Add with-chakra-ui-typescript example (#16561)
This PR adds an example Next.js project with chakra-ui as its component library using TypeScript. 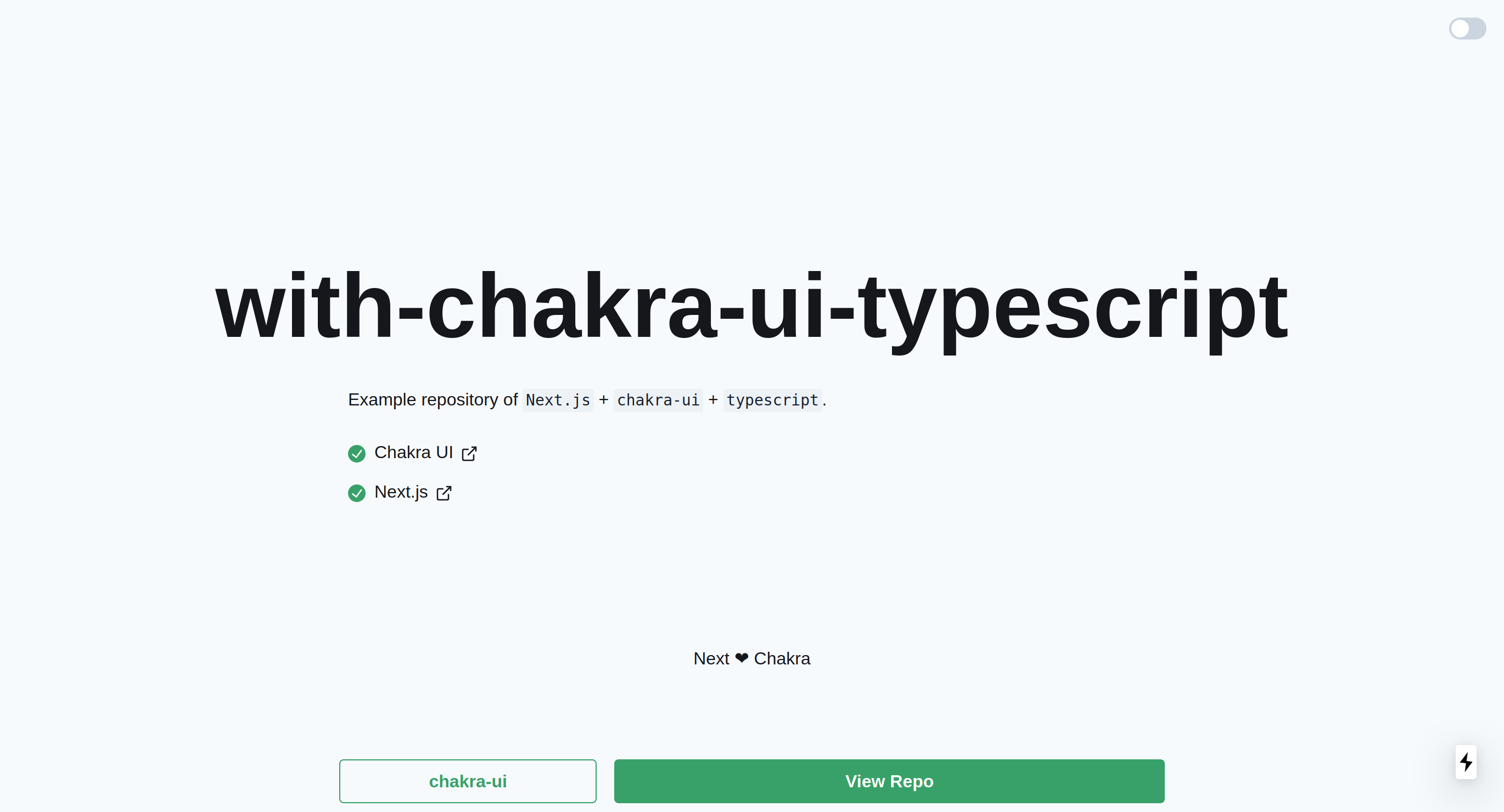 Both libraries has built-in TypeScript declarations. Will help to encourage typed projects, so why not? It's a combination of two existing examples [with-chakra-ui](https://github.com/vercel/next.js/tree/canary/examples/with-chakra-ui) and [with-typescript](https://github.com/vercel/next.js/tree/canary/examples/with-typescript). All credits for their contributors.
Showing
想要评论请 注册 或 登录