# 反转链表 II
给你单链表的头指针 head
和两个整数 left
和 right
,其中 left <= right
。请你反转从位置 left
到位置 right
的链表节点,返回 反转后的链表 。
示例 1:
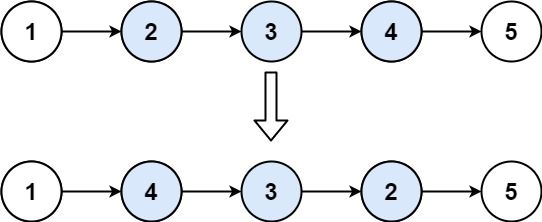
输入:head = [1,2,3,4,5], left = 2, right = 4
输出:[1,4,3,2,5]
示例 2:
输入:head = [5], left = 1, right = 1
输出:[5]
提示:
- 链表中节点数目为
n
1 <= n <= 500
-500 <= Node.val <= 500
1 <= left <= right <= n
进阶: 你可以使用一趟扫描完成反转吗?
以下错误的选项是?
## aop
### before
```cpp
#include
using namespace std;
struct ListNode
{
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
```
### after
```cpp
```
## 答案
```cpp
class Solution
{
public:
ListNode *reverseBetween(ListNode *head, int m, int n)
{
ListNode *dummy = new ListNode(-1), *pre = dummy;
dummy->next = head;
for (int i = 0; i < m; ++i)
pre = pre->next;
ListNode *cur = pre->next;
for (int i = m; i < n - 1; ++i)
{
ListNode *t = cur->next;
cur->next = t->next;
t->next = pre->next;
pre->next = t;
}
return dummy->next;
}
};
```
## 选项
### A
```cpp
class Solution
{
public:
ListNode *reverseBetween(ListNode *head, int m, int n)
{
if (head == nullptr || head->next == nullptr || m == n)
return head;
ListNode *pre = nullptr, *cur = head;
while (m > 1)
{
pre = cur;
cur = cur->next;
m--;
n--;
}
ListNode *tail = cur, *con = pre;
ListNode *third;
while (n > 0)
{
third = cur->next;
cur->next = pre;
pre = cur;
cur = third;
n--;
}
if (con)
{
con->next = pre;
}
else
{
head = pre;
}
tail->next = cur;
return head;
}
};
```
### B
```cpp
class Solution
{
public:
ListNode *reverseBetween(ListNode *head, int m, int n)
{
if (head == NULL || m == n)
return head;
ListNode *pm = head, *pn = head;
ListNode *dummy = new ListNode(-1);
dummy->next = head;
ListNode *pm0 = dummy;
while (m-- > 1)
pm = pm->next, pm0 = pm0->next;
while (n-- > 1)
pn = pn->next;
ListNode *pPre = pm, *pCur = pPre->next, *pn2 = pn->next;
while (pCur != pn2)
{
if (pCur)
{
ListNode *pNext = pCur->next;
pCur->next = pPre;
pPre = pCur;
pCur = pNext;
}
}
pm0->next = pn;
pm->next = pn2;
return dummy->next;
}
};
```
### C
```cpp
class Solution
{
public:
ListNode *reverseBetween(ListNode *head, int m, int n)
{
if ((head == NULL) || (head->next == NULL))
return head;
ListNode *first = new ListNode(0);
first->next = head;
int cnt = 1;
for (int i = 0; i < m - 1; ++i)
{
first = first->next;
cnt++;
}
ListNode *old_pre = first;
ListNode *cur = first->next;
ListNode *cur_next = cur->next;
ListNode *new_pre = cur;
ListNode *temp;
/*反转*/
while ((cur != NULL) && (cnt < n))
{
temp = cur_next->next;
cur_next->next = cur;
cur = cur_next;
cur_next = temp;
cnt++;
}
old_pre->next = cur;
new_pre->next = cur_next;
if (m == 1)
return cur;
else
return head;
}
};
```