }
+
+ void put(int key, int value)
+ {
+ auto iter = umap.find(key);
+ if (iter != umap.end())
+ {
+
+ cache.erase(umap[key]);
+ cache.push_front(make_pair(key, value));
+ umap[key] = cache.begin();
+
+ return;
+ }
+
+ if (cache.size() == _cap)
+ {
+
+ auto iter = cache.back();
+ umap.erase(iter.first);
+ cache.pop_back();
+
+ cache.push_front(make_pair(key, value));
+ umap[key] = cache.begin();
+ }
+ else
+ {
+ cache.push_front(make_pair(key, value));
+ umap[key] = cache.begin();
+ }
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..1eca10031248df8b48d6b18c7702b05394f96cf9
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-36ed7137f34d4fd2b2b5e101ce9756bb",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ae2c83a827f7a1377e0cc81c4c2960918629b4bf
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "bc206a68afdd49898d978634851b9566",
+ "author": "csdn.net",
+ "keywords": "链表,排序",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..cd8e1e2b0a50a6d67db52790c849b328b4f08bc1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/111.exercises/solution.md"
@@ -0,0 +1,96 @@
+# 对链表进行插入排序
+
+对链表进行插入排序。
+
+
+插入排序的动画演示如上。从第一个元素开始,该链表可以被认为已经部分排序(用黑色表示)。
+每次迭代时,从输入数据中移除一个元素(用红色表示),并原地将其插入到已排好序的链表中。
+
+
+
+插入排序算法:
+
+
+ - 插入排序是迭代的,每次只移动一个元素,直到所有元素可以形成一个有序的输出列表。
+ - 每次迭代中,插入排序只从输入数据中移除一个待排序的元素,找到它在序列中适当的位置,并将其插入。
+ - 重复直到所有输入数据插入完为止。
+
+
+
+
+示例 1:
+
+输入: 4->2->1->3
+输出: 1->2->3->4
+
+
+示例 2:
+
+输入: -1->5->3->4->0
+输出: -1->0->3->4->5
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ ListNode *insertionSortList(ListNode *head)
+ {
+ ListNode *dummy = new ListNode(0, head);
+ ListNode
+ *prev = dummy,
+ *lastSorted = head,
+ *curr = head->next;
+
+ while (curr)
+ {
+ lastSorted->next = curr->next;
+ curr->next = prev->next;
+ prev->next = curr;
+ curr = lastSorted->next;
+ }
+ return dummy->next;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7e56dc7033d3f642daa7cbb47d01aa3961b5c93d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-0eb990da86e340998c215d3a1d8aa859",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3e630df8ba1a13763e5c1ce5682d4ddec1096866
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "0f14c22af5474598bd0f688223cb0c68",
+ "author": "csdn.net",
+ "keywords": "链表,双指针,分治,排序,归并排序",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..517ce6fbefb4a2b49be2e2ba215c58a23f8f7a4b
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/112.exercises/solution.md"
@@ -0,0 +1,132 @@
+# 排序链表
+
+给你链表的头结点 head
,请将其按 升序 排列并返回 排序后的链表 。
+
+进阶:
+
+
+ - 你可以在
O(n log n)
时间复杂度和常数级空间复杂度下,对链表进行排序吗?
+
+
+
+
+示例 1:
+
+
+输入:head = [4,2,1,3]
+输出:[1,2,3,4]
+
+
+示例 2:
+
+
+输入:head = [-1,5,3,4,0]
+输出:[-1,0,3,4,5]
+
+
+示例 3:
+
+
+输入:head = []
+输出:[]
+
+
+
+
+提示:
+
+
+ - 链表中节点的数目在范围
[0, 5 * 104]
内
+ -105 <= Node.val <= 105
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ ListNode *sortList(ListNode *head)
+ {
+ return mergesort(head);
+ }
+ ListNode *mergesort(ListNode *node)
+ {
+ if (!node || !node->next)
+ return node;
+ ListNode *fast = node;
+ ListNode *slow = node;
+ ListNode *brek = node;
+ while (fast && fast->next)
+ {
+ fast = fast->next->next;
+ brek = slow;
+ slow = slow->next;
+ }
+ brek->next = nullptr;
+ ListNode *l1 = mergesort(node);
+ ListNode *l2 = mergesort(slow);
+ return merge(l1, l2);
+ }
+ ListNode *merge(ListNode *l1, ListNode *l2)
+ {
+ if (l1 == NULL)
+ {
+ return l2;
+ }
+ if (l2 == NULL)
+ {
+ return l1;
+ }
+ if (l1->val < l2->val)
+ {
+ l1->next = merge(l1->next, l2);
+ return l1;
+ }
+ else
+ {
+ l2->next = merge(l2->next, l1);
+ return l2;
+ }
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..db69f78c170f7bbfd71190fbf0679194892e579f
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-f69b5024f7ea4a72811393706d30e524",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..6c92d05e6ef833742ad573a010c6f11f2ea8a0e9
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "d6df7d868e7648678eb11fff0e8eda92",
+ "author": "csdn.net",
+ "keywords": "栈,数组,数学",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..a6d1f9be85ca18aaef3c67d4c8cf421ab6dad598
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/113.exercises/solution.md"
@@ -0,0 +1,144 @@
+# 逆波兰表达式求值
+
+根据 逆波兰表示法,求表达式的值。
+
+有效的算符包括 +
、-
、*
、/
。每个运算对象可以是整数,也可以是另一个逆波兰表达式。
+
+
+
+说明:
+
+
+ - 整数除法只保留整数部分。
+ - 给定逆波兰表达式总是有效的。换句话说,表达式总会得出有效数值且不存在除数为 0 的情况。
+
+
+
+
+示例 1:
+
+
+输入:tokens = ["2","1","+","3","*"]
+输出:9
+解释:该算式转化为常见的中缀算术表达式为:((2 + 1) * 3) = 9
+
+
+示例 2:
+
+
+输入:tokens = ["4","13","5","/","+"]
+输出:6
+解释:该算式转化为常见的中缀算术表达式为:(4 + (13 / 5)) = 6
+
+
+示例 3:
+
+
+输入:tokens = ["10","6","9","3","+","-11","*","/","*","17","+","5","+"]
+输出:22
+解释:
+该算式转化为常见的中缀算术表达式为:
+ ((10 * (6 / ((9 + 3) * -11))) + 17) + 5
+= ((10 * (6 / (12 * -11))) + 17) + 5
+= ((10 * (6 / -132)) + 17) + 5
+= ((10 * 0) + 17) + 5
+= (0 + 17) + 5
+= 17 + 5
+= 22
+
+
+
+提示:
+
+
+ 1 <= tokens.length <= 104
+ tokens[i]
要么是一个算符("+"
、"-"
、"*"
或 "/"
),要么是一个在范围 [-200, 200]
内的整数
+
+
+
+
+逆波兰表达式:
+
+逆波兰表达式是一种后缀表达式,所谓后缀就是指算符写在后面。
+
+
+ - 平常使用的算式则是一种中缀表达式,如
( 1 + 2 ) * ( 3 + 4 )
。
+ - 该算式的逆波兰表达式写法为
( ( 1 2 + ) ( 3 4 + ) * )
。
+
+
+逆波兰表达式主要有以下两个优点:
+
+
+ - 去掉括号后表达式无歧义,上式即便写成
1 2 + 3 4 + *
也可以依据次序计算出正确结果。
+ - 适合用栈操作运算:遇到数字则入栈;遇到算符则取出栈顶两个数字进行计算,并将结果压入栈中。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int evalRPN(vector &tokens)
+ {
+ stack num;
+ for (int i = 0; i < tokens.size(); ++i)
+ {
+ if (tokens[i] == "+" || tokens[i] == "-" || tokens[i] == "*" || tokens[i] == "/")
+ {
+ int j;
+ int a = num.top();
+ num.pop();
+ int b = num.top();
+ num.pop();
+ if (tokens[i] == "+")
+ j = b + a;
+ else if (tokens[i] == "-")
+ j = b - a;
+ else if (tokens[i] == "*")
+ j = b * a;
+ else
+ j = b / a;
+ num.push(j);
+ }
+ else
+ {
+ num.push(stoi(tokens[i]));
+ }
+ }
+ return num.top();
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..18273cb0d618c91704eabe4d5333dc03aceae889
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-05046db437fa4f03bd4e5f082a388673",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..4d775285c7aaaf7804f01157742760c6982393de
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "cd5c9fb0e79a4ff6814d13a0db4ce918",
+ "author": "csdn.net",
+ "keywords": "双指针,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..4c58948bd19e52958d3c9c068314af2e3bbd4bd7
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/114.exercises/solution.md"
@@ -0,0 +1,161 @@
+# 翻转字符串里的单词
+
+给你一个字符串 s
,逐个翻转字符串中的所有 单词 。
+
+单词 是由非空格字符组成的字符串。s
中使用至少一个空格将字符串中的 单词 分隔开。
+
+请你返回一个翻转 s
中单词顺序并用单个空格相连的字符串。
+
+说明:
+
+
+ - 输入字符串
s
可以在前面、后面或者单词间包含多余的空格。
+ - 翻转后单词间应当仅用一个空格分隔。
+ - 翻转后的字符串中不应包含额外的空格。
+
+
+
+
+示例 1:
+
+
+输入:s = "the sky is blue
"
+输出:"blue is sky the
"
+
+
+示例 2:
+
+
+输入:s = " hello world "
+输出:"world hello"
+解释:输入字符串可以在前面或者后面包含多余的空格,但是翻转后的字符不能包括。
+
+
+示例 3:
+
+
+输入:s = "a good example"
+输出:"example good a"
+解释:如果两个单词间有多余的空格,将翻转后单词间的空格减少到只含一个。
+
+
+示例 4:
+
+
+输入:s = " Bob Loves Alice "
+输出:"Alice Loves Bob"
+
+
+示例 5:
+
+
+输入:s = "Alice does not even like bob"
+输出:"bob like even not does Alice"
+
+
+
+
+提示:
+
+
+ 1 <= s.length <= 104
+ s
包含英文大小写字母、数字和空格 ' '
+ s
中 至少存在一个 单词
+
+
+
+
+
+
+进阶:
+
+
+ - 请尝试使用
O(1)
额外空间复杂度的原地解法。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ string reverseWords(string s)
+ {
+ splitStr(s);
+ return joinStr();
+ }
+
+private:
+ void splitStr(string s)
+ {
+ if (s == "")
+ return;
+ int len = s.length();
+ string tmp = "";
+ for (int i = 0; i < len; i++)
+ {
+ if (s[i] == ' ' && tmp != "")
+ {
+ myStack.push(tmp);
+ tmp = "";
+ continue;
+ }
+ if (s[i] == ' ')
+ continue;
+ tmp += s[i];
+ }
+ if (tmp != "")
+ myStack.push(tmp);
+ return;
+ }
+
+ string joinStr()
+ {
+ if (myStack.empty())
+ return "";
+ string s = "";
+ while (!myStack.empty())
+ {
+ s += myStack.top();
+ s += " ";
+ myStack.pop();
+ }
+ return s.substr(0, s.length() - 1);
+ }
+
+ stack myStack;
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..92b4d313218aea0df694d973fbcc6388f7bbd4ff
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-e33c5f27e8214636a42bc8cf443ac7dc",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e8ceb43bfea6c60e66cb027bd1036b5d97ff04d2
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "5aef5bb4e105434789d7066ad7b6d9c5",
+ "author": "csdn.net",
+ "keywords": "数组,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9bbd637cf9b2c929e18d1395e8ce7d52b8db4b1e
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/115.exercises/solution.md"
@@ -0,0 +1,74 @@
+# 乘积最大子数组
+
+给你一个整数数组 nums
,请你找出数组中乘积最大的连续子数组(该子数组中至少包含一个数字),并返回该子数组所对应的乘积。
+
+
+
+示例 1:
+
+输入: [2,3,-2,4]
+输出: 6
+解释: 子数组 [2,3] 有最大乘积 6。
+
+
+示例 2:
+
+输入: [-2,0,-1]
+输出: 0
+解释: 结果不能为 2, 因为 [-2,-1] 不是子数组。
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int maxProduct(vector &nums)
+ {
+ int ans = -10000000;
+ int n = nums.size();
+ int max1 = 1, min1 = 1, mx, mn;
+ for (int i = 0; i < n; i++)
+ {
+ mx = max1;
+ mn = min1;
+ max1 = max(mx * nums[i], max(nums[i], mn * nums[i]));
+ min1 = min(mn * nums[i], min(nums[i], mx * nums[i]));
+ ans = max(max1, ans);
+ }
+ return ans;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..0e4e10875e0d4b152a5e722b625296c5a05d6e1c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-d7d3abfcdce548ab912b7845ab999a96",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..501ceb169e7c656128cb547bf99e7b34ff2da593
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "5e95ff14e3e34ca8b0c3e643ce327dd3",
+ "author": "csdn.net",
+ "keywords": "数组,二分查找",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..b6e6404fe90e691802f0a68783768952456f25e5
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/116.exercises/solution.md"
@@ -0,0 +1,104 @@
+# 寻找旋转排序数组中的最小值
+
+已知一个长度为 n
的数组,预先按照升序排列,经由 1
到 n
次 旋转 后,得到输入数组。例如,原数组 nums = [0,1,2,4,5,6,7]
在变化后可能得到:
+
+ - 若旋转
4
次,则可以得到 [4,5,6,7,0,1,2]
+ - 若旋转
7
次,则可以得到 [0,1,2,4,5,6,7]
+
+
+注意,数组 [a[0], a[1], a[2], ..., a[n-1]]
旋转一次 的结果为数组 [a[n-1], a[0], a[1], a[2], ..., a[n-2]]
。
+
+给你一个元素值 互不相同 的数组 nums
,它原来是一个升序排列的数组,并按上述情形进行了多次旋转。请你找出并返回数组中的 最小元素 。
+
+
+
+示例 1:
+
+
+输入:nums = [3,4,5,1,2]
+输出:1
+解释:原数组为 [1,2,3,4,5] ,旋转 3 次得到输入数组。
+
+
+示例 2:
+
+
+输入:nums = [4,5,6,7,0,1,2]
+输出:0
+解释:原数组为 [0,1,2,4,5,6,7] ,旋转 4 次得到输入数组。
+
+
+示例 3:
+
+
+输入:nums = [11,13,15,17]
+输出:11
+解释:原数组为 [11,13,15,17] ,旋转 4 次得到输入数组。
+
+
+
+
+提示:
+
+
+ n == nums.length
+ 1 <= n <= 5000
+ -5000 <= nums[i] <= 5000
+ nums
中的所有整数 互不相同
+ nums
原来是一个升序排序的数组,并进行了 1
至 n
次旋转
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int findMin(vector &nums)
+ {
+ int left = 0, right = nums.size() - 1;
+ int mid = (left + right) / 2;
+ while (left < right)
+ {
+ mid = (left + right) / 2;
+ if (nums[mid] > nums[right])
+ left = mid + 1;
+ else
+ right = mid;
+ }
+ return nums[left];
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..30b384ab8bc9e65a9b4c16ccbfddc1054694f7d1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-dd9ab1f390244fc09b52747485de90a5",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..13a3fefdd2a52181f131514bf76a57a92797ea49
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "b64fe0d514344234a872ab6ccb13bb92",
+ "author": "csdn.net",
+ "keywords": "数组,二分查找",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..355c99ca11d4bdc24bf2611bb9a5ef49cc839fd7
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/117.exercises/solution.md"
@@ -0,0 +1,101 @@
+# 寻找峰值
+
+峰值元素是指其值严格大于左右相邻值的元素。
+
+给你一个整数数组 nums
,找到峰值元素并返回其索引。数组可能包含多个峰值,在这种情况下,返回 任何一个峰值 所在位置即可。
+
+你可以假设 nums[-1] = nums[n] = -∞
。
+
+你必须实现时间复杂度为 O(log n)
的算法来解决此问题。
+
+
+
+示例 1:
+
+
+输入:nums = [1,2,3,1]
+输出:2
+解释:3 是峰值元素,你的函数应该返回其索引 2。
+
+示例 2:
+
+
+输入:nums = [
1,2,1,3,5,6,4]
+输出:1 或 5
+解释:你的函数可以返回索引 1,其峰值元素为 2;
+ 或者返回索引 5, 其峰值元素为 6。
+
+
+
+
+提示:
+
+
+ 1 <= nums.length <= 1000
+ -231 <= nums[i] <= 231 - 1
+ - 对于所有有效的
i
都有 nums[i] != nums[i + 1]
+
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int findPeakElement(vector &nums)
+ {
+ int l = 0, r = nums.size() - 1;
+ while (l < r)
+ {
+ int mid = l + (r - l) / 2;
+ if (nums[mid] > nums[mid + 1])
+ {
+ if (mid == 0 || nums[mid] > nums[mid - 1])
+ return mid;
+ else
+ r = mid;
+ }
+ else
+ {
+ if (mid + 1 == nums.size() - 1 || nums[mid + 1] > nums[mid + 2])
+ return mid + 1;
+ else
+ l = mid + 1;
+ }
+ }
+ return l;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..29da4c31aac15f4879dc9f808829068b40411906
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-5e49fb70f7ba4fa581a2b1e1aa9afeca",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ce89d688b6761b606d4be0770152f13d09d055a3
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "18a0dcf78cd549869b8437e46fba25bf",
+ "author": "csdn.net",
+ "keywords": "双指针,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..2fe69070c906edb350411139b58de6e28b0e0d6d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/118.exercises/solution.md"
@@ -0,0 +1,142 @@
+# 比较版本号
+
+给你两个版本号 version1
和 version2
,请你比较它们。
+
+版本号由一个或多个修订号组成,各修订号由一个 '.'
连接。每个修订号由 多位数字 组成,可能包含 前导零 。每个版本号至少包含一个字符。修订号从左到右编号,下标从 0 开始,最左边的修订号下标为 0 ,下一个修订号下标为 1 ,以此类推。例如,2.5.33
和 0.1
都是有效的版本号。
+
+比较版本号时,请按从左到右的顺序依次比较它们的修订号。比较修订号时,只需比较 忽略任何前导零后的整数值 。也就是说,修订号 1
和修订号 001
相等 。如果版本号没有指定某个下标处的修订号,则该修订号视为 0
。例如,版本 1.0
小于版本 1.1
,因为它们下标为 0
的修订号相同,而下标为 1
的修订号分别为 0
和 1
,0 < 1
。
+
+返回规则如下:
+
+
+ - 如果
version1 > version2
返回 1
,
+ - 如果
version1 < version2
返回 -1
,
+ - 除此之外返回
0
。
+
+
+
+
+示例 1:
+
+
+输入:version1 = "1.01", version2 = "1.001"
+输出:0
+解释:忽略前导零,"01" 和 "001" 都表示相同的整数 "1"
+
+
+示例 2:
+
+
+输入:version1 = "1.0", version2 = "1.0.0"
+输出:0
+解释:version1 没有指定下标为 2 的修订号,即视为 "0"
+
+
+示例 3:
+
+
+输入:version1 = "0.1", version2 = "1.1"
+输出:-1
+解释:version1 中下标为 0 的修订号是 "0",version2 中下标为 0 的修订号是 "1" 。0 < 1,所以 version1 < version2
+
+
+示例 4:
+
+
+输入:version1 = "1.0.1", version2 = "1"
+输出:1
+
+
+示例 5:
+
+
+输入:version1 = "7.5.2.4", version2 = "7.5.3"
+输出:-1
+
+
+
+
+提示:
+
+
+ 1 <= version1.length, version2.length <= 500
+ version1
和 version2
仅包含数字和 '.'
+ version1
和 version2
都是 有效版本号
+ version1
和 version2
的所有修订号都可以存储在 32 位整数 中
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int compareVersion(string version1, string version2)
+ {
+ int val1, val2;
+ int idx1 = 0, idx2 = 0;
+ while (idx1 < version1.length() || idx2 < version2.length())
+ {
+ val1 = 0;
+ while (idx1 < version1.length())
+ {
+ if (version1[idx1] == '.')
+ {
+ ++idx1;
+ break;
+ }
+ val1 = val1 * 10 + (version1[idx1] - '0');
+ idx1++;
+ }
+
+ val2 = 0;
+ while (idx2 < version2.length())
+ {
+ if (version2[idx2] == '.')
+ {
+ idx2++;
+ break;
+ }
+ val2 = val2 * 10 + (version2[idx2] - '0');
+ idx2++;
+ }
+ if (val1 > val2)
+ return 1;
+ if (val1 < val2)
+ return -1;
+ }
+ return 0;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..93a37bbadba2b36761148fead8b541ac39760285
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-fac5c17f457d4cf4b5cf2636c80ffa31",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..44a339c4b1ee63e9970472d413ead011a32f5c59
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "1d66b4dc149845938d464a2b38b724f2",
+ "author": "csdn.net",
+ "keywords": "哈希表,数学,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..0df460df108875a8eb98ee69ffa1c68996be4c06
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/119.exercises/solution.md"
@@ -0,0 +1,130 @@
+# 分数到小数
+
+给定两个整数,分别表示分数的分子 numerator
和分母 denominator
,以 字符串形式返回小数 。
+
+如果小数部分为循环小数,则将循环的部分括在括号内。
+
+如果存在多个答案,只需返回 任意一个 。
+
+对于所有给定的输入,保证 答案字符串的长度小于 104
。
+
+
+
+示例 1:
+
+
+输入:numerator = 1, denominator = 2
+输出:"0.5"
+
+
+示例 2:
+
+
+输入:numerator = 2, denominator = 1
+输出:"2"
+
+
+示例 3:
+
+
+输入:numerator = 2, denominator = 3
+输出:"0.(6)"
+
+
+示例 4:
+
+
+输入:numerator = 4, denominator = 333
+输出:"0.(012)"
+
+
+示例 5:
+
+
+输入:numerator = 1, denominator = 5
+输出:"0.2"
+
+
+
+
+提示:
+
+
+ -231 <= numerator, denominator <= 231 - 1
+ denominator != 0
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ string fractionToDecimal(int numerator, int denominator)
+ {
+ if (denominator == 0)
+ return "";
+ string ans;
+ if ((numerator > 0 && denominator < 0) || (numerator < 0 && denominator > 0))
+ ans = "-";
+ long long num = abs(static_cast(numerator));
+ long long den = abs(static_cast(denominator));
+ long long quo = num / den;
+ long long rem = num % den;
+
+ ans = ans + to_string(quo);
+ if (!rem)
+ return ans;
+
+ ans += ".";
+ unordered_map u_m;
+ string s = "";
+ int pos = 0;
+ while (rem)
+ {
+ if (u_m.find(rem) != u_m.end())
+ {
+ s.insert(u_m[rem], "(");
+ s += ")";
+ return ans + s;
+ }
+ u_m[rem] = pos++;
+ s += to_string((rem * 10) / den);
+ rem = (rem * 10) % den;
+ }
+ return ans + s;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..5a516f1a7eb2acbea0814536dfdd2d95b744dccb
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-4a38b31d688641b9843b28b4f373d6e2",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..2239ff4f9945d7a5980c8e839b2fefc6eebad7c4
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "1de12a0f075f46649a65be099ca6af08",
+ "author": "csdn.net",
+ "keywords": "栈,树,设计,二叉搜索树,二叉树,迭代器",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..1e7b0621453a107ce7105cb075967d0a66f0d4c2
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/120.exercises/solution.md"
@@ -0,0 +1,147 @@
+# 二叉搜索树迭代器
+
+实现一个二叉搜索树迭代器类BSTIterator
,表示一个按中序遍历二叉搜索树(BST)的迭代器:
+
+
+
+ BSTIterator(TreeNode root)
初始化 BSTIterator
类的一个对象。BST 的根节点 root
会作为构造函数的一部分给出。指针应初始化为一个不存在于 BST 中的数字,且该数字小于 BST 中的任何元素。
+ boolean hasNext()
如果向指针右侧遍历存在数字,则返回 true
;否则返回 false
。
+ int next()
将指针向右移动,然后返回指针处的数字。
+
+
+
注意,指针初始化为一个不存在于 BST 中的数字,所以对 next()
的首次调用将返回 BST 中的最小元素。
+
+
+
+你可以假设 next()
调用总是有效的,也就是说,当调用 next()
时,BST 的中序遍历中至少存在一个下一个数字。
+
+
+
+示例:
+
+
+输入
+["BSTIterator", "next", "next", "hasNext", "next", "hasNext", "next", "hasNext", "next", "hasNext"]
+[[[7, 3, 15, null, null, 9, 20]], [], [], [], [], [], [], [], [], []]
+输出
+[null, 3, 7, true, 9, true, 15, true, 20, false]
+
+解释
+BSTIterator bSTIterator = new BSTIterator([7, 3, 15, null, null, 9, 20]);
+bSTIterator.next(); // 返回 3
+bSTIterator.next(); // 返回 7
+bSTIterator.hasNext(); // 返回 True
+bSTIterator.next(); // 返回 9
+bSTIterator.hasNext(); // 返回 True
+bSTIterator.next(); // 返回 15
+bSTIterator.hasNext(); // 返回 True
+bSTIterator.next(); // 返回 20
+bSTIterator.hasNext(); // 返回 False
+
+
+
+
+提示:
+
+
+ - 树中节点的数目在范围
[1, 105]
内
+ 0 <= Node.val <= 106
+ - 最多调用
105
次 hasNext
和 next
操作
+
+
+
+
+进阶:
+
+
+ - 你可以设计一个满足下述条件的解决方案吗?
next()
和 hasNext()
操作均摊时间复杂度为 O(1)
,并使用 O(h)
内存。其中 h
是树的高度。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class BSTIterator
+{
+public:
+ BSTIterator(TreeNode *root)
+ {
+
+ for (; root != nullptr; root = root->left)
+ {
+ sti_.push(root);
+ }
+ }
+
+ /** @return the next smallest number */
+ int next()
+ {
+
+ TreeNode *smallest = sti_.top();
+ sti_.pop();
+ int val = smallest->val;
+
+ smallest = smallest->right;
+ for (; smallest != nullptr; smallest = smallest->left)
+ {
+ sti_.push(smallest);
+ }
+ return val;
+ }
+
+ /** @return whether we have a next smallest number */
+ bool hasNext()
+ {
+ return !sti_.empty();
+ }
+
+private:
+ stack sti_;
+};
+
+/**
+ * Your BSTIterator object will be instantiated and called as such:
+ * BSTIterator* obj = new BSTIterator(root);
+ * int param_1 = obj->next();
+ * bool param_2 = obj->hasNext();
+ */
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..6536bbc917fd7b9ac06f9c858eb85eb74b1fcf3d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-734b902304404565abebe151f9f77153",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..626c52cc6ca374b2666bacea0afb5c1bc381e99d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "6a83c0e417c64208bca61e383ecaa225",
+ "author": "csdn.net",
+ "keywords": "贪心,字符串,排序",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..64b10c112350e317610f777cfb12a06200c9ea78
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/121.exercises/solution.md"
@@ -0,0 +1,113 @@
+# 最大数
+
+给定一组非负整数 nums
,重新排列每个数的顺序(每个数不可拆分)使之组成一个最大的整数。
+
+注意:输出结果可能非常大,所以你需要返回一个字符串而不是整数。
+
+
+
+示例 1:
+
+
+输入:
nums = [10,2]
+输出:"210"
+
+示例 2:
+
+
+输入:
nums = [3,30,34,5,9]
+输出:"9534330"
+
+
+示例 3:
+
+
+输入:
nums = [1]
+输出:"1"
+
+
+示例 4:
+
+
+输入:
nums = [10]
+输出:"10"
+
+
+
+
+提示:
+
+
+ 1 <= nums.length <= 100
+ 0 <= nums[i] <= 109
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+bool compare(string a, string b)
+{
+ string s1 = a + b;
+ string s2 = b + a;
+ return s1 > s2;
+}
+
+class Solution
+{
+public:
+ string largestNumber(vector &nums)
+ {
+ string res = "";
+ int n = nums.size();
+ vector tmp;
+ for (int i = 0; i < n; i++)
+ {
+ tmp.push_back(to_string(nums[i]));
+ }
+ sort(tmp.begin(), tmp.end(), compare);
+ for (int i = 0; i < tmp.size(); i++)
+ {
+ res += tmp[i];
+ }
+ if ('0' == res[0])
+ {
+ return "0";
+ }
+ else
+ {
+ return res;
+ }
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b0ac088f11bd06ce5f6d95fdabeacb4c4fabc2b6
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-6bfbac883a8f424d89c5d4bd00c1166a",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f46b74e8ad1156cf9771c7901319f6159b901e00
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "f2f407f63a6641d8a6a6e98f48b5eb14",
+ "author": "csdn.net",
+ "keywords": "位运算,哈希表,字符串,滑动窗口,哈希函数,滚动哈希",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..6337f97aa0136f22cd2ff66a584e9704cd0154a0
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/122.exercises/solution.md"
@@ -0,0 +1,97 @@
+# 重复的DNA序列
+
+所有 DNA 都由一系列缩写为 'A'
,'C'
,'G'
和 'T'
的核苷酸组成,例如:"ACGAATTCCG"
。在研究 DNA 时,识别 DNA 中的重复序列有时会对研究非常有帮助。
+
+编写一个函数来找出所有目标子串,目标子串的长度为 10,且在 DNA 字符串 s
中出现次数超过一次。
+
+
+
+示例 1:
+
+
+输入:s = "AAAAACCCCCAAAAACCCCCCAAAAAGGGTTT"
+输出:["AAAAACCCCC","CCCCCAAAAA"]
+
+
+示例 2:
+
+
+输入:s = "AAAAAAAAAAAAA"
+输出:["AAAAAAAAAA"]
+
+
+
+
+提示:
+
+
+ 0 <= s.length <= 105
+ s[i]
为 'A'
、'C'
、'G'
或 'T'
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ vector findRepeatedDnaSequences(string s)
+ {
+ std::map word_map;
+ std::vector result;
+ for (int i = 0; i < s.length(); i++)
+ {
+ std::string word = s.substr(i, 10);
+ if (word_map.find(word) != word_map.end())
+ {
+ word_map[word] += 1;
+ }
+ else
+ {
+ word_map[word] = 1;
+ }
+ }
+ std::map::iterator it;
+ for (it = word_map.begin(); it != word_map.end(); it++)
+ {
+ if (it->second > 1)
+ {
+ result.push_back(it->first);
+ }
+ }
+ return result;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7fd596725b70fcf3dc9a36b01456bf7ab7487771
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-617ecf41c10541368e4d2585d3a5f77c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f9766c310e58bf2576b91c0ab4f72a52af0275e0
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "e5f17936775e4f7fb4aee5b522a5153e",
+ "author": "csdn.net",
+ "keywords": "数组,数学,双指针",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..e014eb116a4414292ee17ec3f661b388925c5fde
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/123.exercises/solution.md"
@@ -0,0 +1,106 @@
+# 旋转数组
+
+给定一个数组,将数组中的元素向右移动 k
个位置,其中 k
是非负数。
+
+
+
+进阶:
+
+
+ - 尽可能想出更多的解决方案,至少有三种不同的方法可以解决这个问题。
+ - 你可以使用空间复杂度为 O(1) 的 原地 算法解决这个问题吗?
+
+
+
+
+示例 1:
+
+
+输入: nums = [1,2,3,4,5,6,7], k = 3
+输出: [5,6,7,1,2,3,4]
+解释:
+向右旋转 1 步: [7,1,2,3,4,5,6]
+向右旋转 2 步: [6,7,1,2,3,4,5]
+
向右旋转 3 步: [5,6,7,1,2,3,4]
+
+
+示例 2:
+
+
+输入:nums = [-1,-100,3,99], k = 2
+输出:[3,99,-1,-100]
+解释:
+向右旋转 1 步: [99,-1,-100,3]
+向右旋转 2 步: [3,99,-1,-100]
+
+
+
+提示:
+
+
+ 1 <= nums.length <= 2 * 104
+ -231 <= nums[i] <= 231 - 1
+ 0 <= k <= 105
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ void rotate(vector &nums, int k)
+ {
+ if (nums.empty())
+ {
+ return;
+ }
+ int length = nums.size();
+ k %= length;
+ while (k--)
+ {
+ int temp = nums[length - 1];
+ for (int i = length - 1; i > 0; i--)
+ {
+ nums[i] = nums[i - 1];
+ }
+ nums[0] = temp;
+ }
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..06a81890ededab529e8bb065e16d9e8409001e24
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-a4304e9fcd084439be358b1e58347100",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..d72ff744b483d982980027e4114217ddff9f58b9
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "91c2d236f2624016849c993a4c798f98",
+ "author": "csdn.net",
+ "keywords": "数组,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..8262deacb9d1162f19443057873d20181b735b62
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/124.exercises/solution.md"
@@ -0,0 +1,91 @@
+# 打家劫舍
+
+你是一个专业的小偷,计划偷窃沿街的房屋。每间房内都藏有一定的现金,影响你偷窃的唯一制约因素就是相邻的房屋装有相互连通的防盗系统,如果两间相邻的房屋在同一晚上被小偷闯入,系统会自动报警。
+
+给定一个代表每个房屋存放金额的非负整数数组,计算你 不触动警报装置的情况下 ,一夜之内能够偷窃到的最高金额。
+
+
+
+示例 1:
+
+
+输入:[1,2,3,1]
+输出:4
+解释:偷窃 1 号房屋 (金额 = 1) ,然后偷窃 3 号房屋 (金额 = 3)。
+ 偷窃到的最高金额 = 1 + 3 = 4 。
+
+示例 2:
+
+
+输入:[2,7,9,3,1]
+输出:12
+解释:偷窃 1 号房屋 (金额 = 2), 偷窃 3 号房屋 (金额 = 9),接着偷窃 5 号房屋 (金额 = 1)。
+ 偷窃到的最高金额 = 2 + 9 + 1 = 12 。
+
+
+
+
+提示:
+
+
+ 1 <= nums.length <= 100
+ 0 <= nums[i] <= 400
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int rob(vector &nums)
+ {
+ int n = nums.size();
+ if (n == 0)
+ {
+ return 0;
+ }
+
+ vector f(n + 1);
+ f[1] = nums[0];
+ for (int i = 2; i <= n; ++i)
+ {
+ f[i] = max(f[i - 1], f[i - 2] + nums[i - 1]);
+ }
+
+ return f[n];
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..1848bc694c72e363a499f64eb2cb5022bfd5f050
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-96ae9dfe598347d1ab79aa2750046db6",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..0ba241d463dd39584ac357413c5ab4d5cb13d3b2
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "fde5bfc5ffcc4372b5b173f3f8128454",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..a98d76cd2d552bbc35274b947249ba099accf6fd
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/125.exercises/solution.md"
@@ -0,0 +1,116 @@
+# 二叉树的右视图
+
+给定一个二叉树的 根节点 root
,想象自己站在它的右侧,按照从顶部到底部的顺序,返回从右侧所能看到的节点值。
+
+
+
+示例 1:
+
+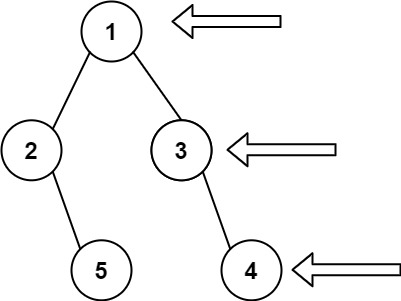
+
+
+输入: [1,2,3,null,5,null,4]
+输出: [1,3,4]
+
+
+示例 2:
+
+
+输入: [1,null,3]
+输出: [1,3]
+
+
+示例 3:
+
+
+输入: []
+输出: []
+
+
+
+
+提示:
+
+
+ - 二叉树的节点个数的范围是
[0,100]
+ -100 <= Node.val <= 100
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ vector rightSideView(TreeNode *root)
+ {
+
+ vector ret;
+ queue queues[2];
+
+ if (root != NULL)
+ queues[0].push(root);
+
+ int i = 0, j = 1, tmp;
+ TreeNode *p;
+ while (!queues[0].empty() || !queues[1].empty())
+ {
+ while (!queues[i].empty())
+ {
+ p = queues[i].front();
+ queues[i].pop();
+ if (p->left)
+ queues[j].push(p->left);
+ if (p->right)
+ queues[j].push(p->right);
+ tmp = p->val;
+ }
+
+ ret.push_back(tmp);
+ i = (i + 1) % 2;
+ j = (j + 1) % 2;
+ }
+
+ return ret;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..1b7b29247ce4772f4bc7b084278eb318dce722b1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-b42a7f99c1f84575bed9b796d6c8bf85",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..04240f254736ccb14ef6cb8bee2597cbad64964d
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "15ff375cf08d4b87b030c8b39867567d",
+ "author": "csdn.net",
+ "keywords": "深度优先搜索,广度优先搜索,并查集,数组,矩阵",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..a745b86d2171fea693b63451b30e3fc4a9b7fe5f
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/126.exercises/solution.md"
@@ -0,0 +1,128 @@
+# 岛屿数量
+
+给你一个由 '1'
(陆地)和 '0'
(水)组成的的二维网格,请你计算网格中岛屿的数量。
+
+岛屿总是被水包围,并且每座岛屿只能由水平方向和/或竖直方向上相邻的陆地连接形成。
+
+此外,你可以假设该网格的四条边均被水包围。
+
+
+
+示例 1:
+
+
+输入:grid = [
+ ["1","1","1","1","0"],
+ ["1","1","0","1","0"],
+ ["1","1","0","0","0"],
+ ["0","0","0","0","0"]
+]
+输出:1
+
+
+示例 2:
+
+
+输入:grid = [
+ ["1","1","0","0","0"],
+ ["1","1","0","0","0"],
+ ["0","0","1","0","0"],
+ ["0","0","0","1","1"]
+]
+输出:3
+
+
+
+
+提示:
+
+
+ m == grid.length
+ n == grid[i].length
+ 1 <= m, n <= 300
+ grid[i][j]
的值为 '0'
或 '1'
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ void DFS(vector> &mark, vector> &grid, int x, int y)
+ {
+ mark[x][y] = 1;
+ static const int dx[] = {-1, 1, 0, 0};
+ static const int dy[] = {0, 0, -1, 1};
+ for (int i = 0; i < 4; i++)
+ {
+ int newx = dx[i] + x;
+ int newy = dy[i] + y;
+ if (newx < 0 || newx >= mark.size() || newy < 0 || newy >= mark[newx].size())
+ {
+ continue;
+ }
+ if (mark[newx][newy] == 0 && grid[newx][newy] == '1')
+ {
+ DFS(mark, grid, newx, newy);
+ }
+ }
+ }
+ int numIslands(vector> &grid)
+ {
+ int island_num = 0;
+ vector> mark;
+ for (int i = 0; i < grid.size(); i++)
+ {
+ mark.push_back(vector());
+ for (int j = 0; j < grid[i].size(); j++)
+ {
+ mark[i].push_back(0);
+ }
+ }
+ for (int i = 0; i < grid.size(); i++)
+ {
+ for (int j = 0; j < grid[i].size(); j++)
+ {
+ if (mark[i][j] == 0 && grid[i][j] == '1')
+ {
+ DFS(mark, grid, i, j);
+ island_num++;
+ }
+ }
+ }
+ return island_num;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..1f29b73d4c6497b3a49122575d924df37b47b744
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-74b08309f19b41869c4320ea58482f6c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3f39fa357385855eaff7e207c4ece390235940aa
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "0efe4da13111471eaabaf79555034398",
+ "author": "csdn.net",
+ "keywords": "树,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..e954232106001d2365def6b27a8da24819df5dc4
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/88.exercises/solution.md"
@@ -0,0 +1,101 @@
+# 二叉树的层序遍历
+
+给你一个二叉树,请你返回其按 层序遍历 得到的节点值。 (即逐层地,从左到右访问所有节点)。
+
+
+
+示例:
+二叉树:[3,9,20,null,null,15,7]
,
+
+
+ 3
+ / \
+ 9 20
+ / \
+ 15 7
+
+
+返回其层序遍历结果:
+
+
+[
+ [3],
+ [9,20],
+ [15,7]
+]
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+
+class Solution
+{
+public:
+ vector> levelOrder(TreeNode *root)
+ {
+ if (root == NULL)
+ return {};
+ queue queue;
+ TreeNode *p = root;
+ vector> res;
+ queue.push(p);
+ while (queue.empty() == false)
+ {
+ vector temp = {};
+ int length = queue.size();
+ for (int i = 0; i < length; i++)
+ {
+ p = queue.front();
+ queue.pop();
+ temp.push_back(p->val);
+ if (p->left)
+ queue.push(p->left);
+ if (p->right)
+ queue.push(p->right);
+ }
+ res.push_back(temp);
+ }
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7f893bd26982afe6513e42419e4b2bbafc2b23ae
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-60c41833285047f3b321443845611bce",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f06972e972a8b63dbaff35676faf1501085f57ed
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "b9f1269194384877b4442ca8e7abf180",
+ "author": "csdn.net",
+ "keywords": "树,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9d0d8c5b066fb0c4d4f518a40296b600a699449c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/89.exercises/solution.md"
@@ -0,0 +1,105 @@
+# 二叉树的锯齿形层序遍历
+
+给定一个二叉树,返回其节点值的锯齿形层序遍历。(即先从左往右,再从右往左进行下一层遍历,以此类推,层与层之间交替进行)。
+
+例如:
+给定二叉树 [3,9,20,null,null,15,7]
,
+
+
+ 3
+ / \
+ 9 20
+ / \
+ 15 7
+
+
+返回锯齿形层序遍历如下:
+
+
+[
+ [3],
+ [20,9],
+ [15,7]
+]
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ vector> zigzagLevelOrder(TreeNode *root)
+ {
+ if (!root)
+ return {};
+ vector> res;
+ vector temp;
+ int level = 0;
+ queue> q;
+ q.push(pair(root, 0));
+ while (!q.empty())
+ {
+ TreeNode *node = q.front().first;
+ level = q.front().second;
+ q.pop();
+ if (res.size() < level)
+ {
+ if (level % 2 == 0)
+ reverse(temp.begin(), temp.end());
+ res.push_back(temp);
+ temp.clear();
+ }
+ temp.push_back(node->val);
+ if (node->left)
+ q.push(pair(node->left, level + 1));
+ if (node->right)
+ q.push(pair(node->right, level + 1));
+ }
+ if (level % 2 != 0)
+ reverse(temp.begin(), temp.end());
+ res.push_back(temp);
+ return res;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f5ff2b0be57139a847d788d3f36dae80bf406862
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-dff4c4e10e6f42a1ac201dd4d0ff8664",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..c86acc0ae09ab1e972186fa6bcb2a67d9c2086cd
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "ba75cf15fbae4173ae855d7975a09c08",
+ "author": "csdn.net",
+ "keywords": "树,数组,哈希表,分治,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..5469faf018853f2bdebfddcec127ae5a14c83dab
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/90.exercises/solution.md"
@@ -0,0 +1,108 @@
+# 从前序与中序遍历序列构造二叉树
+
+给定一棵树的前序遍历 preorder
与中序遍历 inorder
。请构造二叉树并返回其根节点。
+
+
+
+示例 1:
+
+
+Input: preorder = [3,9,20,15,7], inorder = [9,3,15,20,7]
+Output: [3,9,20,null,null,15,7]
+
+
+示例 2:
+
+
+Input: preorder = [-1], inorder = [-1]
+Output: [-1]
+
+
+
+
+提示:
+
+
+ 1 <= preorder.length <= 3000
+ inorder.length == preorder.length
+ -3000 <= preorder[i], inorder[i] <= 3000
+ preorder
和 inorder
均无重复元素
+ inorder
均出现在 preorder
+ preorder
保证为二叉树的前序遍历序列
+ inorder
保证为二叉树的中序遍历序列
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+private:
+ unordered_map inMap;
+
+public:
+ TreeNode *myBuildTree(vector &preorder, int preStart, int preEnd, vector &inorder, int inStart, int inEnd)
+ {
+ if (preStart > preEnd)
+ return nullptr;
+
+ TreeNode *root = new TreeNode(preorder[preStart]);
+
+ int inRoot = inMap[preorder[preStart]];
+ int numsLeft = inRoot - inStart;
+
+ root->left = myBuildTree(preorder, preStart + 1, preStart + numsLeft, inorder, inStart, inRoot - 1);
+ root->right = myBuildTree(preorder, preStart + numsLeft + 1, preEnd, inorder, inRoot + 1, inEnd);
+ return root;
+ }
+
+ TreeNode *buildTree(vector &preorder, vector &inorder)
+ {
+ int n = preorder.size();
+ for (int i = 0; i < n; i++)
+ {
+ inMap[inorder[i]] = i;
+ }
+ return myBuildTree(preorder, 0, n - 1, inorder, 0, n - 1);
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ccf7f2b13b04767c91bbf33e9e18dba601092aba
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-b8f6aa5c87444972987d7e7a1e52a22c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..bf703fd4980885e8997a3308c0144f857da8e3dc
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "114fd3f88e654e509ae581d5b5283b34",
+ "author": "csdn.net",
+ "keywords": "树,数组,哈希表,分治,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9a6d528ea366b43446eb596571ed8fe60b28ac95
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/91.exercises/solution.md"
@@ -0,0 +1,97 @@
+# 从中序与后序遍历序列构造二叉树
+
+根据一棵树的中序遍历与后序遍历构造二叉树。
+
+注意:
+你可以假设树中没有重复的元素。
+
+例如,给出
+
+中序遍历 inorder = [9,3,15,20,7]
+后序遍历 postorder = [9,15,7,20,3]
+
+返回如下的二叉树:
+
+ 3
+ / \
+ 9 20
+ / \
+ 15 7
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ TreeNode *buildTree(vector &inorder, vector &postorder)
+ {
+ if (0 == inorder.size() || 0 == postorder.size())
+ {
+ return NULL;
+ }
+ return build(inorder, 0, inorder.size() - 1, postorder, 0, postorder.size() - 1);
+ }
+
+ TreeNode *build(vector &inorder, int i1, int i2, vector &postorder, int p1, int p2)
+ {
+ TreeNode *root = new TreeNode(postorder[p2]);
+ int i = i1;
+ while (i <= i2 && postorder[p2] != inorder[i])
+ {
+ i++;
+ }
+ int left = i - i1;
+ int right = i2 - i;
+ if (left > 0)
+ {
+ root->left = build(inorder, i1, i - 1, postorder, p1, p1 + left - 1);
+ }
+ if (right > 0)
+ {
+ root->right = build(inorder, i + 1, i2, postorder, p1 + left, p2 - 1);
+ }
+ return root;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..689b2f43813d423f0f4157d0ef68baa64ae05db2
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-d26621ba92b24aeeb003c86d47d36ad6",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..06c5c22621cc568cab27c8560fe14da52cde79a7
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "3d8e9a5ee3b6439d92b567b667c50864",
+ "author": "csdn.net",
+ "keywords": "树,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..80df184b406de92269f34efb7d7829a5236ec2ec
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/92.exercises/solution.md"
@@ -0,0 +1,99 @@
+# 二叉树的层序遍历 II
+
+给定一个二叉树,返回其节点值自底向上的层序遍历。 (即按从叶子节点所在层到根节点所在的层,逐层从左向右遍历)
+
+例如:
+给定二叉树 [3,9,20,null,null,15,7]
,
+
+
+ 3
+ / \
+ 9 20
+ / \
+ 15 7
+
+
+返回其自底向上的层序遍历为:
+
+
+[
+ [15,7],
+ [9,20],
+ [3]
+]
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+
+class Solution
+{
+public:
+ vector> levelOrderBottom(TreeNode *root)
+ {
+
+ vector> res;
+ if (root == NULL)
+ return res;
+ queue q;
+ q.push(root);
+ while (!q.empty())
+ {
+ vector oneLevel;
+ int size = q.size();
+ for (int i = 0; i < size; i++)
+ {
+ TreeNode *node = q.front();
+ q.pop();
+ oneLevel.push_back(node->val);
+ if (node->left)
+ q.push(node->left);
+ if (node->right)
+ q.push(node->right);
+ }
+ res.insert(res.begin(), oneLevel);
+ }
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..9c85b8a4b109e0c0cd0e2a16c18a2a42a980f842
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-935af29ed8e5492cbb0712c8b1d0c653",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..3a2758fa509ef60408277523aa1f6f2cf69c998c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "8532653591304c138feba57d32124c1c",
+ "author": "csdn.net",
+ "keywords": "树,二叉搜索树,链表,分治,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..ebdba906174f4a9f9a9aa162af192a6bab8934da
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/93.exercises/solution.md"
@@ -0,0 +1,95 @@
+# 有序链表转换二叉搜索树
+
+给定一个单链表,其中的元素按升序排序,将其转换为高度平衡的二叉搜索树。
+
+本题中,一个高度平衡二叉树是指一个二叉树每个节点 的左右两个子树的高度差的绝对值不超过 1。
+
+示例:
+
+给定的有序链表: [-10, -3, 0, 5, 9],
+
+一个可能的答案是:[0, -3, 9, -10, null, 5], 它可以表示下面这个高度平衡二叉搜索树:
+
+ 0
+ / \
+ -3 9
+ / /
+ -10 5
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+struct ListNode
+{
+ int val;
+ ListNode *next;
+ ListNode(int x) : val(x), next(NULL) {}
+};
+
+class Solution
+{
+public:
+ vector nums;
+ TreeNode *create(int low, int high)
+ {
+ if (low > high)
+ return NULL;
+ int mid = (low + high) / 2;
+ auto root = new TreeNode(nums[mid]);
+ root->left = create(low, mid - 1);
+ root->right = create(mid + 1, high);
+ return root;
+ }
+ TreeNode *sortedListToBST(ListNode *head)
+ {
+ while (head)
+ {
+ nums.push_back(head->val);
+ head = head->next;
+ }
+
+ return create(0, nums.size() - 1);
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..9a0acacba15a66e3f7ef446957ac370c03ab466a
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-d202c192ccde42a8a79bb7439fc7bf10",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..65ed7d126310408cfb695a82ed21020429d5b44e
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "f891f16e32ea433e9dc188d9ea0d65ee",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,回溯,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..c53bd9dc0e0f0c954a78940c10bd8c01f057b012
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/94.exercises/solution.md"
@@ -0,0 +1,128 @@
+# 路径总和 II
+
+给你二叉树的根节点 root
和一个整数目标和 targetSum
,找出所有 从根节点到叶子节点 路径总和等于给定目标和的路径。
+
+叶子节点 是指没有子节点的节点。
+
+
+
+
+
+
示例 1:
+
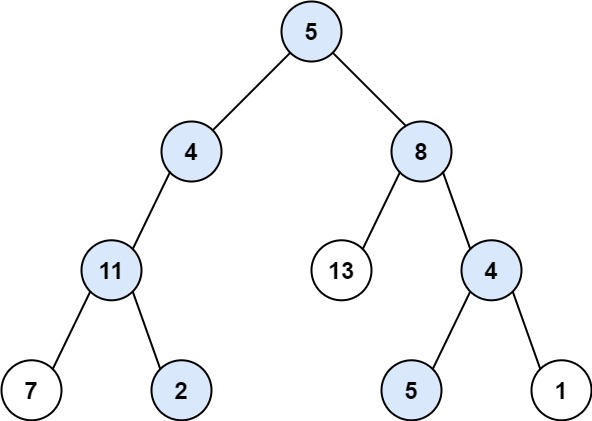
+
+输入:root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22
+输出:[[5,4,11,2],[5,8,4,5]]
+
+
+
示例 2:
+
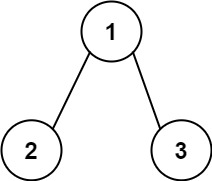
+
+输入:root = [1,2,3], targetSum = 5
+输出:[]
+
+
+
示例 3:
+
+
+输入:root = [1,2], targetSum = 0
+输出:[]
+
+
+
+
+
提示:
+
+
+ - 树中节点总数在范围
[0, 5000]
内
+ -1000 <= Node.val <= 1000
+ -1000 <= targetSum <= 1000
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ vector> pathSum(TreeNode *root, int sum)
+ {
+ vector> res;
+ vector track;
+ backTrack(root, res, track, sum);
+ return res;
+ }
+
+ void backTrack(TreeNode *root, vector> &res, vector track, int sum)
+ {
+
+ if (!root)
+ {
+ return;
+ }
+ if (!root->left && !root->right)
+ {
+ sum -= root->val;
+ track.push_back(root->val);
+ if (sum == 0)
+ {
+ res.push_back(track);
+ }
+ track.pop_back();
+ sum += root->val;
+ return;
+ }
+
+ sum -= root->val;
+ track.push_back(root->val);
+
+ backTrack(root->left, res, track, sum);
+ backTrack(root->right, res, track, sum);
+
+ track.pop_back();
+ sum += root->val;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..85850d525377f24d9ebf3a114fbc5b2360fb266c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-2728da0a5a584b3b8eee17473182c2e2",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e988953e06d3aed896fcb627e3f133e500a0019c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "fd0fc75e932545d8ba6c3ecc71bebf22",
+ "author": "csdn.net",
+ "keywords": "栈,树,深度优先搜索,链表,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..dbe68d297b81c7133be5f5201b97783e8a6ca2a8
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/95.exercises/solution.md"
@@ -0,0 +1,113 @@
+# 二叉树展开为链表
+
+给你二叉树的根结点 root
,请你将它展开为一个单链表:
+
+
+ - 展开后的单链表应该同样使用
TreeNode
,其中 right
子指针指向链表中下一个结点,而左子指针始终为 null
。
+ - 展开后的单链表应该与二叉树 先序遍历 顺序相同。
+
+
+
+
+示例 1:
+
+
+输入:root = [1,2,5,3,4,null,6]
+输出:[1,null,2,null,3,null,4,null,5,null,6]
+
+
+示例 2:
+
+
+输入:root = []
+输出:[]
+
+
+示例 3:
+
+
+输入:root = [0]
+输出:[0]
+
+
+
+
+提示:
+
+
+ - 树中结点数在范围
[0, 2000]
内
+ -100 <= Node.val <= 100
+
+
+
+
+进阶:你可以使用原地算法(O(1)
额外空间)展开这棵树吗?
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ void rconnect(TreeNode *&node, TreeNode *pmove)
+ {
+ if (pmove == nullptr)
+ return;
+ node->right = new TreeNode(pmove->val);
+ node->left = nullptr;
+ node = node->right;
+ rconnect(node, pmove->left);
+ rconnect(node, pmove->right);
+ }
+ void flatten(TreeNode *root)
+ {
+ if (root == nullptr)
+ return;
+ TreeNode *head = new TreeNode();
+ TreeNode *newroot = head;
+ rconnect(head, root);
+ newroot = newroot->right->right;
+ root->right = newroot;
+ root->left = nullptr;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7363f606b4e64b4113714c6266df32be2fe74de5
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-4e7bac103be94ad1b0b11a1ee6256987",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..8bc0d72fd559967b6ad2cd82ff40659c800f4878
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "8a772d0bda8142c3bdddb1b4d18cc62a",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..403d34145c1386c63bda2e345649675a35fee8c6
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/96.exercises/solution.md"
@@ -0,0 +1,131 @@
+# 填充每个节点的下一个右侧节点指针
+
+给定一个 完美二叉树 ,其所有叶子节点都在同一层,每个父节点都有两个子节点。二叉树定义如下:
+
+
+struct Node {
+ int val;
+ Node *left;
+ Node *right;
+ Node *next;
+}
+
+填充它的每个 next 指针,让这个指针指向其下一个右侧节点。如果找不到下一个右侧节点,则将 next 指针设置为 NULL
。
+
+初始状态下,所有 next 指针都被设置为 NULL
。
+
+
+
+进阶:
+
+
+ - 你只能使用常量级额外空间。
+ - 使用递归解题也符合要求,本题中递归程序占用的栈空间不算做额外的空间复杂度。
+
+
+
+
+示例:
+
+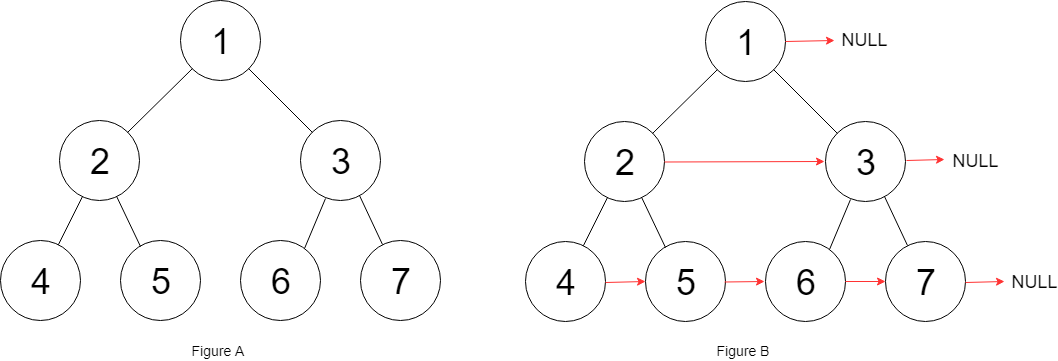
+
+
+输入:root = [1,2,3,4,5,6,7]
+输出:[1,#,2,3,#,4,5,6,7,#]
+解释:给定二叉树如图 A 所示,你的函数应该填充它的每个 next 指针,以指向其下一个右侧节点,如图 B 所示。序列化的输出按层序遍历排列,同一层节点由 next 指针连接,'#' 标志着每一层的结束。
+
+
+
+
+提示:
+
+
+ - 树中节点的数量少于
4096
+ -1000 <= node.val <= 1000
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Node
+{
+public:
+ int val;
+ Node *left;
+ Node *right;
+ Node *next;
+ Node() : val(0), left(NULL), right(NULL), next(NULL) {}
+ Node(int _val) : val(_val), left(NULL), right(NULL), next(NULL) {}
+ Node(int _val, Node *_left, Node *_right, Node *_next)
+ : val(_val), left(_left), right(_right), next(_next) {}
+};
+
+class Solution
+{
+public:
+ Node *connect(Node *root)
+ {
+ if (!root)
+ return nullptr;
+
+ root->next = nullptr;
+ connect_helper(root);
+
+ return root;
+ }
+
+private:
+ void connect_helper(Node *pNode)
+ {
+ if (!pNode)
+ return;
+
+ if (pNode->left == nullptr)
+ return;
+
+ pNode->left->next = pNode->right;
+
+ if (pNode->right == nullptr)
+ return;
+
+ if (pNode->next != nullptr)
+ pNode->right->next = pNode->next->left;
+ else
+ pNode->right->next = nullptr;
+
+ connect_helper(pNode->left);
+ connect_helper(pNode->right);
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..428de3e3a8cad005c2806a62d2806b2d9db8112c
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-acbff7e537ff4e00aafefb58ac6f1bd8",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..49e6602862a78b77b996817033ff5fff2ad5c2a4
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "fd74d5ca92e349568bf63c4e9ebdeed9",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,广度优先搜索,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..d37f6b23bbfc07098e080ccad607cf2ca03127ad
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/97.exercises/solution.md"
@@ -0,0 +1,130 @@
+# 填充每个节点的下一个右侧节点指针 II
+
+给定一个二叉树
+
+
+struct Node {
+ int val;
+ Node *left;
+ Node *right;
+ Node *next;
+}
+
+填充它的每个 next 指针,让这个指针指向其下一个右侧节点。如果找不到下一个右侧节点,则将 next 指针设置为 NULL
。
+
+初始状态下,所有 next 指针都被设置为 NULL
。
+
+
+
+进阶:
+
+
+ - 你只能使用常量级额外空间。
+ - 使用递归解题也符合要求,本题中递归程序占用的栈空间不算做额外的空间复杂度。
+
+
+
+
+示例:
+
+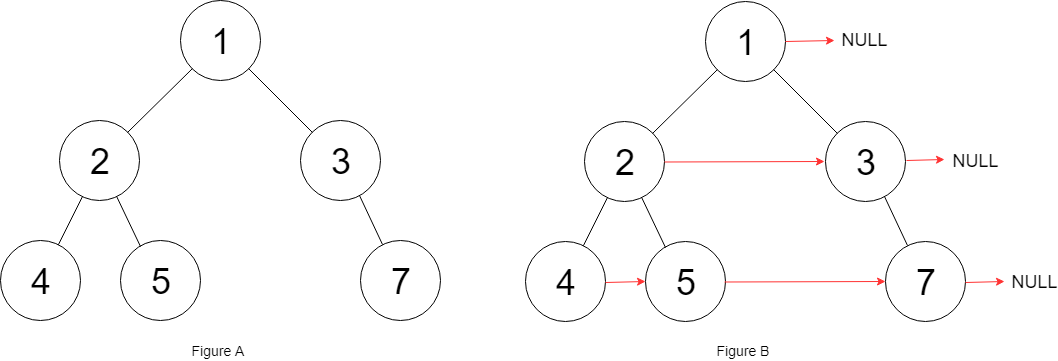
+
+
+输入:root = [1,2,3,4,5,null,7]
+输出:[1,#,2,3,#,4,5,7,#]
+解释:给定二叉树如图 A 所示,你的函数应该填充它的每个 next 指针,以指向其下一个右侧节点,如图 B 所示。序列化输出按层序遍历顺序(由 next 指针连接),'#' 表示每层的末尾。
+
+
+
+提示:
+
+
+ - 树中的节点数小于
6000
+ -100 <= node.val <= 100
+
+
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Node
+{
+public:
+ int val;
+ Node *left;
+ Node *right;
+ Node *next;
+ Node() : val(0), left(NULL), right(NULL), next(NULL) {}
+ Node(int _val) : val(_val), left(NULL), right(NULL), next(NULL) {}
+ Node(int _val, Node *_left, Node *_right, Node *_next)
+ : val(_val), left(_left), right(_right), next(_next) {}
+};
+
+class Solution
+{
+public:
+ Node *connect(Node *root)
+ {
+ if (!root)
+ return NULL;
+ Node *p = root->next;
+ while (p)
+ {
+ if (p->left)
+ {
+ p = p->left;
+ break;
+ }
+ if (p->right)
+ {
+ p = p->right;
+ break;
+ }
+ p = p->next;
+ }
+ if (root->right)
+ root->right->next = p;
+ if (root->left)
+ root->left->next = root->right ? root->right : p;
+ connect(root->right);
+ connect(root->left);
+ return root;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ba6cebd959cde9bfb306ec4cb35921b2a9af8209
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-b84e0ad3b5c8484ab8dbb922eeead085",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e733cba75a83a4fd3e22e03b71c4f8fff9fb6b86
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "383ae4c542d449439fc15dd853dbf9cd",
+ "author": "csdn.net",
+ "keywords": "数组,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..6baaa6197f8f41c4588af014979945381b420ce1
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/98.exercises/solution.md"
@@ -0,0 +1,120 @@
+# 三角形最小路径和
+
+给定一个三角形 triangle
,找出自顶向下的最小路径和。
+
+每一步只能移动到下一行中相邻的结点上。相邻的结点 在这里指的是 下标 与 上一层结点下标 相同或者等于 上一层结点下标 + 1 的两个结点。也就是说,如果正位于当前行的下标 i
,那么下一步可以移动到下一行的下标 i
或 i + 1
。
+
+
+
+示例 1:
+
+
+输入:triangle = [[2],[3,4],[6,5,7],[4,1,8,3]]
+输出:11
+解释:如下面简图所示:
+ 2
+ 3 4
+ 6 5 7
+4 1 8 3
+自顶向下的最小路径和为 11(即,2 + 3 + 5 + 1 = 11)。
+
+
+示例 2:
+
+
+输入:triangle = [[-10]]
+输出:-10
+
+
+
+
+提示:
+
+
+ 1 <= triangle.length <= 200
+ triangle[0].length == 1
+ triangle[i].length == triangle[i - 1].length + 1
+ -104 <= triangle[i][j] <= 104
+
+
+
+
+进阶:
+
+
+ - 你可以只使用
O(n)
的额外空间(n
为三角形的总行数)来解决这个问题吗?
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int minimumTotal(vector> &triangle)
+ {
+ int n = triangle.size();
+ if (n == 0)
+ return 0;
+ if (n == 1)
+ return triangle[0][0];
+ vector> info(n, vector(n, 0));
+ info[0][0] = triangle[0][0];
+ for (int i = 1; i < n; ++i)
+ {
+ for (int j = 0; j <= i; ++j)
+ {
+ if (j == 0)
+ info[i][j] = triangle[i][j] + info[i - 1][j];
+ else if (j == i)
+ info[i][j] = triangle[i][j] + info[i - 1][j - 1];
+ else
+ {
+ int temp = info[i - 1][j] > info[i - 1][j - 1] ? info[i - 1][j - 1] : info[i - 1][j];
+ info[i][j] = temp + triangle[i][j];
+ }
+ }
+ }
+ int res = info[n - 1][0];
+ for (int i = 0; i < n; ++i)
+ {
+ if (info[n - 1][i] < res)
+ {
+ res = info[n - 1][i];
+ }
+ }
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/config.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..16ffcf0fc9ba32d1f2068ab04aa3a3f324d3d417
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-abe1e612615b4a0cb30c4d43d8317bcf",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/solution.json" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..bfb1ba870e69927a44b531707aa3626a8ca08144
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "51ccf4e117df4625be67d2c9ff6bab72",
+ "author": "csdn.net",
+ "keywords": "并查集,数组,哈希表",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/solution.md" "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..fca772aec430ce905ff6fb4ea8286eaa83558cd5
--- /dev/null
+++ "b/data/2.dailycode\344\270\255\351\230\266/1.cpp/99.exercises/solution.md"
@@ -0,0 +1,101 @@
+# 最长连续序列
+
+给定一个未排序的整数数组 nums
,找出数字连续的最长序列(不要求序列元素在原数组中连续)的长度。
+
+请你设计并实现时间复杂度为 O(n)
的算法解决此问题。
+
+
+
+示例 1:
+
+
+输入:nums = [100,4,200,1,3,2]
+输出:4
+解释:最长数字连续序列是 [1, 2, 3, 4]。它的长度为 4。
+
+示例 2:
+
+
+输入:nums = [0,3,7,2,5,8,4,6,0,1]
+输出:9
+
+
+
+
+提示:
+
+
+ 0 <= nums.length <= 105
+ -109 <= nums[i] <= 109
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int longestConsecutive(vector &nums)
+ {
+ unordered_set s;
+ for (auto v : nums)
+ s.insert(v);
+ int res = 0;
+ for (auto v : nums)
+ {
+ if (s.find(v) != s.end())
+ {
+ s.erase(v);
+ int temp = 1;
+ int cur = v;
+ while (s.find(cur - 1) != s.end())
+ {
+ cur--;
+ s.erase(cur);
+ }
+ temp += v - cur;
+ cur = v;
+ while (s.find(cur + 1) != s.end())
+ {
+ cur++;
+ s.erase(cur);
+ }
+ temp += cur - v;
+ res = max(res, temp);
+ }
+ }
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..fb893651364b007a0a5077e608f7c61847ad7f38
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-20b092dea834439fb605c1749d6e268b",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..c41a54d5d64dfccdae970d470eccd8325b5ee2a1
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "4175e229f9cb4ec297b994f018f5bf95",
+ "author": "csdn.net",
+ "keywords": "字符串,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..c877942b2aebee548a1ceff40bc5c4a0b22ec298
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/41.exercises/solution.md"
@@ -0,0 +1,99 @@
+# 不同的子序列
+
+给定一个字符串 s
和一个字符串 t
,计算在 s
的子序列中 t
出现的个数。
+
+字符串的一个 子序列 是指,通过删除一些(也可以不删除)字符且不干扰剩余字符相对位置所组成的新字符串。(例如,"ACE"
是 "ABCDE"
的一个子序列,而 "AEC"
不是)
+
+题目数据保证答案符合 32 位带符号整数范围。
+
+
+
+示例 1:
+
+
+输入:s = "rabbbit", t = "rabbit"
+输出
:3
+
解释:
+如下图所示, 有 3 种可以从 s 中得到 "rabbit" 的方案
。
+rabbbit
+rabbbit
+rabbbit
+
+示例 2:
+
+
+输入:s = "babgbag", t = "bag"
+输出
:5
+
解释:
+如下图所示, 有 5 种可以从 s 中得到 "bag" 的方案
。
+babgbag
+babgbag
+babgbag
+babgbag
+babgbag
+
+
+
+
+提示:
+
+
+ 0 <= s.length, t.length <= 1000
+ s
和 t
由英文字母组成
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int numDistinct(string s, string t)
+ {
+ long dp[t.size() + 1][s.size() + 1];
+ for (int i = 0; i <= s.size(); ++i)
+ dp[0][i] = 1;
+ for (int i = 1; i <= t.size(); ++i)
+ dp[i][0] = 0;
+ for (int i = 1; i <= t.size(); ++i)
+ {
+ for (int j = 1; j <= s.size(); ++j)
+ {
+ dp[i][j] = dp[i][j - 1] + (t[i - 1] == s[j - 1] ? dp[i - 1][j - 1] : 0);
+ }
+ }
+ return dp[t.size()][s.size()];
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..12e788ab2e6b8e0e2857644d228a3d22147b2e64
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-b21a1bcfe07c466d894abf1624a2e27b",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..9ca9cb4ea8e52aa26e63fa70616b04b0496a52ab
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "14750c7919bf4d9cb1cc1b9dc589acd9",
+ "author": "csdn.net",
+ "keywords": "数组,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..e779d2fe09c233e70936fbb3e3fc3257ce5a680b
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/42.exercises/solution.md"
@@ -0,0 +1,117 @@
+# 买卖股票的最佳时机 III
+
+给定一个数组,它的第 i
个元素是一支给定的股票在第 i
天的价格。
+
+设计一个算法来计算你所能获取的最大利润。你最多可以完成 两笔 交易。
+
+注意:你不能同时参与多笔交易(你必须在再次购买前出售掉之前的股票)。
+
+
+
+示例 1:
+
+
+输入:prices = [3,3,5,0,0,3,1,4]
+输出:6
+解释:在第 4 天(股票价格 = 0)的时候买入,在第 6 天(股票价格 = 3)的时候卖出,这笔交易所能获得利润 = 3-0 = 3 。
+ 随后,在第 7 天(股票价格 = 1)的时候买入,在第 8 天 (股票价格 = 4)的时候卖出,这笔交易所能获得利润 = 4-1 = 3 。
+
+示例 2:
+
+
+输入:prices = [1,2,3,4,5]
+输出:4
+解释:在第 1 天(股票价格 = 1)的时候买入,在第 5 天 (股票价格 = 5)的时候卖出, 这笔交易所能获得利润 = 5-1 = 4 。
+ 注意你不能在第 1 天和第 2 天接连购买股票,之后再将它们卖出。
+ 因为这样属于同时参与了多笔交易,你必须在再次购买前出售掉之前的股票。
+
+
+示例 3:
+
+
+输入:prices = [7,6,4,3,1]
+输出:0
+解释:在这个情况下, 没有交易完成, 所以最大利润为 0。
+
+示例 4:
+
+
+输入:prices = [1]
+输出:0
+
+
+
+
+提示:
+
+
+ 1 <= prices.length <= 105
+ 0 <= prices[i] <= 105
+
+
+
+## template
+
+```cpp
+
+class Solution
+{
+public:
+ int maxProfit(vector &prices)
+ {
+ int length = prices.size();
+ if (length < 2)
+ {
+ return 0;
+ }
+ vector former(length, 0);
+ vector later(length, 0);
+ int curMin = prices[0];
+ int curProfit = 0;
+ for (int i = 1; i < length; i++)
+ {
+ curProfit = max(curProfit, prices[i] - curMin);
+ curMin = min(curMin, prices[i]);
+ former[i] = curProfit;
+ }
+ int curMax = prices[length - 1];
+ curProfit = 0;
+ for (int i = length - 2; i >= 0; i--)
+ {
+ curProfit = max(curProfit, curMax - prices[i]);
+ curMax = max(curMax, prices[i]);
+ later[i] = curProfit;
+ }
+ int maxProfit = 0;
+ for (int i = 0; i < length; i++)
+ maxProfit = max(maxProfit, former[i] + later[i]);
+ return maxProfit;
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7d26412d416f474a5983e012ae3a03c20b4cddf2
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-d58de695f661474f8a2f72bf151c32f4",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..471c0d73dd4124295f9c4ae55fb46a6a7378c79a
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "9cb85eede3984b70b08147e8b4525294",
+ "author": "csdn.net",
+ "keywords": "树,深度优先搜索,动态规划,二叉树",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..6076c4909f0923b8e9be7f302e1feb106c908c15
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/43.exercises/solution.md"
@@ -0,0 +1,130 @@
+# 二叉树中的最大路径和
+
+路径 被定义为一条从树中任意节点出发,沿父节点-子节点连接,达到任意节点的序列。同一个节点在一条路径序列中 至多出现一次 。该路径 至少包含一个 节点,且不一定经过根节点。
+
+路径和 是路径中各节点值的总和。
+
+给你一个二叉树的根节点 root
,返回其 最大路径和 。
+
+
+
+示例 1:
+
+
+输入:root = [1,2,3]
+输出:6
+解释:最优路径是 2 -> 1 -> 3 ,路径和为 2 + 1 + 3 = 6
+
+示例 2:
+
+
+输入:root = [-10,9,20,null,null,15,7]
+输出:42
+解释:最优路径是 15 -> 20 -> 7 ,路径和为 15 + 20 + 7 = 42
+
+
+
+
+提示:
+
+
+ - 树中节点数目范围是
[1, 3 * 104]
+ -1000 <= Node.val <= 1000
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct TreeNode
+{
+ int val;
+ TreeNode *left;
+ TreeNode *right;
+ TreeNode(int x) : val(x), left(NULL), right(NULL) {}
+};
+
+class Solution
+{
+public:
+ int maxPathSum(TreeNode *root)
+ {
+ if (!root)
+ return 0;
+ vector ss;
+ unordered_map val;
+ ss.push_back(root);
+ int len = 1;
+ queue q{{root}};
+ while (!q.empty())
+ {
+ TreeNode *t = q.front();
+ q.pop();
+ cout << t->val << endl;
+ if (t->left)
+ {
+ len++;
+ q.push(t->left);
+ ss.push_back(t->left);
+ }
+ if (t->right)
+ {
+ len++;
+ q.push(t->right);
+ ss.push_back(t->right);
+ }
+ }
+
+ int res = INT_MIN;
+
+ while (len > 0)
+ {
+ TreeNode *node = ss[--len];
+ int ps = node->val;
+ int s = ps;
+
+ int ls = max(0, val[node->left]);
+ int rs = max(0, val[node->right]);
+
+ ps += max(ls, rs);
+ val[node] = ps;
+
+ s += ls + rs;
+ res = max(s, res);
+ }
+
+ return res;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..f57f5fe98a42b3da7d0818d0302238861c2c6310
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-d92de43caa3540a4b5aa693d35035468",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..5b6ef48064b90e1878044914723ce85509099626
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "bc43d567d92b494b84197834566161dd",
+ "author": "csdn.net",
+ "keywords": "广度优先搜索,哈希表,字符串,回溯",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..f3303bb6c8396e4549091115edc3bc6ceb65eda0
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/44.exercises/solution.md"
@@ -0,0 +1,153 @@
+# 单词接龙 II
+
+按字典 wordList
完成从单词 beginWord
到单词 endWord
转化,一个表示此过程的 转换序列 是形式上像 beginWord -> s1 -> s2 -> ... -> sk
这样的单词序列,并满足:
+
+
+
+
+ - 每对相邻的单词之间仅有单个字母不同。
+ - 转换过程中的每个单词
si
(1 <= i <= k
)必须是字典 wordList
中的单词。注意,beginWord
不必是字典 wordList
中的单词。
+ sk == endWord
+
+
+
给你两个单词 beginWord
和 endWord
,以及一个字典 wordList
。请你找出并返回所有从 beginWord
到 endWord
的 最短转换序列 ,如果不存在这样的转换序列,返回一个空列表。每个序列都应该以单词列表 [beginWord, s1, s2, ..., sk]
的形式返回。
+
+
+
+
示例 1:
+
+
+输入:beginWord = "hit", endWord = "cog", wordList = ["hot","dot","dog","lot","log","cog"]
+输出:[["hit","hot","dot","dog","cog"],["hit","hot","lot","log","cog"]]
+解释:存在 2 种最短的转换序列:
+"hit" -> "hot" -> "dot" -> "dog" -> "cog"
+"hit" -> "hot" -> "lot" -> "log" -> "cog"
+
+
+
示例 2:
+
+
+输入:beginWord = "hit", endWord = "cog", wordList = ["hot","dot","dog","lot","log"]
+输出:[]
+解释:endWord "cog" 不在字典 wordList 中,所以不存在符合要求的转换序列。
+
+
+
+
+
提示:
+
+
+ 1 <= beginWord.length <= 7
+ endWord.length == beginWord.length
+ 1 <= wordList.length <= 5000
+ wordList[i].length == beginWord.length
+ beginWord
、endWord
和 wordList[i]
由小写英文字母组成
+ beginWord != endWord
+ wordList
中的所有单词 互不相同
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ bool differ_one(string &s, string &t)
+ {
+ int n = 0;
+ for (int i = 0; i < s.size(); ++i)
+ if ((n += s[i] != t[i]) > 1)
+ return false;
+ return n == 1;
+ }
+ vector> fa;
+ vector tmp;
+ vector> ans;
+ void dfs(int index, vector &wordList)
+ {
+ if (fa[index].empty())
+ {
+ reverse(tmp.begin(), tmp.end());
+ ans.push_back(tmp);
+ reverse(tmp.begin(), tmp.end());
+ }
+ for (auto &x : fa[index])
+ {
+ tmp.push_back(wordList[x]);
+ dfs(x, wordList);
+ tmp.pop_back();
+ }
+ }
+ vector> findLadders(string beginWord, string endWord, vector &wordList)
+ {
+
+ int b = -1, e = -1, x;
+ for (int i = 0; i < wordList.size(); ++i)
+ {
+ if (wordList[i] == beginWord)
+ b = i;
+ if (wordList[i] == endWord)
+ e = i;
+ }
+ if (e == -1)
+ return ans;
+ if (b == -1)
+ wordList.push_back(beginWord), b = wordList.size() - 1;
+ queue que;
+ fa.assign(wordList.size(), vector());
+ vector index(wordList.size(), 0);
+ que.push(b), index[b] = 1;
+ while (!que.empty())
+ {
+ x = que.front(), que.pop();
+ if (index[e] && index[x] >= index[e])
+ break;
+ for (int i = 0; i < wordList.size(); ++i)
+ if ((index[i] == 0 || index[x] + 1 == index[i]) && differ_one(wordList[x], wordList[i]))
+ if (index[i] == 0)
+ index[i] = index[x] + 1, que.push(i), fa[i].push_back(x);
+ else
+ fa[i].push_back(x);
+ }
+ if (index[e] == 0)
+ return ans;
+ tmp.push_back(endWord);
+ dfs(e, wordList);
+ tmp.pop_back();
+ return ans;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7302e5533e5c6e0ee18d80a218633ee88762ef6f
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-98daf8a40cf94f16b451b22387358559",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..aedef69a9a9246f0420bc7c2a4478c4849e79b89
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "d8fd443f1d594cca97e0cbba610a3249",
+ "author": "csdn.net",
+ "keywords": "广度优先搜索,哈希表,字符串",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..f4cb1a5b54badf098fbeb5993825a56e8fa28bf6
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/45.exercises/solution.md"
@@ -0,0 +1,129 @@
+# 单词接龙
+
+字典 wordList
中从单词 beginWord
和 endWord
的 转换序列 是一个按下述规格形成的序列:
+
+
+ - 序列中第一个单词是
beginWord
。
+ - 序列中最后一个单词是
endWord
。
+ - 每次转换只能改变一个字母。
+ - 转换过程中的中间单词必须是字典
wordList
中的单词。
+
+
+给你两个单词 beginWord
和 endWord
和一个字典 wordList
,找到从 beginWord
到 endWord
的 最短转换序列 中的 单词数目 。如果不存在这样的转换序列,返回 0。
+
+
+示例 1:
+
+
+输入:beginWord = "hit", endWord = "cog", wordList = ["hot","dot","dog","lot","log","cog"]
+输出:5
+解释:一个最短转换序列是 "hit" -> "hot" -> "dot" -> "dog" -> "cog", 返回它的长度 5。
+
+
+示例 2:
+
+
+输入:beginWord = "hit", endWord = "cog", wordList = ["hot","dot","dog","lot","log"]
+输出:0
+解释:endWord "cog" 不在字典中,所以无法进行转换。
+
+
+
+提示:
+
+
+ 1 <= beginWord.length <= 10
+ endWord.length == beginWord.length
+ 1 <= wordList.length <= 5000
+ wordList[i].length == beginWord.length
+ beginWord
、endWord
和 wordList[i]
由小写英文字母组成
+ beginWord != endWord
+ wordList
中的所有字符串 互不相同
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int ladderLength(string beginWord, string endWord, vector &wordList)
+ {
+
+ unordered_set dict(wordList.begin(), wordList.end());
+ if (dict.find(endWord) == dict.end())
+ return 0;
+
+ queue> q;
+ q.push(make_pair(beginWord, 1));
+
+ string tmp;
+ int step;
+
+ while (!q.empty())
+ {
+ auto p = q.front();
+
+ if (p.first == endWord)
+ return p.second;
+ tmp = p.first;
+ step = p.second;
+ q.pop();
+
+ char old_ch;
+ for (int i = 0; i < tmp.size(); ++i)
+ {
+ old_ch = tmp[i];
+
+ for (char c = 'a'; c <= 'z'; ++c)
+ {
+
+ if (c == old_ch)
+ continue;
+ tmp[i] = c;
+
+ if (dict.find(tmp) != dict.end())
+ {
+ q.push(make_pair(tmp, step + 1));
+ dict.erase(tmp);
+ }
+ }
+ tmp[i] = old_ch;
+ }
+ }
+ return 0;
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..fe8dbc51a753d676e804670afaad84d959f36545
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-623ee98952304b7e9b919c3d7e146a19",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..a5e02f0ef5634219eb3c405f1b1024f93c5335a1
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "a8246082dcfb4ea5afea0cf24bb12dc2",
+ "author": "csdn.net",
+ "keywords": "字符串,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..0f6086a2a122d728fb448773d31c347982eadb92
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/46.exercises/solution.md"
@@ -0,0 +1,113 @@
+# 分割回文串 II
+
+给你一个字符串 s
,请你将 s
分割成一些子串,使每个子串都是回文。
+
+返回符合要求的 最少分割次数 。
+
+
+
+
+
+
示例 1:
+
+
+输入:s = "aab"
+输出:1
+解释:只需一次分割就可将 s 分割成 ["aa","b"] 这样两个回文子串。
+
+
+
示例 2:
+
+
+输入:s = "a"
+输出:0
+
+
+
示例 3:
+
+
+输入:s = "ab"
+输出:1
+
+
+
+
+
提示:
+
+
+ 1 <= s.length <= 2000
+ s
仅由小写英文字母组成
+
+
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int minCut(string s)
+ {
+
+ const int N = s.size();
+ if (N <= 1)
+ return 0;
+
+ int i, j;
+
+ bool isPalin[N][N];
+
+ fill_n(&isPalin[0][0], N * N, false);
+
+ int minCuts[N + 1];
+ for (i = 0; i <= N; ++i)
+ minCuts[i] = i - 1;
+
+ for (j = 1; j < N; ++j)
+ {
+ for (i = j; i >= 0; --i)
+ {
+
+ if ((s[i] == s[j]) && ((j - i < 2) || isPalin[i + 1][j - 1]))
+ {
+ isPalin[i][j] = true;
+ minCuts[j + 1] = min(minCuts[j + 1], 1 + minCuts[i]);
+ }
+ }
+ }
+ return minCuts[N];
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..4a2e56b81dc0e3d468dcaad5724392623610d083
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-37990a05873740ab861f20f4e78be70b",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..2f954a44f0603028e3f57581da103f54096bf821
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "11ebd5d979fb4925941083ff52136c4e",
+ "author": "csdn.net",
+ "keywords": "贪心,数组",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..e236f6a3400f6a729066ccaa099907bf3e166f9c
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/47.exercises/solution.md"
@@ -0,0 +1,93 @@
+# 分发糖果
+
+老师想给孩子们分发糖果,有 N 个孩子站成了一条直线,老师会根据每个孩子的表现,预先给他们评分。
+
+你需要按照以下要求,帮助老师给这些孩子分发糖果:
+
+
+ - 每个孩子至少分配到 1 个糖果。
+ - 评分更高的孩子必须比他两侧的邻位孩子获得更多的糖果。
+
+
+那么这样下来,老师至少需要准备多少颗糖果呢?
+
+
+
+示例 1:
+
+
+输入:[1,0,2]
+输出:5
+解释:你可以分别给这三个孩子分发 2、1、2 颗糖果。
+
+
+示例 2:
+
+
+输入:[1,2,2]
+输出:4
+解释:你可以分别给这三个孩子分发 1、2、1 颗糖果。
+ 第三个孩子只得到 1 颗糖果,这已满足上述两个条件。
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int candy(vector &ratings)
+ {
+ int len = ratings.size();
+ if (len < 2)
+ return len;
+ int candy[len + 1];
+ candy[0] = 1;
+ for (int i = 1; i < len; i++)
+ {
+ if (ratings[i] > ratings[i - 1])
+ candy[i] = candy[i - 1] + 1;
+ else
+ candy[i] = 1;
+ }
+ int ans = 0;
+ for (int i = len - 1; i > 0; i--)
+ {
+ if (candy[i] >= candy[i - 1] && ratings[i] < ratings[i - 1])
+ candy[i - 1] = max(candy[i - 1], candy[i] + 1);
+ ans += candy[i];
+ }
+ return ans + candy[0];
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b78c7daad661d2c7e0f3f8035a36b31dc342fe75
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-dc71d0ff18eb49d79792f969fe3e4e12",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..1e1246b15c4f88f2dbe026904bf871df311dbc60
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "104810fc2b7b43be9636eda4d798839b",
+ "author": "csdn.net",
+ "keywords": "字典树,记忆化搜索,哈希表,字符串,动态规划,回溯",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..05bdff80431fd0056f8725387cc8070d48fa265a
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/48.exercises/solution.md"
@@ -0,0 +1,129 @@
+# 单词拆分 II
+
+给定一个非空字符串 s 和一个包含非空单词列表的字典 wordDict,在字符串中增加空格来构建一个句子,使得句子中所有的单词都在词典中。返回所有这些可能的句子。
+
+说明:
+
+
+ - 分隔时可以重复使用字典中的单词。
+ - 你可以假设字典中没有重复的单词。
+
+
+示例 1:
+
+输入:
+s = "catsanddog
"
+wordDict = ["cat", "cats", "and", "sand", "dog"]
+输出:
+[
+ "cats and dog",
+ "cat sand dog"
+]
+
+
+示例 2:
+
+输入:
+s = "pineapplepenapple"
+wordDict = ["apple", "pen", "applepen", "pine", "pineapple"]
+输出:
+[
+ "pine apple pen apple",
+ "pineapple pen apple",
+ "pine applepen apple"
+]
+解释: 注意你可以重复使用字典中的单词。
+
+
+示例 3:
+
+输入:
+s = "catsandog"
+wordDict = ["cats", "dog", "sand", "and", "cat"]
+输出:
+[]
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ vector res;
+ unordered_set wordset;
+ unordered_set lenset;
+ vector wordBreak(string s, vector &wordDict)
+ {
+ for (const auto &w : wordDict)
+ {
+ wordset.insert(w);
+ lenset.insert(w.size());
+ }
+ vector dp(s.size() + 1, 0);
+ dp[0] = 1;
+ for (int i = 1; i <= s.size(); ++i)
+ {
+ for (const auto &j : lenset)
+ {
+ if (i >= j && dp[i - j] && wordset.count(s.substr(i - j, j)))
+ dp[i] = 1;
+ }
+ }
+
+ if (dp.back() == 0)
+ return res;
+ backtrack(dp, 0, s, "");
+ return res;
+ }
+
+ void backtrack(vector &dp, int idx, string &s, string tmp)
+ {
+ if (idx == s.size())
+ {
+ tmp.pop_back();
+ res.push_back(tmp);
+ return;
+ }
+
+ for (int i = idx + 1; i < dp.size(); ++i)
+ {
+ if (dp[i] == 1 && wordset.count(s.substr(idx, i - idx)))
+ {
+ backtrack(dp, i, s, tmp + s.substr(idx, i - idx) + " ");
+ }
+ }
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..76f8c6b975ea3d6ef991f4021021b384b568774d
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-19c4d2ed874a4229bed1cfffb35fa37c",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..e6449a05f288fcad8da01dd6c75f6524fa08991b
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "3140fe1100a441829c2fa398e3987f00",
+ "author": "csdn.net",
+ "keywords": "几何,哈希表,数学",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..33c07367782b0e45734dfb1a815158104ed77aca
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/49.exercises/solution.md"
@@ -0,0 +1,110 @@
+# 直线上最多的点数
+
+给你一个数组 points
,其中 points[i] = [xi, yi]
表示 X-Y 平面上的一个点。求最多有多少个点在同一条直线上。
+
+
+
+示例 1:
+
+
+输入:points = [[1,1],[2,2],[3,3]]
+输出:3
+
+
+示例 2:
+
+
+输入:points = [[1,1],[3,2],[5,3],[4,1],[2,3],[1,4]]
+输出:4
+
+
+
+
+提示:
+
+
+ 1 <= points.length <= 300
+ points[i].length == 2
+ -104 <= xi, yi <= 104
+ points
中的所有点 互不相同
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+struct Point
+{
+ int x;
+ int y;
+ Point() : x(0), y(0) {}
+ Point(int a, int b) : x(a), y(b) {}
+};
+
+class Solution
+{
+public:
+ int maxPoints(vector &points)
+ {
+ int ans = 0;
+ for (int i = 0; i < points.size(); ++i)
+ {
+ map, int> m;
+ int p = 1;
+ for (int j = i + 1; j < points.size(); ++j)
+ {
+ if (points[i].x == points[j].x && (points[i].y == points[j].y))
+ {
+ ++p;
+ continue;
+ }
+ int dx = points[j].x - points[i].x;
+ int dy = points[j].y - points[i].y;
+ int d = gcd(dx, dy);
+ ++m[{dx / d, dy / d}];
+ }
+ ans = max(ans, p);
+ for (auto it = m.begin(); it != m.end(); ++it)
+ {
+ ans = max(ans, it->second + p);
+ }
+ }
+ return ans;
+ }
+ int gcd(int a, int b)
+ {
+ return (b == 0) ? a : gcd(b, a % b);
+ }
+};
+
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..82c65ebcf5d750832f823970c750ec35e46e0378
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-16b098a417204953a4caabb304f6db9f",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..4a7942fa78c674ded8372c5b8f6131f89968b661
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "eec0e728b9714adaa9f3b0d1958b3834",
+ "author": "csdn.net",
+ "keywords": "数组,二分查找",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..21c12edbba038cd87b8dd99155a25ca958a4cb29
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/50.exercises/solution.md"
@@ -0,0 +1,103 @@
+# 寻找旋转排序数组中的最小值 II
+
+已知一个长度为 n
的数组,预先按照升序排列,经由 1
到 n
次 旋转 后,得到输入数组。例如,原数组 nums = [0,1,4,4,5,6,7]
在变化后可能得到:
+
+ - 若旋转
4
次,则可以得到 [4,5,6,7,0,1,4]
+ - 若旋转
7
次,则可以得到 [0,1,4,4,5,6,7]
+
+
+注意,数组 [a[0], a[1], a[2], ..., a[n-1]]
旋转一次 的结果为数组 [a[n-1], a[0], a[1], a[2], ..., a[n-2]]
。
+
+给你一个可能存在 重复 元素值的数组 nums
,它原来是一个升序排列的数组,并按上述情形进行了多次旋转。请你找出并返回数组中的 最小元素 。
+
+
+
+示例 1:
+
+
+输入:nums = [1,3,5]
+输出:1
+
+
+示例 2:
+
+
+输入:nums = [2,2,2,0,1]
+输出:0
+
+
+
+
+提示:
+
+
+ n == nums.length
+ 1 <= n <= 5000
+ -5000 <= nums[i] <= 5000
+ nums
原来是一个升序排序的数组,并进行了 1
至 n
次旋转
+
+
+
+
+进阶:
+
+
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int findMin(vector &nums)
+ {
+
+ int left = 0, right = nums.size() - 1;
+ while (left < right)
+ {
+ int mid = left + (right - left) / 2;
+ if (nums[mid] < nums[right])
+ right = mid;
+ else if (nums[mid] > nums[right])
+ left = mid + 1;
+ else if (nums[mid] == nums[right])
+ right--;
+ }
+ return nums[right];
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..fded30b1dcb53244de8f749ded648e79c0030cc3
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-d7a599592aa24aff848013c6ec15cf10",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..760164dc5d312b3eb31296b28affc6cc2a529a36
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "8ae901e74c254416b4765e3f64e86f2c",
+ "author": "csdn.net",
+ "keywords": "数组,桶排序,基数排序,排序",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..f5ddf1629a9ae743f23e1f7ec0d6aa1ebdeb40df
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/51.exercises/solution.md"
@@ -0,0 +1,83 @@
+# 最大间距
+
+给定一个无序的数组,找出数组在排序之后,相邻元素之间最大的差值。
+
+如果数组元素个数小于 2,则返回 0。
+
+示例 1:
+
+输入: [3,6,9,1]
+输出: 3
+解释: 排序后的数组是 [1,3,6,9], 其中相邻元素 (3,6) 和 (6,9) 之间都存在最大差值 3。
+
+示例 2:
+
+输入: [10]
+输出: 0
+解释: 数组元素个数小于 2,因此返回 0。
+
+说明:
+
+
+ - 你可以假设数组中所有元素都是非负整数,且数值在 32 位有符号整数范围内。
+ - 请尝试在线性时间复杂度和空间复杂度的条件下解决此问题。
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int maximumGap(vector &nums)
+ {
+ set st(nums.begin(), nums.end());
+ int res = 0, pre = INT_MIN;
+ for (auto iter = st.begin(); iter != st.end(); ++iter)
+ {
+ if (pre == INT_MIN)
+ {
+ pre = *iter;
+ continue;
+ }
+ else
+ {
+ res = max(res, *iter - pre);
+ pre = *iter;
+ }
+ }
+ return res;
+ }
+};
+
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..ea35684627ec034c6d3a4c201d11aaac9edc9791
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-296ab2fd88934525b5653ef7114a0358",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..d1413c7e036fe6ddc2001940debad0be87e94133
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "7e1ff136064f405ea80673bf66bfbbfa",
+ "author": "csdn.net",
+ "keywords": "数组,动态规划,矩阵",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..6bd57595b872430b008b4d5d1251554a30e6c352
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/52.exercises/solution.md"
@@ -0,0 +1,122 @@
+# 地下城游戏
+
+
+
+一些恶魔抓住了公主(P)并将她关在了地下城的右下角。地下城是由 M x N 个房间组成的二维网格。我们英勇的骑士(K)最初被安置在左上角的房间里,他必须穿过地下城并通过对抗恶魔来拯救公主。
+
+骑士的初始健康点数为一个正整数。如果他的健康点数在某一时刻降至 0 或以下,他会立即死亡。
+
+有些房间由恶魔守卫,因此骑士在进入这些房间时会失去健康点数(若房间里的值为负整数,则表示骑士将损失健康点数);其他房间要么是空的(房间里的值为 0),要么包含增加骑士健康点数的魔法球(若房间里的值为正整数,则表示骑士将增加健康点数)。
+
+为了尽快到达公主,骑士决定每次只向右或向下移动一步。
+
+
+
+编写一个函数来计算确保骑士能够拯救到公主所需的最低初始健康点数。
+
+例如,考虑到如下布局的地下城,如果骑士遵循最佳路径 右 -> 右 -> 下 -> 下
,则骑士的初始健康点数至少为 7。
+
+
+
+-2 (K) |
+-3 |
+3 |
+
+
+-5 |
+-10 |
+1 |
+
+
+10 |
+30 |
+-5 (P) |
+
+
+
+
+
+
+说明:
+
+
+ -
+
骑士的健康点数没有上限。
+
+ - 任何房间都可能对骑士的健康点数造成威胁,也可能增加骑士的健康点数,包括骑士进入的左上角房间以及公主被监禁的右下角房间。
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int calculateMinimumHP(vector> &dungeon)
+ {
+ int row = dungeon.size();
+ int col = dungeon[0].size();
+ int dp[row][col] = {0};
+ dp[row - 1][col - 1] = max(1 - dungeon[row - 1][col - 1], 1);
+ for (int i = row - 2; i >= 0; i--)
+ {
+ dp[i][col - 1] = max(dp[i + 1][col - 1] - dungeon[i][col - 1], 1);
+ }
+ for (int i = col - 2; i >= 0; i--)
+ {
+ dp[row - 1][i] = max(dp[row - 1][i + 1] - dungeon[row - 1][i], 1);
+ }
+ for (int i = row - 2; i >= 0; i--)
+ {
+ for (int j = col - 2; j >= 0; j--)
+ {
+ dp[i][j] = max(min(dp[i + 1][j], dp[i][j + 1]) - dungeon[i][j], 1);
+ }
+ }
+
+ return dp[0][0];
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..cdb36e93c09049c7e77107fad2f0e0f026d51b39
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-4aeca3661ce143d0bbfd73f6e72f11e9",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..30aed40d6167618a80f7ad947fff635b70d5a34a
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "72ab423ada734563aa9655cef722af73",
+ "author": "csdn.net",
+ "keywords": "数组,动态规划",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..9fb07084453735012ae91373c98730e9e29a63b7
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/53.exercises/solution.md"
@@ -0,0 +1,101 @@
+# 买卖股票的最佳时机 IV
+
+给定一个整数数组 prices
,它的第 i
个元素 prices[i]
是一支给定的股票在第 i
天的价格。
+
+设计一个算法来计算你所能获取的最大利润。你最多可以完成 k 笔交易。
+
+注意:你不能同时参与多笔交易(你必须在再次购买前出售掉之前的股票)。
+
+
+
+示例 1:
+
+
+输入:k = 2, prices = [2,4,1]
+输出:2
+解释:在第 1 天 (股票价格 = 2) 的时候买入,在第 2 天 (股票价格 = 4) 的时候卖出,这笔交易所能获得利润 = 4-2 = 2 。
+
+示例 2:
+
+
+输入:k = 2, prices = [3,2,6,5,0,3]
+输出:7
+解释:在第 2 天 (股票价格 = 2) 的时候买入,在第 3 天 (股票价格 = 6) 的时候卖出, 这笔交易所能获得利润 = 6-2 = 4 。
+ 随后,在第 5 天 (股票价格 = 0) 的时候买入,在第 6 天 (股票价格 = 3) 的时候卖出, 这笔交易所能获得利润 = 3-0 = 3 。
+
+
+
+提示:
+
+
+ 0 <= k <= 100
+ 0 <= prices.length <= 1000
+ 0 <= prices[i] <= 1000
+
+
+
+## template
+
+```cpp
+
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ int maxProfit(int k, vector &prices)
+ {
+ const int len = prices.size();
+ if (len <= 1 || k == 0)
+ return 0;
+ if (k > len / 2)
+ k = len / 2;
+ const int count = k;
+ int buy[count];
+ int sell[count];
+ for (int i = 0; i < count; ++i)
+ {
+ buy[i] = -prices[0];
+ sell[i] = 0;
+ }
+ for (int i = 1; i < len; ++i)
+ {
+ buy[0] = max(buy[0], -prices[i]);
+ sell[0] = max(sell[0], buy[0] + prices[i]);
+ for (int j = count - 1; j > 0; --j)
+ {
+ buy[j] = max(buy[j], sell[j - 1] - prices[i]);
+ sell[j] = max(buy[j] + prices[i], sell[j]);
+ }
+ }
+ return sell[count - 1];
+ }
+};
+```
+
+## 答案
+
+```cpp
+
+```
+
+## 选项
+
+### A
+
+```cpp
+
+```
+
+### B
+
+```cpp
+
+```
+
+### C
+
+```cpp
+
+```
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/config.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/config.json"
new file mode 100644
index 0000000000000000000000000000000000000000..b871df9624cfbde181d67b2aef7355d6d8b576d3
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/config.json"
@@ -0,0 +1,10 @@
+{
+ "node_id": "dailycode-ab7610050b14427bb38fe76c6e8710c5",
+ "keywords": [],
+ "children": [],
+ "keywords_must": [],
+ "keywords_forbid": [],
+ "export": [
+ "solution.json"
+ ]
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/solution.json" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/solution.json"
new file mode 100644
index 0000000000000000000000000000000000000000..7b05d736b8d2e3fc9cfbe53c4c7ff1b1f587cf11
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/solution.json"
@@ -0,0 +1,8 @@
+{
+ "type": "code_options",
+ "source": "solution.md",
+ "exercise_id": "25f9587f51f1491795478d8603b141c9",
+ "author": "csdn.net",
+ "keywords": "字典树,数组,字符串,回溯,矩阵",
+ "notebook_enable": true
+}
\ No newline at end of file
diff --git "a/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/solution.md" "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/solution.md"
new file mode 100644
index 0000000000000000000000000000000000000000..3b47be88bd12a40f8f38fe890ec1994c4bd74918
--- /dev/null
+++ "b/data/3.dailycode\351\253\230\351\230\266/1.cpp/54.exercises/solution.md"
@@ -0,0 +1,159 @@
+# 单词搜索 II
+
+给定一个 m x n
二维字符网格 board
和一个单词(字符串)列表 words
,找出所有同时在二维网格和字典中出现的单词。
+
+单词必须按照字母顺序,通过 相邻的单元格 内的字母构成,其中“相邻”单元格是那些水平相邻或垂直相邻的单元格。同一个单元格内的字母在一个单词中不允许被重复使用。
+
+
+
+示例 1:
+
+
+输入:board = [["o","a","a","n"],["e","t","a","e"],["i","h","k","r"],["i","f","l","v"]], words = ["oath","pea","eat","rain"]
+输出:["eat","oath"]
+
+
+示例 2:
+
+
+输入:board = [["a","b"],["c","d"]], words = ["abcb"]
+输出:[]
+
+
+
+
+提示:
+
+
+ m == board.length
+ n == board[i].length
+ 1 <= m, n <= 12
+ board[i][j]
是一个小写英文字母
+ 1 <= words.length <= 3 * 104
+ 1 <= words[i].length <= 10
+ words[i]
由小写英文字母组成
+ words
中的所有字符串互不相同
+
+
+
+## template
+
+```cpp
+#include
+using namespace std;
+
+class Solution
+{
+public:
+ struct Node
+ {
+ bool isflag = false;
+ Node *next[27] = {};
+ };
+ set res;
+ vector ans;
+ Node *root;
+ vector dirx{0, 0, 1, -1};
+ vector diry{1, -1, 0, 0};
+ bool flag;
+
+ void createtree(vector &words)
+ {
+ root = new Node();
+
+ for (auto w : words)
+ {
+ Node *p = root;
+ for (int i = 0; i < w.length(); i++)
+ {
+ if (p->next[w[i] - 'a'] == NULL)
+ {
+ p->next[w[i] - 'a'] = new Node();
+ }
+ p = p->next[w[i] - 'a'];
+ }
+ p->isflag = true;
+ }
+ }
+ void backtrack(vector> &board, vector> visited, int row, int col, Node *roott, string cur)
+ {
+ cur += board[row][col];
+ roott = roott->next[board[row][col] - 'a'];
+ if (!roott)
+ return;
+ if (roott->isflag == true)
+ {
+
+ res.insert(cur);
+ flag = true;
+ }
+ visited[row][col] = true;
+
+ for (int i = 0; i < 4; i++)
+ {
+
+ int nx = row + dirx[i];
+ int ny = col + diry[i];
+ if (nx < 0 || ny < 0 || nx >= board.size() || ny >= board[0].size())
+ continue;
+ if (visited[nx][ny] == false)
+ {
+ backtrack(board, visited, nx, ny, roott, cur);
+ }
+ }
+ }
+ vector findWords(vector> &board, vector &words)
+ {
+
+ if (board.size() == 0 || words.size() == 0)
+ return ans;
+ createtree(words);
+
+ for (int i = 0; i < board.size(); i++)
+ {
+ for (int j = 0; j < board[i].size(); j++)
+ {
+ Node *p = root;
+ flag = false;
+ if (p->next[board[i][j] - 'a'])
+ {
+ vector> visited{board.size(), vector(board[0].size(), false)};
+ backtrack(board, visited, i, j, p, "");
+ }
+ }
+ }
+ set